`git grep` is a powerful command that allows you to search for specific patterns or text within your git repository's tracked files.
git grep "search_term"
What is Git Grep?
Git Grep is a powerful command-line utility within Git that allows developers to search for specified patterns in the codebase quickly. It functions similarly to the traditional `grep` command but is enhanced with Git's version control capabilities. This means you can search through your project history, branches, and files with increased efficiency and relevance.
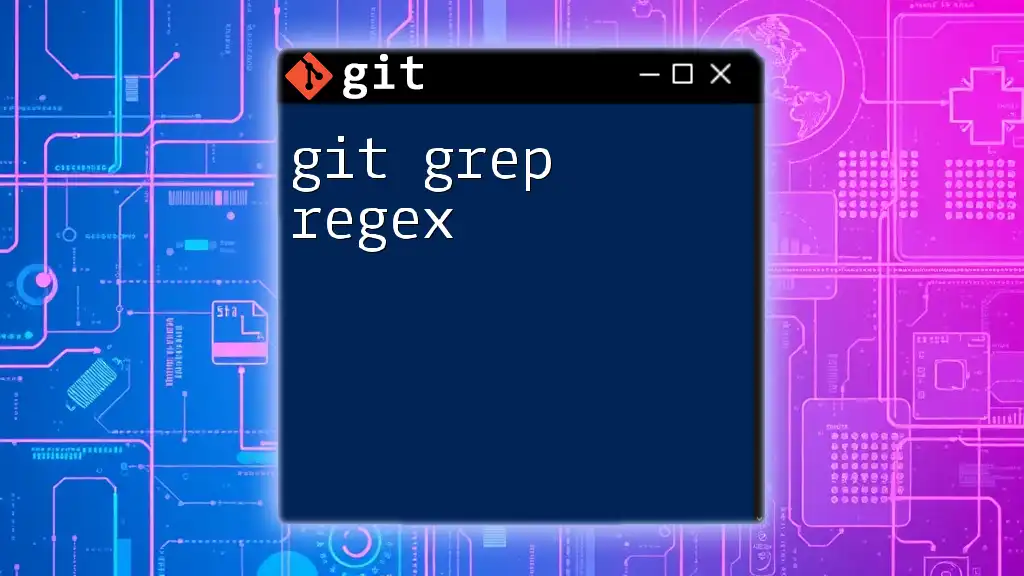
Benefits of Using Git Grep
Using Git Grep offers several key advantages:
- Speed: Git Grep is optimized to search through repositories rapidly, making it a preferred choice for developers working with large codebases.
- Git-aware: Unlike standard `grep`, Git Grep integrates seamlessly with Git's features, enabling it to understand the context of your code, including file changes and commit history.
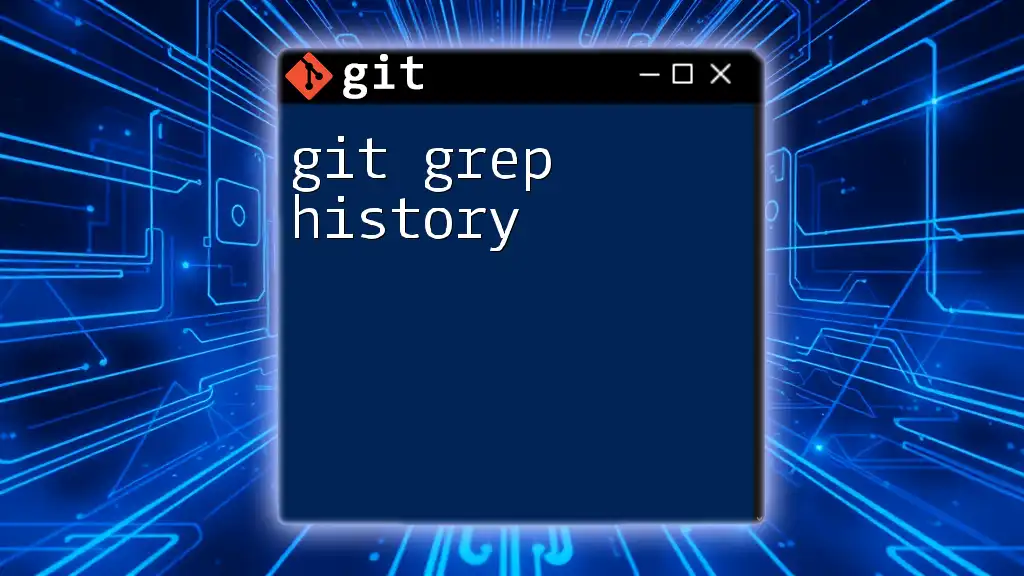
Understanding Git Grep Command
Basic Syntax of Git Grep
The foundational structure of the Git Grep command looks like this:
git grep <pattern> <options>
This syntax allows you to specify a search pattern and optionally include a range of options for a more tailored search.
Common Use Cases
Developers use Git Grep for various tasks, such as:
- Searching for a specific function definition within a large code repository.
- Identifying where a particular variable is used throughout the code.
For instance, if you want to find all instances of the term "function" within your repository, you can execute:
git grep "function"
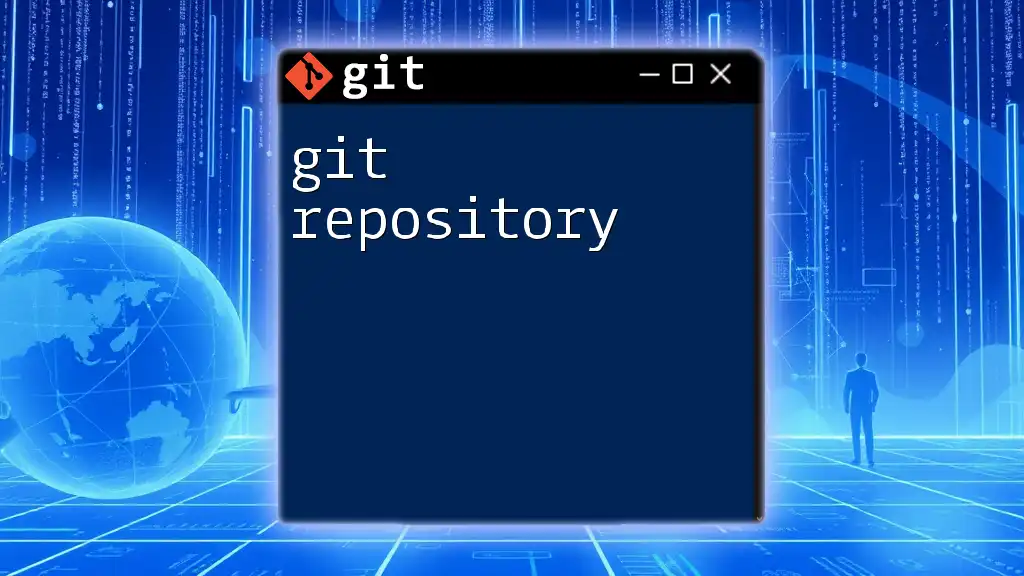
Using Git Grep Effectively
Searching with Simple Patterns
Basic Pattern Search: Git Grep enables straightforward searching for terms or phrases. If you're seeking the string "function," the command is easy to remember and execute:
git grep "function"
Case Sensitivity Options: By default, Git Grep is case-sensitive. However, if you want to perform a search that ignores case, you can leverage the `-i` option, allowing you to find variations such as "Function", "FUNCTION", or "function":
git grep -i "function"
Searching with Regular Expressions
Introduction to Regex
Regular expressions (regex) are sequences of characters that form search patterns. They are a powerful tool for developers, allowing complex searches based on specific criteria.
Using Regex with Git Grep
Git Grep supports regex, giving you the opportunity to search for more sophisticated patterns. For example, if you want to find all functions that are followed by one or more digits, use the `-E` (for extended regex) option:
git grep -E "func[[:digit:]]+"
This command will match any instance of "func" followed directly by one or more numerals.
Limiting Your Search Scope
Searching Specific Directories or Files
You can refine your search by targeting specific directories or files. If you want to search only within a subdirectory called `src`, the command would be:
git grep "function" src/
Additionally, if you're interested in only JavaScript files, you can filter by file type:
git grep "function" -- '*.js'
Including or Excluding Certain Commits
Searching in Specific Commits
One of the powerful features of Git Grep is its capability to search through specific commits. By using a commit hash, you can limit your query to the state of the repository at that point in time:
git grep "function" abc1234
Excluding Commits
You can also avoid certain commits from your search if needed. While this is less common, it may be useful in certain scenarios where you want to focus on recent changes.
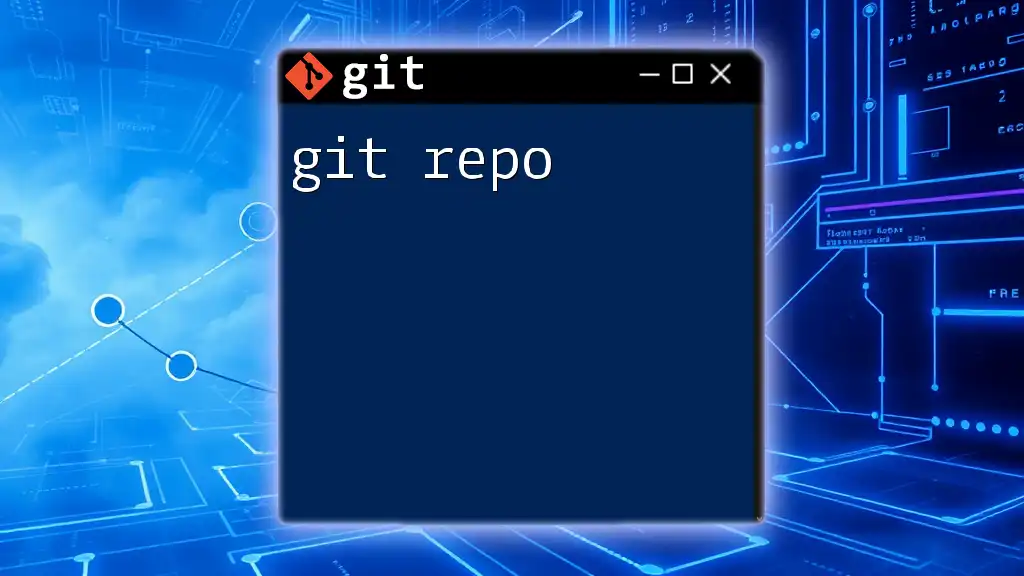
Advanced Git Grep Features
Combining Git Grep with Other Git Commands
Piping Outputs
Git Grep can be seamlessly integrated with other command-line utilities to extend its functionality. For example, if you want to count the number of occurrences of the term "function" across all files, you can use:
git grep -c "function"
Using Git Grep with `git log`
Another interesting application of Git Grep is in connection to commit messages. To find mentions of specific functions or terms in your commit history, combine it with `git log`:
git log --grep="function"
Customizing Git Grep Output
Formatting the Output
Git Grep allows you to format the search results to suit your needs. For instance, adding the `-n` option will display line numbers alongside matches, while the `-H` option shows the filename:
git grep -nH "function"
This can be helpful when you need to pinpoint the exact location of a match within the code.
Performance Considerations
When to Use Git Grep
It's important to recognize when Git Grep is most useful. Best practices suggest using Git Grep in larger repositories or when rapidly iterating on specific functionalities. Its performance shines when compared to more general search approaches.
Limiting Resource Usage
When working with very large codebases, your search can become resource-heavy. Consider limiting the depth of your search or targeting specific branch states to maintain optimal performance.
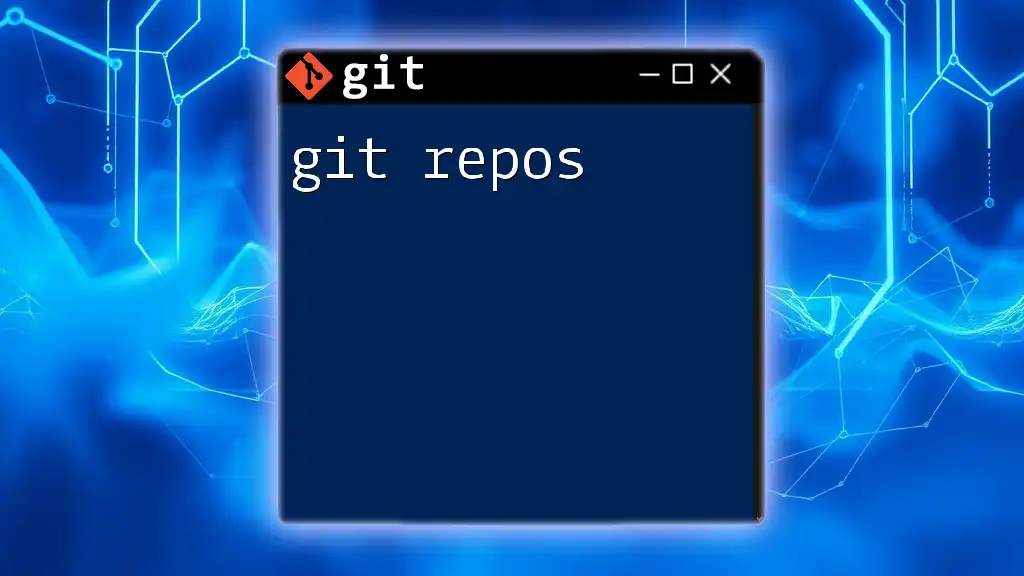
Troubleshooting Common Issues
When Git Grep Doesn't Return Expected Results
Many users encounter situations where their Git Grep command doesn’t return expected results. Common pitfalls include typos in the search pattern and case sensitivity.
Version Compatibility Issues
Ensure that your Git version supports the latest Git Grep features. Some advanced functionalities may not be present in older releases, which can lead to confusion if expectations don’t match reality.
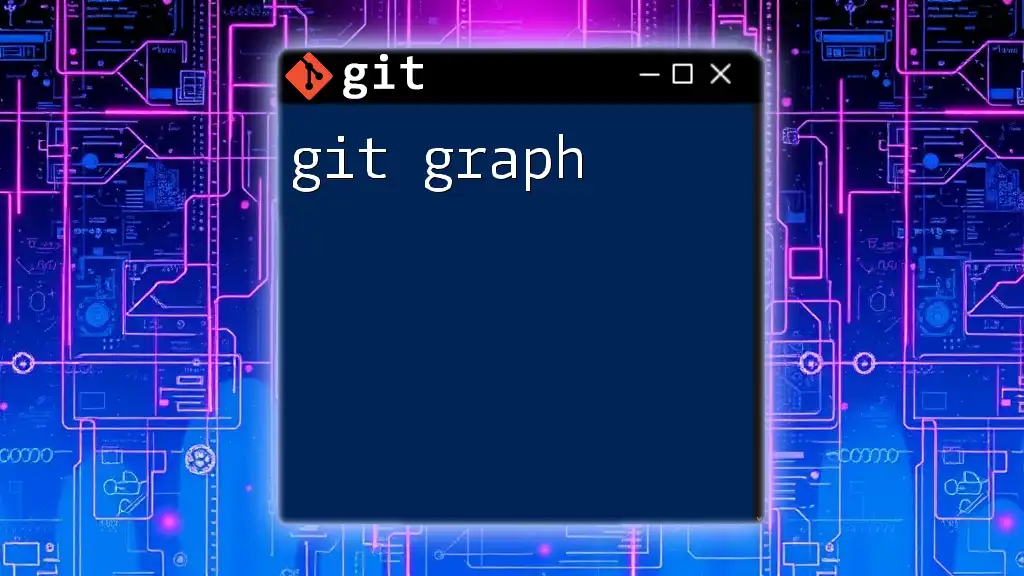
Conclusion
Mastering Git Grep is an invaluable skill that can greatly enhance your coding efficiency. Its ability to quickly search through code efficiently is unmatched, and by practicing the commands outlined above, you can significantly improve your productivity.
Experimenting with Git Grep in your projects will provide real-time context and insight, polishing your Git command line skills and making you a more effective developer.
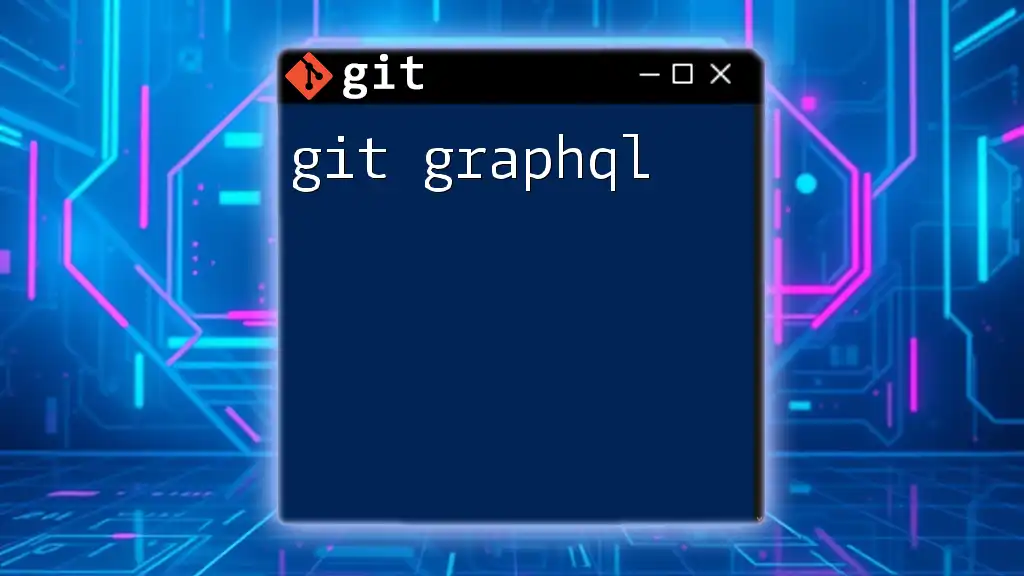
Further Resources
- Official Git Documentation on Grep: A comprehensive resource to dive deeper into the command.
- Recommended Tutorials and Books: Explore further learning materials to expand your Git knowledge.
- Community Forums for Git Discussions: Join discussions with experts to troubleshoot and learn from real-world usage.