`git apply` is a command used to apply a patch file to your current working directory, which can modify files and stage changes for the next commit.
Here's an example of how to use it:
git apply my_patch.patch
Understanding Patches
What is a Patch?
A patch is a file that contains differences between two versions of files, typically created to update or fix code. In the context of Git, patches serve as a way to share changes made in the codebase without having to push the entire modified branch.
Patches are created using the `git diff` command and represent a set of changes in a format that can be easily applied to a working directory.
Example: Creating a Simple Patch To create a patch file of the uncommitted changes in your working directory, execute the following command:
git diff > my_changes.patch
This command generates a file named `my_changes.patch` that contains the differences in the form of a patch.
When to Use Patches
Using patches is advantageous in several situations, such as when you want to:
- Share specific changes with collaborators without pushing to the main repository.
- Apply changes from an external source, like an email or another repository.
- Manage code in environments where direct commits cannot be made.
By using patches, you simplify the version control process and maintain the integrity of your codebase.
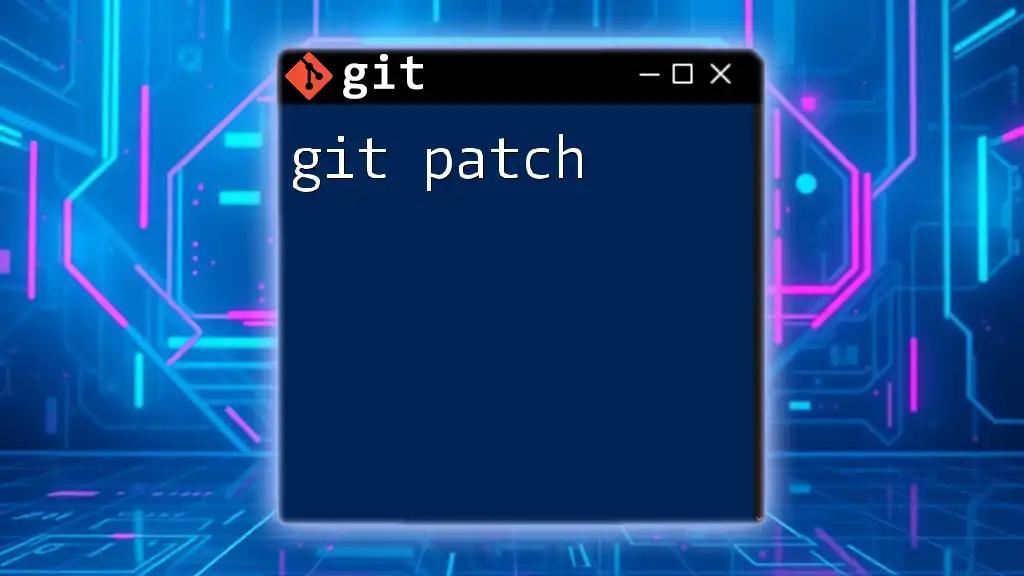
Overview of `git apply` Command
Syntax of `git apply`
The basic syntax for using `git apply` is straightforward and consists of the command followed by specific options and the patch file path:
git apply [OPTIONS] <PATCH>
This flexibility allows users to modify how the patch is applied based on the context of their workflow.
Common Options
Understanding the options available with `git apply` can significantly enhance its effectiveness:
-
`--check`: Runs a dry run to check if the patch can be applied without issues.
Example:
git apply --check my_changes.patch
-
`--reverse`: Allows you to apply the patch in reverse, effectively undoing the changes.
-
`--reject`: If certain parts of a patch cannot be applied cleanly, this option generates `.rej` files where conflicts occur.
-
`-p` or `--strip`: Modifies the path prefix for the files in the patch, which can be useful if the patch does not align perfectly with your project structure.
Knowing the appropriate options lowers the chance of errors while applying patches and helps manage your code more effectively.
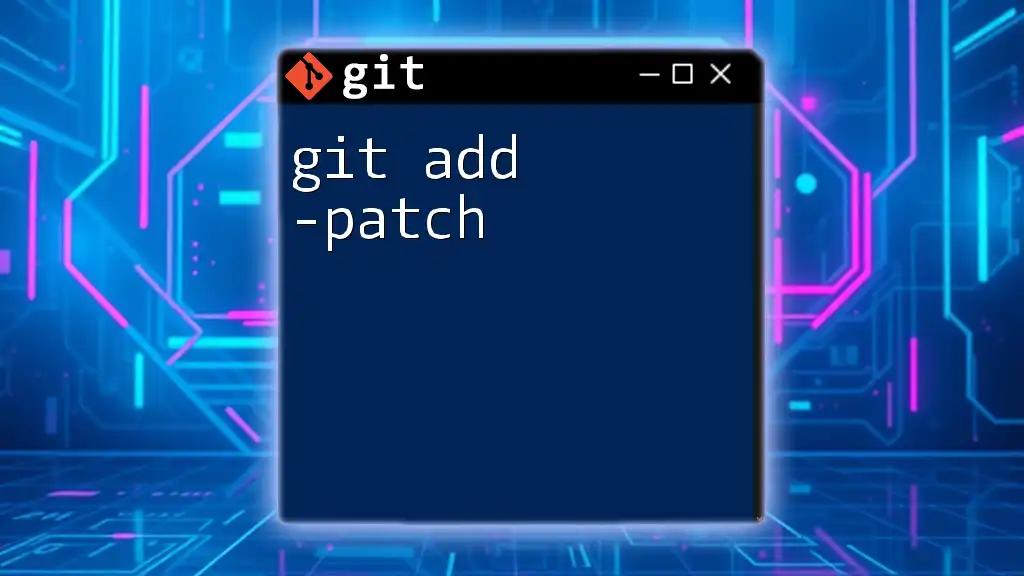
Applying a Patch with `git apply`
Basic Usage
Applying a patch is a straightforward process. Once you have your patch file ready, you can execute:
git apply my_changes.patch
This command reads the changes from the `my_changes.patch` file and applies them directly to your working directory. After executing this command, the changes are staged for commit, and you can then review and finalize them as needed.
Handling Errors
While applying patches is generally straightforward, conflicts can arise. Here are ways to address common errors:
-
Common errors occur when the changes in the patch overlap with modifications made in the working directory. To troubleshoot conflicts, you can run the `--check` option before applying, which helps identify issues in advance.
-
If conflicts occur when you attempt to apply a patch, using the `--reject` option helps manage these situations by creating `.rej` files for the chunks that could not be applied:
git apply --reject my_changes.patch
This allows you to address conflicts on your own terms and aids in a smoother integration of changes.
Practical Scenarios
Applying an Incoming Patch
When you receive a patch from a collaborator, the process involves saving the patch file and using `git apply` to incorporate their changes. This approach ensures that you integrate specific enhancements or bug fixes without merging whole branches indiscriminately.
Creating and Sharing Patches
Sharing your changes with others is easy using patches. You can create a patch with:
git format-patch -1
This command generates a patch file for the most recent commit, which you can then send via email or share through other means.
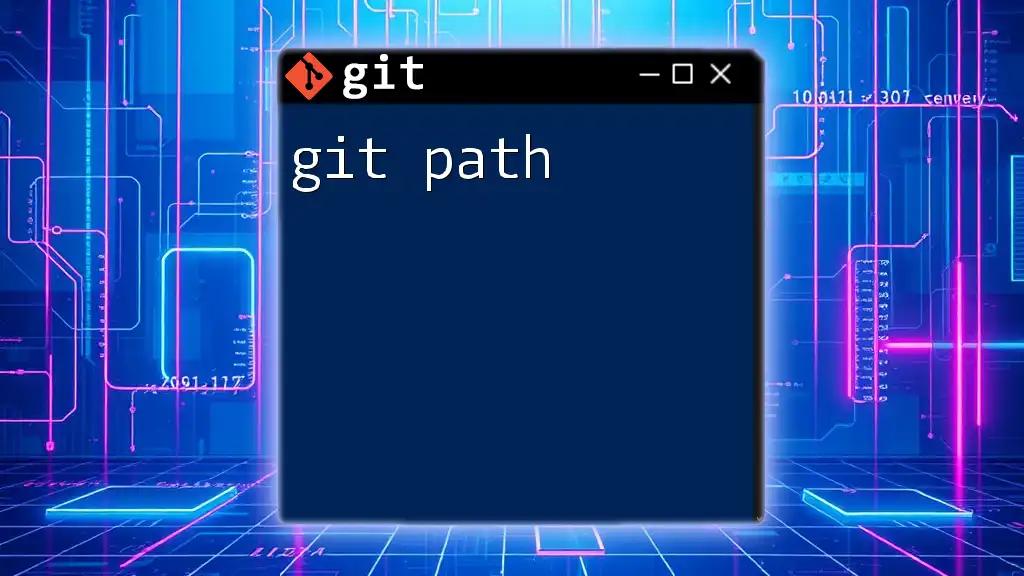
Advanced Usage of `git apply`
Applying Multiple Patches
In many cases, you may have multiple patches that need to be applied consecutively. For efficiency, you can batch apply all patches in a directory:
git apply *.patch
This command applies every `.patch` file available in the current directory, streamlining your workflow and reducing repetitive tasks.
Combining `git apply` with Other Commands
Integrating `git apply` with other Git commands can enhance your workflow. For example, you might want to stash your current changes before applying a new patch to avoid conflicts:
git stash && git apply my_changes.patch
This sequence’ ensures a clean working directory and enables you to smoothly integrate changes without losing your current progress.
Performance Considerations
In large projects with extensive histories, applying patches with `git apply` can be significantly faster than performing a full merge. Skills in using patches can improve both the workflow and development speeds, especially when managing external contributions or temporary changes.
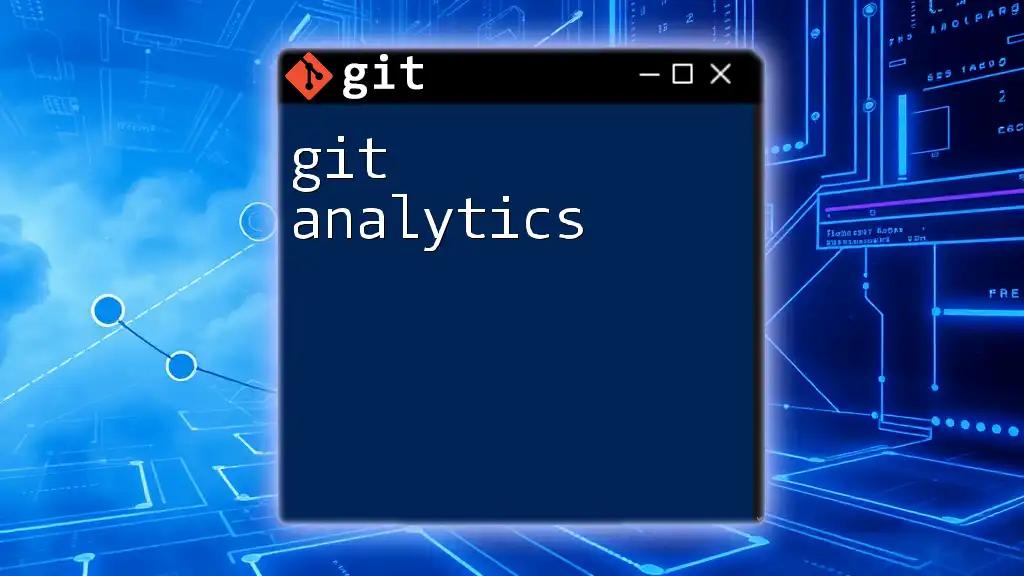
Conclusion
Utilizing the `git apply patch` command is an invaluable skill in efficiently managing your project's code. Understanding how to create, apply, and troubleshoot patches empowers developers to collaborate seamlessly and maintain clean version control histories.
Experimenting with `git apply` in your daily routine can enhance your proficiency in Git and improve your overall development experience. For further learning, explore online courses and resources that dive deeper into Git commands and workflows.
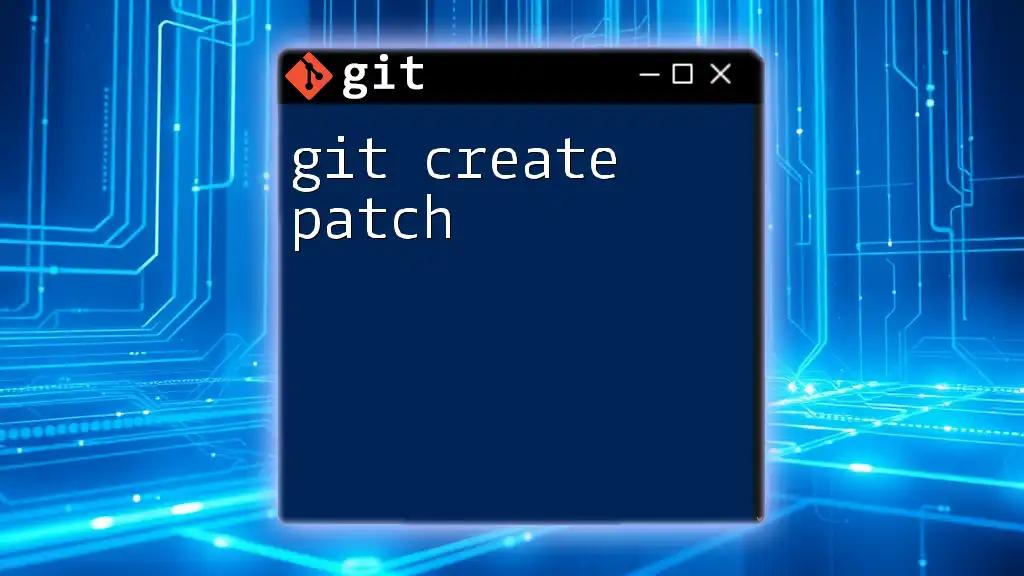
FAQs
What if my patch doesn't apply?
If you encounter difficulties applying a patch, check for overlap with existing changes. Using `git apply --check` allows you to pre-emptively identify and resolve these conflicts.
Can I apply a patch from a different branch?
Yes, you can apply patches created from different branches, but be aware of the potential for conflicts if the codebases have diverged significantly. Always ensure your working directory is clean to minimize issues.
Is `git apply` different from `git am`?
Yes, `git apply` applies patch files directly without creating any corresponding commit, while `git am` applies patches generated by `git format-patch`, which include commit information and can create commits automatically. Understanding when to use each command is crucial for effective version control practices.