Git is a version control system that allows multiple developers to collaborate on projects, while GitHub is a platform that hosts Git repositories and provides tools for managing and sharing code.
Here's a simple example of how to clone a GitHub repository using Git:
git clone https://github.com/username/repository.git
Understanding Git
What is Git?
Git is a distributed version control system that allows developers to track changes in their code, collaborate with others, and manage various versions of their projects seamlessly. It was created by Linus Torvalds in 2005 for the development of the Linux kernel and has since become a cornerstone of modern software development.
Key features of Git include its ability to work offline, full history tracking, and powerful branching capabilities. Unlike traditional version control systems that use a central server, Git enables every user to have a local copy of the entire repository, which enhances collaboration and provides greater flexibility.
Basic Git Concepts
Repository
A repository (or repo) is a container for your project's files and their change history. It can exist either locally on your machine or remotely on platforms such as GitHub.
- Local Repository: Contains your own personal copy of the project files and history.
- Remote Repository: A central repository where changes can be shared with other collaborators.
You can create a new local repository with the command:
git init
Commit
A commit is essentially a snapshot of your project at a given point in time. Every commit in Git is accompanied by a unique hash, along with a message that describes the changes made.
The structure of a commit includes the following elements:
- Hash: A unique identifier for the commit.
- Message: A brief description of what changes were made.
- Author: The person who made the changes.
- Date: When the changes were made.
To create a commit, you can use the following command:
git commit -m "Your commit message here"
It's vital to write clear, descriptive commit messages to facilitate understanding of the project's history.
Branching
Branching is a powerful feature in Git that allows you to create separate lines of development. This means you can work on new features or bug fixes without affecting the main codebase.
Creating a new branch is simple:
git branch new-feature
When you finish your work on the new feature, you can merge it back into the main branch, maintaining a clean and organized project structure.
Working with Repositories
Cloning a Repository
Cloning a repository allows you to create a local copy of an existing repository. This is especially useful when you want to contribute to a project hosted on a platform like GitHub.
To clone a Git repository, use:
git clone https://github.com/user/repo.git
This command downloads all the project files, history, and branches, making it easy to start contributing immediately.
Staging and Unstaging
The staging area (also known as the index) is where you prepare changes before committing them to your repository.
To stage a file, use:
git add filename.txt
To unstage a file, you can use:
git reset filename.txt
It is important to carefully manage your staging area to ensure that only the intended changes are committed.
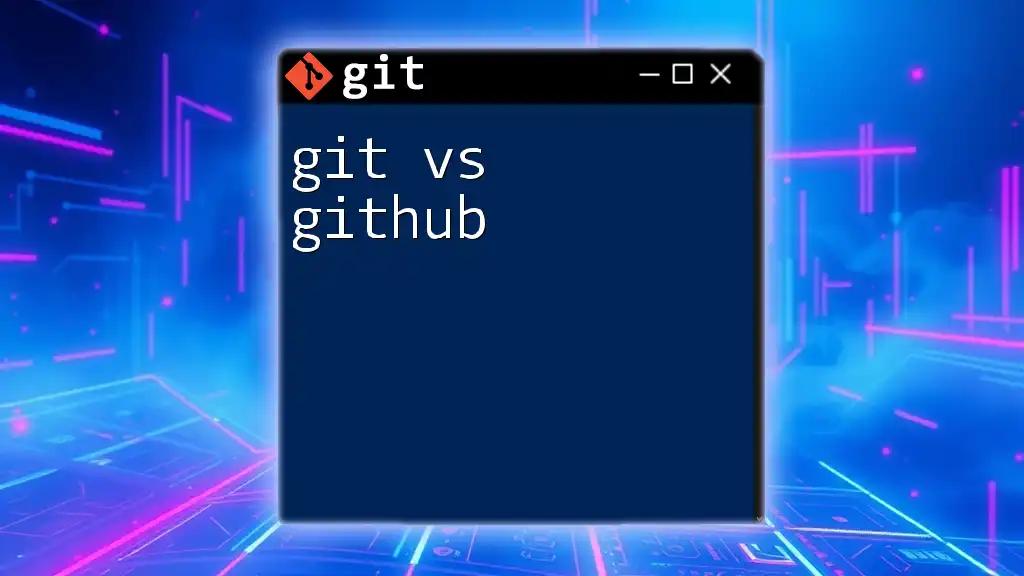
Setting Up GitHub
What is GitHub?
GitHub is a web-based platform that uses Git for version control and is widely used for hosting, sharing, and collaborating on projects. It provides additional features like issue tracking, pull requests, and comprehensive third-party integrations.
GitHub allows developers to collaborate efficiently, manage project workflows, and share open-source projects with a global community.
Creating a GitHub Account
To get started with GitHub, you first need to create an account. Visit the GitHub website and follow these steps:
- Click on the Sign Up button.
- Enter your email address, create a password, and choose a username.
- Follow the verification process (email confirmation) to activate your account.
- Once your account is created, take a moment to set up your profile with relevant information about your skills and projects.
Creating a New Repository on GitHub
Creating a new repository on GitHub is straightforward:
- After logging in, click the New button in your GitHub dashboard.
- Choose a meaningful name for your repository.
- Set the repository to Public or Private according to your needs.
- Optionally, initialize with a README file and select a .gitignore template to exclude certain files from your repository.
This simple process lays the groundwork for your project on GitHub, enabling efficient collaboration and version control.
Linking Local Repository with GitHub
To connect your local Git repository to your GitHub repository, you will need to set the remote origin. This links your local repository's changes to the repository on GitHub.
Run the following command from your local repository:
git remote add origin https://github.com/user/repo.git
This step allows you to push your changes directly to GitHub.
Pushing Local Changes to GitHub
Pushing your changes makes them available on GitHub. When you are ready to share your work, use the command below:
git push origin main
This command uploads your committed changes from the local `main` branch to the remote repository on GitHub.
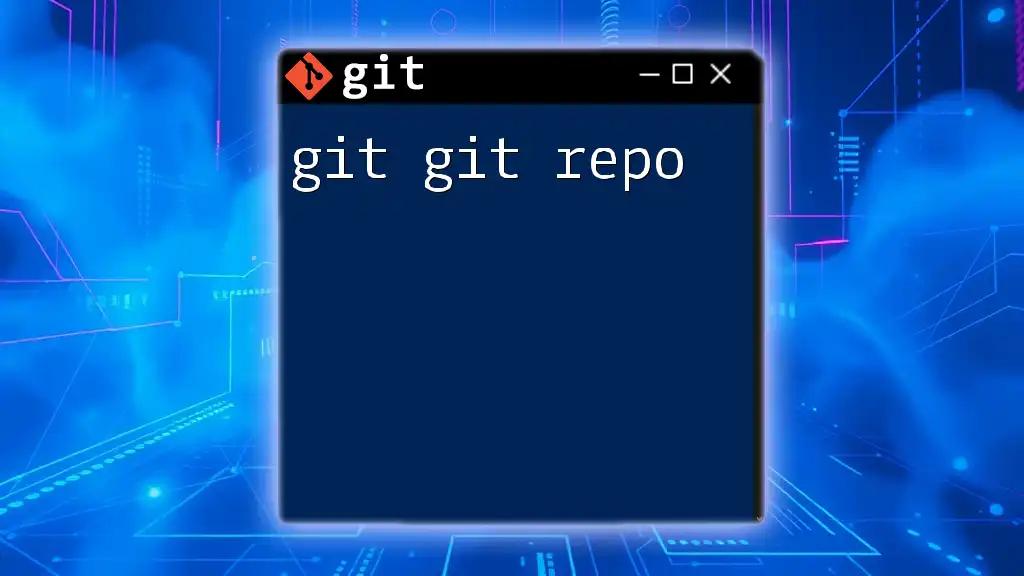
Collaboration with GitHub
Forking a Repository
Forking a repository allows you to create your own copy of someone else's project on GitHub. This is a common way to contribute to open-source projects.
To fork a repository, click the Fork button at the top of the repo page. Once you have forked a repo, you can clone it to your local machine using:
git clone https://github.com/your-username/forked-repo.git
This enables you to make changes without affecting the original project.
Pull Requests
A pull request (PR) is a way of proposing changes to a project when you've made your modifications in a forked repo. It's an essential feature of GitHub that facilitates collaboration.
To create a pull request:
- Push your changes to your forked repo.
- Go to the original repository on GitHub.
- Click on the Pull Requests tab.
- Click on New Pull Request and select the branch you want to merge.
When submitting a PR, provide a clear explanation of your changes and any relevant information for reviewers.
Merging and Resolving Conflicts
When working with multiple collaborators, you may encounter merge conflicts. These happen when two or more contributors make changes to the same line in a file or when one contributor deletes a file another is trying to modify.
To merge a branch into the main branch, use:
git merge feature-branch
If there are conflicts, Git will notify you and mark the conflicted files. To resolve conflicts, edit the affected files, removing conflict markers, and then stage the resolved files using:
git add conflicted-file.txt
git commit
This ensures a clean merge without losing any crucial changes.
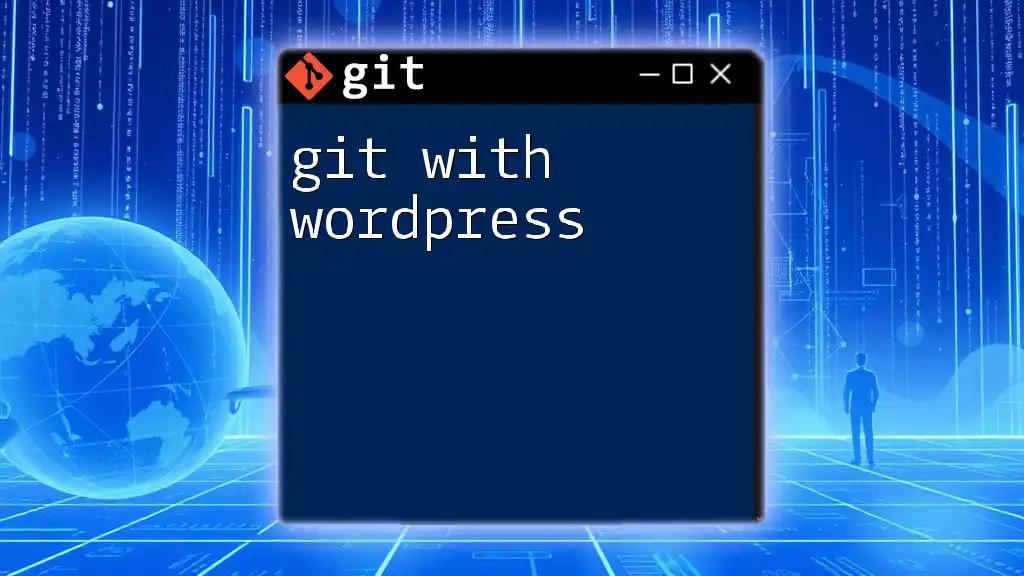
Advanced Git Commands
Rebasing
Rebasing is an alternative to merging, allowing you to apply your changes on top of another branch’s commits. This results in a cleaner project history.
To rebase your changes onto another branch, use:
git rebase main
This command rewrites commit history, so it should be used with care, particularly in shared repositories.
Advanced Branching Strategies
There are several branching strategies to help manage your workflow effectively. Two popular strategies are:
- Git Flow: Focused on releases with defined branches for features, releases, and hotfixes.
- GitHub Flow: Simpler, allowing for a single main branch with short-lived feature branches.
Choosing the right strategy can help structure your development process and streamline collaboration.
Tagging Releases
Tagging is a way to mark specific points in your project’s history as significant, often used for releases.
To create a tag, run:
git tag -a v1.0 -m "Version 1.0"
git push origin --tags
Tags help you reference particular versions easily, making it simpler to track changes over time.
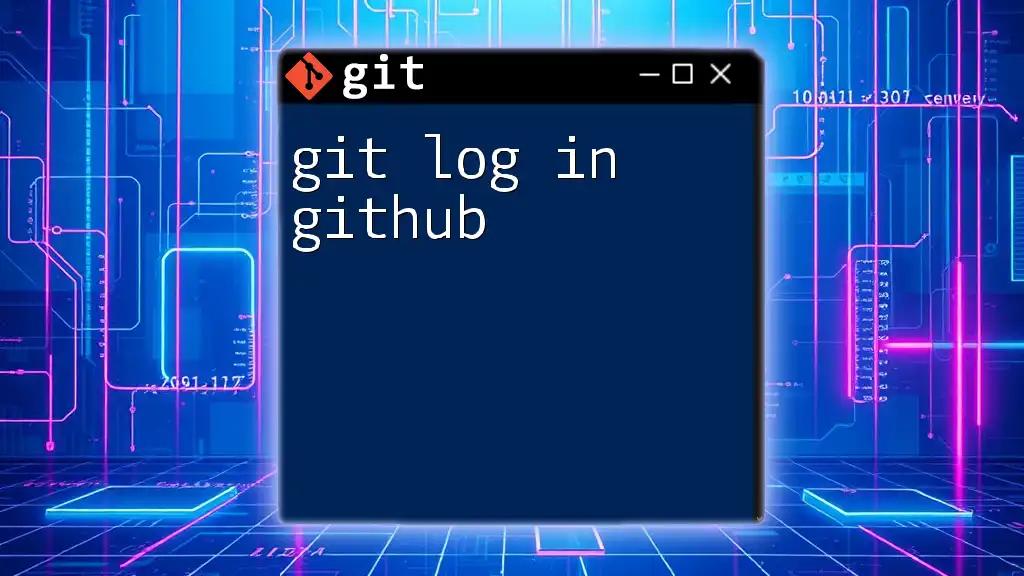
Conclusion
Mastering Git and GitHub is essential for any developer today. With a good understanding of these tools, you can effectively manage your code, collaborate with others, and contribute to projects on a global scale.
Regular practice and exploration of more advanced features will greatly enhance your skills. Remember, the journey of learning Git and GitHub is ongoing and filled with opportunities for growth and collaboration. Join our workshops and courses to deepen your knowledge and expertise!
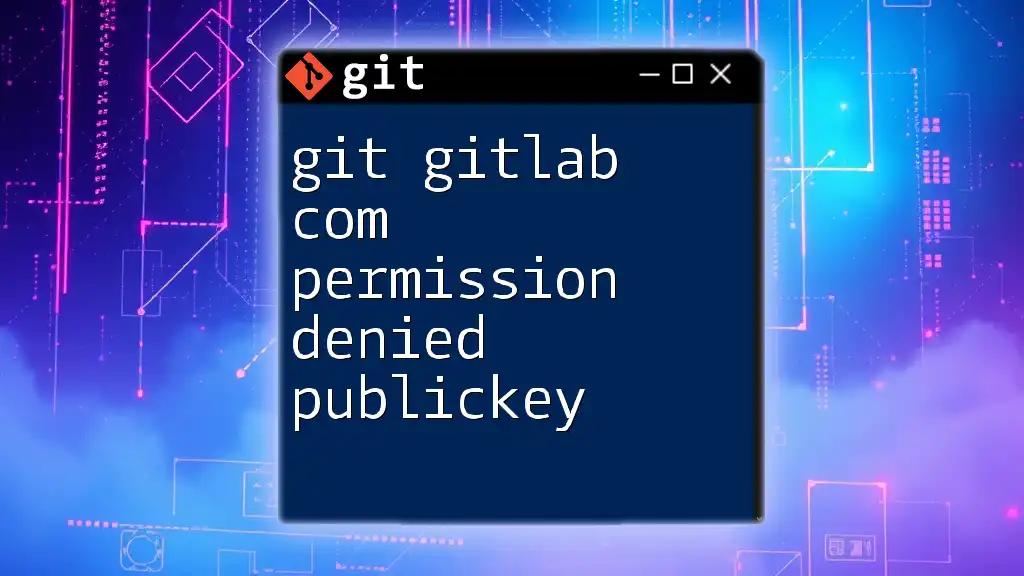
Additional Resources
For more in-depth learning, check out the official [Git](https://git-scm.com/doc) and [GitHub](https://docs.github.com/en) documentation, along with recommended books and tutorials that will further enhance your understanding of these powerful tools.