A "Git Crash Course" provides a fast and efficient introduction to essential Git commands for beginners, enabling them to quickly navigate version control.
# Initialize a new Git repository
git init
# Check the status of your repository
git status
# Add changes to the staging area
git add .
# Commit the changes
git commit -m "Initial commit"
# Push changes to the remote repository
git push origin main
Getting Started with Git
Installing Git
To begin your journey in understanding Git, the first step is to install it on your machine. Git is available for multiple platforms:
- Windows: Download the Git installer from the [official Git website](https://git-scm.com/download/win) and follow the installation prompts.
- macOS: You can install Git via Homebrew using the command:
brew install git
- Linux: For Debian/Ubuntu, run:
sudo apt-get install git
After installation, it’s crucial to set up your user information. This step ensures that your commits are attributed to the correct author. Use the following commands to configure your Git username and email:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Understanding Basic Concepts
Before diving into Git commands, it's essential to grasp some foundational concepts.
Repositories are where your project’s files and history are stored. They can exist locally on your machine or remotely on platforms like GitHub.
Commits capture the state of your project at specific points in time. Each commit is essentially a snapshot of your files. Learning to create meaningful commit messages is vital for tracking changes effectively.
Branches allow you to develop features or fixes in isolation from your main codebase. This feature enables you to work on different versions of your project simultaneously without interference.
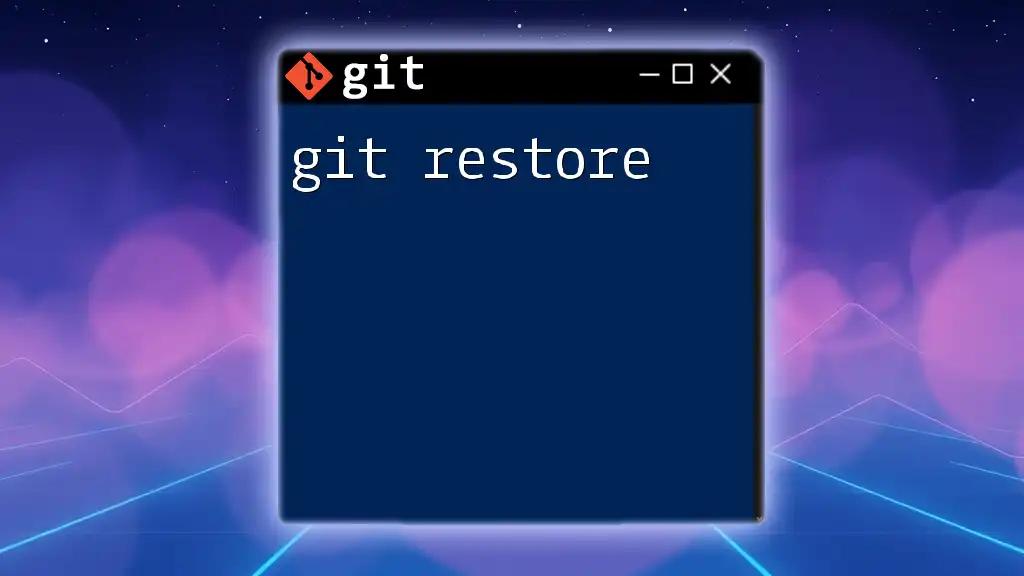
Basic Git Commands
Creating a Repository
To start a new project, you need to create a repository. You can initialize a new repository with the command:
git init my-project
This command sets up a new Git repository in the `my-project` directory, allowing you to start tracking changes immediately.
If you want to work on an existing project, you can clone it using:
git clone https://github.com/user/repository.git
Cloning downloads the entire repository, including its history, to your local machine.
Tracking Changes
Once you have a repository, the next step is to track your changes.
To start tracking a file, you add it to the staging area with:
git add filename
This command tells Git that you want to include this file in your next commit.
To actually save your changes to the repository, use:
git commit -m "Your commit message"
A well-worded commit message helps others understand what changes have been made and why.
Viewing Status and Logs
To check the current status of your files and see which changes are staged, use:
git status
This command lets you know about modified files, files staged for commit, and untracked files.
You can view the history of your commits with:
git log
This command provides a detailed list of commits made in the repository, along with their timestamps and messages.
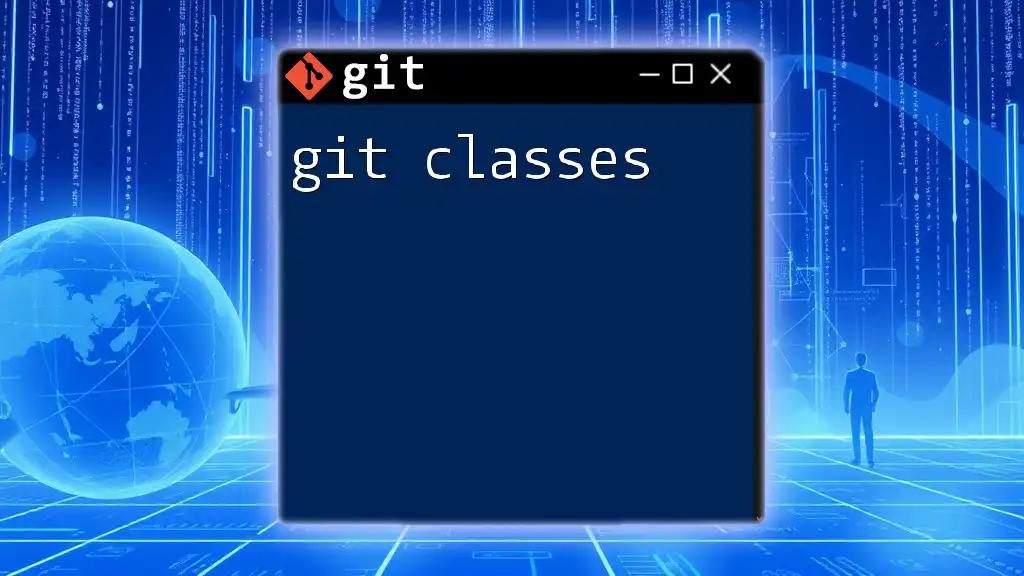
Working with Branches
Creating and Switching Branches
Git’s power lies significantly in its branching capabilities. To create a new branch, use:
git branch new-branch
This command creates a new branch named "new-branch".
To switch to the newly created branch, use:
git checkout new-branch
Now, you’re working in that branch, allowing you to make changes without affecting the main codebase.
Merging Branches
Once you’ve implemented your changes in a branch, you may want to merge those changes back into the main branch. First, switch to the branch you want to merge into (often `main`):
git checkout main
Then, run:
git merge new-branch
This command merges the changes from "new-branch" into the current branch. If there are no conflicts, the merge will proceed smoothly.
Deleting a Branch
After merging, it’s a good practice to clean up by deleting unused branches. You can do this with:
git branch -d branch-name
This command safely removes the specified branch, but only if it has been merged.
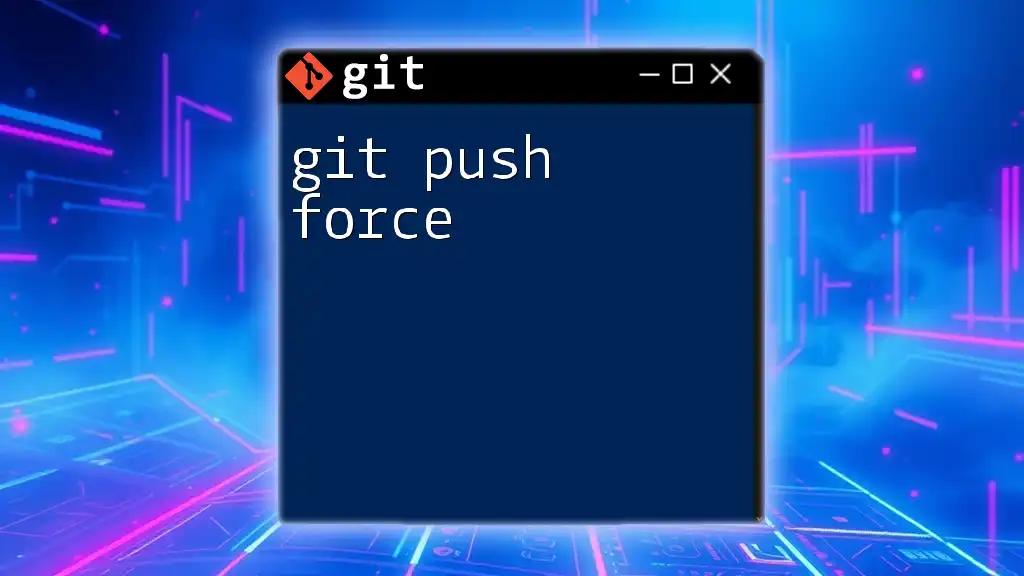
Remote Repositories
Connecting to a Remote Repository
To collaborate with others, you need to connect your local repository to a remote one. Use the following command to add a remote:
git remote add origin https://github.com/user/repository.git
This command names the remote `"origin"`, which is a conventional name for the main remote version of your repository.
Pushing Changes
To send your local commits to the remote repository, you use:
git push origin main
This command uploads your changes, making them available to others working on the same project.
Pulling Changes
If others have made changes to the remote repository, you can fetch and merge those changes into your local repository using:
git pull origin main
This command ensures your local repository remains up to date with any alterations made by collaborators.
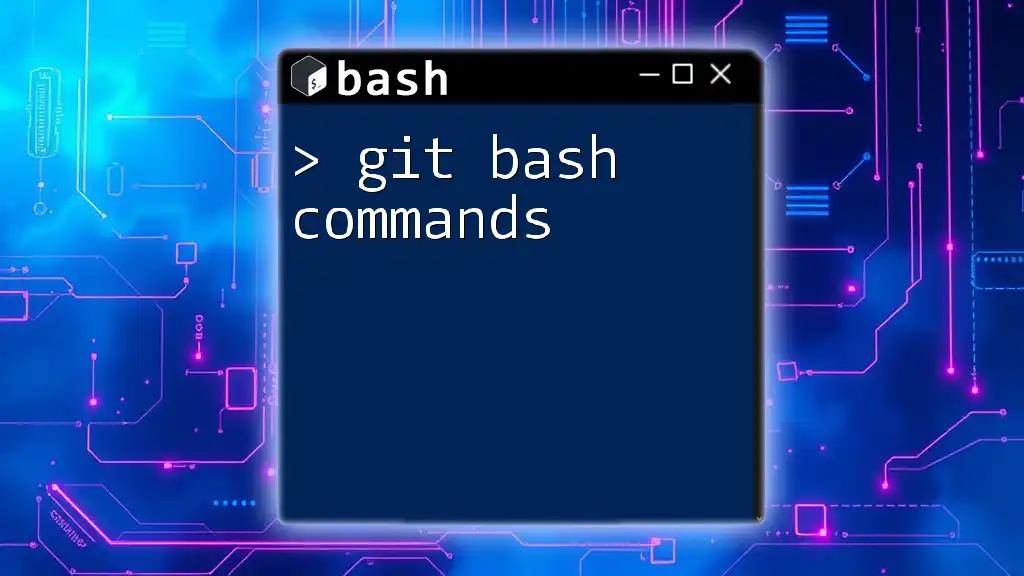
Advanced Git Concepts
Handling Merge Conflicts
When merging branches, conflicts may arise if changes overlap. If this occurs, Git will indicate conflicts in affected files. To resolve them, first check the status:
git status
This command will show which files are in conflict. Open those files, edit as necessary to resolve the conflict, and then mark them as resolved by staging them:
git add filename
Finally, commit the resolved merge:
git commit
Using Tags
Tags serve as markers in your project's history, often used to denote releases or important milestones. To create a tag, you can use:
git tag -a v1.0 -m "Version 1.0"
This command tags the latest commit with "v1.0" along with a meaningful message.
Reverting Changes
If you want to undo a previous commit, you can use:
git revert commit-hash
This command creates a new commit that reverses the changes made in the specified commit, allowing you to maintain a clear project history.
Stashing Changes
If you need to switch branches but aren't ready to commit changes, you can stash your changes temporarily with:
git stash
This command saves your current modifications and cleans your working directory. To retrieve your stashed changes later, use:
git stash pop
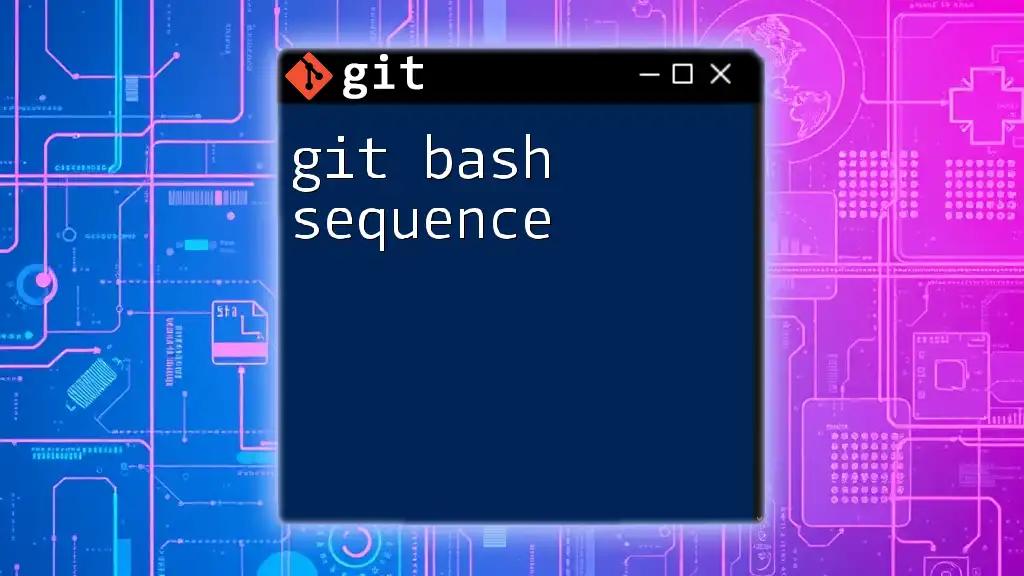
Best Practices
Commit Messages
Writing effective commit messages is crucial. A good format to follow includes a brief summary (50 characters or less) followed by a more detailed description if necessary. For example:
Fix login bug
- Corrected null pointer exception during user login
Branching Strategies
Adopting a reliable branching strategy can improve your workflow. Popular models include Git Flow and Feature Branching, which foster organized collaboration and streamlined development.
Regular Backups
Pushing your changes to a remote repository regularly not only backs up your work but also enhances collaboration. Avoid working for extended periods without syncing your changes, as this can lead to complications when integrating later.
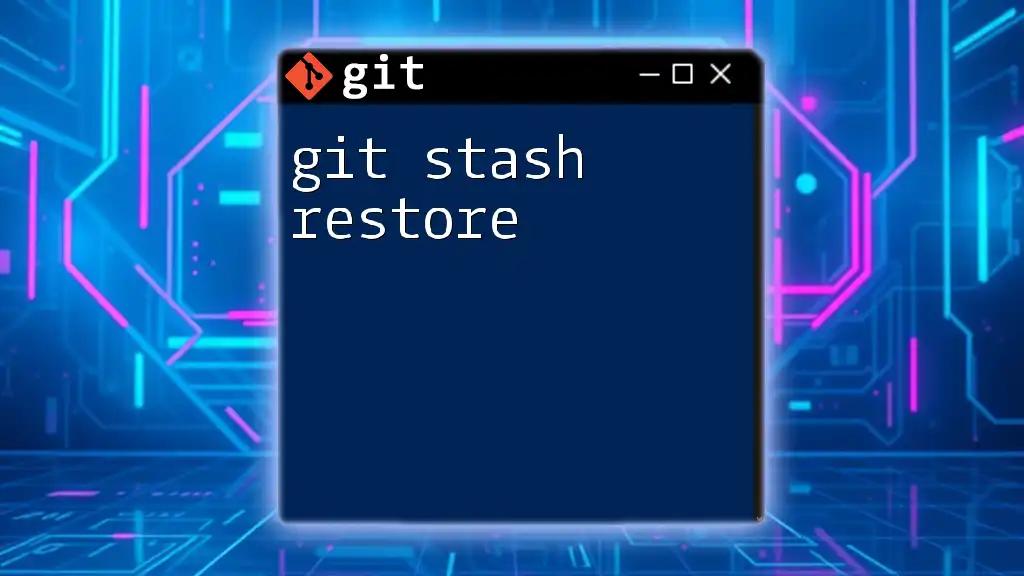
Conclusion
In completing this git crash course, you've obtained a solid foundation in Git essentials, from creating repositories to effectively branching and collaborating with others. Understanding Git empowers you to manage your projects efficiently and makes you a more effective team member.
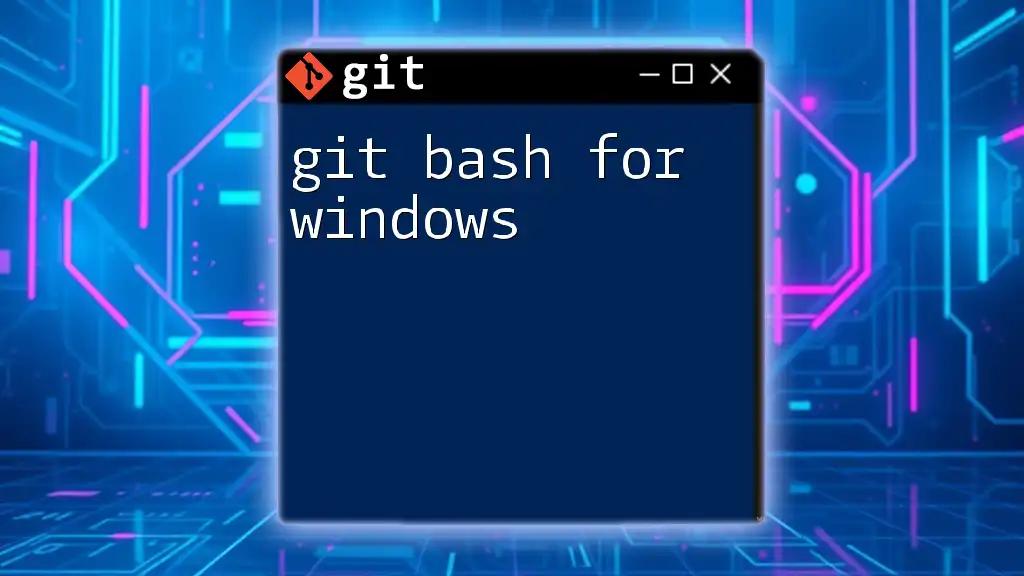
Call to Action
If you’re keen to deepen your understanding of Git, consider joining our comprehensive courses where you can explore advanced topics and best practices in more detail. Let's master Git together!