To stash a single file while leaving others unchanged, you can use the following command which specifies the file you want to stash.
git stash push -m "Stashing one file" path/to/your/file
Understanding Git Stash
What is Git Stash?
Git stash is a command that allows developers to save changes made to their working directory temporarily without committing them. This is particularly useful when you need to switch branches or pull in updates but want to retain your current uncommitted changes. By using the stash, you can come back to your work at any point without losing your progress.
Why Use Stash for One File?
Stashing a single file can be beneficial in various scenarios. For example, if you are working on a feature but encounter a critical issue in another part of your codebase, you may want to stash just the file associated with your feature implementation. This prevents accidental inclusion in a later commit and helps maintain a clean history.
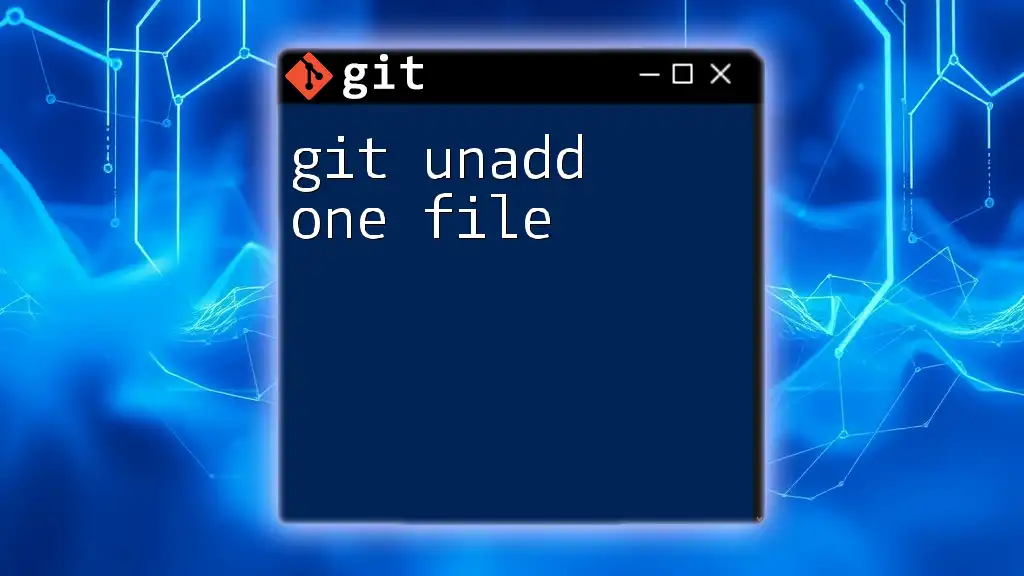
Preparing to Use `git stash`
Ensure Your Repository is Clean
Before stashing a file, it is essential to ensure that your repository is clean or has the necessary changes staged for commit. You can do this by running:
git status
This command will show you the current state of the working directory and the staging area. A clean repository means no untracked files or changes that haven't been staged.
Identify the File to Stash
Once you've confirmed a clean state, locate the modifications you wish to stash. You can use the `git diff` command to see unstaged changes. This command provides a view of all modifications made to tracked files:
git diff
This will help you identify precisely which files and lines of code you wish to stash.
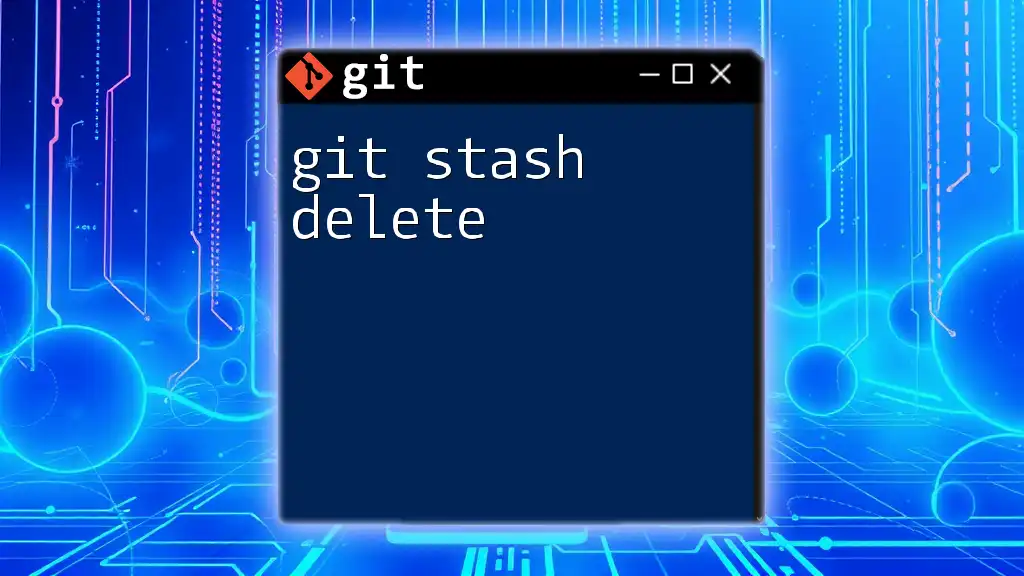
Using `git stash` for a Single File
Step-by-Step Guide to Stash One File
Selecting the File
Once you have identified the file you want to stash, you can use the command:
git stash push <file-path>
This command stashes only the specified file, leaving all other changes in your working directory intact.
Example of Stashing One File
Consider a case where you’ve made changes to a file named `example.txt` located in a folder called `docs`. To stash this specific file, you would run:
git stash push docs/example.txt
After executing this command, Git will save the changes from `example.txt` and leave your working directory clean.
Checking the Stash List
To see what you have stashed, you can list your stashes with:
git stash list
This command provides an indexed list of stashed changes. Each entry is referred to as `stash@{n}`, facilitating easy reference for applying or managing them.
Example Output and Explanation
The output might look something like this:
stash@{0}: WIP on master: 3a1b2c3 Your commit message here
This indicates that the most recent stash was created on the `master` branch and includes a summary of your last commit, giving context about the changes.
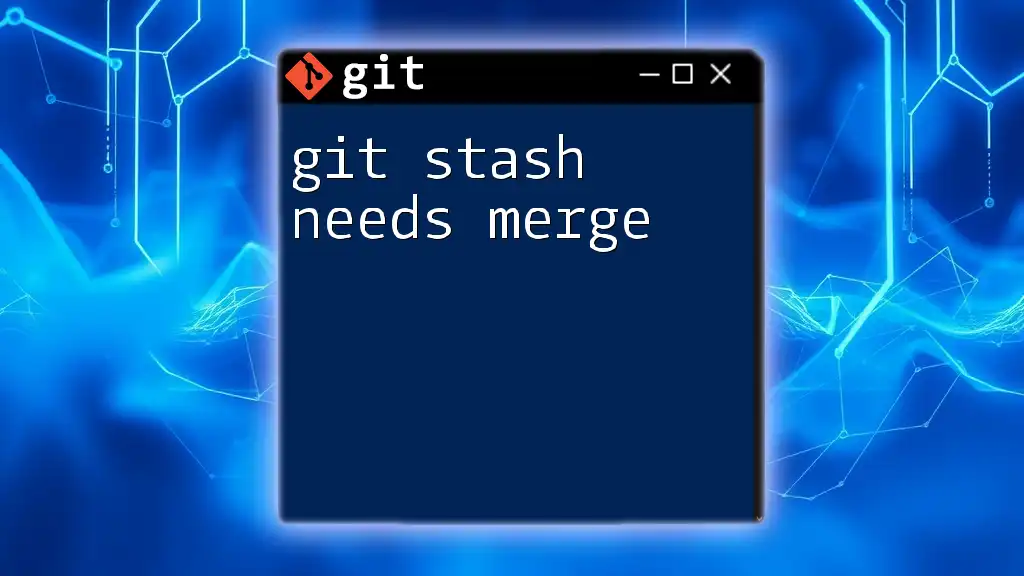
Applying Stashed Changes back to Your Work
Fetching Your Stashed File
When you're ready to bring back the stashed changes, you can apply the stash to your current branch with:
git stash apply stash@{n}
Example: Applying the Stash
If you've stashed a file and wish to apply the most recent stash, you would execute:
git stash apply stash@{0}
Executing this command brings back the changes from `example.txt` into your current working directory. After the application, consider verifying any conflicts or changes.
Resolving Conflicts
Sometimes, applying stashed changes may lead to conflicts. This typically occurs if the file you stashed has undergone modifications in the meantime. Git will highlight the conflicting areas in your file. It’s essential to resolve these conflicts manually, ensuring that your code reflects the intended logic before finalizing the changes.
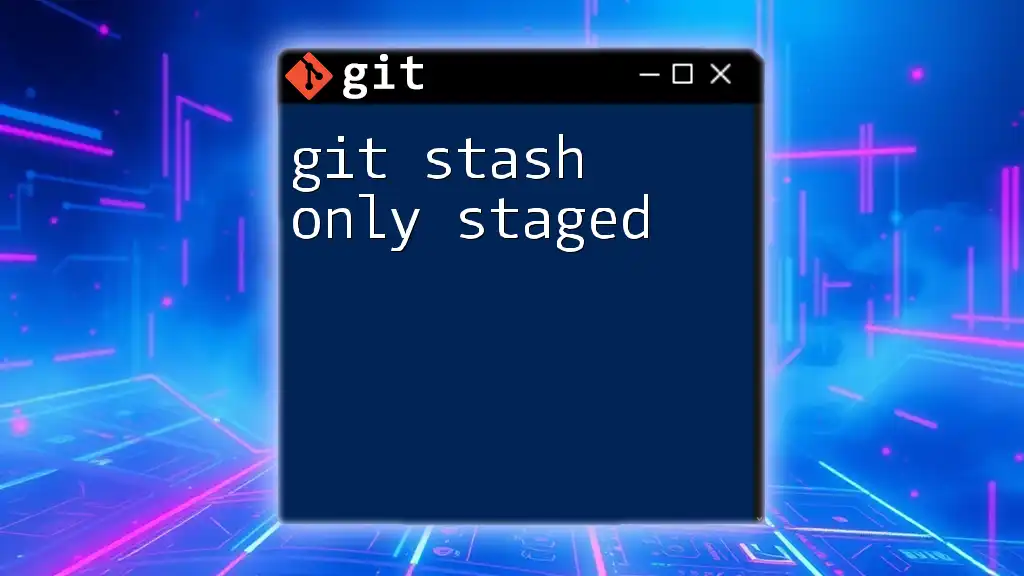
Managing Your Stash
Listing Stored Stashes
To view the contents of your stash in detail, use:
git stash show -p stash@{n}
This command provides a patch of the specific stash, enabling you to review the changes in context.
Deleting a Stashed Entry
Once you have applied the stashed changes and no longer need the stash, you can delete it to keep your stash list manageable. Use the following command:
git stash drop stash@{n}
If you prefer to remove all stashes at once, utilize:
git stash clear
This command erases all stashed entries, freeing you from clutter.
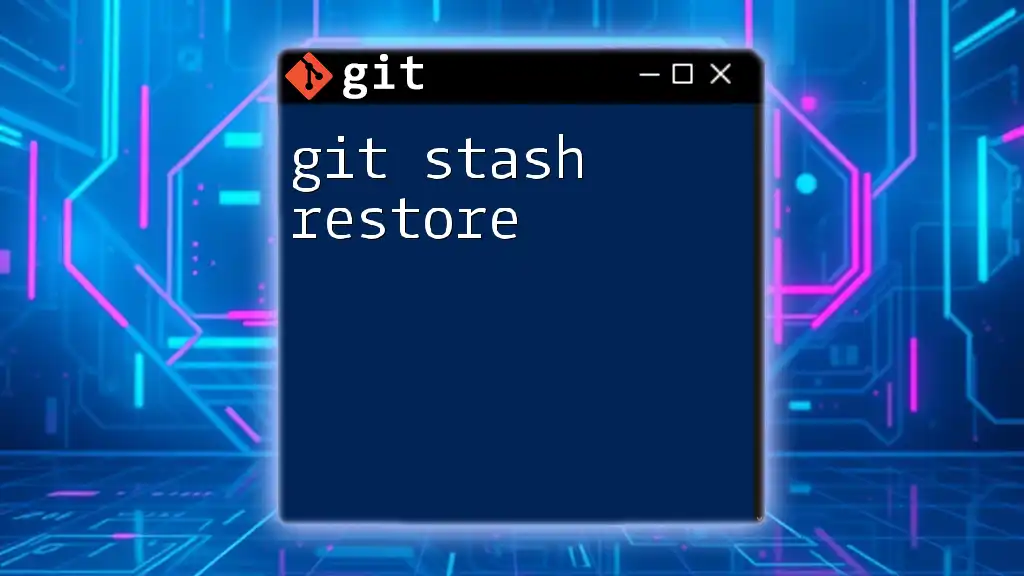
Best Practices for Using `git stash`
When to Stash Changes
It’s crucial to know when it’s appropriate to use git stash. Stash changes when you are in the middle of a specific task but need to switch contexts, like addressing a bug or reviewing urgent client feedback. It’s a way to pause your progress without committing incomplete work.
Keeping Your Stashes Organized
Maintaining clarity in stashes can prevent confusion later. Use meaningful messages when stashing your changes to provide context:
git stash push -m "Fixing the header layout bug" docs/example.txt
This approach lends clarity to your stashes and improves workflow efficiency.
Avoiding Common Mistakes
Some common pitfalls involve failing to apply or delete stashes promptly, which can lead to lost work or clutter. Frequently review your stashes, and remember that stashed changes are not permanent. If not applied or dropped, they can be lost if the stash list exceeds the limit.
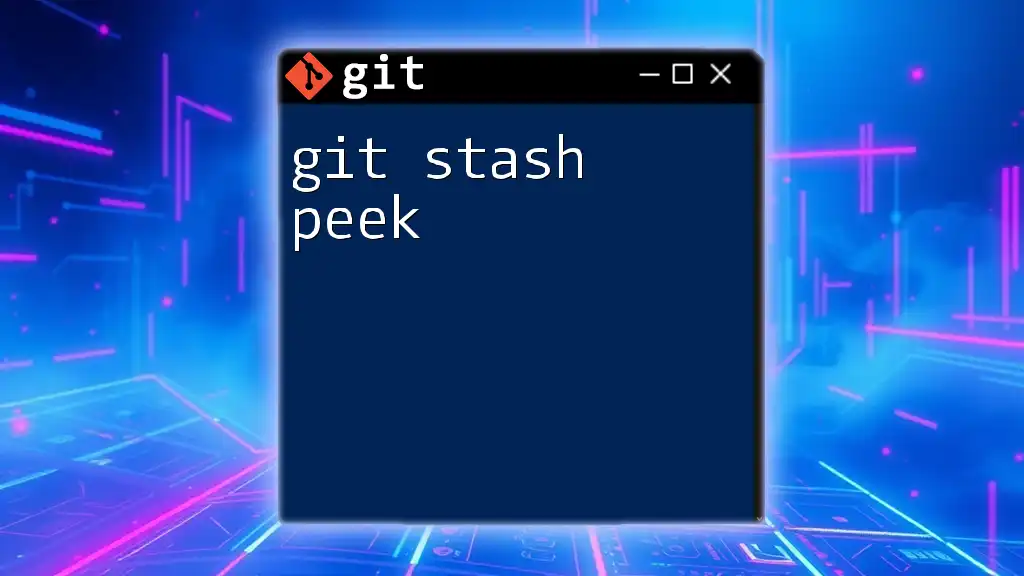
Conclusion
Recap of Using `git stash` for One File
By utilizing `git stash`, you can effectively manage file-specific changes in your workflow. The ability to stash one file offers flexibility, allowing you to maintain a clean working directory while addressing urgent issues.
Encouragement to Practice
Mastering the use of git commands, especially stashing, will enhance your proficiency in version control systems. Embrace the tool and practice frequently to solidify your understanding.
Additional Resources
For further reading and a deep dive into git functionalities, consult the official Git documentation. Additionally, consider online courses that provide guided practice and community support.