A Git repository (repo) is a storage space for your project that tracks changes to files and allows for collaboration through version control.
Here's a basic command to create a new Git repository:
git init my-project
Understanding Git Repositories
What is a Git Repository?
A Git repository, commonly referred to as a git repo, is a collection of files and directories that are tracked by Git. Each git repo contains all the project's version history, which allows developers to manage and track changes to their code over time. The significance of utilizing a git repo lies in its ability to facilitate collaboration, maintain historical records, and enable version control.
Types of Git Repositories
Local Repositories
A local repository exists on your own machine. When you create a new project and initialize it with Git, you create a local repository that stores the entire version history on your computer. This allows you to work offline and commit changes at your convenience.
Remote Repositories
A remote repository is hosted on a server and can be accessed by multiple users. Common platforms for hosting remote git repos include GitHub, GitLab, and Bitbucket. By syncing your local repo with a remote one, you can collaborate with others and share your code more effectively.
Bare Repositories
A bare repository is essentially a remote repository without a working directory. It is typically used for sharing because it does not contain any files other than the Git versioning information. When team members push their changes, they do so to a bare repository to keep a clean history.
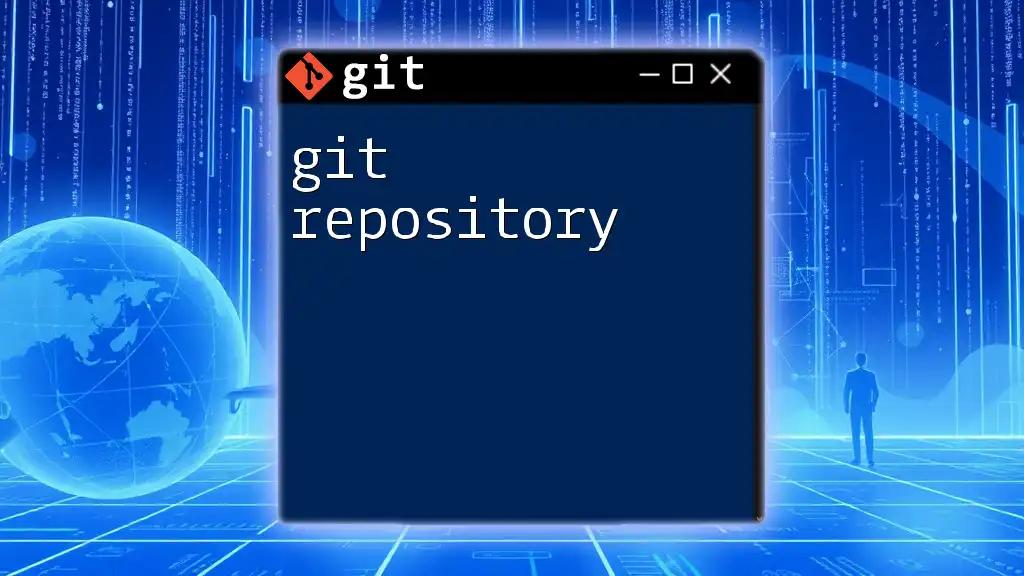
Setting Up a Git Repository
Installing Git
Before you can work with a git repo, you need to install Git on your machine. Installation steps vary depending on your operating system:
- For Windows, download the installer from the official Git website and follow the installation prompts.
- For macOS, you can either install it via Homebrew using `brew install git` or download the installer directly.
- For Linux, use your package manager, for example, `sudo apt-get install git` for Ubuntu.
Initializing a Local Repository
To start a new project, you can initialize a local repository. This is done using the following command:
mkdir my-project
cd my-project
git init
This command creates a new directory for your project and initializes it as a git repo, setting up the necessary hidden `.git` folder that Git uses to track changes.
Cloning a Remote Repository
If you want to work with an existing project, you can clone a remote repository using the `git clone` command. This will create a local copy of the repo on your machine.
git clone https://github.com/user/repo.git
This command pulls down all the files and version history from the specified remote repo, allowing you to work with it locally as if it were your own.
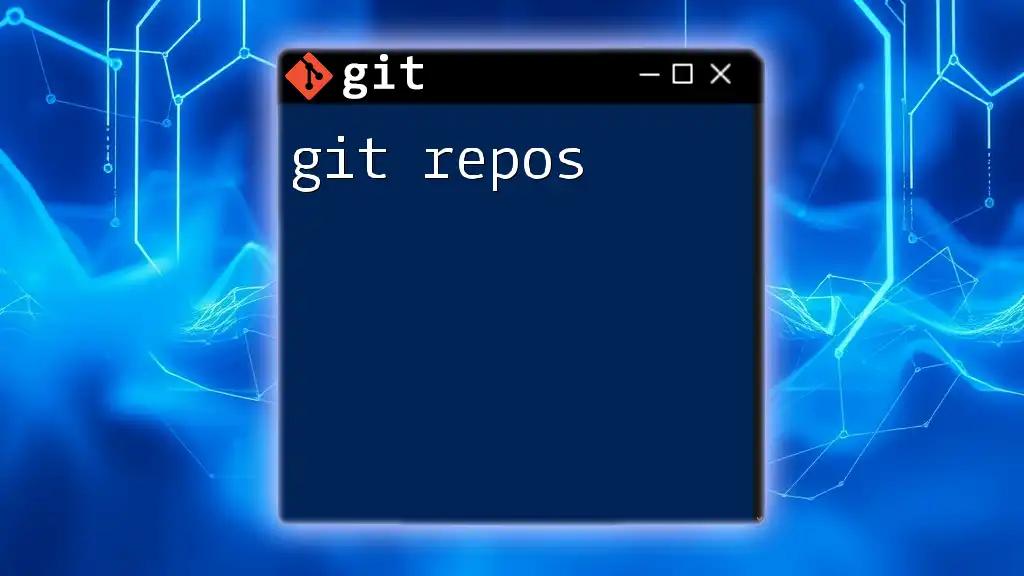
Working with Git Repositories
Adding and Committing Changes
Staging Changes
To track changes in your files, you need to stage them using the `git add` command. This command signals Git to include changes in the next commit. You can stage individual files or all changes in the directory.
git add file1.txt
git add .
The first command stages a specific file, while the second stages all modified files, making it easy to prepare your changes for committing.
Committing Changes
Once you’ve staged your changes, you can commit them to the git repo using the `git commit` command. It is essential to provide a meaningful commit message that summarizes what changes were made.
git commit -m "Initial commit"
Good commit messages help you and your collaborators understand the history of changes and the context behind them.
Viewing Repository Status
To check the current state of your git repo, use the `git status` command. This command provides valuable information about staged, unstaged, and untracked files, which allows you to know what changes need to be committed.
Viewing Commit History
You can see the history of commits in your git repo using the `git log` command. This command displays the commit history in reverse chronological order, including commit messages, authors, and timestamps.
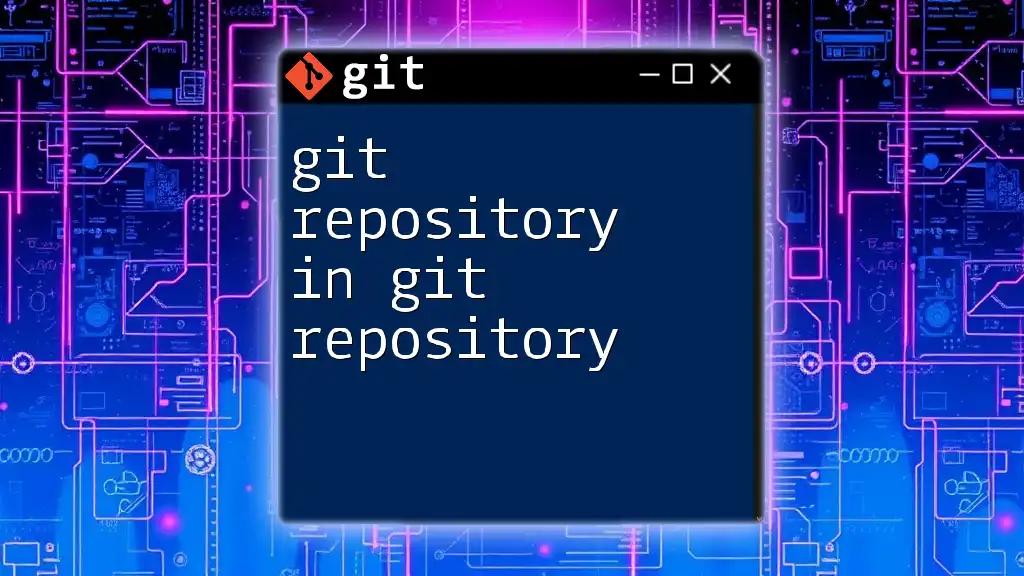
Managing Remote Repositories
Adding a Remote Repository
To sync your local git repo with a remote one, you need to add it as a remote origin. This is done with the `git remote add` command, which tells Git where to push and pull changes.
git remote add origin https://github.com/user/repo.git
This command adds a remote repository called `origin`, which is a common convention for the primary remote repo.
Fetching and Pulling Changes
Fetching Changes
Use the `git fetch` command to download changes from the remote repo without merging them into your local branch. This allows you to review the changes before integrating them into your codebase.
Pulling Changes
The `git pull` command combines the functionalities of `git fetch` and `git merge`, downloading changes from the remote repo and merging them into your current branch. This is a convenient way to stay updated with your collaborators’ work.
Pushing Changes to Remote
Once you’ve committed your changes locally, you can share them with others by pushing to the remote repository.
git push origin master
This command uploads your commits to the `master` branch of the remote repository, ensuring that your work is available for your collaborators.
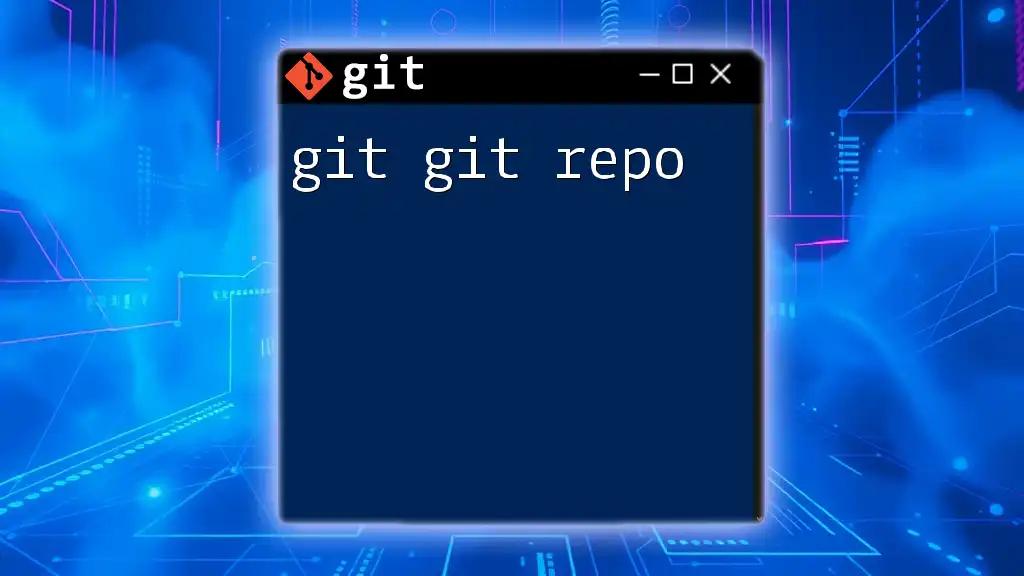
Branching and Merging in Repositories
What is a Branch?
A branch in Git represents an independent line of development. You create a branch to work on a new feature or make changes without affecting the main codebase. This makes collaboration and experimentation much safer.
Creating a New Branch
You can create a new branch using the `git branch` command. This allows you to start development on a separate line without affecting the main branch.
git branch feature-branch
Switching Between Branches
To switch between branches, use the `git checkout` command. This allows you to navigate your project’s development history easily.
git checkout feature-branch
Merging Branches
To bring changes from one branch into another, you use the `git merge` command. This integrates the specified branch's changes into your current branch.
git merge feature-branch
It’s essential to resolve any conflicts during this process to ensure a smooth integration of changes.
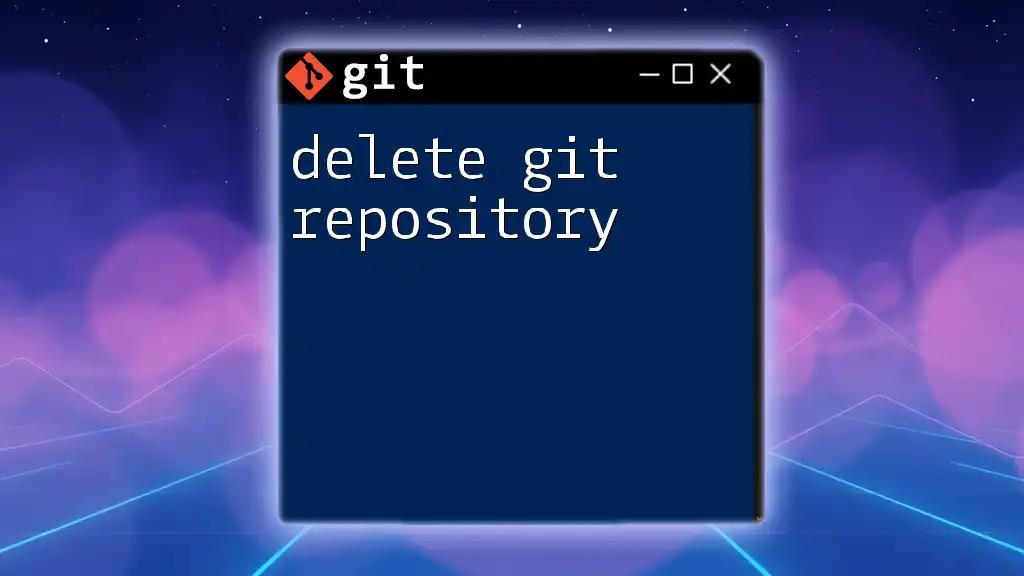
Best Practices for Using Git Repositories
Commit Often and with Clear Messages
Regular commits capture progress and allow for easier rollback if needed. Each commit should have a clear and descriptive message to explain what changes were made.
Regularly Pull Changes
Consistently pulling changes from the remote repository keeps your local branch up-to-date, preventing large merge conflicts and ensuring you’re always working with the latest code.
Use Meaningful Branch Names
Naming your branches descriptively helps collaborators understand their purpose. A good practice is to include the feature being developed or the issue being fixed in the branch name.
Clean Up Unused Branches
To maintain a clean git history, delete branches that are no longer needed. Use the following command to remove a branch:
git branch -d feature-branch
This helps prevent confusion and clutter in your repository.
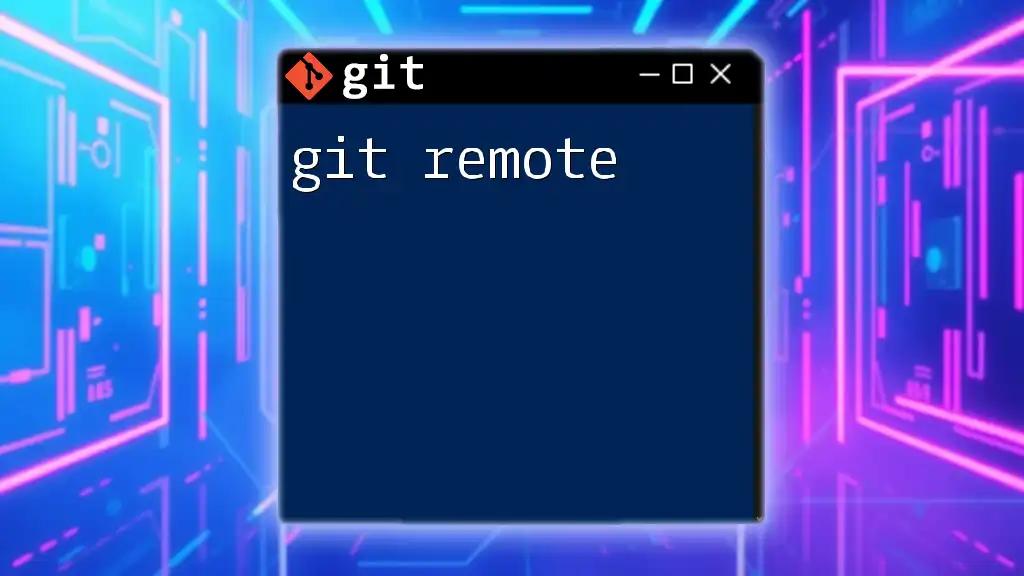
Conclusion
Recap of Git Repositories
In summary, understanding git repos is crucial for effective collaboration and version control in software development. By mastering the concepts of initializing, adding, committing, managing, branching, and merging, you can streamline your workflow.
Encouragement to Practice
To truly grasp the functionalities of git repos, hands-on experience is critical. Start your own projects, work with existing repositories, and explore additional resources to deepen your understanding of Git. With practice, you’ll become proficient in using git commands effectively.