The `git rev-parse` command is used to convert Git references (like branch names or tags) into SHA-1 hashes or to examine the current state of the repository in a concise manner.
git rev-parse HEAD
What is `git rev-parse`?
`git rev-parse` is a command in Git that serves as a key tool for parsing and resolving references to Git objects. This command acts as a bridge between user-friendly references like branch names and commit hashes to the actual SHA-1 object identifiers that Git uses under the hood. Understanding how to navigate this command opens up powerful capabilities, particularly when working in complex repositories.
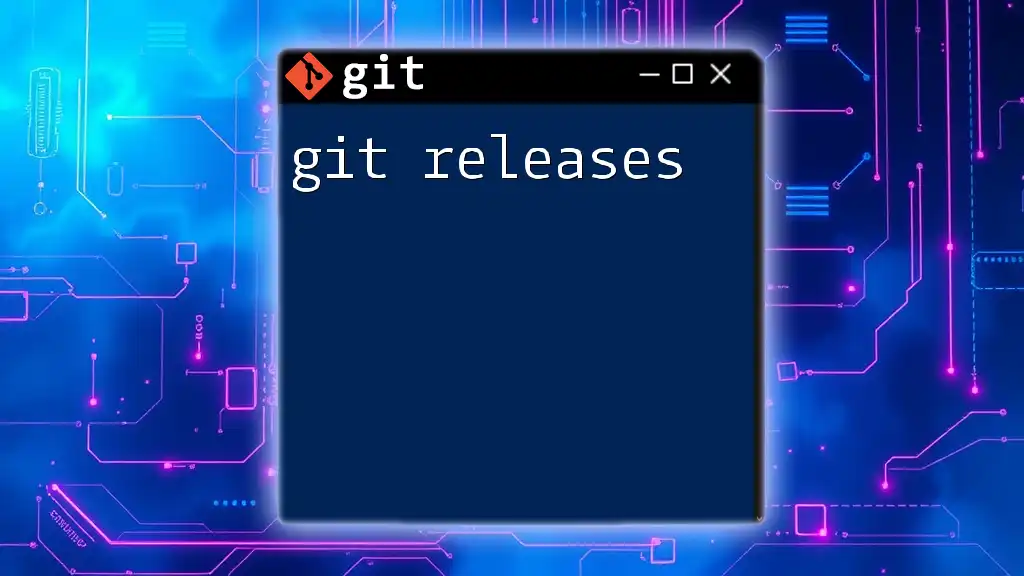
When to Use `git rev-parse`
Being adept with `git rev-parse` becomes vital when you need to resolves object identifiers during scripts, automation processes, or simply verifying the current state of your repository. For instance, if you are coordinating merges or identifying changes between branches, being able to resolve these references accurately saves you from potential pitfalls.
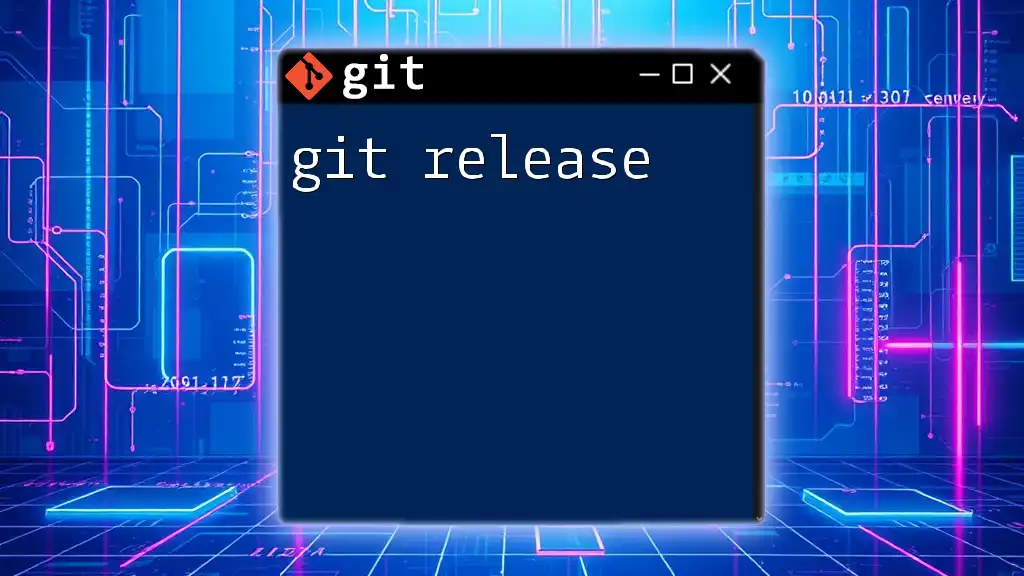
Understanding Git Object Identifiers
What are Git Objects?
Every piece of information in a Git repository is represented as objects. These include:
- Commits: Points in the project's history.
- Trees: Directories that contain commits and other trees or files.
- Blobs: The actual file data.
Each object has a unique identifier—a SHA-1 hash—that makes it easy to reference. The persistent nature of Git means that once these objects are created, they remain in the repository's history.
The Role of References in Git
In addition to objects, Git uses references to help manage and navigate those objects. Some key references include:
- Branches: These point to the latest commit in a sequence of development.
- Tags: Mark significant points in history, such as releases.
- HEAD: A special reference that indicates the current commit your working directory is based on.
Understanding how these references tie back to object identifiers is crucial for effective version control.
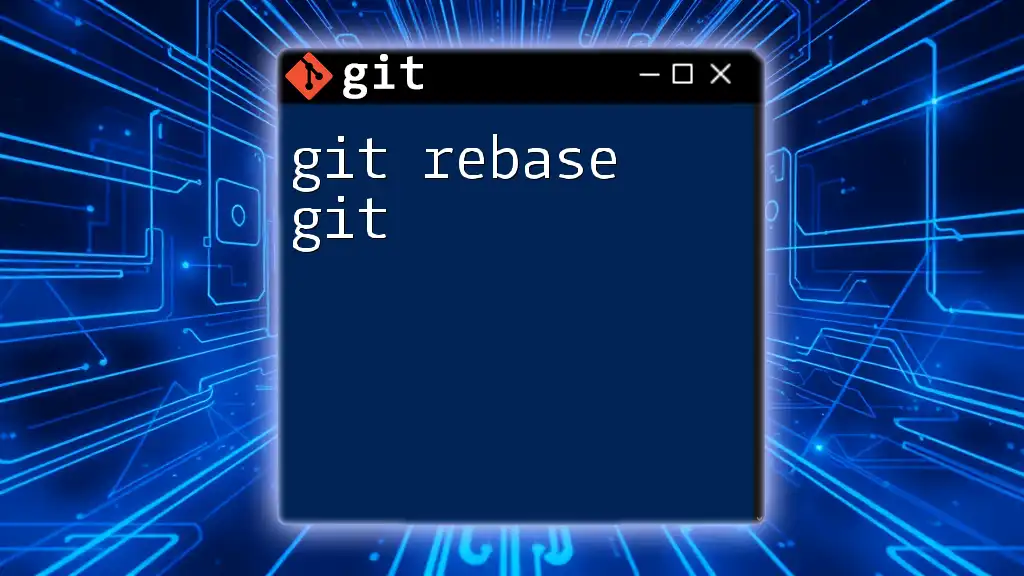
Basic Usage of `git rev-parse`
Syntax and Parameters
The basic syntax for `git rev-parse` is as follows:
git rev-parse <options> <args>
Common Use Cases
One primary use for `git rev-parse` is to resolve a commit hash from a branch name:
git rev-parse main
This will return the SHA-1 hash corresponding to the current commit in the `main` branch, allowing you to reference it in other commands that require an object ID.
Another common scenario is converting relative references, such as:
git rev-parse HEAD~1
This command translates `HEAD~1` (the commit one step before the current HEAD) into the specific commit hash it points to.
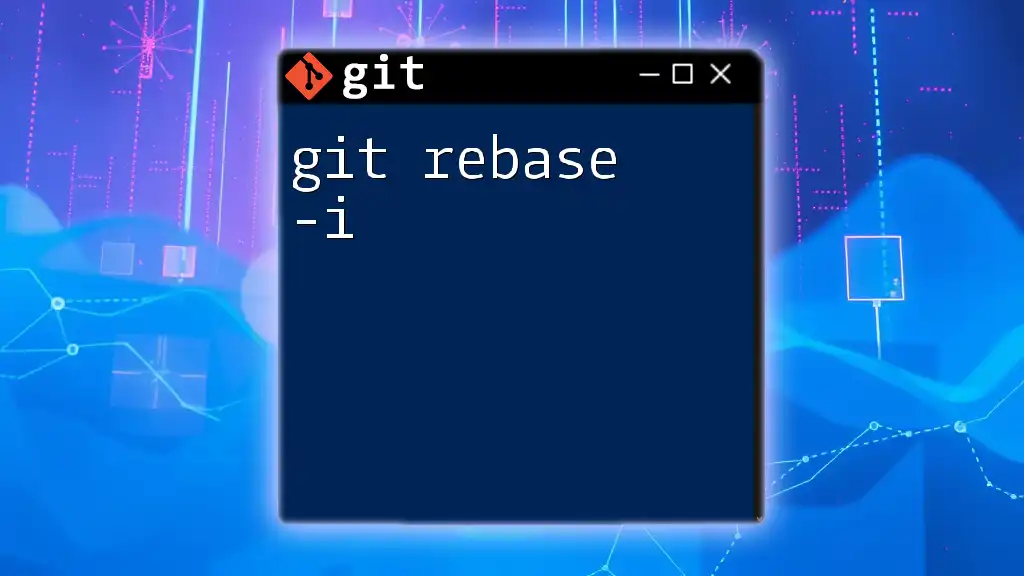
Key Features of `git rev-parse`
Retrieving the Current Branch Name
Using `git rev-parse`, you can ascertain the name of the currently checked-out branch. This is crucial for avoiding confusion in environments where multiple branches are in play:
git rev-parse --abbrev-ref HEAD
This command delivers the current branch name, enabling your scripts or commands to adapt accordingly.
Extracting Configuration Values
Another powerful aspect of `git rev-parse` is its ability to extract specific configuration values. For instance, you can find the root directory of your Git repository with:
git rev-parse --show-toplevel
This is particularly useful in scripts where you need to operate relative to your repository's root, ensuring paths are consistently referenced.
Getting the Object ID for a Tag or Commit
If you want to retrieve the object ID for a specific tag or commit, you can use:
git rev-parse v1.0.0
This will return the SHA-1 hash associated with that specific tagged version, making it easier to refer back to it later.
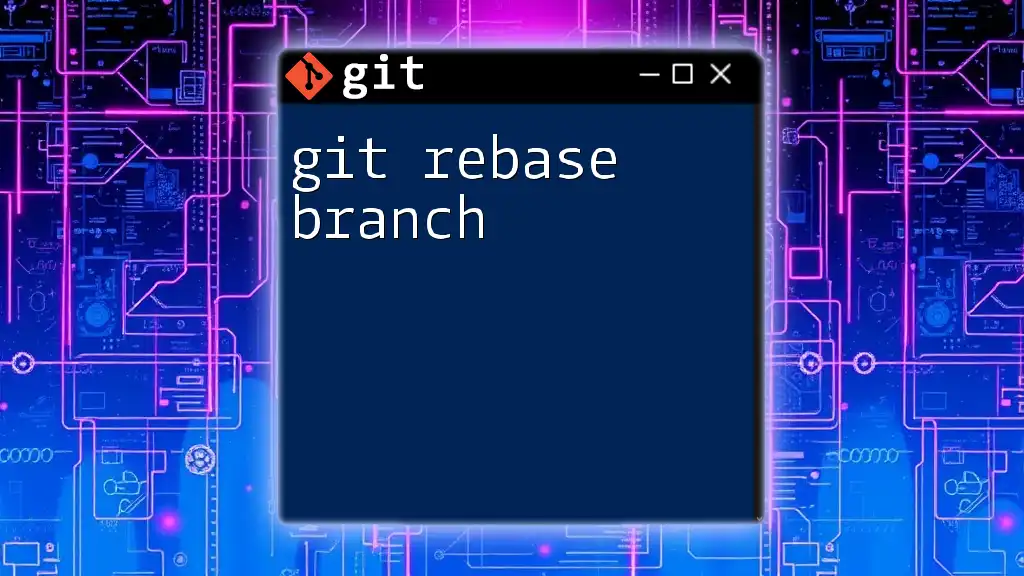
Advanced Usage of `git rev-parse`
Exploring Options and Flags
`git rev-parse` boasts several available options that expand its utility:
-
`--short`: Produce a shorter, abbreviated object name that is easier to read or display.
Example:
git rev-parse --short HEAD
-
`--verify`: Validate that a reference exists.
Example:
git rev-parse --verify HEAD
-
`--git-dir`: Identify the path to the .git directory which is key for scripts looking to access Git's underlying data directly.
Example:
git rev-parse --git-dir
Each of these options can simplify tasks and make interactions with your repository more efficient.
Integrating `git rev-parse` in Scripts
To maximize the functionality of `git rev-parse`, consider using it in personalized Git scripts. For example, you could write a script to automate deployment processes:
#!/bin/bash
# Get the current commit hash
COMMIT_HASH=$(git rev-parse HEAD)
# Deploy the current commit
echo "Deploying commit $COMMIT_HASH..."
# Deployment commands go here
This kind of automation saves time and reduces errors, especially in complex workflows.
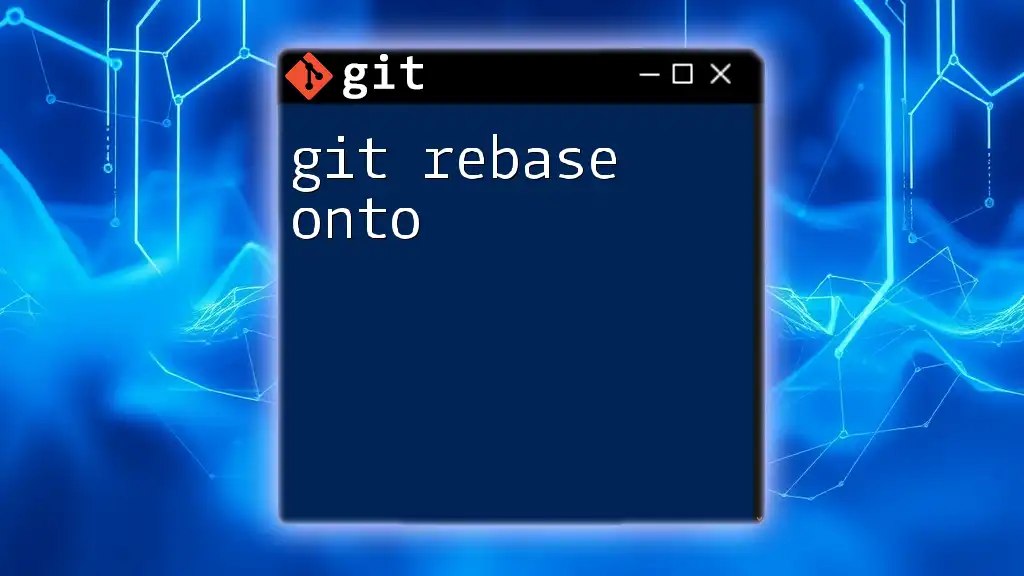
Common Issues and Troubleshooting
Understanding Errors with `git rev-parse`
Even though `git rev-parse` is useful, it may throw common error messages that can perplex new users. Examples include:
-
"fatal: ambiguous argument": Indicates the command was unable to interpret a command line argument correctly, often due to whitespace issues or invalid references.
-
"fatal: bad revision": This occurs when a specified reference does not exist, signaling a need to double-check the spelling or existence of the referenced commit or branch.
How to Resolve Reference Issues
When troubleshooting reference issues, a good first step is to ensure you are in the correct repository and that your branches are up to date. Use commands like `git fetch` to synchronize with any remote changes and verify the state of your local references.
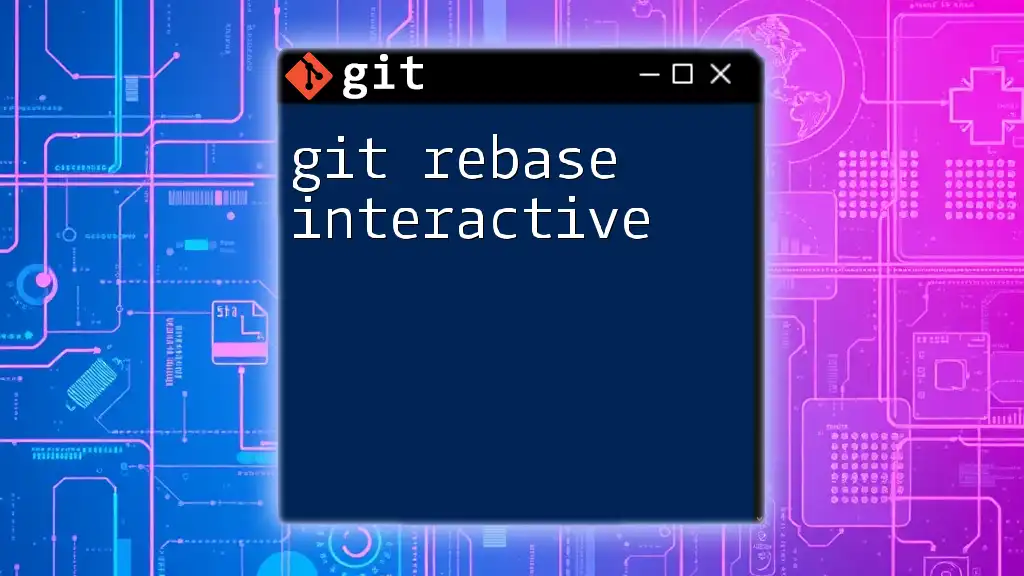
Conclusion
The `git rev-parse` command is an indispensable tool in the Git toolkit. Its ability to resolve references and provide clarity in the midst of a version-controlled environment can significantly enhance your workflow. Its utilization in scripts can automate tasks and reduce manual errors, streamlining your development process.
As you become familiar with the nuances of `git rev-parse`, consider experimenting with its capabilities in your projects. This practice not only reinforces your understanding but also positions you to leverage the full power of Git effectively.
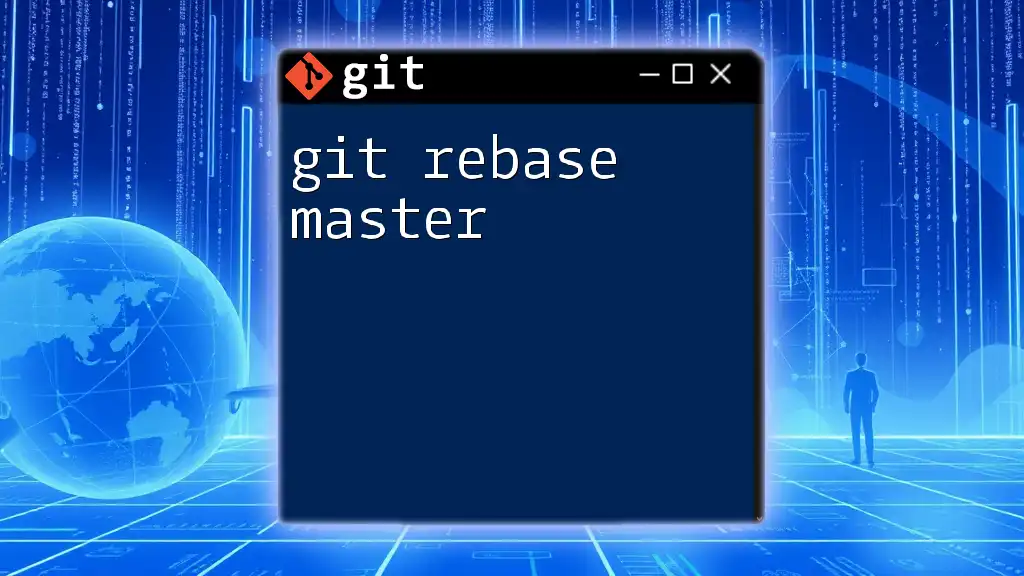
Additional Resources
For those eager to expand their knowledge, several resources are available. The official Git documentation provides a comprehensive overview and detailed explanations of `git rev-parse` and related commands.
Consider engaging in practical exercises where you can apply the concepts discussed above and reinforce your learning through real-world application. A deeper understanding of `git rev-parse` and how it fits into the Git ecosystem is bound to elevate your version control skills to new heights.