When you encounter the "git stash needs merge" message, it indicates that you have stashed changes that conflict with the current branch, preventing Git from applying the stashed modifications until the conflicts are resolved.
Here's how you can check the status and resolve the conflicts:
git status
git stash pop
# Resolve any merge conflicts
git add <file>
git commit -m "Resolved merge conflicts"
What is Git Stash?
Git Stash is a powerful command in Git that allows you to temporarily save changes you've made to your working directory, without committing them. This feature is especially useful when you're working on a feature or a bug fix and suddenly need to switch contexts, such as pulling the latest changes from a remote repository or changing branches to address a critical issue. By stashing, you can keep your workspace clean and avoid messy commits.
Basic Stash Commands
Here are some basic commands related to `git stash`:
git stash # Stash your changes
git stash list # List all stashes
git stash apply # Apply the stashed changes
git stash pop # Apply and remove the stash
Key Benefits:
- Workspace Cleanliness: Keeps your working directory free from half-finished work.
- Flexibility: Allows you to switch branches and contexts without losing progress on your current work.
- Quick Recovery: Easily retrieve saved stashed changes when you're ready to integrate them back into your workflow.
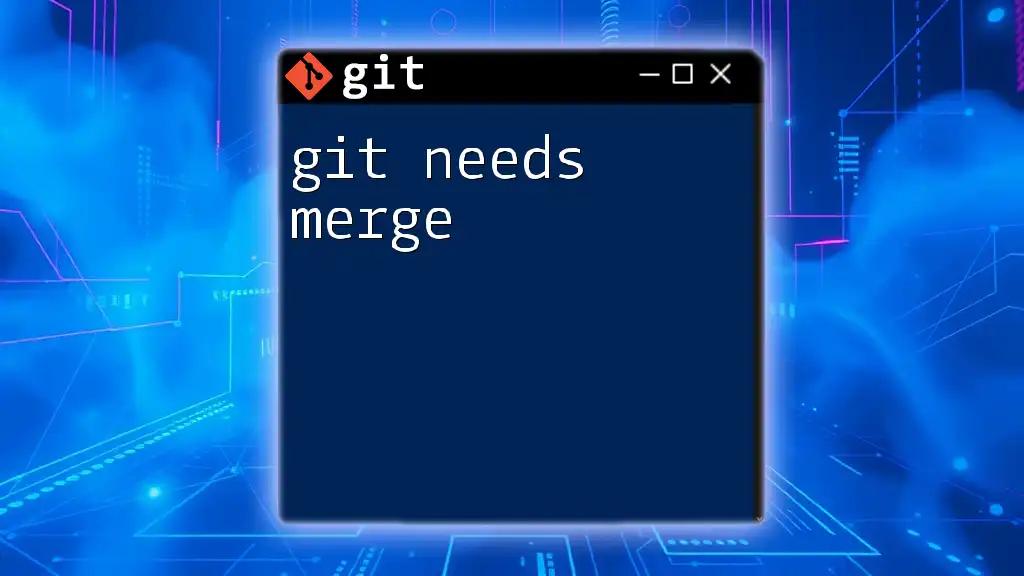
Common Scenarios for "git stash needs merge"
Understanding when you might encounter the message "git stash needs merge" is crucial to navigating your Git workflow efficiently. This message typically appears when you try to apply stashed changes onto a branch that has diverged and has conflicting changes.
When Does This Message Occur?
The "needs merge" error most commonly appears when you attempt to apply `git stash` while on a branch that has changes conflicting with those in your stash. For instance, if you stashed changes while on a feature branch and then made different commits on the target branch, applying the stash will likely trigger merge conflicts.
Example Scenario
Consider the following sequence of commands:
git checkout feature-branch # Switch to your feature branch
git stash # Stash local changes
git checkout main # Switch to main branch
git pull origin main # Update local main with remote changes
git checkout feature-branch # Return to feature branch
git stash apply # Attempt to apply stashed changes
If there are conflicting changes in the code between your stashed work and what currently exists in `feature-branch`, you will see the "git stash needs merge" message. This means Git requires your attention to resolve the conflicts before you can proceed.
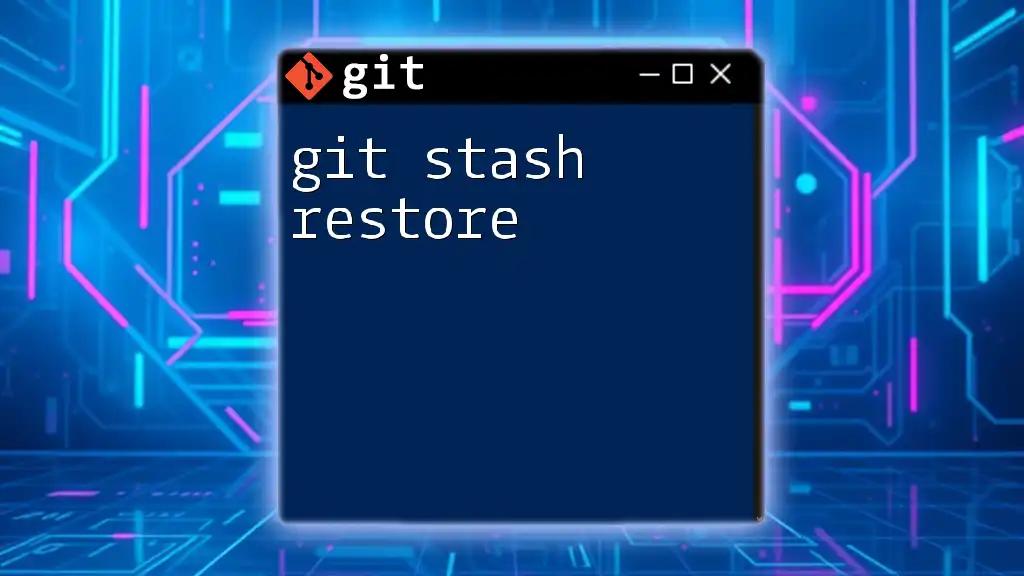
Understanding Merge Conflicts in Git
What is a Merge Conflict?
A merge conflict occurs when Git cannot automatically resolve differences in code between two branches. This typically happens when two branches have made changes to the same line of code or when one branch deletes a file that another branch has modified. The introduction of your stash may involve trying to merge incoming changes that conflict with your stashed changes, leading to the "git stash needs merge" scenario.
Common Conflict Indicators
When a merge conflict arises, Git provides several visual indicators. You can identify conflicted files by running:
git status
In the output, conflicted files will be marked as "both modified." Inside the files, conflict markers will appear where you need to resolve the differences:
<<<<<<< HEAD
// code from the current branch
=======
// code from the stash
>>>>>>> stash
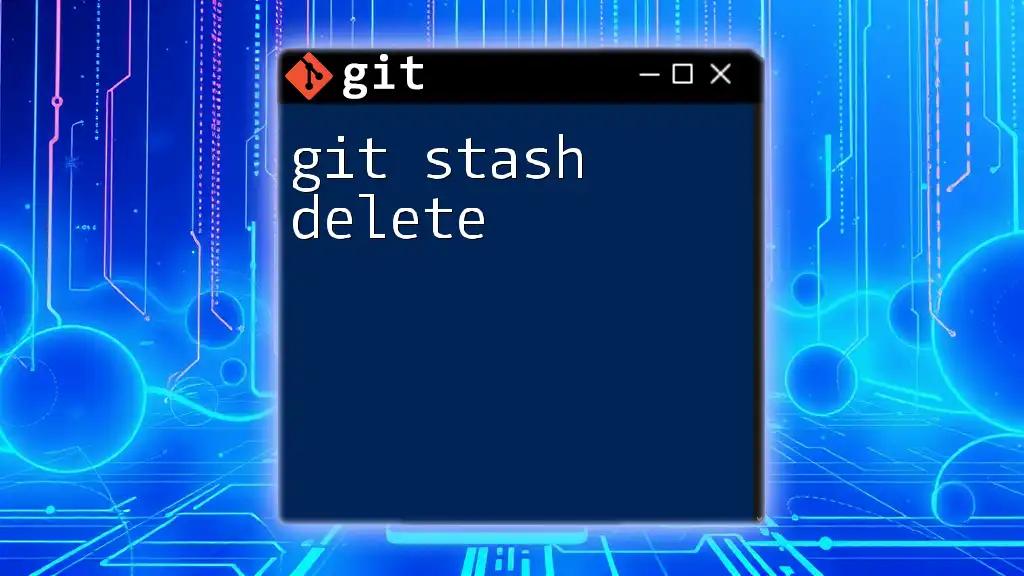
Resolving "git stash needs merge"
Step-by-Step Resolution Guide
To effectively resolve the "git stash needs merge" situation, follow these steps:
- Identify Conflicted Files
After trying to apply your stash, first run:
git status
This command will help you identify which files are in conflict.
- Open and Resolve Conflicted Files
Open each conflicted file in your code editor. Review the conflicting sections and decide how to merge the changes. Remove the conflict markers (<<<<<<<, =======, >>>>>>>) and integrate the code as necessary.
- Mark the Conflict as Resolved
Once you've resolved the conflicts, stage your changes:
git add <file>
Finally, commit the merge resolution:
git commit
Using Interactive Tools
In addition to command-line resolution, several GUI tools can simplify the process of resolving conflicts visually. Tools like GitHub Desktop or Sourcetree not only provide a clear visual representation of changes but often include built-in conflict resolution features. Using these tools can improve your workflow, especially if you're working with complex merges.
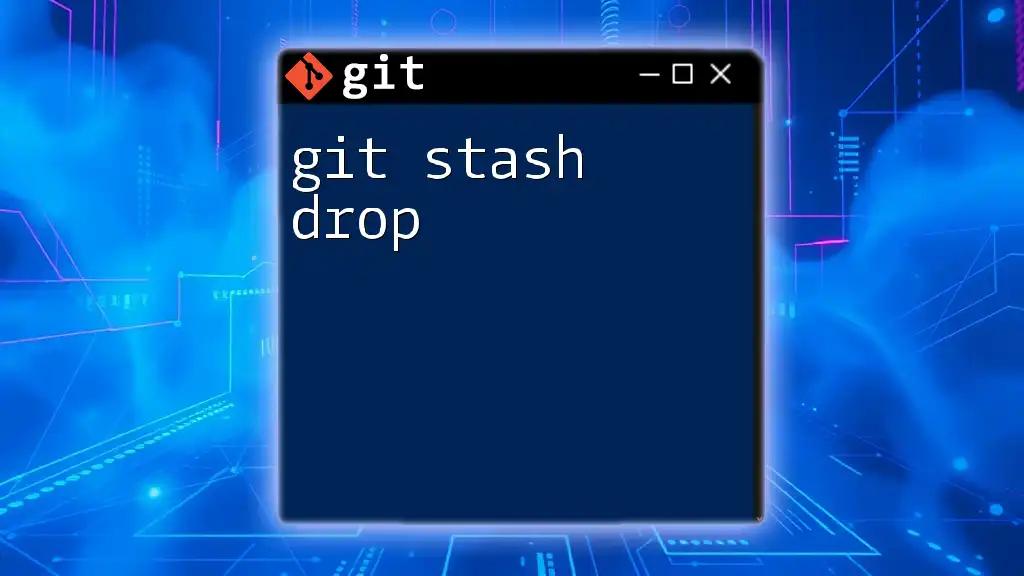
Best Practices for Using Git Stash
When to Use `git stash`
While `git stash` can be a lifesaver, it's crucial to use it judiciously. Stash should primarily be used when you need to halt your current work due to higher-priority tasks. Frequent stashing can lead to a convoluted history that makes it difficult to track changes over time.
Avoiding "git stash needs merge"
To minimize the likelihood of encountering the "git stash needs merge" message, consider the following strategies:
- Communicate with Your Team: Ensure everyone is aware of significant changes before branching out.
- Sync Regularly: Frequently pull changes from the remote repository to minimize divergence.
- Plan Ahead: Before starting major work on a feature branch, check the state of the main branch and make sure it's up to date.
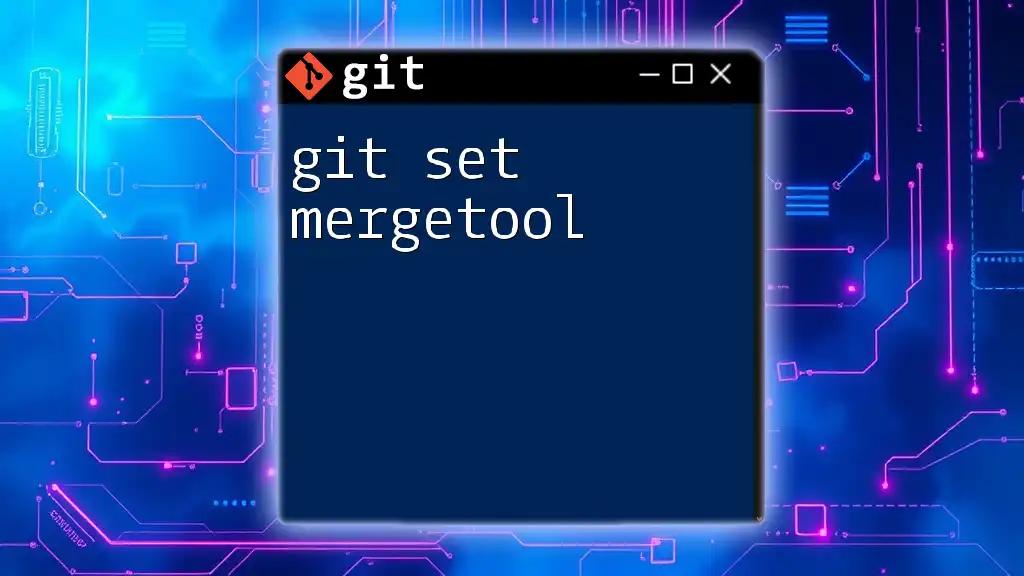
Conclusion
Understanding the implications of "git stash needs merge" is essential for maintaining a smooth Git workflow. By effectively using `git stash`, resolving conflicts, and adhering to best practices in version control, you can enhance your productivity and collaboration. Donโt shy away from practicing these techniques regularly to build confidence in using Git's advanced features.
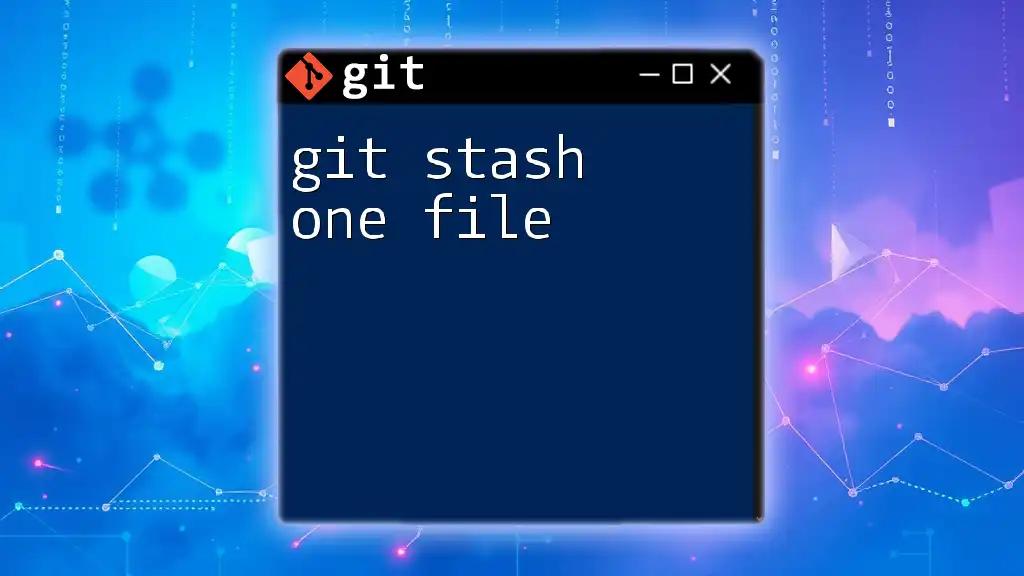
Additional Resources
For further exploration, check the official [Git documentation](https://git-scm.com/doc) and seek out tutorials focused on advanced Git commands and conflict resolution techniques. This will complement your skills and boost your Git proficiency.

FAQs
-
What should I do if I forget what I've stashed?
- Use `git stash list` to review your stashed changes.
-
Can I create multiple stashes?
- Yes, you can stash changes multiple times; each stash will be added to the stash list.
-
Is there a way to delete a stash?
- You can use `git stash drop stash@{index}` to remove a specific stash or `git stash clear` to remove all stashes.
By mastering these concepts and commands, you will be better equipped to handle the intricacies of Git, including the common issues that arise with stashing and merging changes.