A Git patch is a file that contains differences between two versions of a repository, allowing you to share and apply changes using the `git apply` command.
Here's a simple example of creating and applying a patch:
# Create a patch from the last commit
git format-patch -1 HEAD
# Apply a patch
git apply my_patch_file.patch
What is a Git Patch?
A patch in the context of Git is a simple yet powerful file that captures the differences between one or more files. It reflects the changes made to source code, making it easier to share those specific changes without distributing the entire codebase. While similar to commits, patches focus solely on the changes rather than the entire snapshot of the repository at a given point in time.
Understanding the difference between a patch and a commit is crucial. A commit encapsulates a complete state of the project, whereas a patch encapsulates only the differences—essentially, a summary of what has changed since a specific point. This granularity allows for more flexibility in version control practices, making patches a useful tool for various workflows.
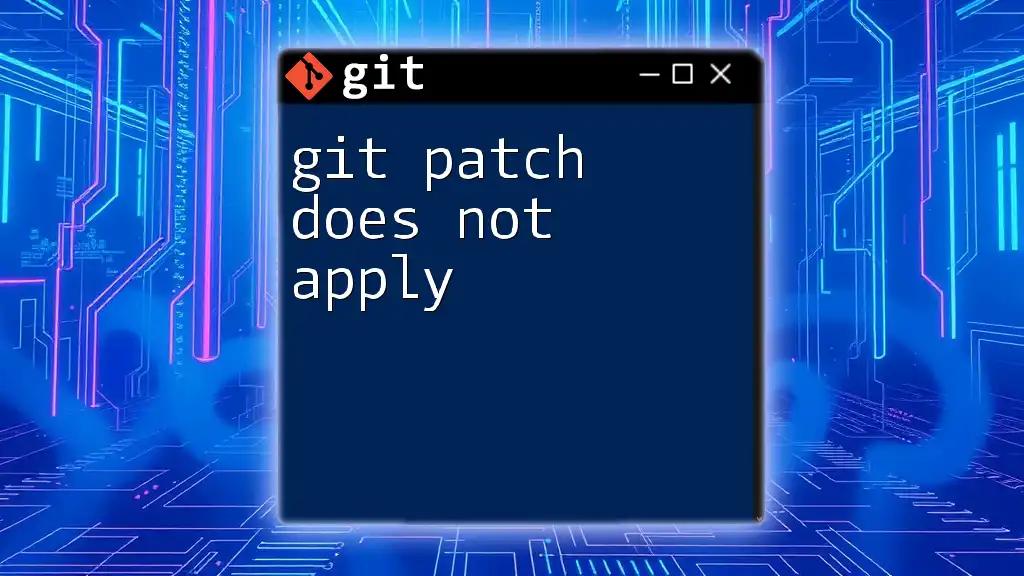
Creating a Git Patch
Using the `git diff` Command
The `git diff` command is your go-to for generating patches on the fly. It compares your working directory with the index and outputs the changes made.
For instance, to create a patch file that captures changes since your last commit, you can use:
git diff > changes.patch
This command creates a `changes.patch` file in your current directory, containing the differences in a unified format. You can open this file to review and share the specific changes made.
Creating Patches from Commits
Another common method for creating patches is through the `git format-patch` command. This is particularly useful when you want to create patches based on committed changes.
Generating a Patch from the Last Commit
To generate a patch for the most recent commit, you can use:
git format-patch -1 HEAD
This command produces a patch file reflecting the changes made in the most recent commit, making it easy to share those changes without needing to share the whole repository.
Generating Patches for Multiple Commits
You can also create patches for multiple commits by specifying a range. For example:
git format-patch HEAD~3..HEAD
This command generates patch files for the last three commits, allowing collaborators to apply these patches individually or together, depending on their workflow requirements.
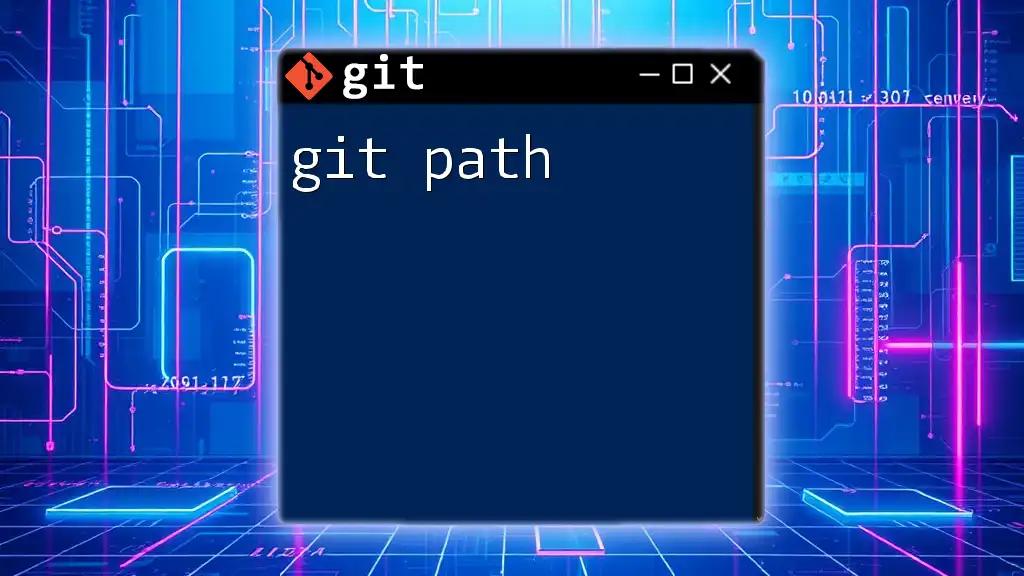
Applying and Reverting Patches
Applying a Patch with `git apply`
Once you or someone else has created a patch, applying it to a repository can be done using the `git apply` command. This command applies the changes described in the patch file to your current working directory without creating any commits.
For example:
git apply changes.patch
This command reads the differences stored in `changes.patch` and applies them to the current files accordingly. It's a straightforward way to incorporate shared changes.
Applying a Patch with `git am`
In some situations, you might want to apply patches that also include commit messages and authorship information. In this case, the `git am` command is appropriate. It is especially useful for applying patches generated by `git format-patch`.
You can use it as follows:
git am < changes.patch
This command applies the patch and creates a new commit, capturing the commit message and author information from the patch itself.
Reverting a Patch
Reverting the changes introduced by a patch is as valuable as applying them. If you've mistakenly applied a patch or no longer need it, you can reverse its effects using the `-R` option with `git apply`:
git apply -R changes.patch
Using the `-R` flag tells Git to reverse the changes specified in the patch file, restoring the original state of the files.
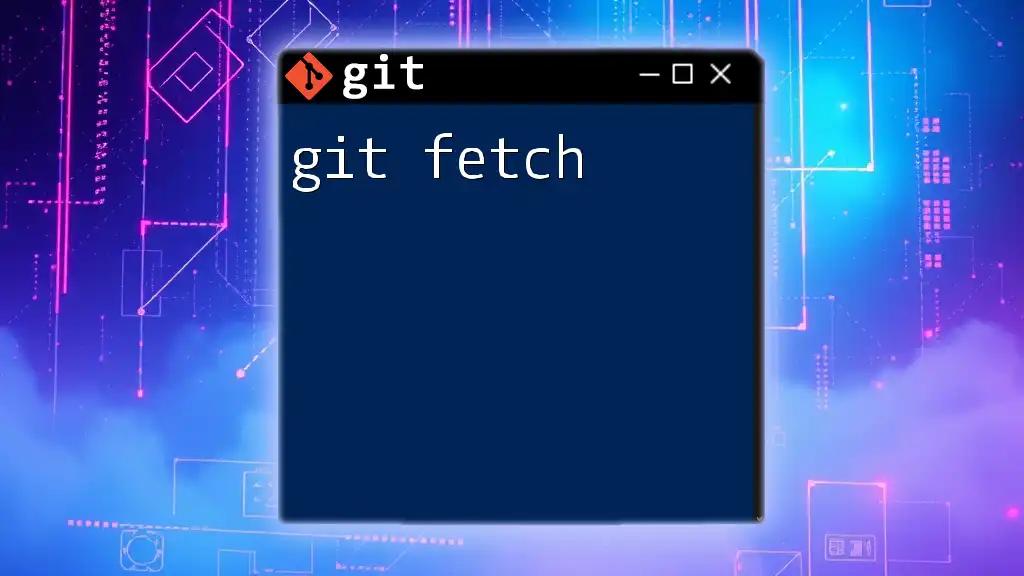
Best Practices for Using Git Patches
When to Use Patches
Using patches can be particularly beneficial in collaborative environments where team members may not have direct access to one another’s branches. Patches allow for an exchange of specific changes without a large merge. They are also helpful in integrating changes from a team member's branch if you cannot directly pull from it.
Organizing and Managing Patches
Proper management of patch files is crucial. When saving patches, consider adopting a consistent naming convention that reflects the content or purpose of the changes. For example, `feature-update-x.patch` provides context about the content. Additionally, keeping detailed documentation comments within patch files can help convey the necessity for changes during code reviews.
Version Control for Patches
Maintaining a dedicated repository for patches can help keep track of all modifications made outside the primary Git history. Tools around automation, including scripts that create patches from branches automatically, can significantly streamline your workflow, especially with frequent changes.
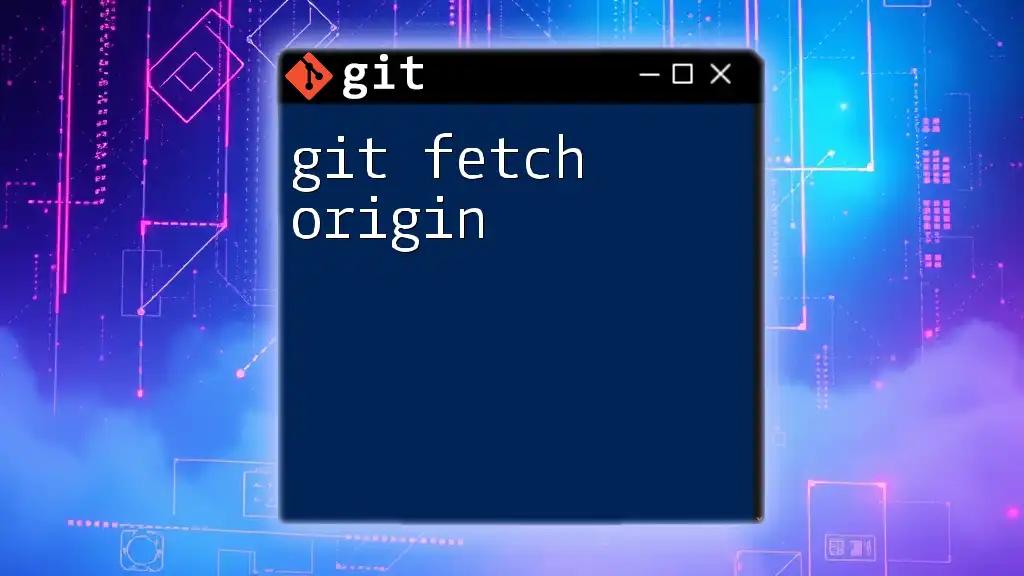
Common Pitfalls and Solutions
Conflicts When Applying Patches
One of the risks when applying patches is encountering conflicts, especially if the code base has diverged significantly since the patch was created. When this occurs, Git outputs conflict markers that you'll need to manually resolve in the affected files. It’s advisable to review the patch and the code carefully, ensuring that the most appropriate changes are retained.
Troubleshooting Tips
Common error messages related to the application of patches include notifications about line number mismatches or conflicts. If you encounter errors, double-check the context of where the patch is applied, make sure you are on the correct branch, and consider the state of your working directory. Using version control commands like `git status` can help clarify the state of your repository at any given time.
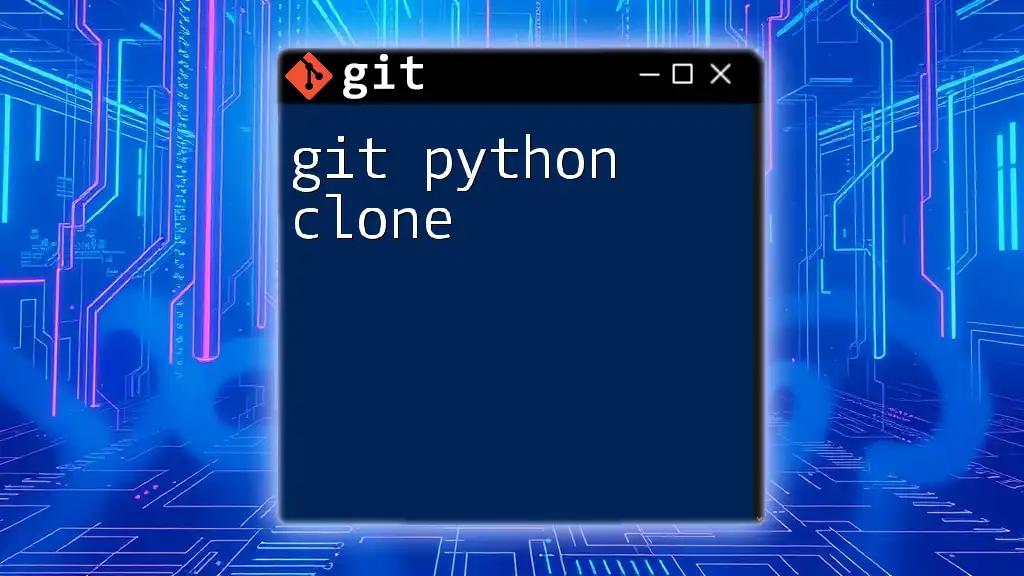
Conclusion
Understanding and utilizing git patches is a valuable skill for developers navigating collaborative coding environments. They offer a flexible way to share, apply, and recover changes without needing to resort to heavier pull or merge requests. As you incorporate patches into your workflow, you'll find them an essential tool for maintaining clean and manageable code.
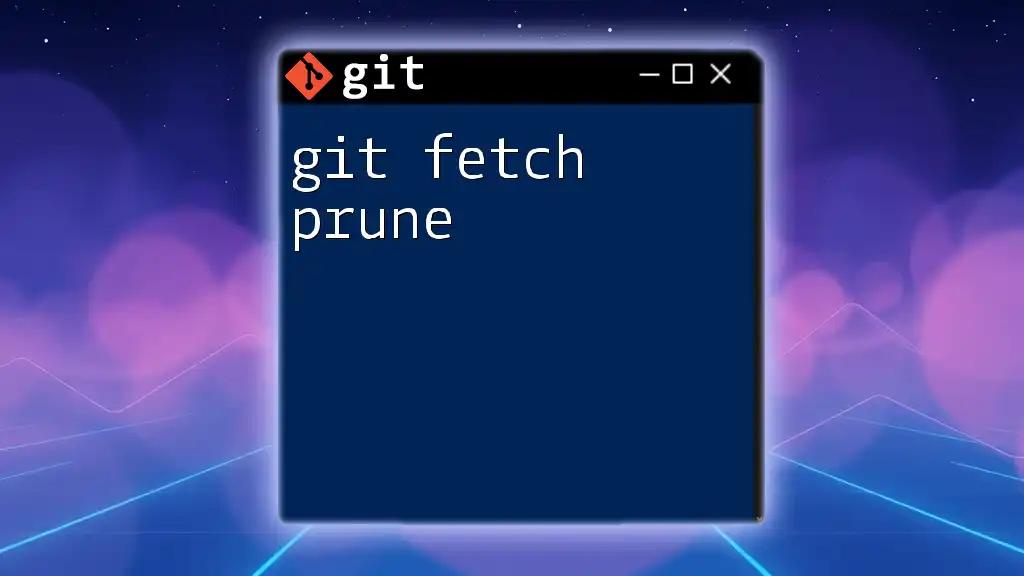
Additional Resources
To deepen your understanding of git patches, consider exploring Git's official documentation, engaging with tutorials focused on practical applications, and investing time in mastering various advanced features around version control. Books that cover Git in detail can also provide a structured learning path for mastering the subtleties of patches and branches in your projects.