To upload your local commits to a remote GitHub repository, use the `git push` command followed by the name of the remote and the branch you want to push to. Here's how you can do it:
git push origin main
Understanding Git Push
What is Git Push?
The `git push` command is a fundamental part of using Git and GitHub, serving as a mechanism to transfer commits from a local repository to a remote repository. When you make local changes and want to share them with others or save them in a central location, you use `git push`. This command updates the remote repository with your committed changes, allowing collaborators to access the latest version of your project.
Key Concepts
To effectively understand how `git push` works, it’s essential to grasp a few foundational concepts in Git.
-
Local Repository vs. Remote Repository: The local repository is situated on your machine, whereas the remote repository, like those hosted on GitHub, is accessible over the internet. When you want your local changes to be visible to others, you push them from your local repository to the remote repository on GitHub.
-
Tracking branches and upstream branches: By default, Git branches are tracked locally. An upstream branch represents the remote counterpart of your local branch, allowing you to push and pull changes easily.
-
The role of commits and the commit history: Each time you make a commit in Git, you are saving a snapshot of your changes. This history is what you are pushing to GitHub when you execute the `git push` command.
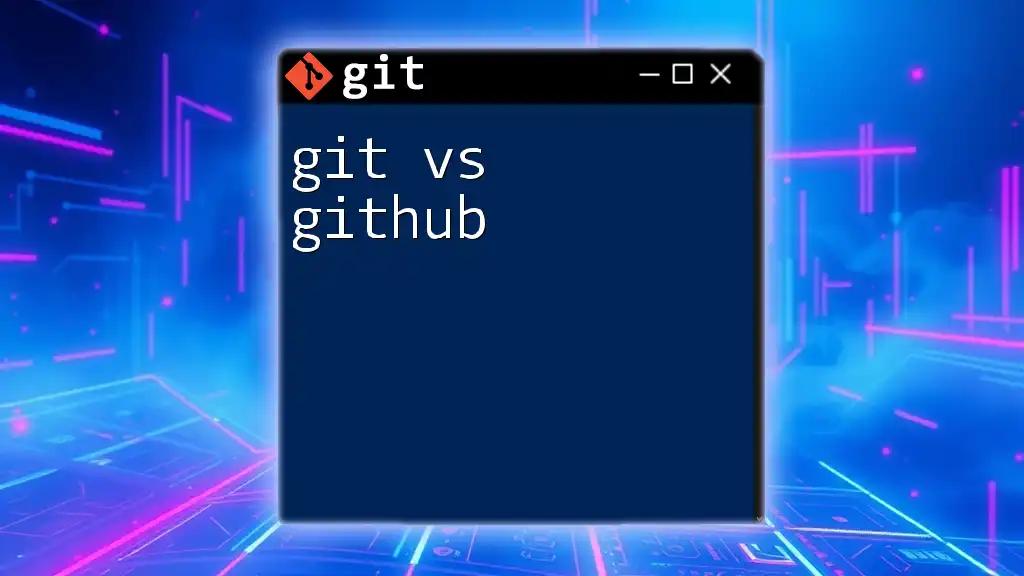
Preparing to Push to GitHub
Setting up a GitHub Repository
Before you can push to GitHub, you need to create a repository in your GitHub account. Follow these steps to set it up:
- Log in to your GitHub account.
- Click the New button on the repositories page.
- Fill out necessary details such as the repository name, description, and visibility setting (public or private).
- Click Create repository to finalize the setup.
Local Repository Setup
Next, you’ll want to initialize your local repository and link it to the newly created GitHub repository.
To initialize your repository, open your terminal and run the following commands:
git init my-repo
cd my-repo
After your local repository is set up, you need to connect it to your GitHub repository with this command:
git remote add origin https://github.com/username/my-repo.git
Make sure to replace `username` and `my-repo` with your actual GitHub username and repository name.
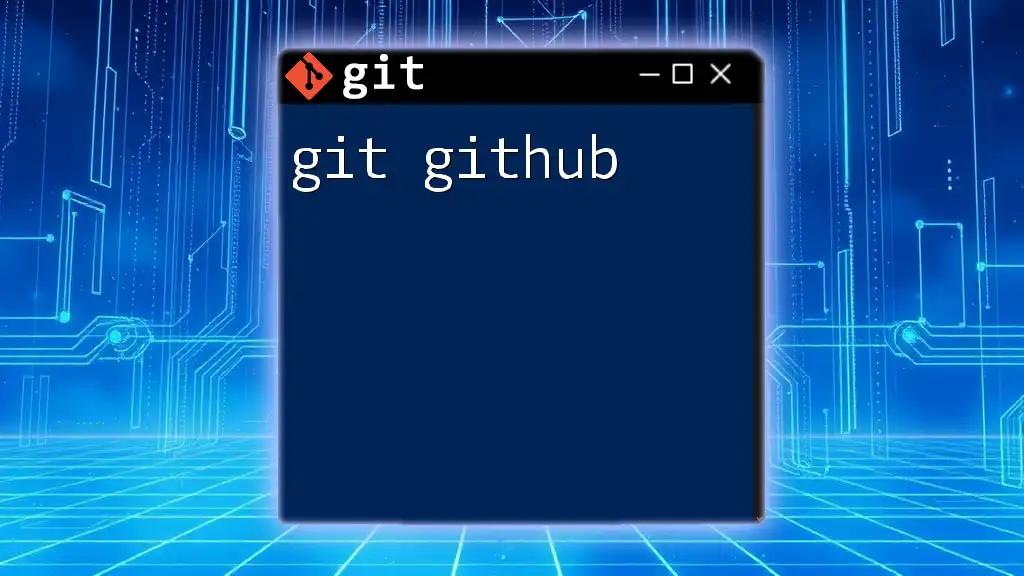
Executing git push
Syntax of git push Command
The basic syntax of the `git push` command is straightforward:
git push [remote-name] [branch-name]
- remote-name: Typically, this is named `origin` by default, representing the remote repository.
- branch-name: This specifies the branch you want to push changes to (e.g., `main` or `develop`).
Pushing Changes to GitHub
Committing Changes Locally
Before you can push anything to GitHub, you must commit your changes locally. Committing involves staging your changes and writing a meaningful commit message.
Here’s how you can stage and commit changes:
git add .
git commit -m "Your commit message"
The dot (`.`) in `git add .` signifies that you want to add all modified files to staging.
Executing the Push Command
Now that your changes are committed, you can execute the push command to send them to GitHub:
git push origin main
Here, `origin` refers to your remote repository and `main` refers to the branch you want to update. After executing this command, you should see output indicating the successful push of your commits to GitHub.
Verifying the Push
It’s crucial to verify that your changes have been pushed successfully. Visit your GitHub repository in the browser to check that the updates appear as expected. The commit history should now include your recent changes, and the files will be updated in the repository.
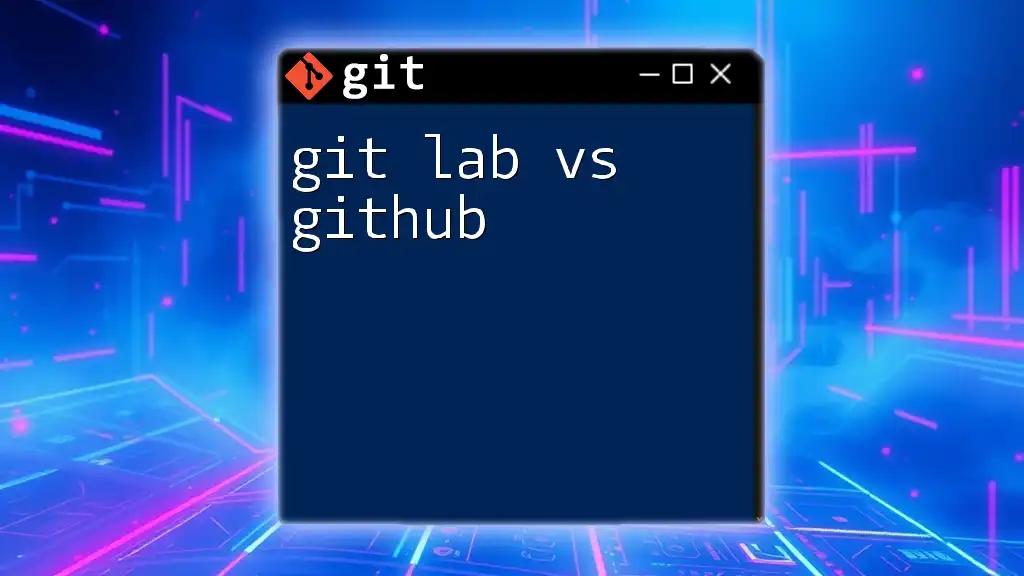
Common Issues and Solutions
Permission Errors
Often, you might encounter permission errors when trying to push. Common examples include authentication issues tied to SSH keys or HTTPS credentials. To resolve permission issues, you may need to generate and add SSH keys to your GitHub account.
To generate SSH keys, use the following command:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
After generating the SSH key, copy the key to your GitHub account’s SSH settings.
Conflict Resolution
Conflicts can occur when your local repository diverges from the remote repository. To avoid these issues, always pull the latest changes from GitHub before pushing:
git pull origin main
If there are merge conflicts, Git will alert you, and you’ll have to resolve these conflicts before you can push your changes.
The Importance of Syncing with Remote
To prevent conflicts, it’s a best practice to regularly synchronize your local repository with the remote repository before you start working and before pushing changes.
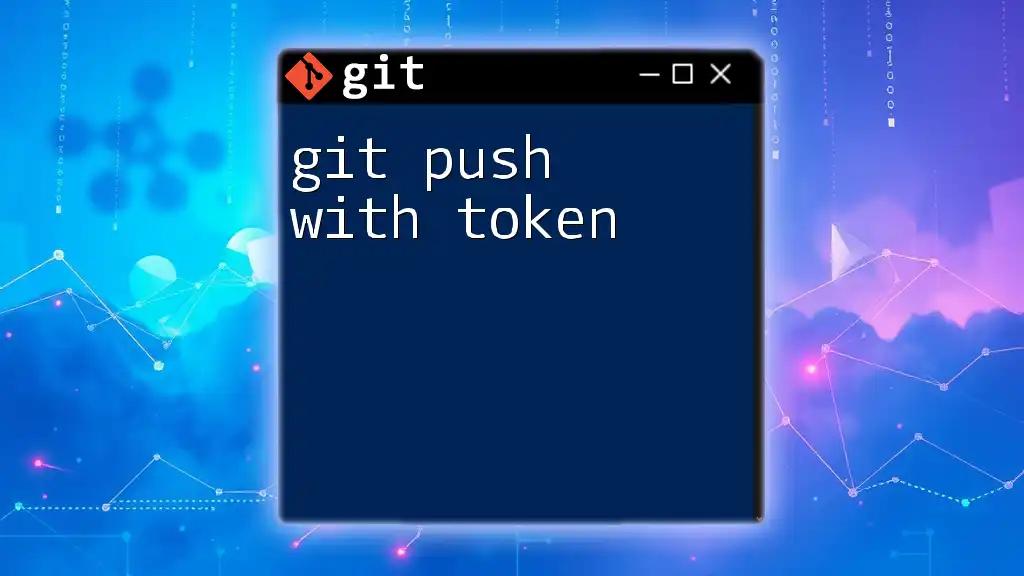
Advanced Push Features
Pushing Tags
Git tags are useful for marking specific points in your commit history, such as release versions. To push a tag to GitHub, simply create the tag and push it:
git tag v1.0
git push origin v1.0
This command allows you to push a specific tag corresponding to a given version.
Pushing to Other Branches
You might need to push changes to different branches as part of your workflow. To do this, specify the branch name in your push command:
git push origin feature-branch
This command uploads your changes to the specified feature branch on GitHub.
Force Push (with caution)
In some cases, it may be necessary to use a force push, especially if you need to overwrite changes on the remote repository. This command is powerful and should be used cautiously:
git push --force origin main
Warning: Force pushing can overwrite commits in the remote repository, potentially causing others to lose their work. Always ensure you comprehensively communicate with your team before executing a force push.
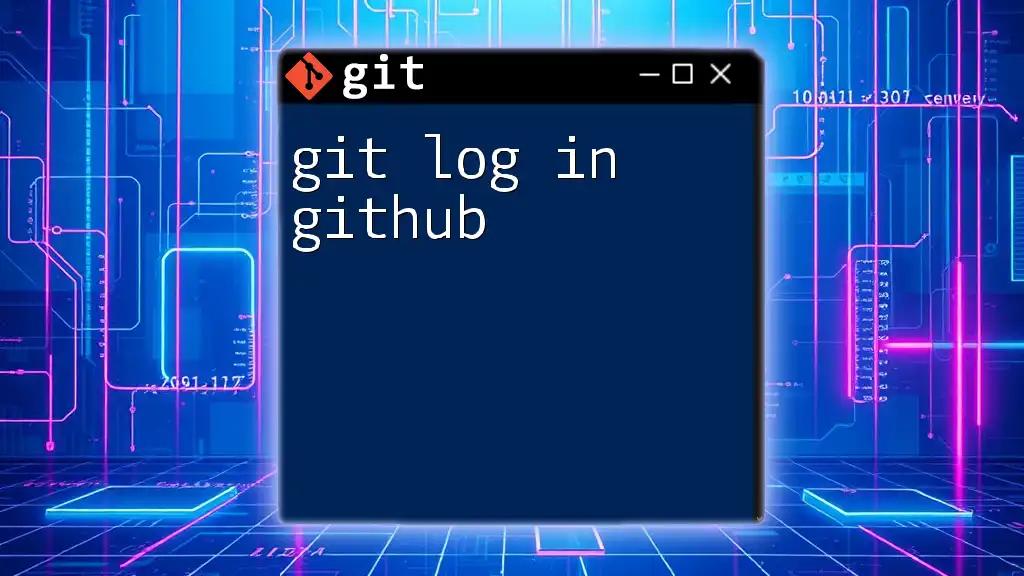
Conclusion
The `git push to GitHub` command is an essential skill for anyone working with Git and version control. It allows you to share your progress with others, maintain a backup of your work, and collaborate effectively. By following the steps outlined in this guide, you can become proficient in pushing changes to GitHub and handling common issues as they arise.
Practice by creating your repositories and pushing changes. The more you use Git, the more comfortable you will become. For further learning, consider exploring additional Git commands and workflows to enhance your version control skills.
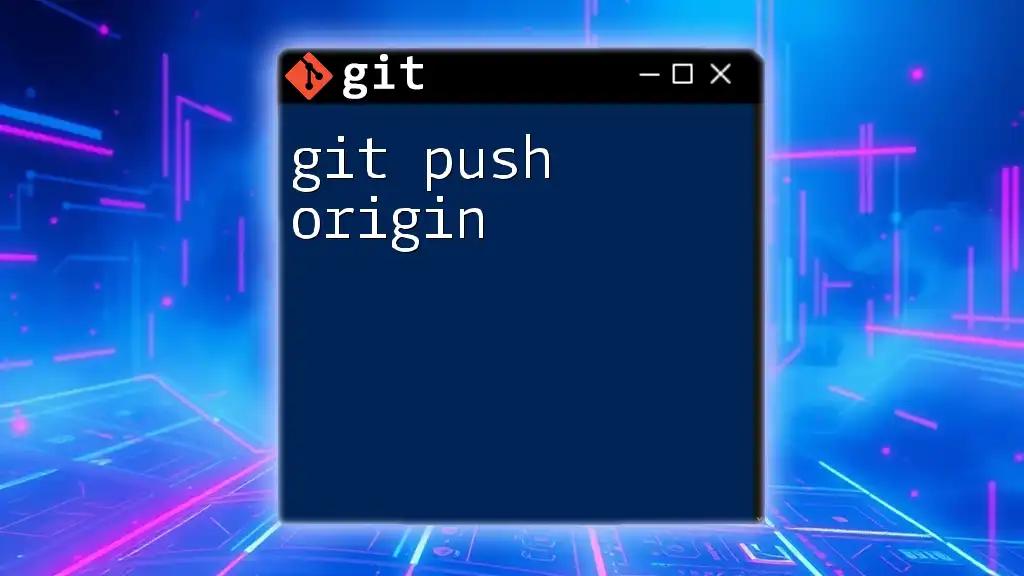
Additional Resources
For a deeper dive into Git and GitHub, explore the official documentation, online courses, and community support forums to stay updated with best practices and advanced features.