The `git grep` command allows you to search through your Git repository's files for a specific pattern, and when used with the `--cached` flag and a specific commit hash, it can help you search the history of the committed files for that pattern.
Here’s an example of how to use it:
git grep "pattern" <commit-hash>
Understanding Git Grep
What is Git Grep?
`git grep` is a powerful command-line utility that allows users to search through the working directory and tracked files in a Git repository. Its primary purpose is to search for specific patterns (such as text strings or regular expressions) effectively within the content of files. Unlike other search methods, such as using `grep` in the file system, `git grep` takes advantage of Git's indexing, making it faster and more efficient as it operates directly on versioned content.
Why Use Git Grep?
The distinctive advantage of using `git grep` lies in its performance, especially in large projects with numerous files. By leveraging Git's internal structures, it streamlines the search process, enabling users to quickly locate code snippets, comments, or any specified patterns across the project. This increases productivity and minimizes time spent on code reviews and debugging.
Basic Syntax of Git Grep
The syntax for `git grep` is straightforward and intuitive, allowing users to customize their searches quickly. The general command format is as follows:
git grep [options] <pattern> [<commit>...]
In this structure:
- `<pattern>` is the text string or regex pattern you want to search for.
- `[options]` are command-line flags that modify the behavior of the search.
- `[<commit>...]` allows specifying a range or specific commits in history to search.
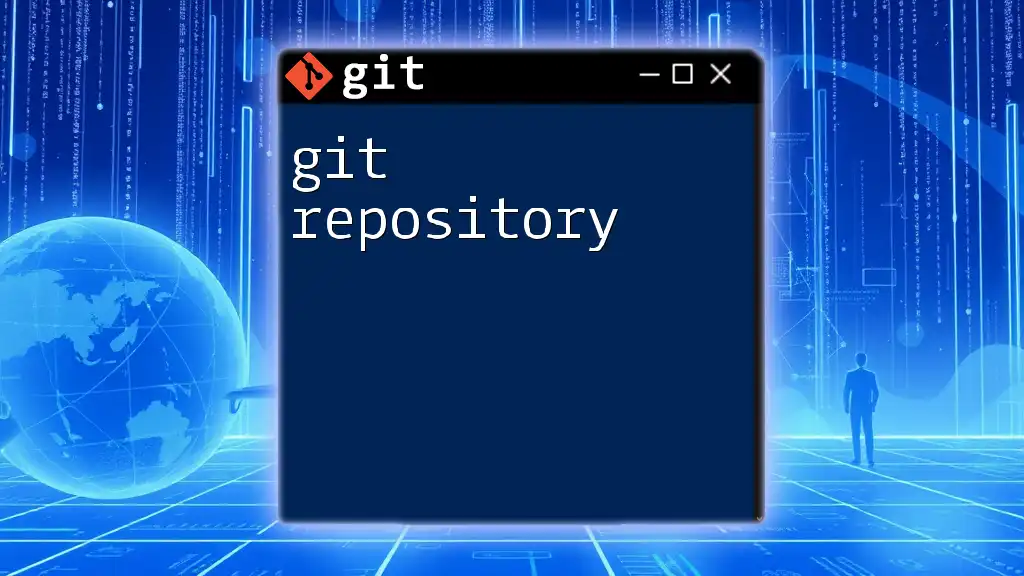
Searching Through Commit History
The Basics of Searching History
Understanding how commit history works in Git is crucial for effective searching. Each commit captures the state of the project at a particular point in time, along with a unique identifier (commit hash), author information, and commit message. Searching through the history can yield insights about code changes and help trace modifications made over time.
Performing a Basic Search
To search for a specific pattern in the most recent commit, simply use the following command:
git grep "pattern"
The output will display the files containing the specified pattern, along with their respective line numbers where matches occur. This allows users to jump straight to the relevant sections of the code base quickly.
Searching Specific Commits
To search through a specific commit, one can use the commit hash with the `git grep` command:
git grep "pattern" <commit-hash>
To find the `<commit-hash>`, use:
git log
The `git log` command provides a historical view of previous commits, displaying important details like the commit hash, author, and date, essential for pinpointing the commit you want to investigate.
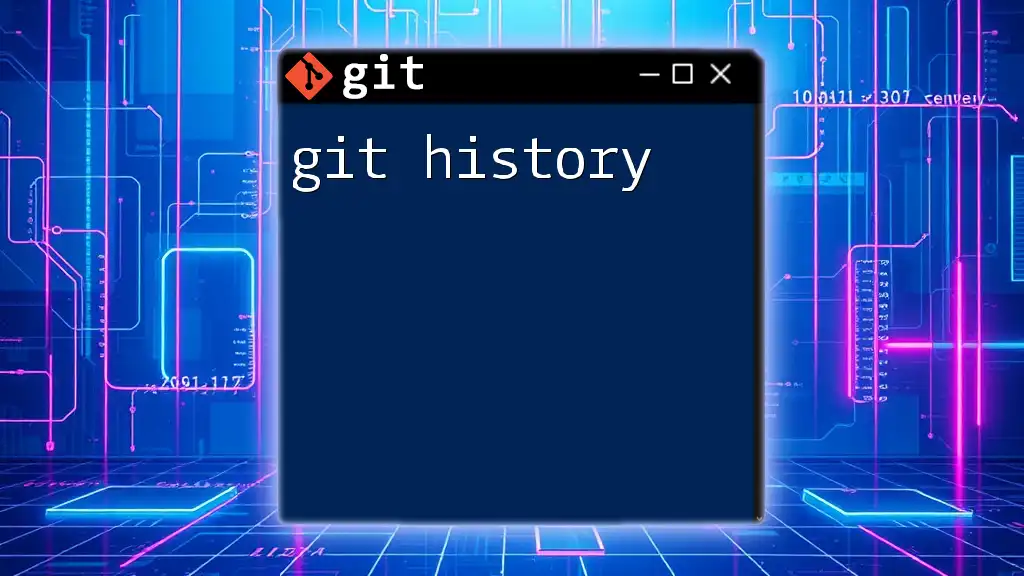
Advanced Git Grep Features
Using Regular Expressions
`git grep` supports regular expressions, allowing users to define complex search patterns. For instance, if you want to find a pattern that includes a sequence of digits, you could execute:
git grep "pattern[0-9]*"
This added flexibility can significantly enhance your search capabilities, especially in large and diverse code bases.
Combining Git Grep with Other Git Commands
Searching with Git Log
Integrating `git log` with `git grep` can provide deeper insights into changes made in the code over time. For instance, you can pipe the output of `git log` to `git grep` like this:
git log -p | git grep "pattern"
This combination allows you to search through the detailed change history, showcasing how certain patterns have evolved across commits.
Limiting Search Scope
Using various flags with `git grep` can limit the searches and enhance their effectiveness. For example, using the `-n` flag shows line numbers, while the `-i` flag ignores case:
git grep -n "pattern" --cached
The `--cached` option performs the search in staged files, helping you to verify changes before committing.
Searching in Different Branches
You can also search for patterns in specific branches using the following syntax:
git grep "pattern" branch-name
This is particularly useful when you want to evaluate how a specific feature or change has been implemented differently across branches, allowing for an effective comparison.
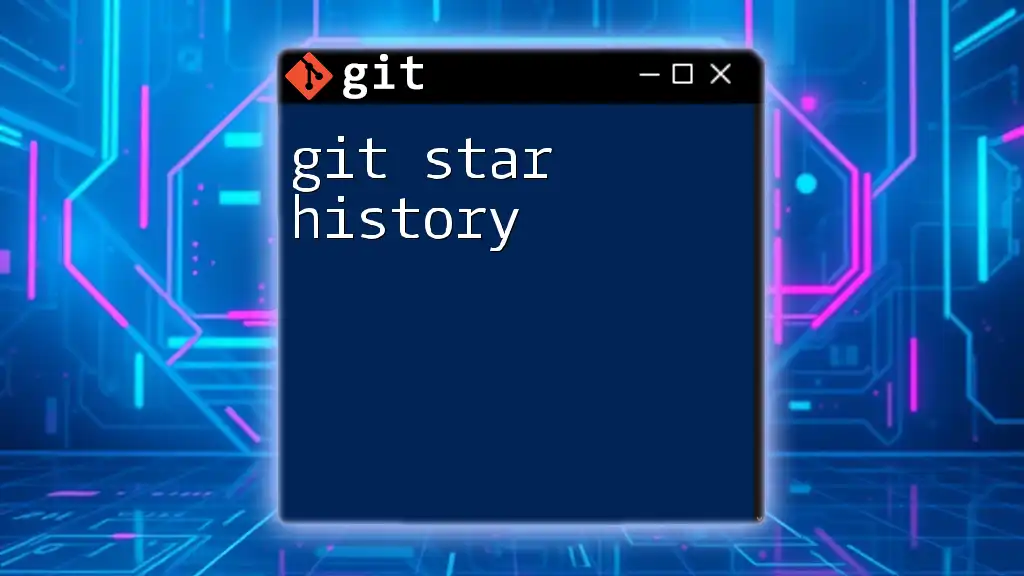
Real-World Use Cases
Debugging Code
In debugging scenarios, `git grep` can be indispensable. For instance, if you're trying to trace a bug that was introduced in a specific function, you could search for that function's name in previous commits. This can dramatically speed up the troubleshooting process by pinpointing when changes were made:
git grep "functionName" HEAD~10..HEAD
Finding References Quickly
Another practical use of `git grep` is locating references or dependencies within large projects. Suppose you're looking for all instances of a package or an interface. By using multiple patterns, you can streamline the search across your project:
git grep -e "FirstPattern" -e "SecondPattern"
This can improve your efficiency during code reviews or refactoring tasks.
Audit and Code Review
For code audits and reviews, `git grep` can help assess code quality and maintainability. By searching for patterns that might indicate hard-coded values or deprecated functions, developers can ensure that the codebase adheres to best practices:
git grep "pattern" HEAD~10..HEAD
This command searches for a given pattern across the last ten commits, letting developers spot potential issues before merging branches.
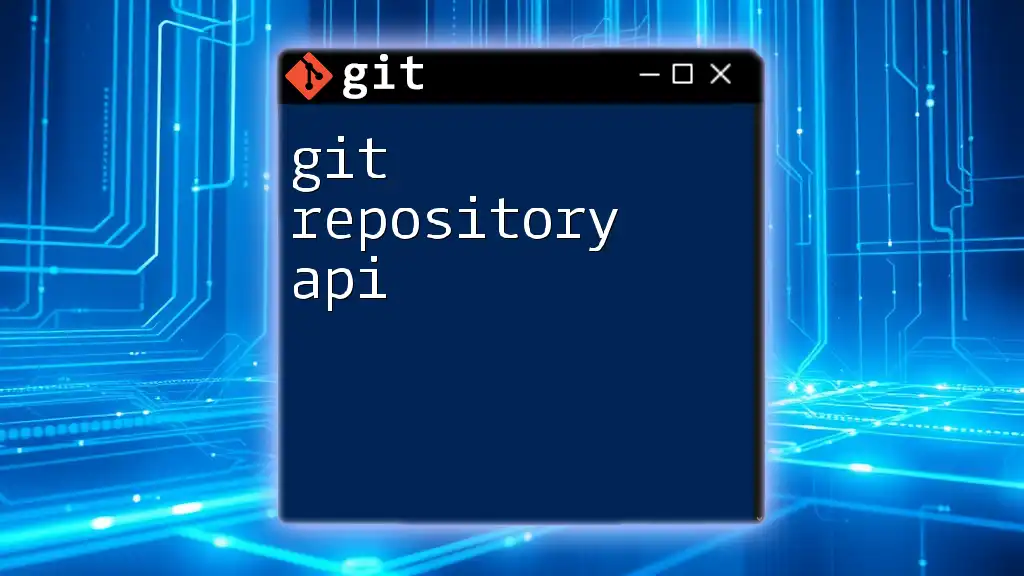
Best Practices for Using Git Grep
Structuring Your Searches
Creating efficient search patterns is crucial for maximizing the utility of `git grep`. It is advisable to make use of clear and descriptive patterns, which not only enhance and speed up your searches but also make it easier for colleagues to understand your intentions when collaborating.
Handling Performance
In large repositories, performance can become a concern. To optimize your searches, consider using `git grep` in combination with small scoped commands or using flags that constrain the search to a selective range of commits or branches. Efficient search strategies can significantly cut down search time, allowing you to focus on code quality rather than getting lost in results.
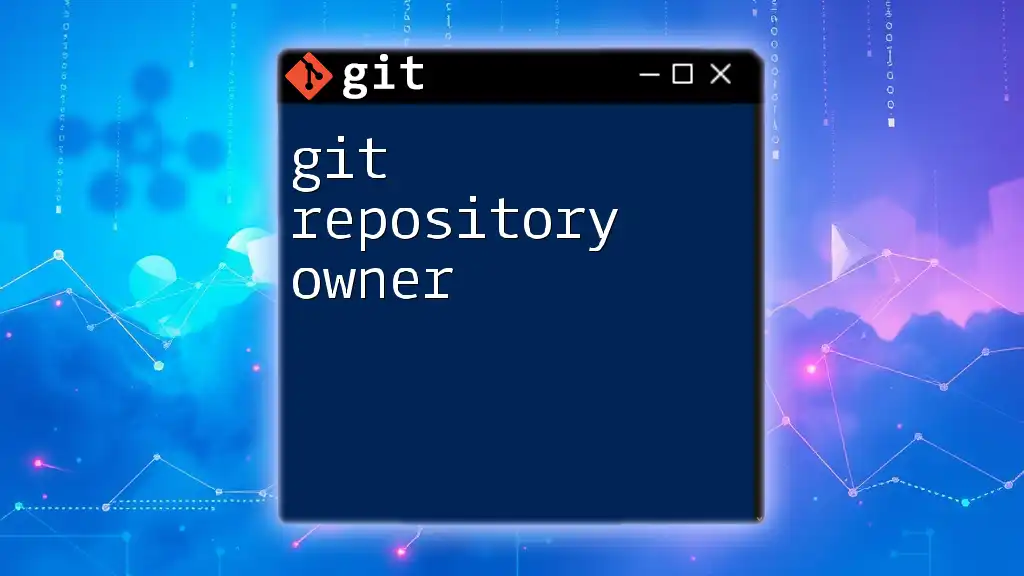
Conclusion
Exploring `git grep history` demonstrates how powerful and efficient this command can be in navigating through a project's history. With its ability to execute fast searches over specific commits, branches, and patterns, developers can improve their workflows significantly. Embracing `git grep` not only enhances productivity but also empowers programmers with vital insights about their code.
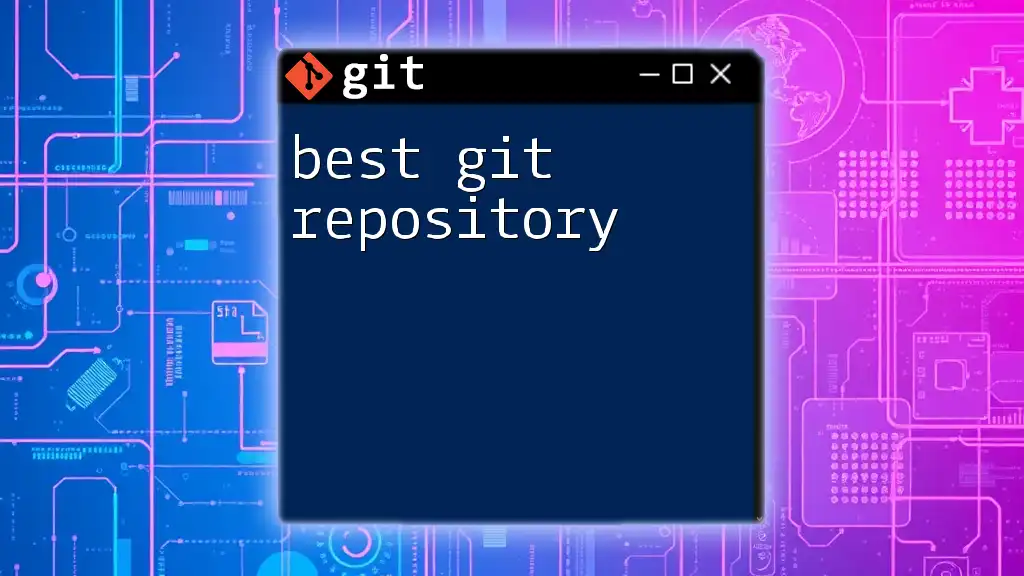
Additional Resources
For those looking to expand their knowledge beyond this guide, links to official Git documentation can provide a wealth of information. Additionally, consider exploring advanced tutorials that dive deeper into Git commands and their applications. Lastly, discover recommended plugins or tools that can enhance your experience with `git grep`, helping you become more adept in your Git usage.