Git GraphQL is a metadata query language for your Git repositories, allowing you to fetch and manipulate data about your version control directly through a flexible API.
Here's a simple example of using Git commands in a GraphQL structure to fetch the latest commit history:
git log --pretty=format:'{"commit": "%H", "author": "%an", "date": "%ad", "message": "%s"}' --date=short -n 5
Setting Up Your Environment
Prerequisites
Before you start working with Git and GraphQL, ensure that you have the necessary tools and software installations ready for a seamless development experience. It's important to have a good IDE (Integrated Development Environment), ideally one that supports both JavaScript and Git functionalities. Some popular choices include Visual Studio Code, Atom, and WebStorm.
Installation Guide
Installing Git
To get started with Git, you’ll need to install it on your local machine:
- Windows: Download the latest version from the [Git official website](https://git-scm.com/) and follow the installation instructions.
- macOS: Use Homebrew to install Git by running:
brew install git
- Linux: Install Git using your package manager. For Debian-based systems, run:
sudo apt-get install git
After installation, verify that Git is working correctly by running:
git --version
Setting Up a GraphQL Server
Once Git is properly installed, you can set up a basic GraphQL server using Node.js. Make sure you have Node.js installed; if not, visit the [Node.js website](https://nodejs.org/) for installation instructions.
To initialize a new GraphQL project:
mkdir my-graphql-server
cd my-graphql-server
npm init -y
npm install express graphql express-graphql
Then create an `index.js` file with a simple GraphQL server setup:
const express = require('express');
const { graphqlHTTP } = require('express-graphql');
const { buildSchema } = require('graphql');
const schema = buildSchema(`
type Query {
hello: String
}
`);
const root = {
hello: () => 'Hello, World!',
};
const app = express();
app.use('/graphql', graphqlHTTP({
schema: schema,
rootValue: root,
graphiql: true,
}));
app.listen(4000, () => console.log('Server running at http://localhost:4000/graphql'));
With this, your GraphQL server will be running on port 4000.
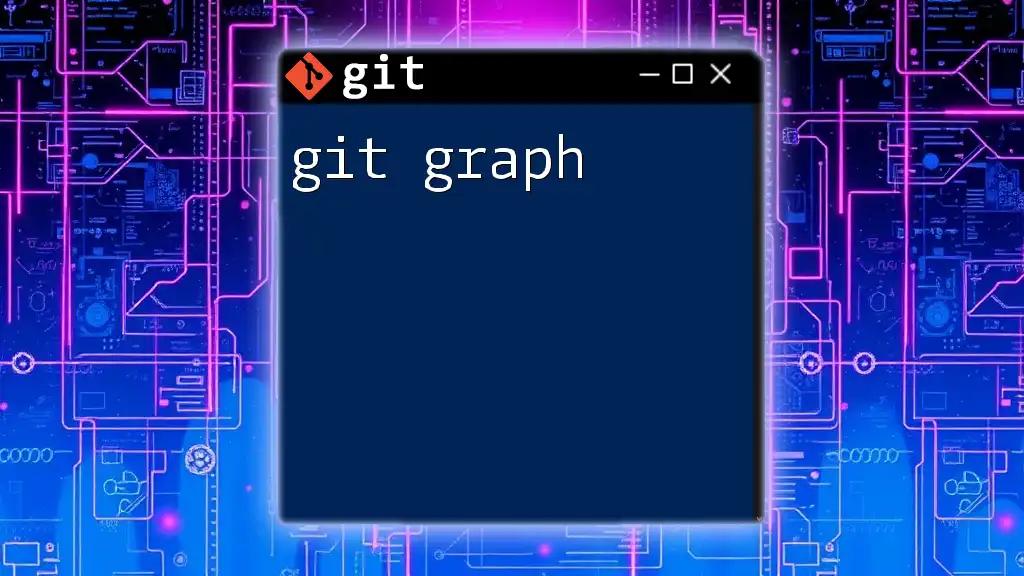
Git Basics for GraphQL Developers
Understanding Git Commands
To effectively manage your GraphQL project, it's imperative to understand core Git concepts such as repositories, commits, branches, and merges. Committing changes frequently and ensuring clear commit messages is essential for project maintenance.
Essential Git Commands
-
`git clone`: This command allows you to obtain a copy of an existing repository. The typical usage is:
git clone https://github.com/username/repository.git
-
`git commit`: Make changes in your project, stage those changes, and write an effective commit message:
git add . git commit -m "Add hello query to GraphQL schema"
-
`git push` and `git pull`: Push your local commits to a remote repository and pull the latest changes respectively:
git push origin main git pull origin main
Branching and Merging in Git
Branches are fundamental to Git as they allow you to work on new features without affecting the main codebase. Here's how to effectively manage branches:
Understanding Branches
Creating a new branch for a feature related to GraphQL can help in clean code management. To create and checkout a new branch, use:
git checkout -b feature/graphql-query
Merging Branches
When your feature is ready, it's time to merge it back into the main branch. Familiarize yourself with different merging techniques. A fast-forward merge can be performed as follows:
git checkout main
git merge feature/graphql-query
In the event of merge conflicts while merging, Git will pause the merge. You’ll then need to resolve the conflicts manually in your IDE, stage the resolved files, and finalize the merge with:
git add .
git commit -m "Resolve merge conflicts and merge GraphQL query feature"
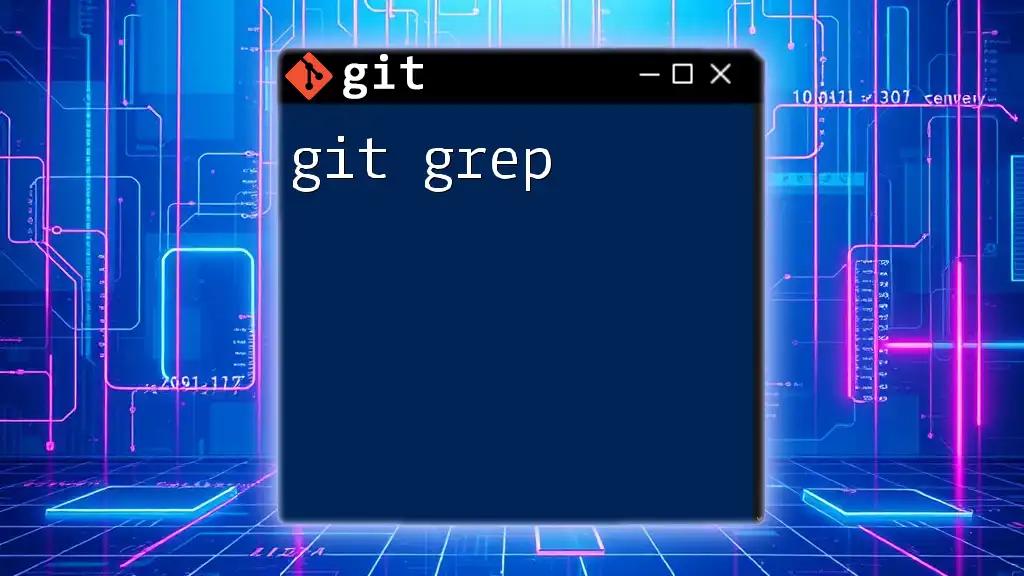
Creating and Managing GraphQL Schemas
Defining Schemas with Git
With GraphQL, managing your schema effectively is crucial. Versioning schemas using Git allows for intelligent tracking of changes. Each schema evolution can be treated as a separate branch, emphasizing changes and enhancements over time.
Implementing Schema Changes
When introducing new queries, use Git to handle updates with minimal disruption. Follow a branching strategy where you create feature branches for new queries or mutations:
git checkout -b feature/add-user-query
After implementing your changes, commit them with a detailed message indicating the purpose:
git commit -m "Add user query to retrieve user data"
Once your changes are tested and ready, push them to your remote repository confidently.
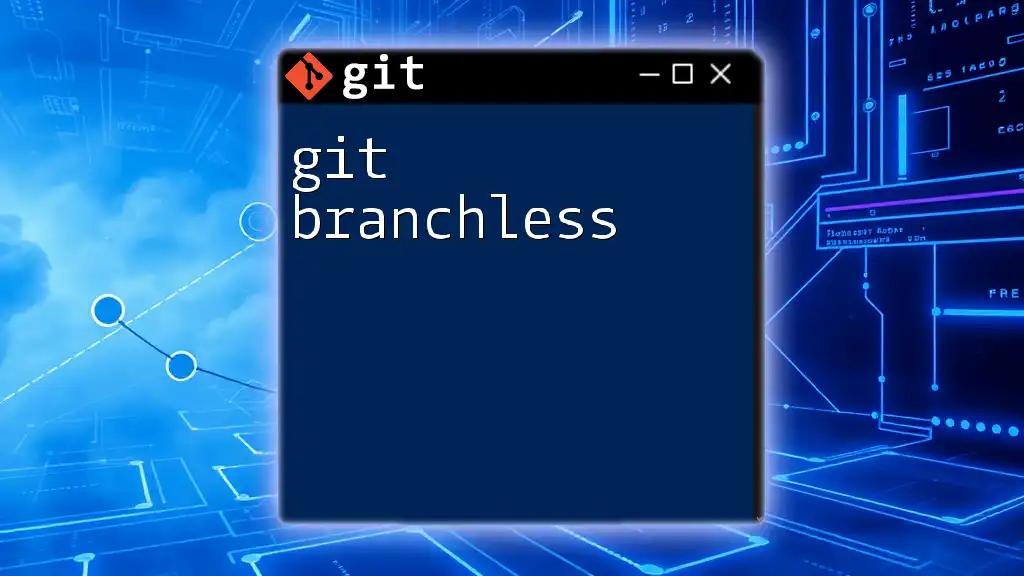
Integrating Git with GraphQL Development
Workflow Integration
The relationship between Git and GraphQL becomes particularly beneficial when you start a new feature. It is best practice to follow a workflow that includes branch creation for every new feature or bug fix.
Using Pull Requests for Code Reviews
Incorporate pull requests (PRs) into your workflow for effective code reviews. PRs serve as a platform to discuss code changes and implement feedback, ensuring that your GraphQL API evolves with quality. Always encourage thorough code review practices and discussions within your team.
Deployment Best Practices
Once your project is ready for deployment, consider setting up Continuous Integration/Continuous Deployment (CI/CD) pipelines. Integrating CI/CD automates testing and deployment processes for your GraphQL server, enhancing efficiency. Make use of platforms such as GitHub Actions or CircleCI, which can be configured to automatically deploy your changes whenever a new version is merged into the main branch.
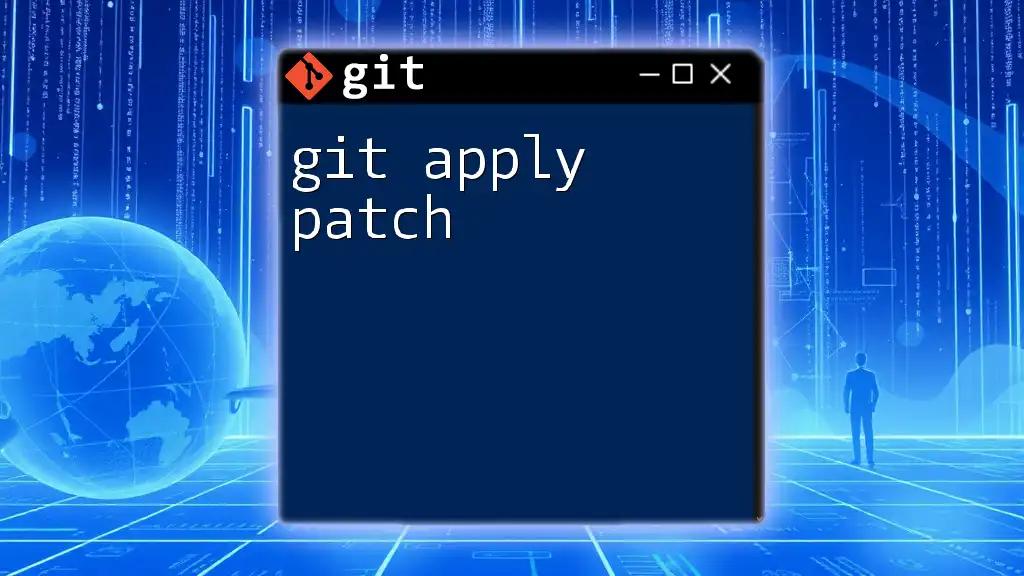
Advanced Git Techniques for GraphQL Projects
Using Git Hooks
Git hooks can automate tasks in your development process. The pre-commit hook is particularly useful for running tests or linting your code before a commit is finalized. Create a `.git/hooks/pre-commit` file with executable permissions and add your linting command:
#!/bin/sh
npm run lint
This ensures that every time you commit, your code is checked for style issues, maintaining code quality.
Submodules and Monorepos
When managing multiple GraphQL services in a larger architecture, Git submodules can assist in maintaining versioning across projects. Submodules allow you to include repositories within your repository, providing modular management of GraphQL services.
Alternatively, consider using monorepos for maintaining multiple relevant projects under a single repository. This organization can ease dependency management for GraphQL services.
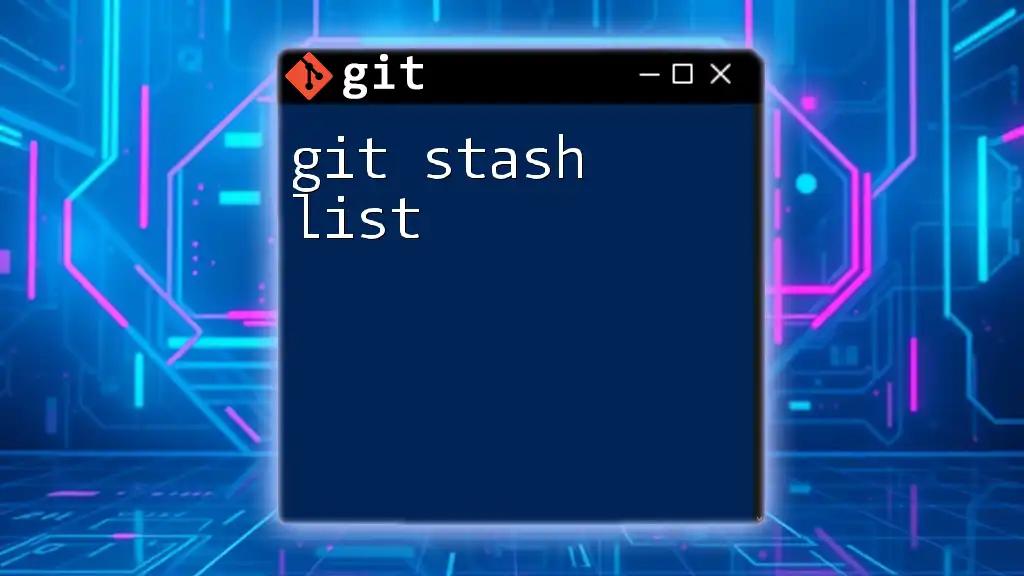
Troubleshooting Common Issues
Version Control Conflicts
Merge conflicts often arise in collaborative projects. They present an opportunity for teams to improve communication and collaboration. In case you encounter conflicts while working with GraphQL projects, Git will output notifications specifying the conflicting files:
Automatic merge failed; fix conflicts and then commit the result.
Manually resolve the conflicts in your IDE, stage the resolved files, and complete the merge with a precise commit message.
Best Practices for Version Control
To keep a clean Git history, enforce consistency by committing changes frequently and writing well-descriptive commit messages. Regularly update your branches to incorporate the latest changes from the main branch, further enhancing integration.
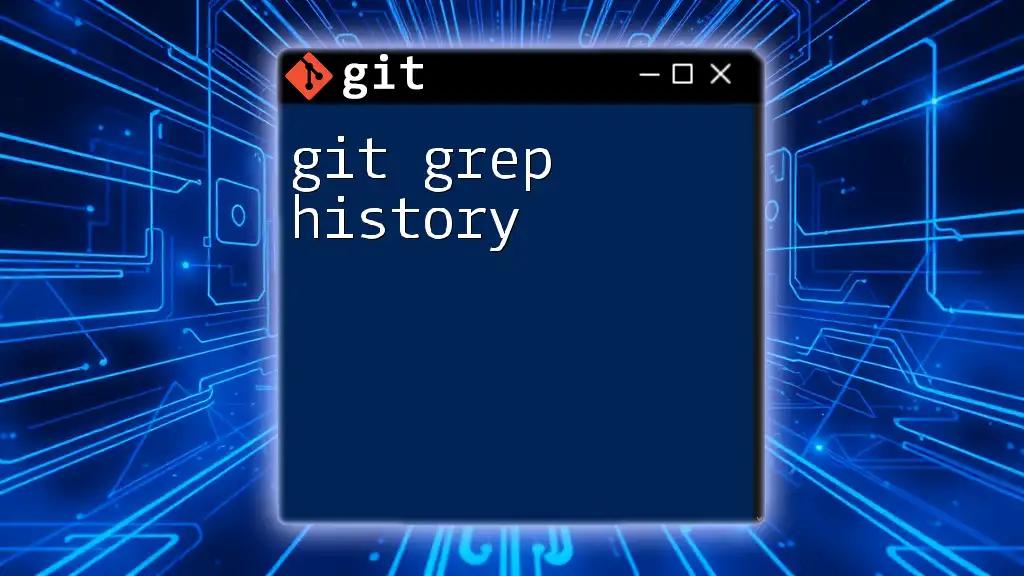
Conclusion
By leveraging Git for your GraphQL development process, you streamline version control while allowing your projects to grow sustainably. Understanding and utilizing Git commands effectively will enhance your productivity and maintain the integrity of your GraphQL API. Now, it's time to apply these concepts and elevate your development workflow!
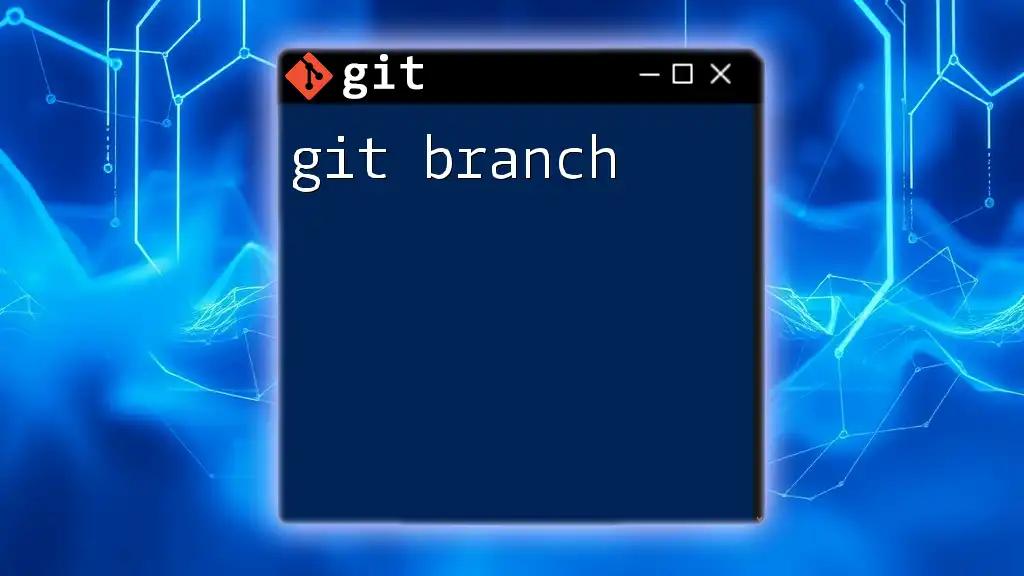
Additional Resources
To continue your journey in mastering Git and GraphQL, consider checking the official documentation for both technologies, along with community forums where you can exchange ideas and troubleshoot issues with fellow developers.