The `git python clone` command is typically used to create a local copy of a remote repository, allowing you to work with the code on your machine.
Here’s how to use the command:
git clone https://github.com/username/repository.git
Understanding Git and Its Importance
What is Git?
Git is a distributed version control system that allows multiple developers to work on projects simultaneously. It tracks changes, coordinates work on files, and allows multiple versions of a project to coexist. Originally created by Linus Torvalds in 2005, it has since gained significant popularity in both open-source and commercial software development.
Why Use Git?
Using Git provides numerous benefits: it enables collaboration among developers, allows for tracking changes over time, and supports easy recovery of previous versions of a project. Basic Git commands, such as `clone`, are crucial for ensuring that developers can effectively share and maintain code.
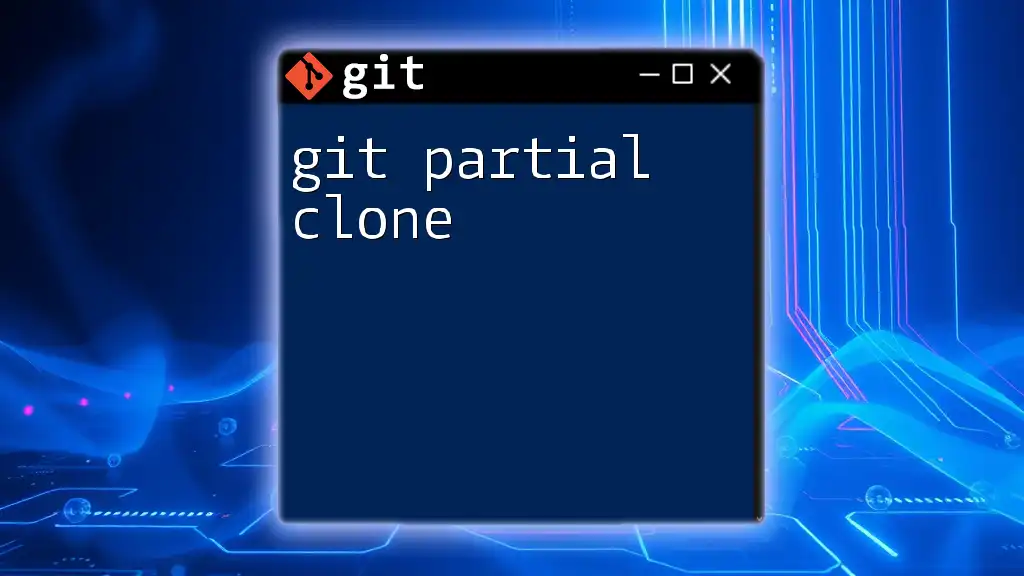
Introduction to `git clone`
What is `git clone`?
The `git clone` command is a foundational command in Git. It creates a copy of an existing Git repository into a new directory on your local machine. This is essential when you want to contribute to a project or start using a project developed by someone else.
Cloning vs. Forking
While both operations create a copy of a repository, cloning creates a local version of a repository, allowing you to work on it directly. In contrast, forking creates a separate repository on platforms like GitHub, enabling users to modify the code without affecting the original project. Forking is typically used in collaborative projects where further modifications are needed.
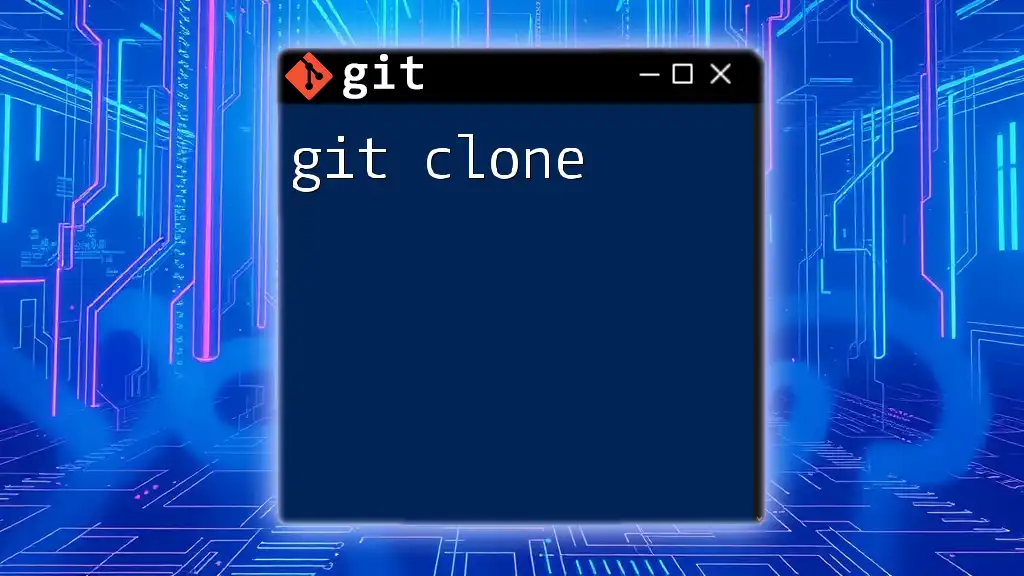
Getting Started with `git clone`
Prerequisites for Cloning
Before you can clone a repository, ensure that you have Git installed on your computer. Below are brief installation steps for different operating systems:
- Windows: Download and install Git from [git-scm.com](https://git-scm.com).
- macOS: Install via Homebrew with the command `brew install git` or download from the website.
- Linux: Use your package manager, e.g., `sudo apt-get install git` for Debian-based systems.
You should also have a GitHub account or an account on your preferred code hosting service to access repositories.
The Syntax of `git clone`
The basic command structure for cloning a repository in Git is as follows:
git clone <repository-url>
Where `<repository-url>` can be in SSH, HTTPS, or Git protocol formats. Each format may require different access permissions and configurations.
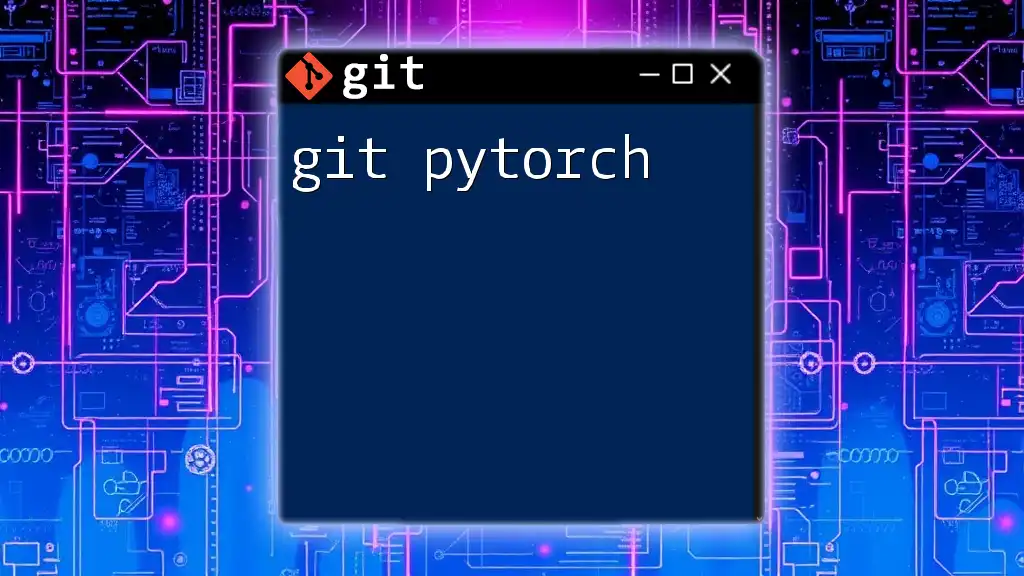
Step-by-Step Guide to Cloning a Repository
Finding a Repository to Clone
To clone a repository, you'll first need to find one. Most commonly, you'll search on GitHub or similar platforms. Consider factors such as the programming language, popularity (indicated by stars), and recent activity before selecting a repository.
Cloning a Repository
Using HTTPS
To clone a repository using the HTTPS URL, you would use the following command:
git clone https://github.com/user/repo.git
When you execute this command, Git will create a new directory named `repo` in your current location, download all project files, and initialize a local `.git` directory containing all version control information.
Using SSH
If you prefer using SSH, you must first set up your SSH keys. Here’s how you can do that:
- Generate SSH keys on your local machine using:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
- Add the public key to your GitHub account by copying the contents of your `~/.ssh/id_rsa.pub` file.
After that, you can clone your repository using:
git clone git@github.com:user/repo.git
Executing this command works similarly to the HTTPS method, but may offer a more secure connection after initial setup.
Specifying a Directory Name
If you want to clone a repository into a specific directory, you can provide a second argument:
git clone <repository-url> <directory-name>
For example:
git clone https://github.com/user/repo.git my-repo
This command will create a new directory called `my-repo`, containing the cloned repository.
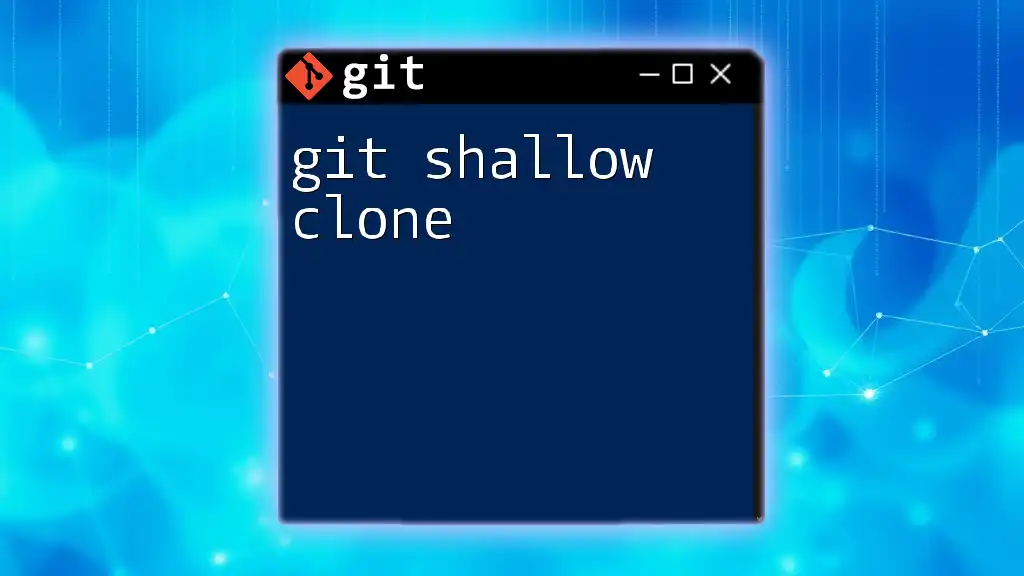
After Cloning: What Next?
Navigating the Directory
Once cloning is complete, you should navigate into your newly created directory. You can do this easily with the following command:
cd my-repo
This changes the current working directory to `my-repo` so you can begin working on it.
Understanding the Cloned Files
After cloning, it's crucial to understand the structure of the cloned files. The most notable components are:
- .git Folder: This hidden folder contains all the version control data, including commit history and configuration settings.
- README.md: A markdown file that typically contains project information, installation instructions, and other relevant documentation.
Fetching Updates from the Remote
To ensure your local repository remains in sync with the remote one, use the `git pull` command. This command retrieves the latest changes from the branch you are tracking:
git pull origin main
Always pull before starting to work on new features or bug fixes to avoid conflicts.
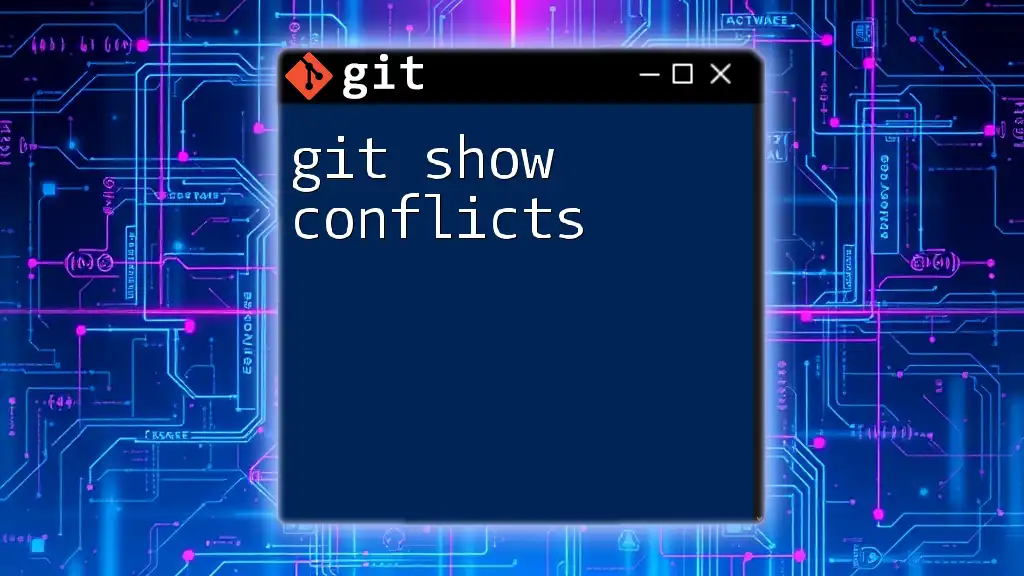
Common Issues and Troubleshooting
Error Messages and Solutions
One common problem that users encounter is related to SSH key issues. These often manifest as “Permission denied” errors. Ensure that your public SSH key is correctly added to your GitHub account, and that the corresponding private key is accessible on your local machine.
Another frequent problem is encountering "Repository not found" errors. This may indicate that the URL is incorrect or that you don’t have permission to access the repository.
Best Practices for Cloning
Before cloning a repository, always verify the source to ensure it is a trusted repository. Cloning from unknown or unverified sources can lead to security risks. Additionally, when cloning private repositories, ensure you have the necessary permissions and access rights.
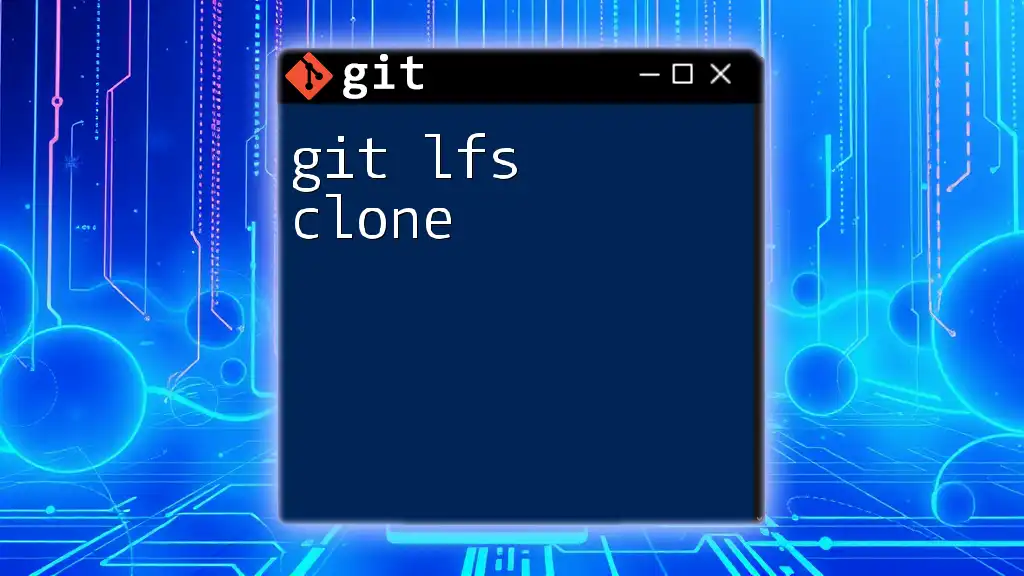
Conclusion
In this guide, you learned about the `git clone` command and its importance in version control. From understanding the differences between cloning and forking to actually cloning repositories using HTTPS or SSH, you are now equipped with the knowledge to start using Git more effectively.
Encouraging Further Learning
Mastering Git takes time and practice. We encourage you to explore additional resources, such as the official Git documentation, tutorials, and community forums, to deepen your understanding and expand your skills in using Git and version control.
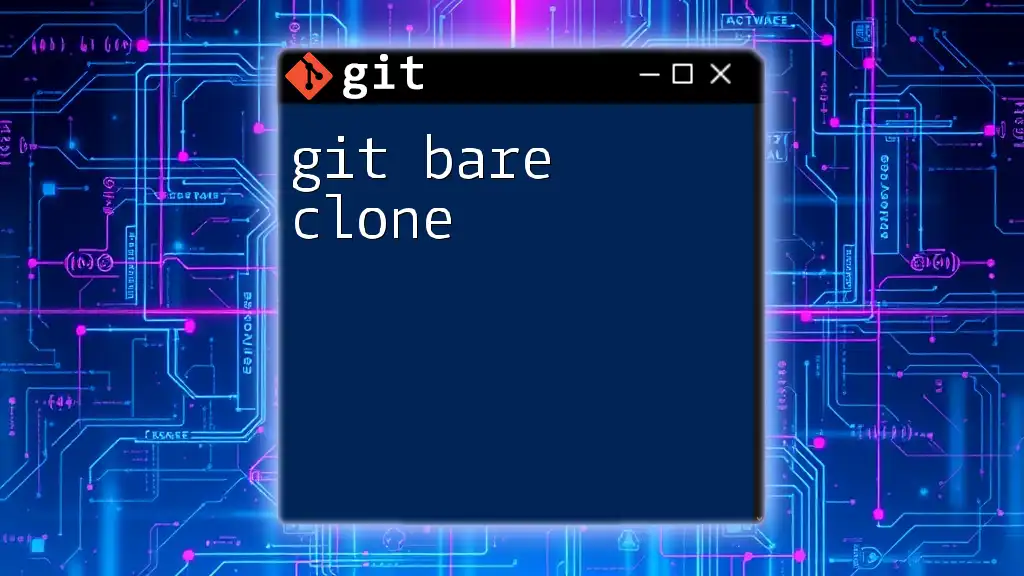
Code Snippets Collection
Common Commands Summary
Here’s a quick reference of the commands discussed in this article:
git clone <repository-url> # Basic clone command
git clone <repository-url> <dir-name> # Clone with a specific directory name
cd <directory-name> # Navigate to the cloned directory
git pull origin main # Fetch updates from the remote
With this foundational knowledge, you're ready to start using `git python clone` commands and take on version control with confidence!