The `git unmerge` command is not a standard Git command, but if you want to revert a merge situation and go back to a previous state, you can use the `git reset` command to undo the merge by resetting to a commit before the merge occurred.
git reset --hard HEAD^
What is `git unmerge`?
In Git, unmerging refers to the process of reverting or undoing a merge that has already occurred. This can become essential when you mistakenly merge the wrong branch or encounter conflicts that take too much time to resolve. Understanding how to effectively unmerge is crucial for maintaining a clean and functional Git history.
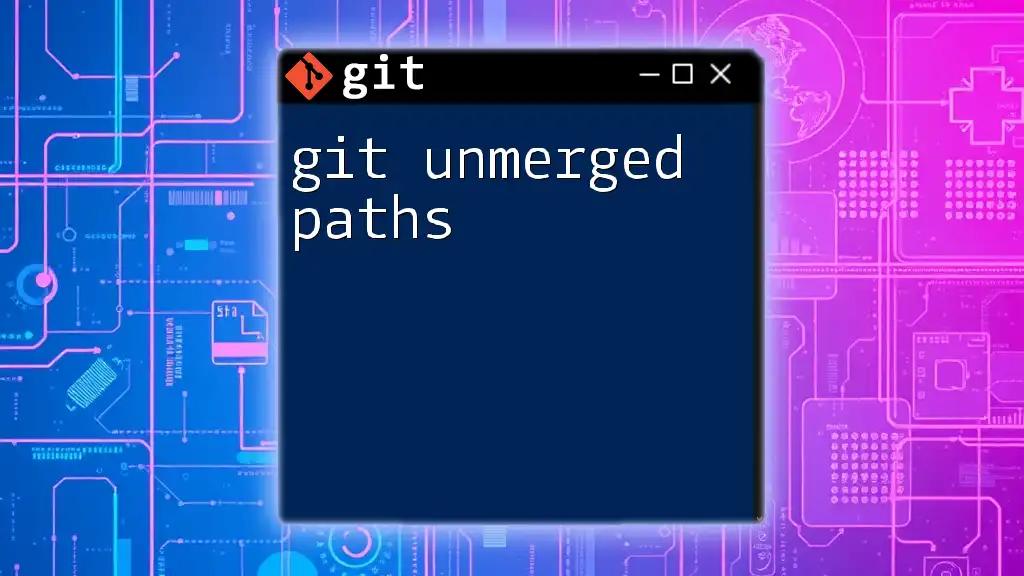
Why You Might Need to Unmerge
There are several scenarios when you may find it necessary to unmerge:
-
Unintentional Merges: Merging the wrong branch can lead to an undesirable state in your codebase. For instance, if you accidentally merge a feature branch that hasn't been tested yet, immediately realizing the mistake would prompt the need for unmerging.
-
Dealing with Merge Conflicts: Sometimes, during a merge, conflicts arise that are too complex to resolve on the fly. If conflicts are more challenging than anticipated, deciding to backtrack can be prudent.
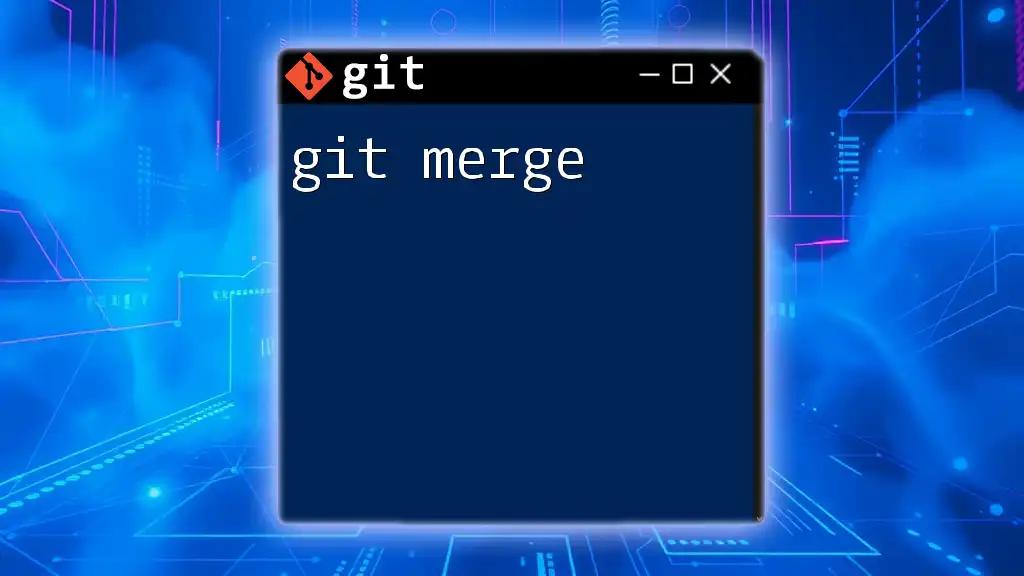
Basics of Merging in Git
The `git merge` command is used to integrate changes from one branch into another. When executed, Git takes the commits from the specified branch and combines them with the current branch, creating a merge commit if there are no conflicts.
An example of a typical merge command is as follows:
git merge feature-branch
This command would take changes from the `feature-branch` and merge them into your current branch.
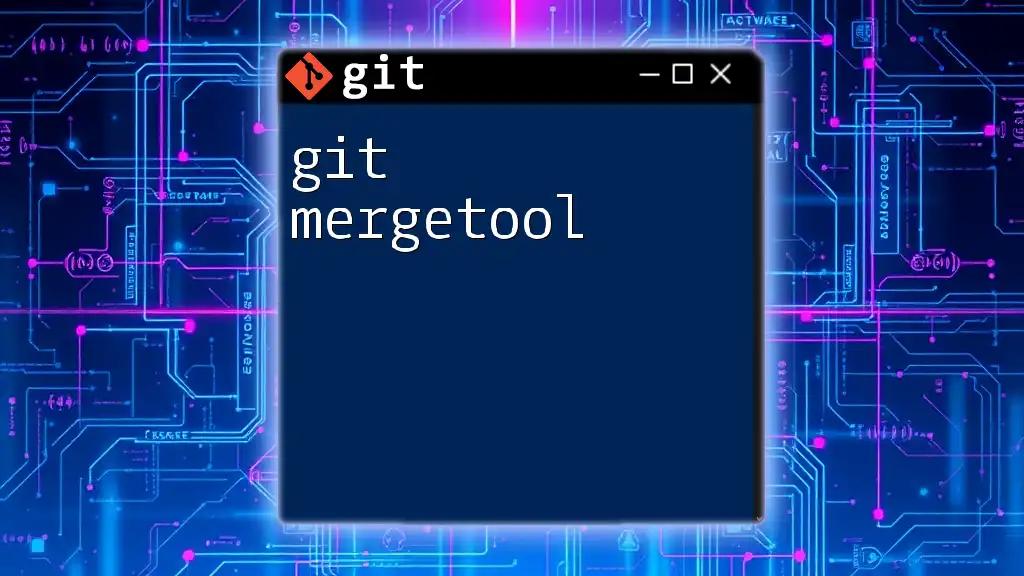
What Happens During a Merge?
During the merging process, Git performs several key tasks:
-
Fast-forward Merge: If the current branch has no new commits since diverging from the merged branch, Git simply moves the pointer forward, with no extra commit created.
-
Three-way Merge: In cases where both branches have diverged, Git will perform a three-way merge (using the common ancestor of the branches). This leads to the creation of a new merge commit that captures the newly integrated changes.
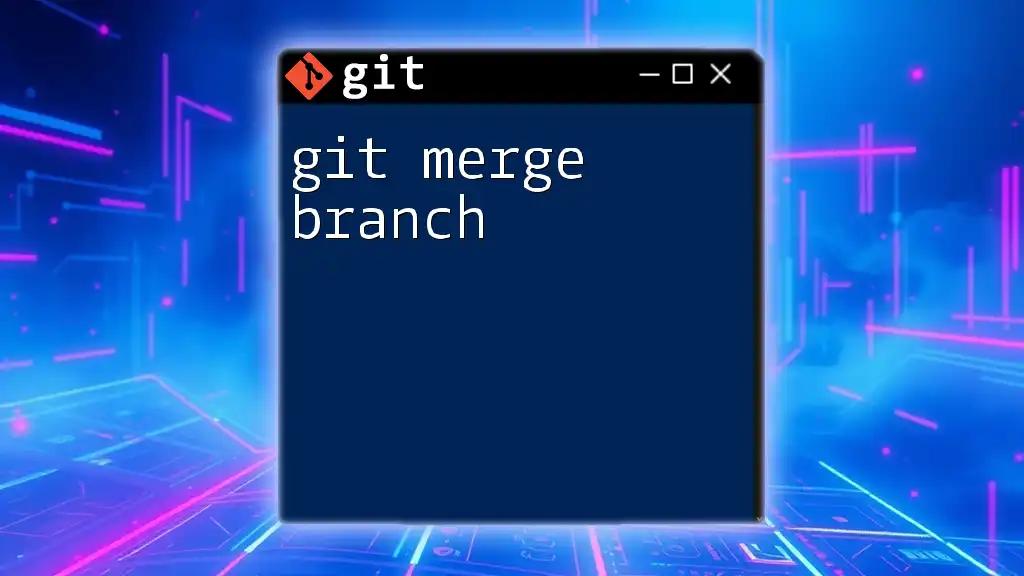
The Need for Unmerging
Now that you understand the basics of merging, the significance of unmerging becomes clearer. It provides an option to rectify any mistakes that were made during the merge process.
Unintentional Merges can create a chaotic repository state, making it vital to know how to revert those changes.
Dealing with Merge Conflicts can sometimes lead to the desire to start fresh from a known good state. Learning to unmerge empowers you to take control over your version history.
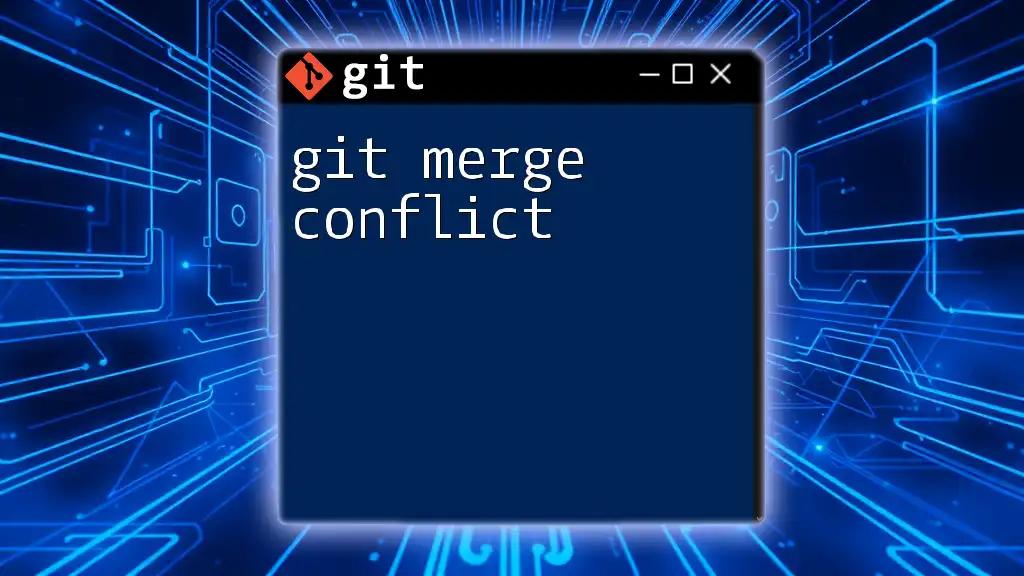
Methods of Unmerging
Using `git reset`
Overview of `git reset`
The `git reset` command allows you to reset your current branch to a specific state. Depending on the level of reset you choose (soft, mixed, hard), it can either preserve changes in the staging area or discard them entirely.
How to Unmerge Using Git Reset
To unmerge using `git reset`, follow these steps:
-
Identify the Merge Commit Hash: You need the commit hash of the merge you want to revert. You can find this using:
git log
-
Execute Git Reset: Choose the appropriate reset type based on your needs. For instance:
git reset --hard HEAD~1
This command will reset your current branch to the state it was one commit before the current head, effectively undoing the last merge.
Using `git revert`
What is Git Revert?
Unlike `reset`, which changes the commit history, `git revert` creates a new commit that undoes the changes of a previous commit. This approach is particularly useful when you want to maintain the history of changes while still reversing the effects of a merge.
Unmerging with Git Revert
To unmerge using `git revert`:
-
Find the Merge Commit Hash: Again, use the `git log` to find the correct hash.
-
Revert the Specific Merge: Use the following command to revert the merge commit:
git revert -m 1 <merge_commit_hash>
Here, `-m 1` specifies to revert the first parent of the merge commit, effectively undoing the changes brought in by the merged branch.
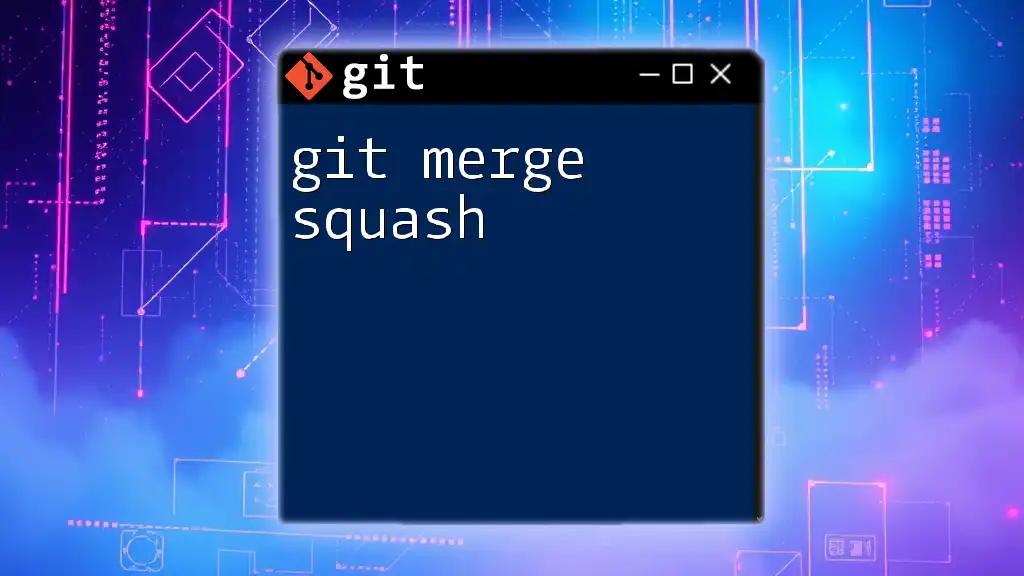
Verifying the Unmerge
Once you've performed either a reset or revert, it's vital to verify that the unmerge was successful.
You can use:
git log
to review your commit history and confirm that the changes have been appropriately reverted. Additionally, use `git status` to check your working directory and staging area for any inconsistencies.
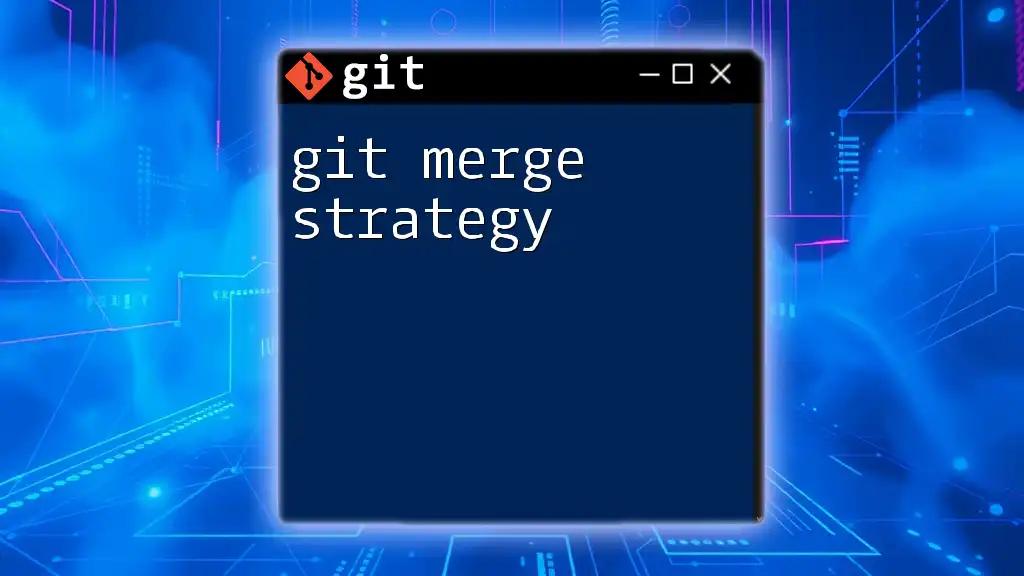
Recovering from Errors
Even seasoned developers can make missteps. Here are common mistakes and how to avoid them during the unmerging process:
-
Mistakenly Resetting the Wrong Branch: Always double-check which branch you are on before executing a reset command.
-
Permanent Loss of Changes: Using `git reset --hard` can lead to permanent loss of changes unless those changes are saved in another branch or have been committed previously. Consider using `git stash` to temporarily save changes before reset.
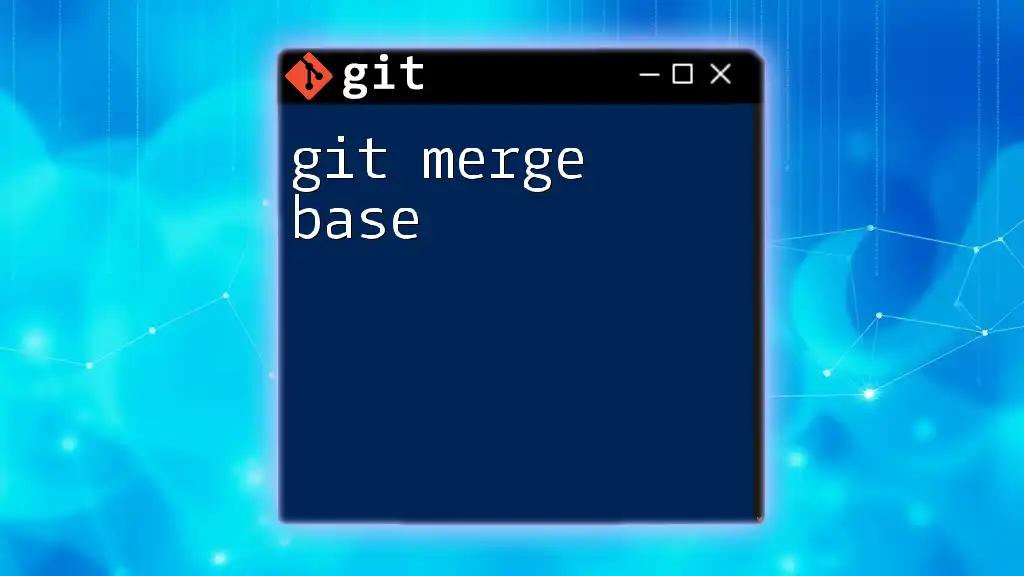
Best Practices for Unmerging
Prevention Strategies
To avoid needing to unmerge in the future, consider these best practices:
- Conduct thorough code reviews before merging.
- Utilize feature branches for experimental or unfinished work, limiting the chances of merging directly into a stable branch.
Using Branches Effectively
Branches are your best allies in Git. Keeping your work separate makes it easier to manage changes and mitigates the disruptive effects of unintentional merges. Always ensure you’re working in the correct branch context.
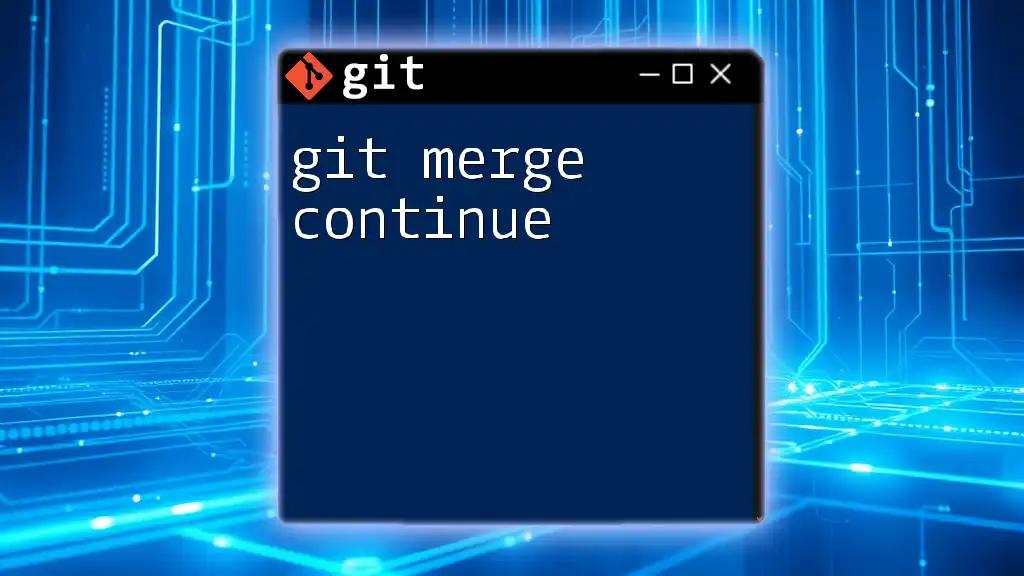
Recap of Key Takeaways
Understanding `git unmerge` equips you with the tools to backtrack when mistakes are made during the merging process. Whether you choose to reset or revert, knowing how to manage your commit history can save you significant headaches.
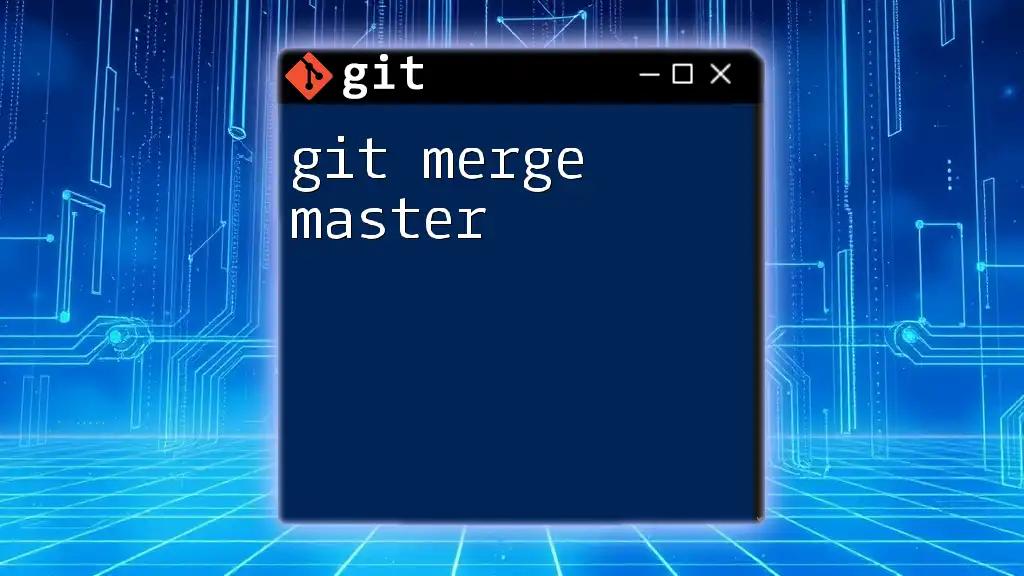
Further Reading
For deeper insights into Git commands and workflows, consult the official Git documentation. Being part of communities or forums can also provide additional support as you refine your Git skills.
By practicing these commands on a test repository, you can build confidence in using Git effectively, ensuring a smoother workflow in your projects.