A Git repository (repo) is a storage space where your project's files and the entire revision history of your project are kept, allowing for version control and collaboration.
Here's a code snippet to create a new git repository:
git init my-repo
Understanding Git Repositories
What is a Git Repository?
A Git repository is a storage space where your project files and the entire history of changes made to those files are kept. It allows developers to efficiently manage and track their work.
There are two main types of repositories: Local and Remote.
- Local Repositories reside on your machine. This is where you work on your files, make changes, and commit those changes.
- Remote Repositories are hosted on the internet, such as on platforms like GitHub or GitLab. These act as a central hub for collaboration, allowing multiple users to access the same project from different locations.
Why Use a Git Repository?
Utilizing a Git repository comes with numerous benefits.
- Version Control: It maintains a history of changes, enabling you to revert to previous versions of your files easily. This offers peace of mind when experimenting with new code.
- Collaboration: Git allows multiple developers to work together on the same project simultaneously without conflicts. This is crucial in team environments.
- Backup: By storing your code in a remote repository, you protect it from hardware failures or accidental deletions. Your work is securely backed up in the cloud.
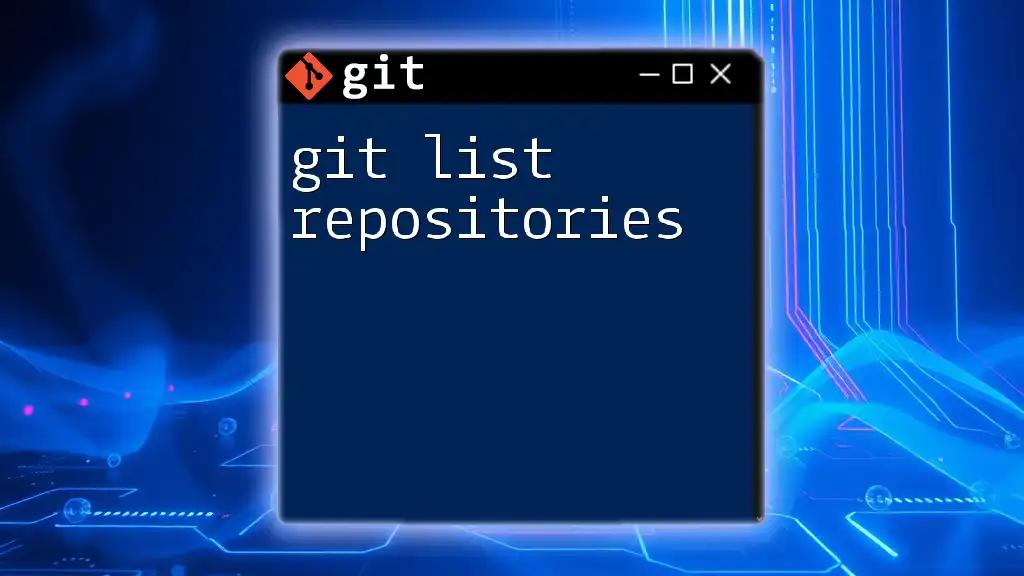
Setting Up Your Git Repository
Initializing a New Git Repository
To start using Git for a new project, you need to create a new directory for your files and initialize it as a Git repository.
First, create a new directory:
mkdir my-project
cd my-project
Next, initialize the Git repository by running:
git init
This command sets up the `.git` directory, making it ready for Git to start tracking your files.
Cloning an Existing Repository
If you want to work on an existing repository, you can easily clone it using the following command:
git clone https://github.com/user/repo.git
This command downloads the entire repository and creates a new directory with the same name as the repository, allowing you to start working locally.
Inspecting Your Repository
To see the status of your files within the repository, you can use:
git status
This command provides useful information about which files are staged for commit, which are unstaged, and any untracked files present in the directory.
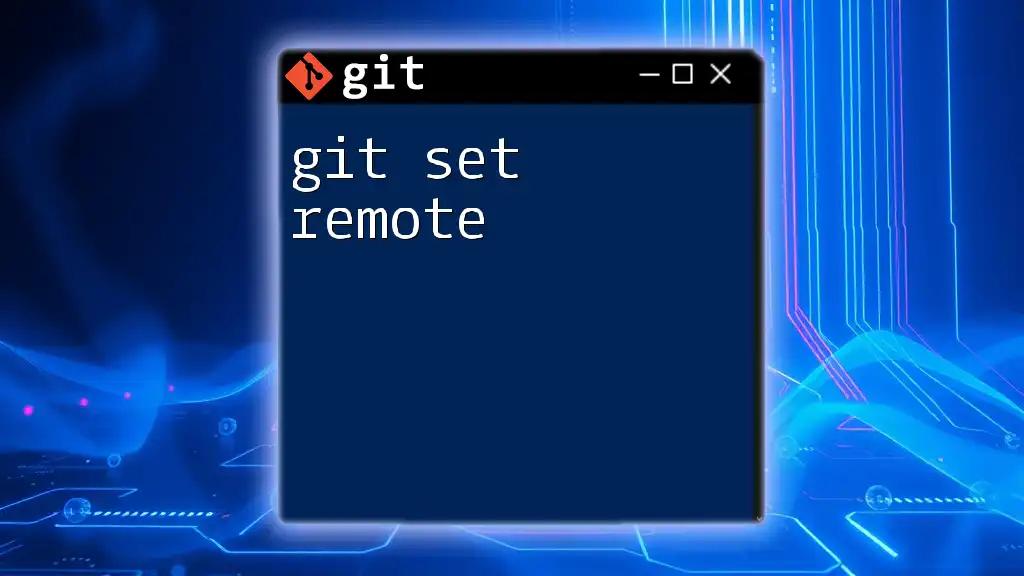
Managing Files in Your Git Repository
Staging Changes
Once you’ve made changes to your files, you need to stage them before committing. To add a specific file, use:
git add filename
If you want to stage all modified and untracked files, you can use:
git add .
Staging prepares your changes for the next commit, allowing you to control what you want to include.
Committing Changes
After staging your changes, it’s time to create a commit, which is a snapshot of your repository at that moment. Use the following command:
git commit -m "Your commit message here"
It’s important to write clear and concise commit messages as they help provide context for your changes, making it easier to understand the project history.
Viewing Commit History
To see a history of your commits, run:
git log
This command displays a list of commits, including commit hashes, authors, dates, and messages, giving you insight into the evolution of your project.
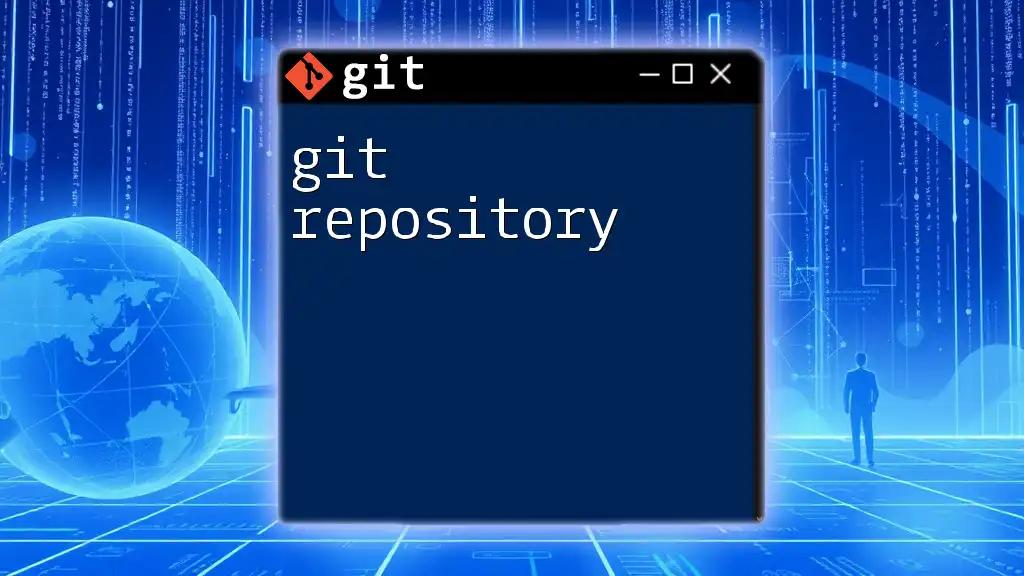
Managing Remote Repositories
Adding a Remote Repository
Once you've initialized your local repository, you may want to link it to a remote repository. Use the command:
git remote add origin https://github.com/user/repo.git
By adding a remote, you can easily push and pull changes to and from that repository.
Pushing Changes to Remote
After committing your changes locally, you'll want to send them to the remote repository. To push your commits, use:
git push origin main
This command uploads your changes to the `main` branch of the remote repository, making them available to others.
Pulling Changes from Remote
To keep your local repository updated with changes made in the remote repository, you can use:
git pull origin main
This command fetches and merges updates from the remote repository into your local branch.
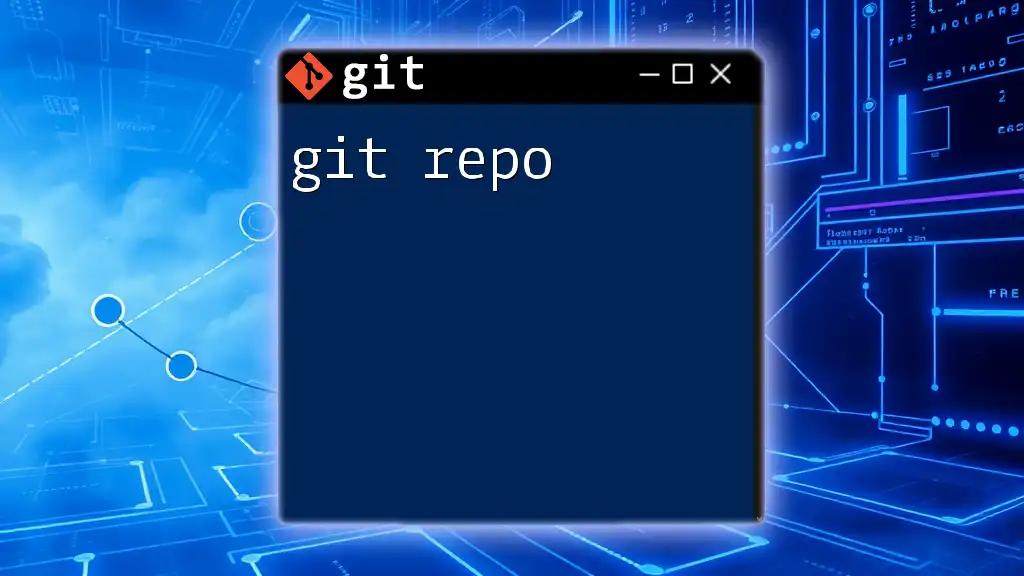
Best Practices for Managing Git Repositories
Structuring Your Repository
A well-organized repository helps maintain clarity. Keep files in logical directories according to their purposes. Moreover, including a `README.md` file at the root can provide important information about the project, such as setup instructions, usage, and contribution guidelines.
Branching Strategies
Using branches effectively allows you to manage developments and features separately from the main codebase. To create a new branch for a feature or bug fix, use:
git checkout -b feature-branch
Once your work is complete, you can merge it back into the main branch by switching to the main branch and running:
git checkout main
git merge feature-branch
This approach keeps the main branch stable while you continue development on separate branches.
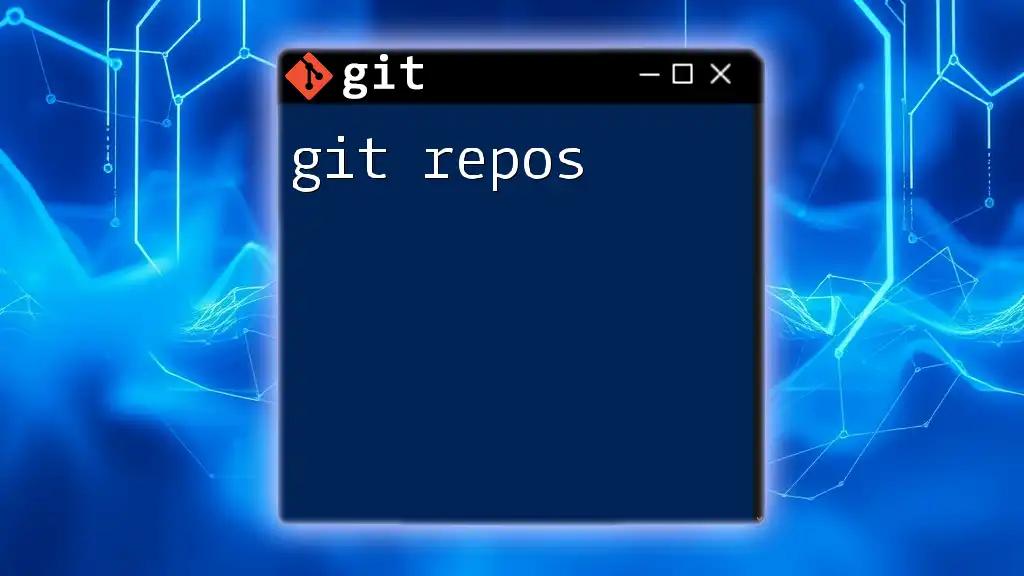
Troubleshooting Common Issues
Resolving Merge Conflicts
A merge conflict occurs when two branches have competing changes to the same part of a file. To resolve this, you'll need to open the affected files and edit them manually. After resolving conflicts, mark the files as resolved:
git add resolved-file
Undoing Changes
Should you realize you want to discard changes made to a file, you can revert it back to its last committed state with:
git checkout -- filename
If you need to undo the last commit completely, you can reset your branch with:
git reset --hard HEAD~1
However, be cautious with this command, as it will erase uncommitted changes.
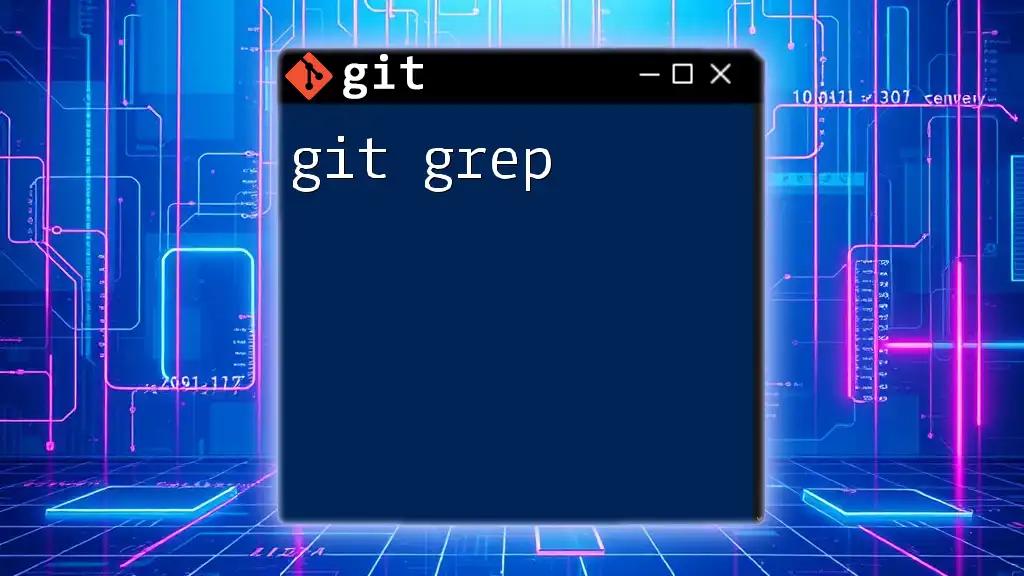
Conclusion
In this comprehensive guide, we've explored the essentials of git git repo. From setting up your repositories to managing files and collaborating with others, understanding these concepts is crucial for efficient version control. Use best practices to structure your repositories and manage branches, and be prepared to troubleshoot common issues as you progress in your development journey.
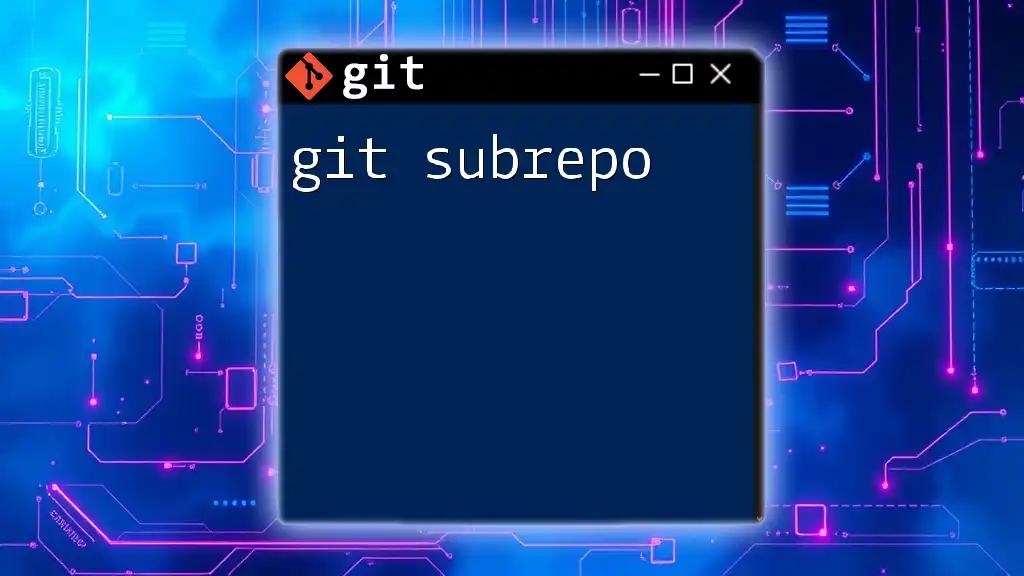
Additional Resources
For further reading, explore tutorials and official documentation, along with active Git communities where you can ask questions and share knowledge. These resources will help deepen your understanding and mastery of Git.