A Git repository (repo) is a storage space where your project files and the complete version history of your code are maintained, allowing you to track changes and collaborate efficiently.
Here’s a code snippet demonstrating how to create a new Git repository:
git init my-repo
Understanding Git Repositories
What is a Git Repository?
A Git repository (often referred to as a repo) is a centralized place where project files and their histories are stored using Git, a powerful version control system. It allows developers to track changes, collaborate on projects, and manage various versions of their files effortlessly.
There are two primary types of Git repositories: local and remote.
- Local repositories are instances of Git stored on your computer. They contain all project files as well as the history of all changes made.
- Remote repositories are hosted on servers or cloud platforms like GitHub, GitLab, or Bitbucket. They make it easy to collaborate with other developers by allowing them access to the project's files.
Types of Git Repositories
Local Repositories
Local repositories serve as your personal workspace where you can create, modify, and save files. To initiate a local repository, navigate to your desired project directory and run:
git init
This command creates a `.git` directory in your project folder, signifying that it's now a Git repository. You can start adding files and committing changes immediately.
Remote Repositories
Remote repositories function as the shared version of your projects hosted online. They allow multiple users to collaborate on the same project from different locations. After you set up a local repository, you can link it to a remote repository using:
git remote add origin <repository-url>
Here, `<repository-url>` would be the URL of the remote repo you want to link to. This connection is crucial for sharing your work and collaborating effectively with others.
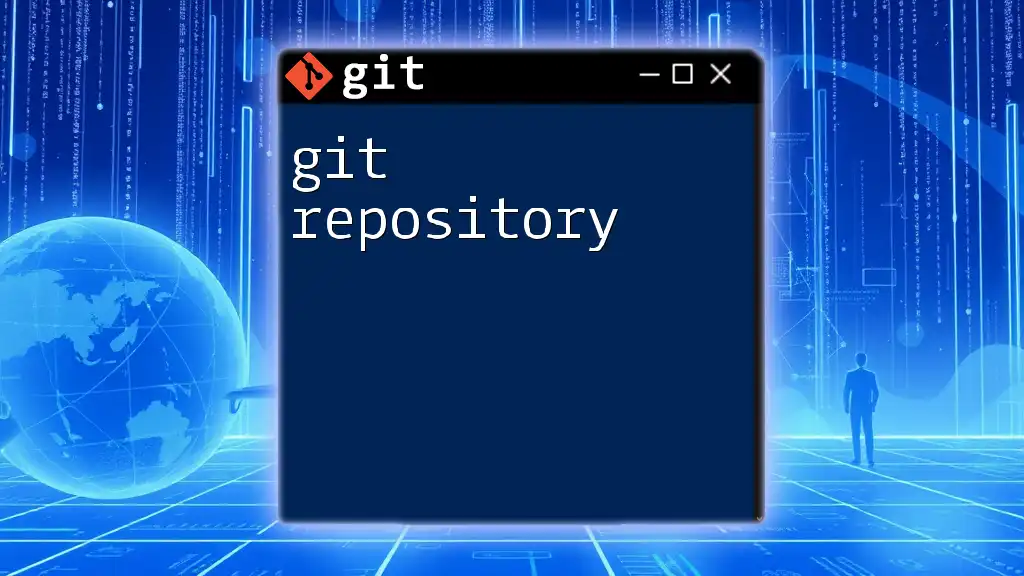
Working with Git Repositories
Cloning a Repository
To clone a repository means to create a copy of an existing remote repository on your local machine. This is a common practice when you want to contribute to an open-source project or collaborate with a team. To clone a repository, use the command:
git clone <repository-url>
For example, if you want to clone a public repository from GitHub, you would run the above command with the appropriate URL. This command not only downloads the files but also sets up the connection to the remote repository, enabling easy pushes and pulls in the future.
Creating a New Repository
To create a new repository from scratch, navigate to the desired directory and run:
git init
This initializes a new Git repository, allowing you to start adding files and tracking changes. It's essential to maintain a clear and organized directory structure to make collaboration and file management easier.
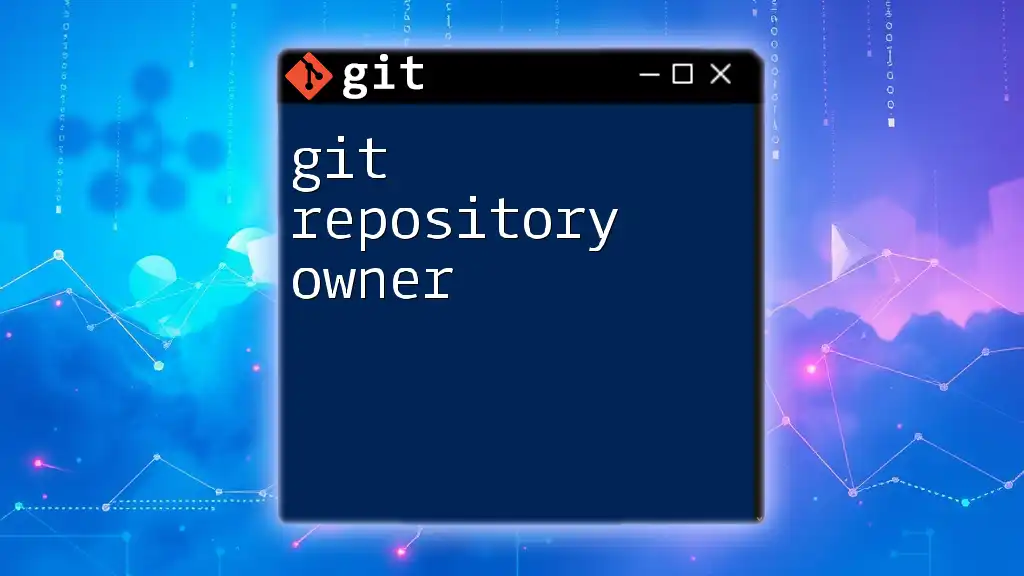
Managing Git Repositories
Adding Files to a Repository
After creating or cloning a repository, the next step is to add files. When you add files to a Git repository, they go through a two-step process: staging and committing.
To add a file to the staging area, use:
git add <file-name>
To add all modified files, you can run:
git add .
Staging your files prepares them to be committed to the repository, allowing you to review your changes before making them permanent.
Committing Changes
Once files are staged, the next step is to commit your changes. A commit represents a snapshot of your project at a particular moment in time. To commit changes, execute:
git commit -m "Your commit message"
It's crucial to write a meaningful commit message that describes the changes made. For instance, instead of a generic message like “Updated files,” you could write “Fixed bug in authentication logic.”
Pushing Changes to a Remote Repository
After making commits in your local repository, you may want to share these changes with others. To do this, you'll need to push your changes to a remote repository. Use the command:
git push origin main
Make sure to replace `main` with your active branch name if it differs. This command uploads your local commits to the specified remote repository. Be aware that pushing too frequently with unclear messages can clutter the project’s history.
Pulling Changes from a Remote Repository
To stay updated with others' changes, you’ll need to pull changes from the remote repository periodically. Use the following command:
git pull origin main
By executing this, Git fetches changes from the specified branch on the remote repository and merges them into your current branch. Understanding the difference between `git pull` and `git fetch` is also vital; while `git pull` fetches and merges in one step, `git fetch` only retrieves updates without merging them.
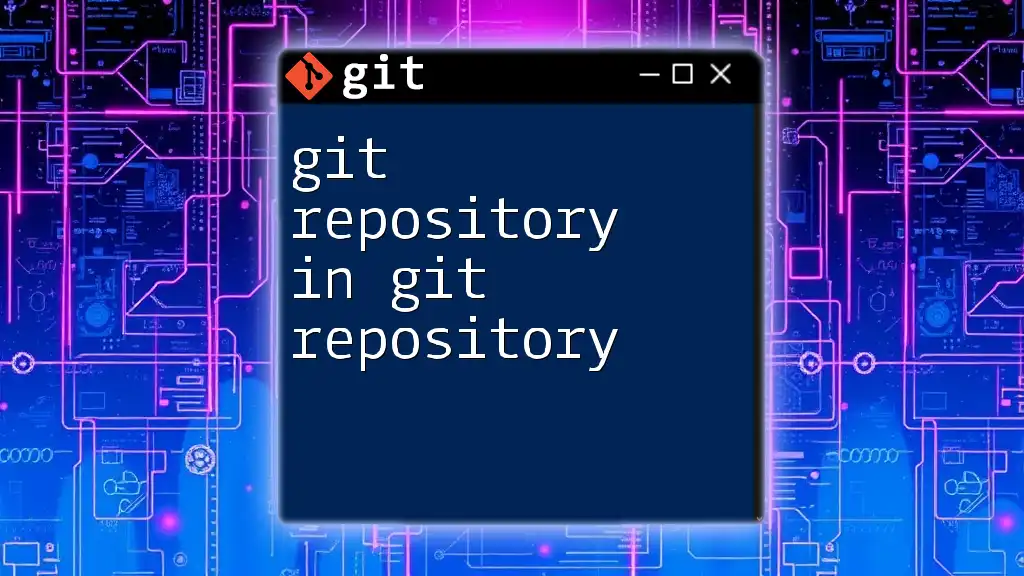
Working with Branches
Introduction to Branches
Branches in Git act as parallel versions of your project. Using branches, you can work on different features or bug fixes without affecting the main codebase. This separation allows multiple people to work on a project simultaneously without conflict.
Creating and Switching Branches
Creating a branch is easy with Git. You can create a new branch with:
git branch <branch-name>
To switch to that branch, use:
git checkout <branch-name>
You can also create and switch in a single command by combining them in this way:
git checkout -b <branch-name>
This is particularly useful in feature development or experimentation.
Merging Branches
Once your branch work is complete, it's time to merge these changes back into your main branch (often called `main` or `master`). First, switch to the branch you want to merge into:
git checkout main
Then, use the merge command:
git merge <branch-name>
If there are conflicting changes between the branches, Git will notify you. It's essential to resolve any conflicts manually before completing the merge.
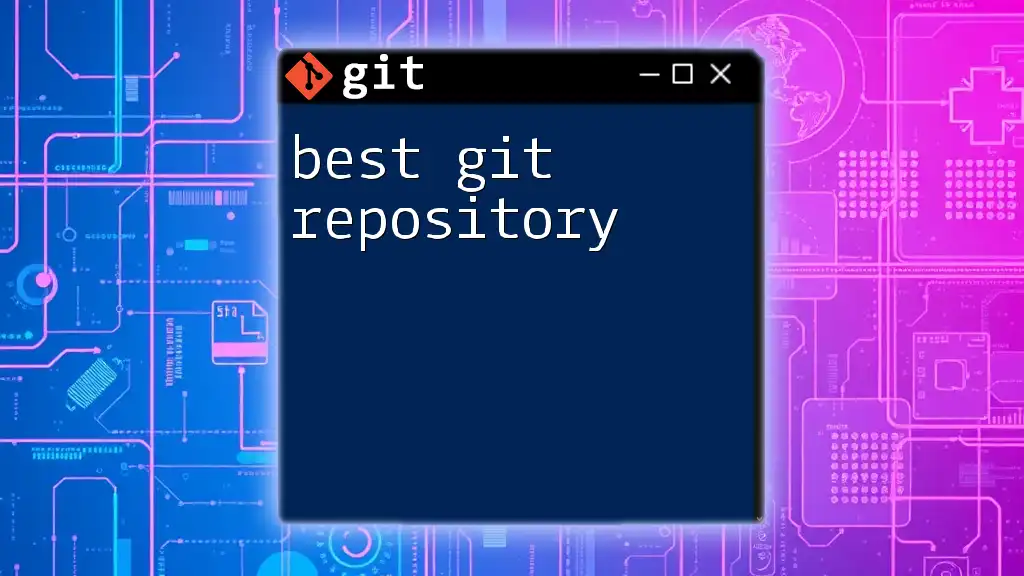
Best Practices for Git Repositories
Organizing Your Repository
Maintaining an organized structure within your Git repository is crucial for team collaboration and project manageability. Ensure that your repository has clear directories for different aspects of your project, such as `src/` for source files, `docs/` for documentation, and `tests/` for testing files.
Creating a README file that explains the project and a .gitignore file to exclude unnecessary files (such as compiled binaries or dependency files) from being tracked can significantly enhance project clarity.
Commit Frequency and Messaging
The frequency of your commits can greatly affect project clarity. Committing often helps document the development process, making it easier for teams to understand changes. Each commit should encapsulate a logical unit of work.
When crafting commit messages:
- Use the imperative mood (e.g., "Fix bug" instead of "Fixed bug").
- Be concise yet descriptive.
- Reference any issues addressed by the commit if applicable.
Collaboration Tips
Collaborating on a single Git repository requires clear communication and organization. Use features like issue trackers and pull requests to manage changes effectively. Always perform code reviews where possible to maintain quality and share knowledge within the team.
By following these practices, you’ll ensure a smoother collaborative workflow, making it easier to tackle complex projects and fostering a healthier development environment.
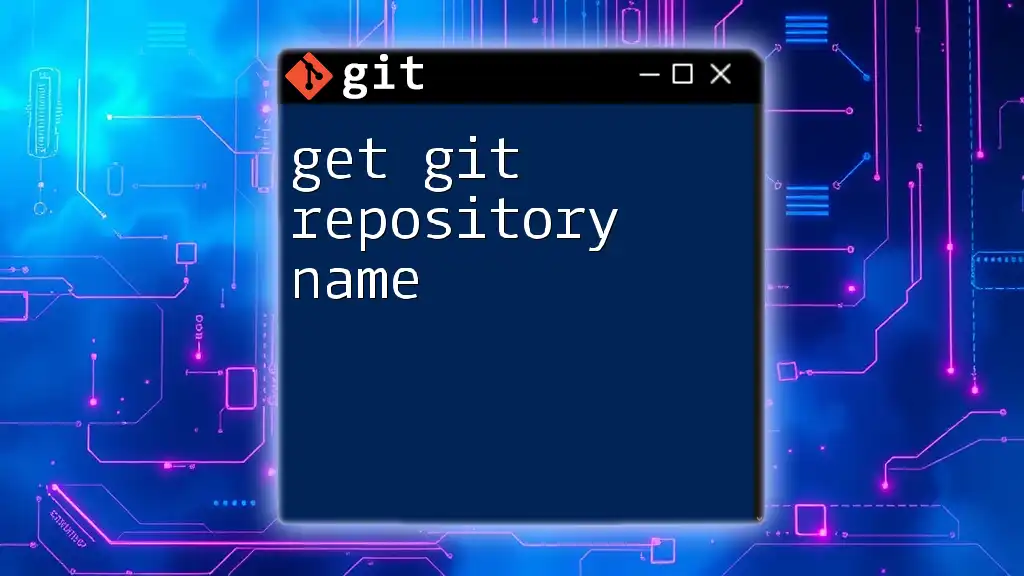
Conclusion
Git repositories serve as the backbone of modern software development, making it easier to track, manage, and collaborate on projects. By mastering the intricacies of git repos, such as creating them, managing branches, and maintaining clarity through effective commit messaging, you lay the groundwork for a successful coding journey. Remember, the more you practice using these Git commands, the more proficient you will become in your development skills.