To retrieve the name of the current Git repository, navigate to the repository’s root directory in your terminal and use the following command:
git rev-parse --basename --show-cdup
What is a Git Repository?
A Git repository is a storage space where your project and its history are kept. It enables you to manage different versions of your project over time. There are two basic types of repositories: local and remote.
- A local repository exists on your own computer and allows you to make changes offline. You can use Git commands to track your changes, commit them, and later push them to a remote repository.
- A remote repository, often hosted on platforms like GitHub or GitLab, serves as a central location that team members can access to collaborate on the project.
The repository name is crucial as it allows you to identify your project and facilitates collaboration among team members.
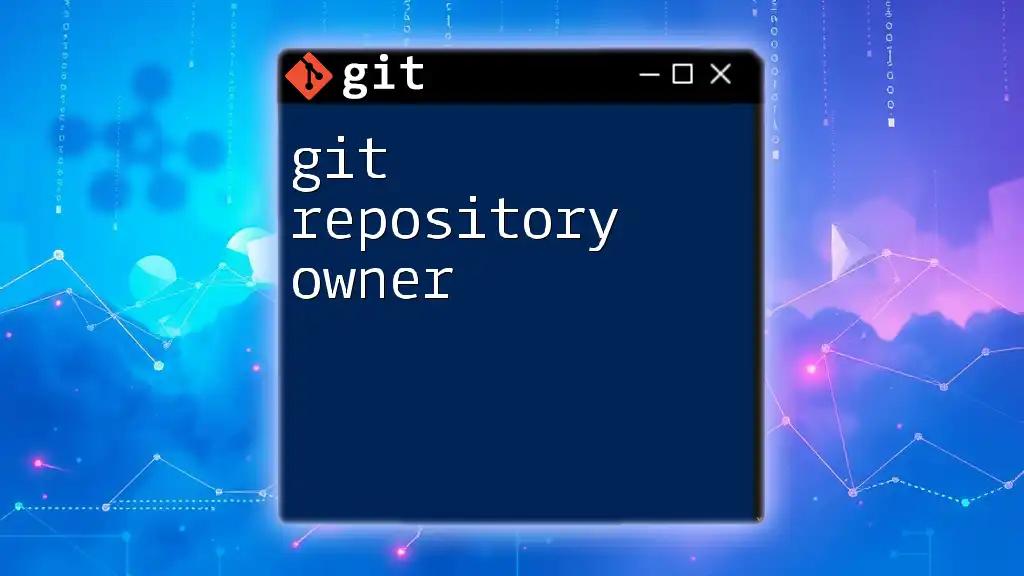
How to Access the Git Repository Name
Using the Command Line
The command line is a powerful way to interact with your Git repository. One of the simplest commands to get the repository name is:
git remote -v
When you execute this command, it provides you with the remote connections to your repository, showing the associated URLs for fetching and pushing. The output typically looks something like this:
origin https://github.com/username/repo-name.git (fetch)
origin https://github.com/username/repo-name.git (push)
In this case, the repository name is repo-name. Understanding how to read this output is essential not just for retrieving the name, but also for managing your project effectively.
Using the Git Configuration File
Every Git repository contains a special file called `.git/config`. This file includes configurations for your repository, including information about remotes.
To find the repository name, you can access this configuration file. Here’s an example snippet:
[remote "origin"]
url = https://github.com/username/repo-name.git
fetch = +refs/heads/*:refs/remotes/origin/*
In the `[remote "origin"]` section, the `url` provides the repository’s full path. Essentially, the last part of the URL (after the last `/`) represents the repository name: repo-name.
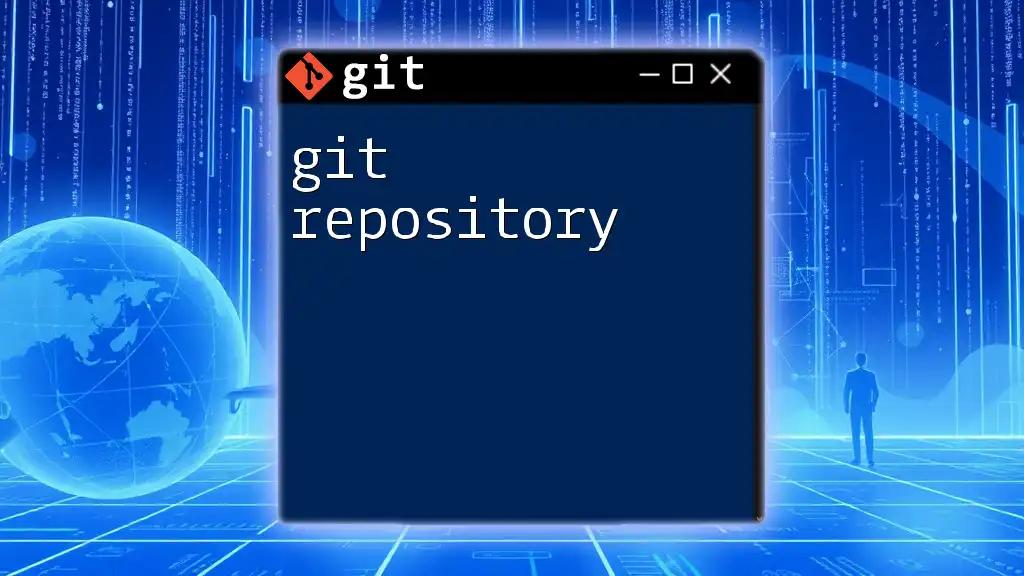
Getting Repository Name for Cloned Repositories
From the URL
If you have cloned a repository and want to quickly get the repository name, you can utilize this command:
git config --get remote.origin.url
Running this command retrieves the complete URL of the remote repository. You can then extract the repository name from the URL. Understanding the URL structure will help you identify the name effectively.
Using the Git Command Line Interface
If you want a more straightforward way to find the repository name from your current directory, you can navigate to your project's folder and use this command:
cd path/to/repo
ls -l
This command lists the contents of your project directory. If the `.git` directory is present, it indicates that you’re in a valid Git repository. To get the top-level directory and, indirectly, the repository's name, run:
git rev-parse --show-toplevel
This command outputs the top level of your Git working directory. The output will be a path, from which you can again derive the repository name by looking at the last part of the path.
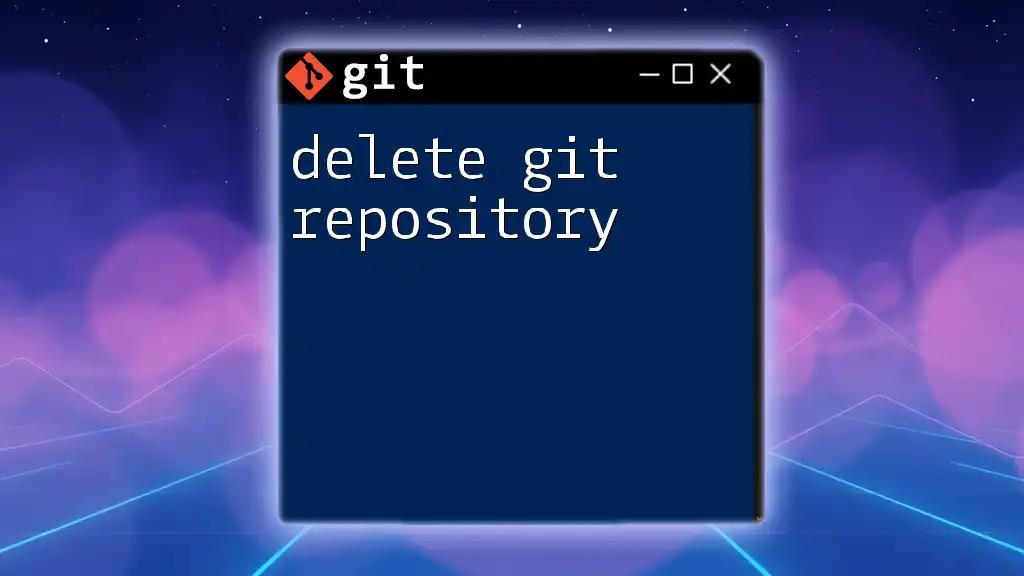
Fetching the Repository Name Programmatically
Using Scripting Languages
For those who prefer a programmatic approach, you can use scripting languages like Python to access Git repository information. Here’s a simple example:
import subprocess
def get_repo_name():
result = subprocess.run(['git', 'rev-parse', '--show-toplevel'], stdout=subprocess.PIPE)
return result.stdout.decode('utf-8').strip().split('/')[-1]
print(f"Repository Name: {get_repo_name()}")
This Python script uses the `subprocess` module to run a Git command that retrieves the top-level directory of the repository. The `split('/')[-1]` segment extracts the repository name efficiently. Thus, you can get the repository name programmatically, which can be beneficial in automated workflows.
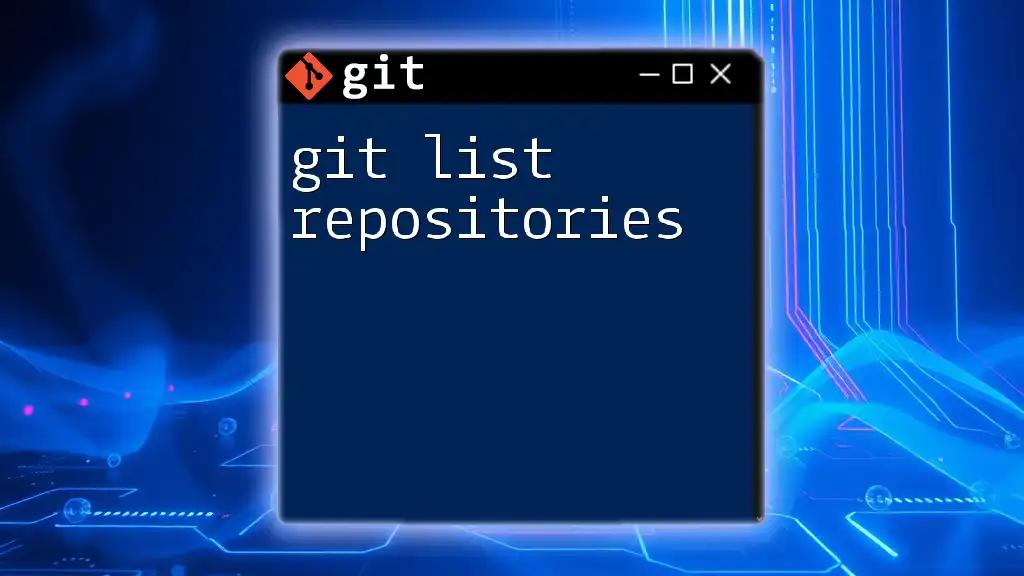
Conclusion
In this guide, we have covered multiple ways to get the Git repository name. We demonstrated how to utilize both command-line tools and desktop file systems to pinpoint the repository name. Recognizing the repository name has practical implications in project management and collaboration among team members.
By understanding these methods, you will become more proficient in using Git, allowing you to navigate your repositories with confidence. Remember, having quick access to this type of information can significantly enhance your efficiency and productivity when working with Git.
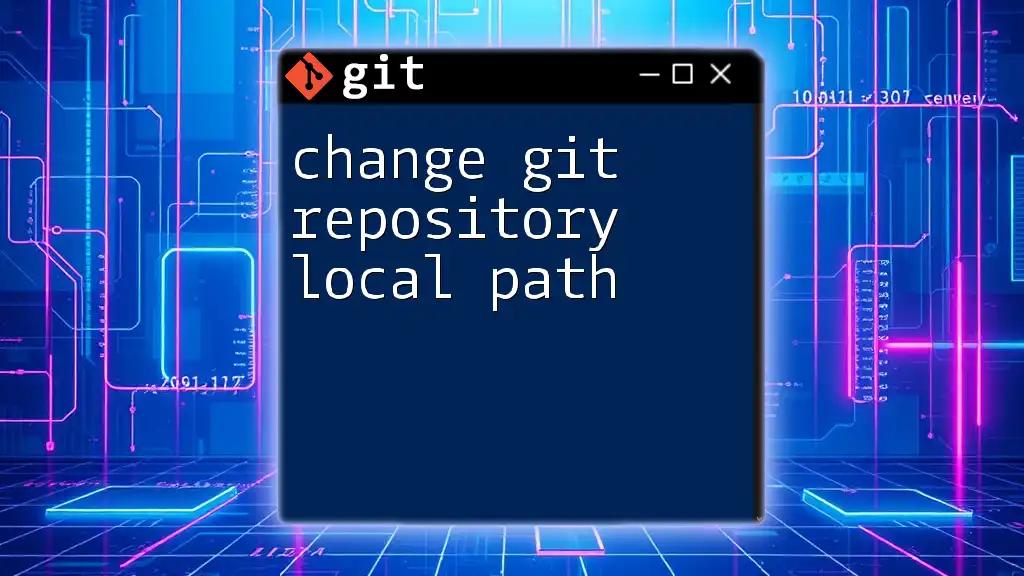
Additional Resources
For those eager to learn more, consider exploring further Git documentation and tutorials available on platforms like GitHub, GitLab, and various educational websites.
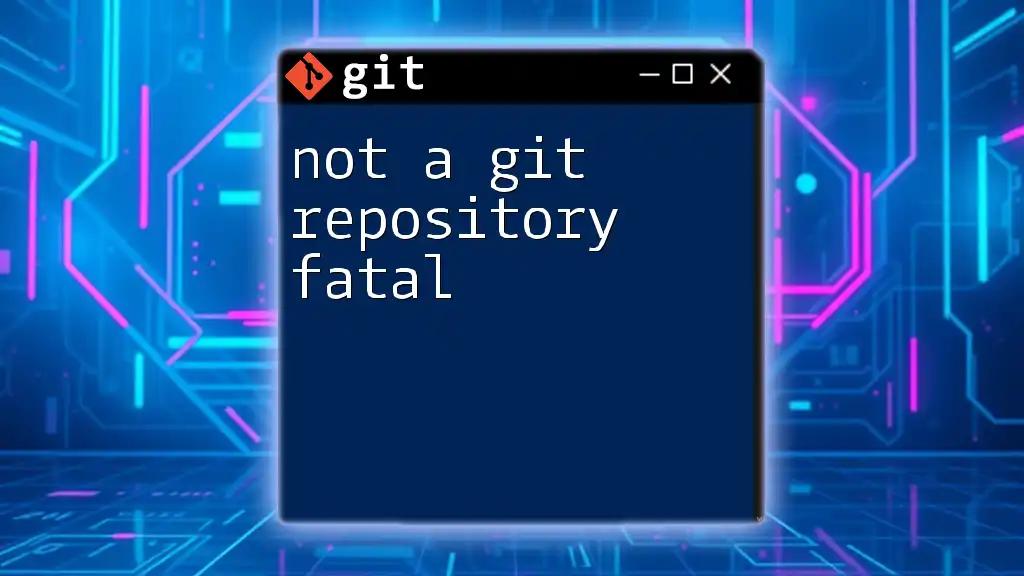
Calls to Action
Stay connected for more insightful articles and resources on Git commands! Sign up for our newsletter and receive a free guide on essential Git commands that every developer should know.