The `git pull --hard` command is not a standard Git command, but if you aim to forcefully update your local branch with changes from the remote repository while discarding all local changes, you should first reset your branch and then pull, like this:
git fetch origin && git reset --hard origin/main
Replace `main` with your desired branch name.
Understanding `git pull`
Before diving into the specifics of `git pull --hard`, it's essential to understand the `git pull` command itself. The `git pull` command is one of the most frequently used commands for synchronizing local repositories with remote ones. Essentially, it fetches the latest changes from a remote repository and merges them into your current branch. This allows developers to stay updated with the changes made by other team members or maintainers.
However, `git pull` does more than just fetch; it brings local branches up to date with their remote counterparts. It’s crucial to differentiate `git pull` from `git fetch`. While `git fetch` only downloads changes without altering your working directory or current branch, `git pull` integrates those changes at the same time.
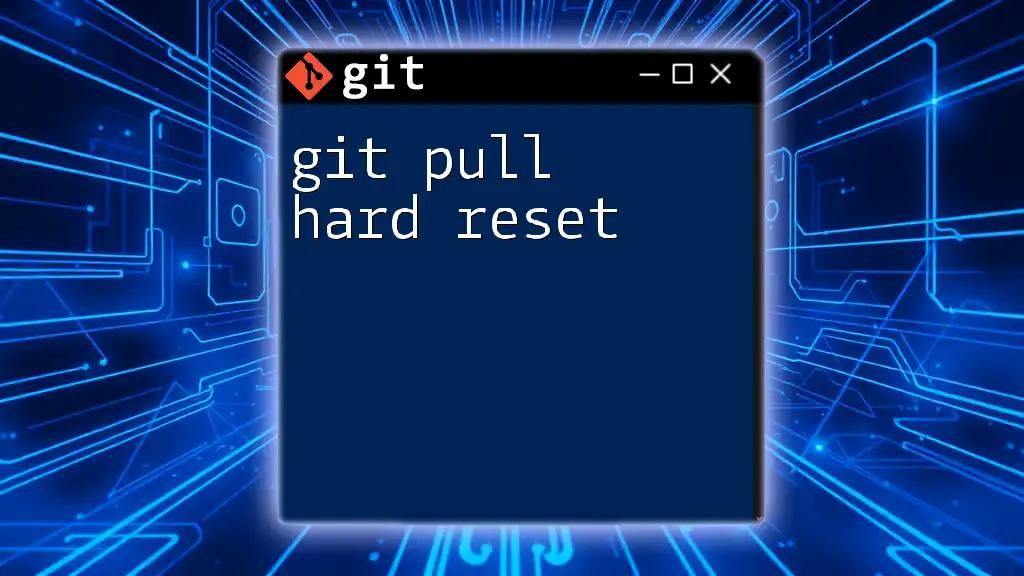
Overview of `git pull --hard`
The `git pull --hard` command is a specific option that performs a more drastic operation than the standard `git pull`. First, it's important to clarify the purpose of the `--hard` flag.
When you execute `git pull --hard`, you are essentially instructing Git to discard any local changes you have made. This feature is particularly useful in situations where your local branch has diverged significantly from its remote version, and you wish to completely reset it and synchronize.
The Impact of `--hard`
The `--hard` flag is pivotal because it omits all local modifications. Here’s what happens when you run this command:
- Overwriting Local Changes: Any uncommitted changes in your working directory will be lost permanently when you use `--hard`. This means if you have modifications that are not saved in a commit, running this command will erase them completely.
- Working Directory and Index Reset: The command resets both your working directory and the index. Your local branch will match the state of the remote branch you're pulling from, discarding any differences.
When to Use `git pull --hard`
Using `git pull --hard` should be approached with caution. It is best utilized in scenarios where:
- You are sure that your local changes are unnecessary: This could be when you're experimenting with some code or debugging and decide that starting fresh is the best route.
- There are significant discrepancies between your branch and the remote: For example, during the merging process, if conflicts arise that you don't want to deal with, a hard pull can save time.
However, this command comes with significant risks. If you have local changes that you value but forget to back them up or commit them, you will lose them permanently. Therefore, always ensure you have either committed or stashed your work before executing a hard pull.
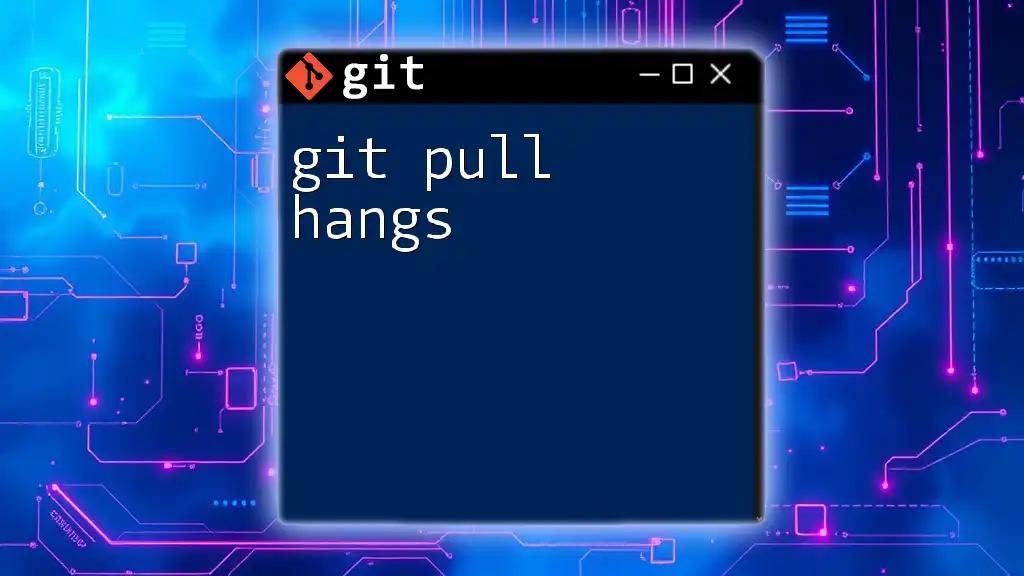
Practical Examples
Example 1: Basic `git pull --hard`
To perform a basic hard pull, you would generally use the command:
git pull origin main --hard
This command attempts to pull changes from the remote `main` branch, forcing an overwrite of your local changes without preserving any work you have done.
Example 2: Discarding Local Changes
A more nuanced approach might involve checking your current changes before pulling:
git checkout -- .
git pull origin development --hard
Here, the `git checkout -- .` command first reverts all changes made in the working directory (similar to a soft reset). This allows you to ensure that there are no lingering changes before the pull, which guarantees a clean slate.
Example 3: Combining Commands
Sometimes, you may want to have extra control over the pull process. You can achieve this by separating the fetching and resetting commands:
git fetch origin
git reset --hard origin/main
In this example, you're fetching updates from the remote repository first, which allows you to see the latest changes without affecting your local files. The `reset --hard` command then sets your current branch to match the state of the remote `main`, discarding any local changes.
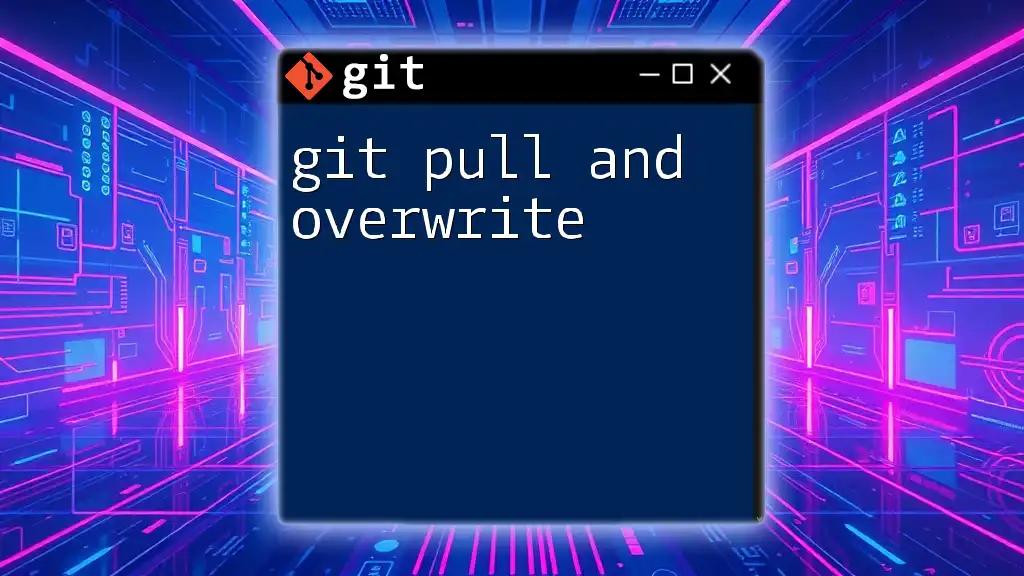
Best Practices for Using `git pull --hard`
Backup Before You Pull
Always consider backing up your work before a hard pull. This could mean creating a temporary branch to store current changes, which can be done as follows:
git checkout -b backup-branch
This command creates a new branch called `backup-branch`, allowing you to save your work before performing more destructive commands.
Alternative Commands to Consider
There are often better alternatives that could achieve similar results without the risks associated with `--hard`.
- Using `git stash`: Instead of discarding changes, you can stash your changes first:
git stash
git pull origin main
git stash pop
This procedure saves your uncommitted changes temporarily so you can pull updates without losing your work.
- Using `git checkout` to switch branches: If you’re working across multiple branches, switching might be a better option. You can use:
git checkout another-branch
This command allows you to conveniently handle different sets of changes without sacrificing your local modifications.
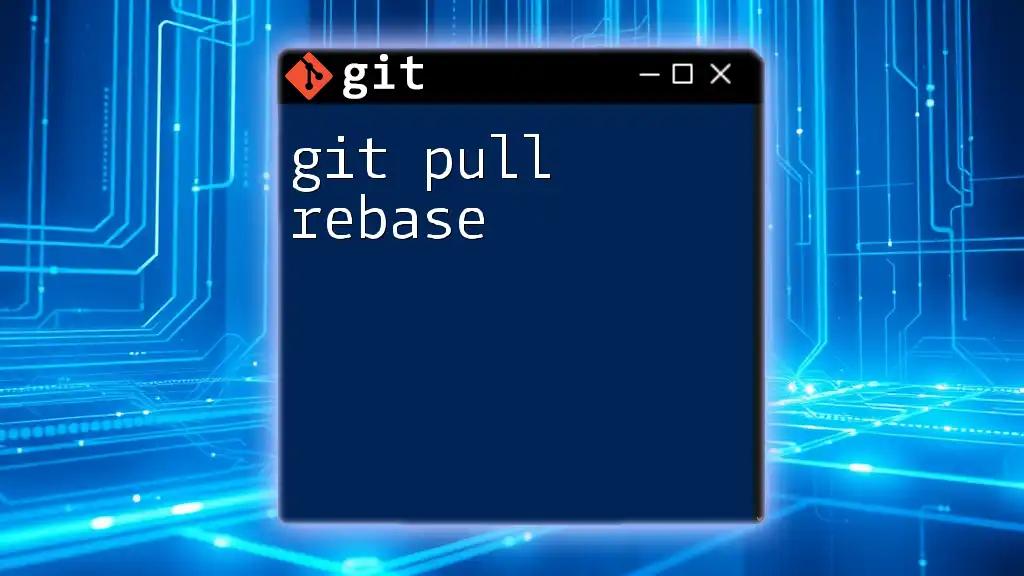
Common Issues and Troubleshooting
Problems You May Encounter
Using `git pull --hard` can lead to some issues if not managed correctly. One common problem is encountering untracked files that may hinder a clean pull from the remote repository. Your working directory can also accumulate conflicts if a soft reset was not done prior.
How to Resolve Issues
If you find yourself facing untracked files that prevent you from pulling, consider this command to clean your working directory:
git clean -fd
This command will remove untracked files and directories, ensuring a tidy environment before your pull.
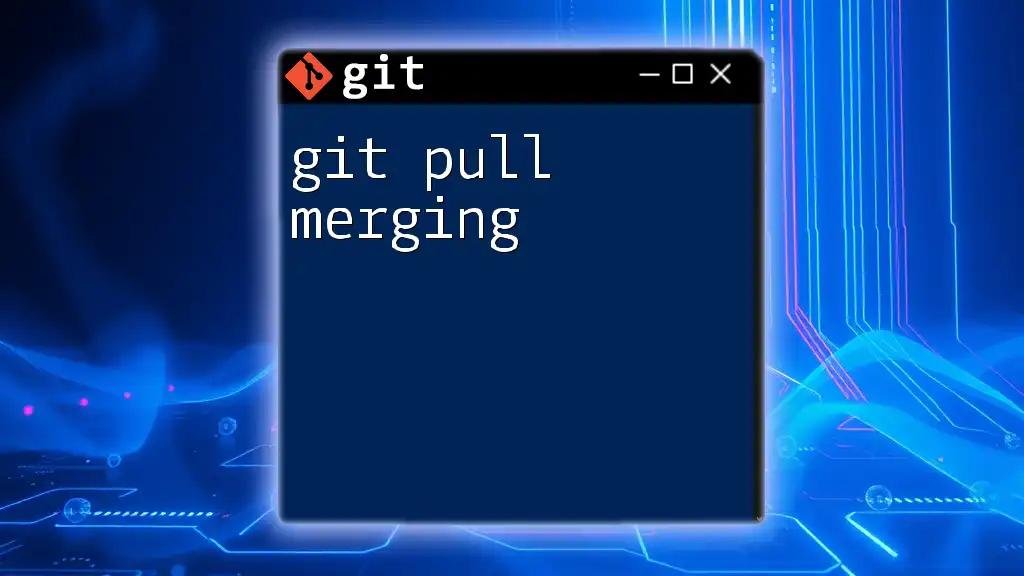
Conclusion
In summary, the `git pull --hard` command is a powerful tool within the Git environment that allows you to synchronize your local branches with remote counterparts explicitly. However, it is inherently dangerous due to its ability to overwrite and delete local changes permanently.
Understanding when and how to use `git pull --hard`, practicing safe backups, and being aware of alternatives can significantly enhance your efficiency as a developer. Always remember to master Git commands thoroughly before using them to avoid unnecessary losses or conflicts.