The `git update-clone` command is used to create a new clone of an existing repository that is updated from the latest remote commits without affecting any current clones.
git update-clone <repository-url>
Understanding the Need for Updates in Cloned Repositories
Why Keep Your Cloned Repositories Updated?
Keeping your cloned repositories updated is crucial in collaborative software development. When multiple contributors work on a project, changes are continuously made to the original repository (often referred to as the "remote repository"). By regularly updating your local copy, you ensure that you are working with the latest features, bug fixes, and other essential changes. This practice not only enhances your productivity but also minimizes the potential for conflicts when merging your changes back to the main codebase.
Common Scenarios Requiring Updates
There are several situations where you need to update your cloned repositories:
- Working in a team where other members push changes frequently.
- Contributing to open-source projects that are active and receive regular updates.
- Maintaining your own projects when you re-clone a repository to try new features or experiments.
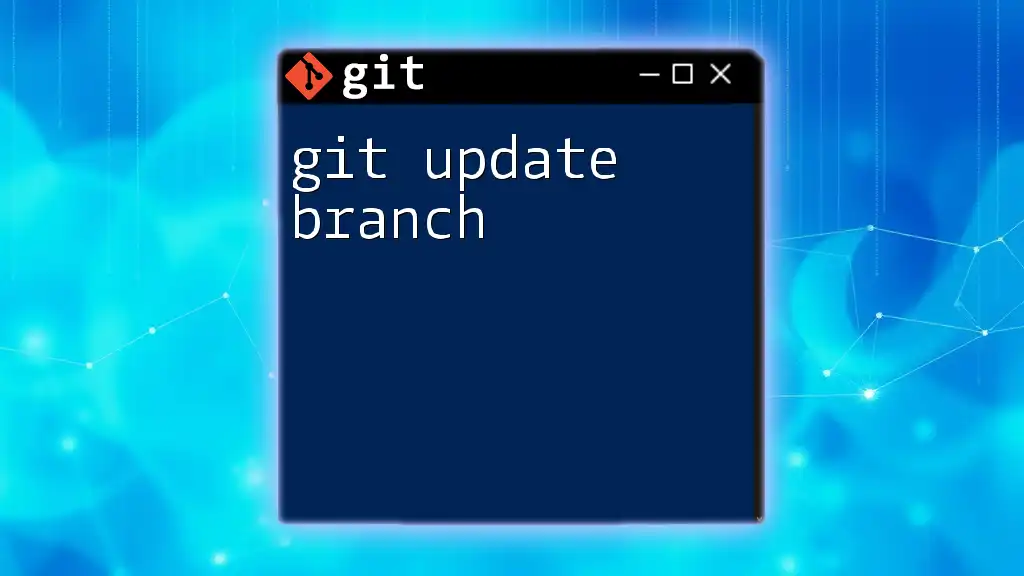
The Mechanics of Updating a Cloned Repository
Using Git Fetch and Pull
To effectively update a cloned repository, you usually utilize two primary commands: `git fetch` and `git pull`. Each command serves a distinct purpose that is important to understand.
Overview of `git fetch`
The `git fetch` command is designed to retrieve updates from a remote repository without merging them into your local branch. This allows you to see changes made by other contributors without altering your working files.
What It Does: The command contacts the remote repository and fetches changes, like new commits or branches. However, it does not modify your current working branch.
Code Snippet:
git fetch origin
After running the above command, you can check out the fetched changes using `git log` or `git status` to see what has been updated upstream.
Overview of `git pull`
On the other hand, `git pull` simplifies the process by executing two commands in one: it first performs a `git fetch` followed by a `git merge`. This command updates your current branch with the latest changes from the remote repository.
What It Does: `git pull` not only retrieves the latest commits but also attempts to automatically merge them with your local commits.
Code Snippet:
git pull origin main
This command is not only quicker but also effective for straightforward updates, especially if you are certain there won't be any merge conflicts.
Understanding the Differences
Understanding when to use `git fetch` versus `git pull` is essential for effective version control. Use `git fetch` when you want to review incoming changes without affecting your local work. Use `git pull` when you are ready to integrate changes and you are confident that your local work is in sync.
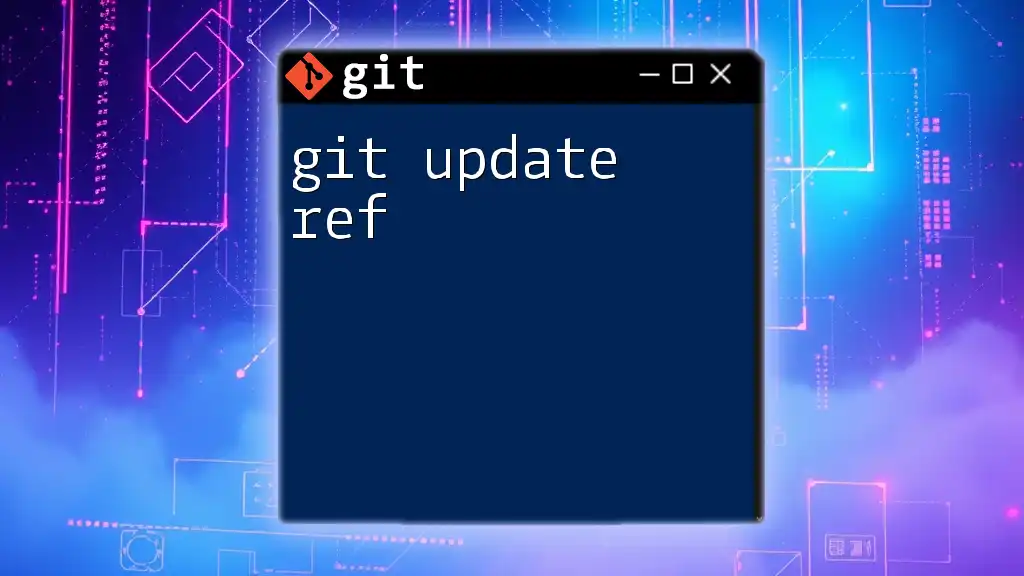
The `git update` Command and Its Implications
What is `git update`?
It's important to note that the `git update` command does not exist in Git terminology. Some users may mistakenly believe that this is a valid command for updating a cloned repository. Instead, the correct commands to consider for updates are `git fetch`, `git pull`, and – to a lesser extent – `git remote update`.
Using `git remote update`
If you're interested in updating all remote tracking branches rather than just the one you're currently working on, you can use the `git remote update` command.
What It Does: This command updates all of your remote tracking branches, allowing you to have a complete perspective of changes happening in the remote repository.
Code Snippet:
git remote update
This is particularly useful if you are working with multiple branches or remotes and want to be aware of all changes.
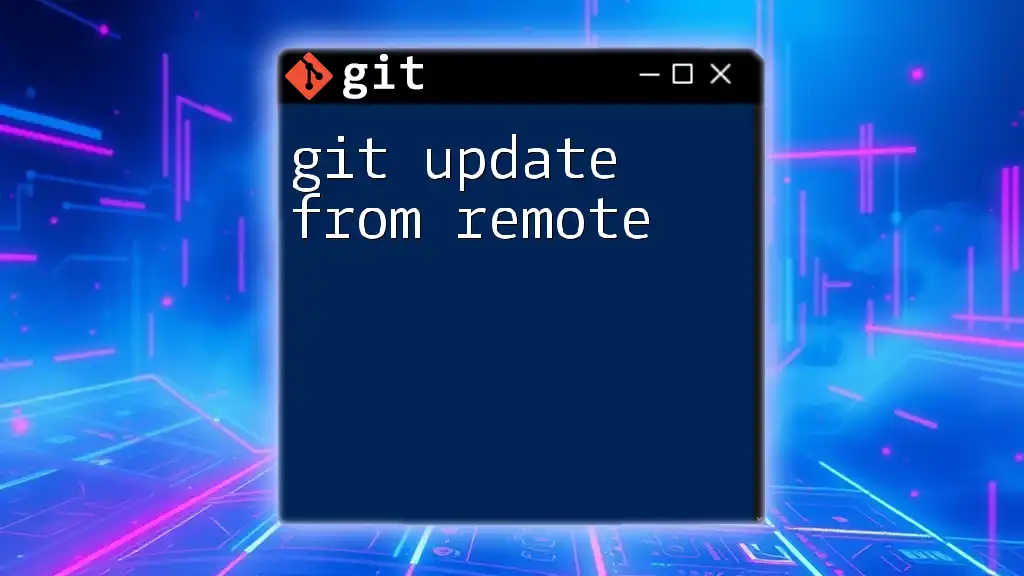
Best Practices for Updating Cloned Repositories
Regularly Pull Changes
It’s advisable to consistently pull changes when working in a collaborative environment. Here are some guidelines on how often you should sync:
- Daily Updates: If you're working in a team that pushes changes regularly, aim to pull updates daily to stay in sync.
- Before Major Changes: Always fetch or pull updates before starting significant feature development. This minimizes conflicts with the changes in the remote repository.
Handling Merge Conflicts
Merge conflicts arise when two branches have diverging changes that cannot be automatically reconciled. When you encounter a merge conflict, Git will notify you upon execution of `git pull` or `git merge`.
Here are some basic strategies for resolving conflicts:
- Review Conflicted Files: Git will mark conflicted areas within the files; review each segment carefully.
- Choose the Changes to Keep: Edit the files manually to select the changes you wish to retain.
- Finalizing the Merge: Once you've resolved all conflicts, run:
git add <file> git commit
Keeping a Clean Local Repository
Before you fetch or pull updates, ensure that your local repository is clean and committed. Uncommitted changes can lead to complications during the update process. If you have unsaved work, consider stashing it:
Using `git stash`
This command allows you to save your uncommitted changes temporarily while you update your repository:
Code Snippet:
git stash
git pull origin main
git stash pop
This way, you can update the repository without compromising your current work.
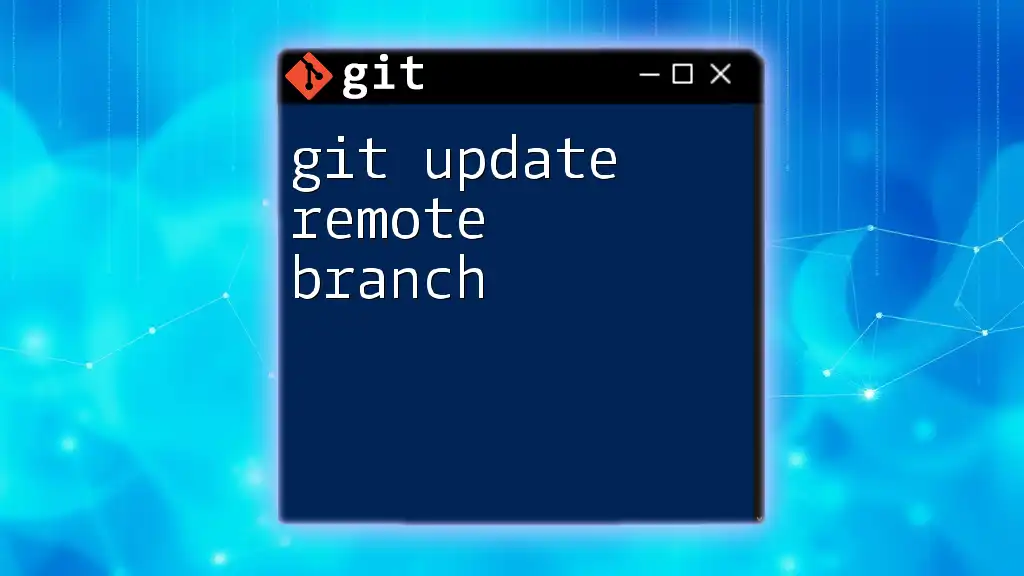
Examples and Use Cases
Real-World Example: Collaborating on a Team Project
Imagine you are on a team working on a web application. Here's how to proceed with cloning, updating, and merging contributions:
-
Clone the Repository:
git clone https://github.com/username/repo.git
-
Make some changes in a feature branch:
git checkout -b feature-branch // make some edits... git add . git commit -m "Completed feature"
-
Before pushing, update your local branch:
git pull origin main
If there are conflicts, resolve them as outlined earlier and then push your changes:
git push origin feature-branch
Case Study: Open Source Contributions
When contributing to an open-source project, you would typically:
- Fork the repository and clone it.
- Create a new branch for your changes.
- Periodically update your fork by using:
git fetch upstream git merge upstream/main
- Keep committing your changes and push them back to your fork.
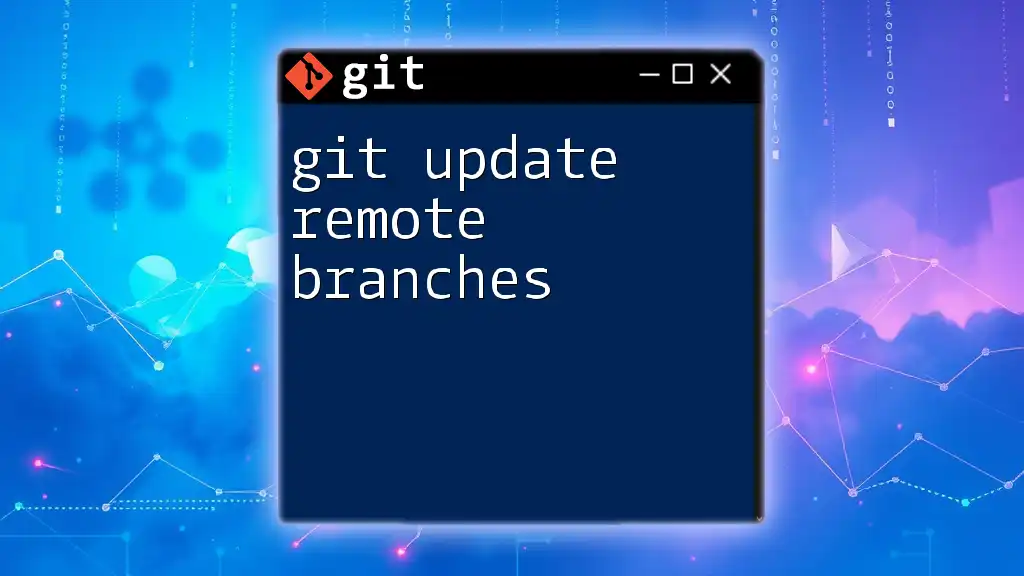
Troubleshooting Common Issues
Updating Failures
Complications during the update process, such as diverging branches or uncommitted changes, can often stall your workflow. If you run into an issue while pulling changes, first check for any uncommitted files:
git status
If necessary, stash or commit your changes before attempting to update again.
When Clone Issues Arise
Occasionally, you may find that your clone is outdated or corrupted. Signs of troubles include unexpected behavior or error messages. In such cases, it might be best to reclone the repository:
git clone https://github.com/username/repo.git
This ensures you have a fresh and functional version of the repository.
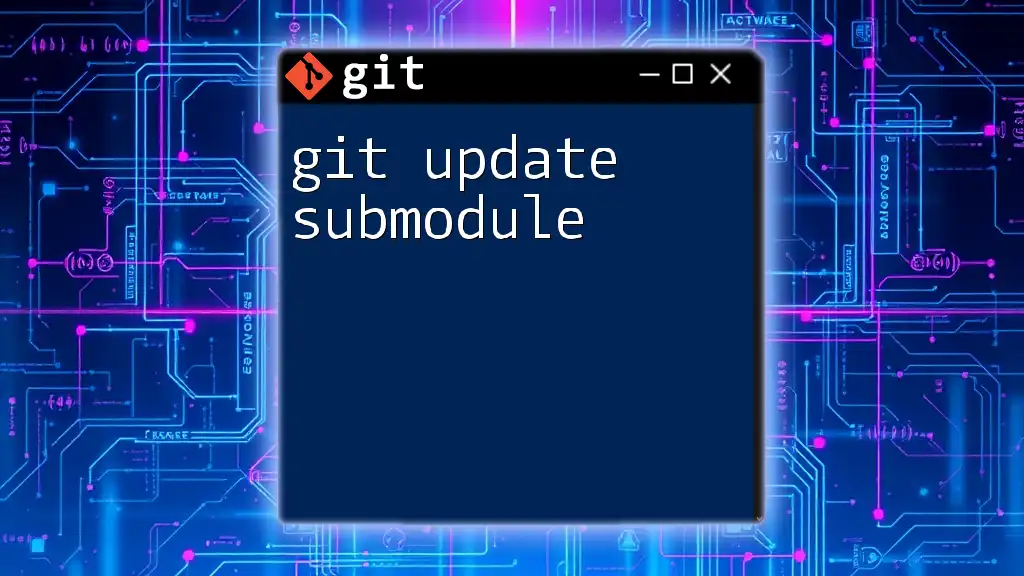
Conclusion
In summary, understanding how to effectively manage your cloned repositories is critical in the world of software development. Remember, "git update clone" refers not to an existing command but rather to the essential practices surrounding `git fetch`, `git pull`, and `git remote update`. Regularly applying these commands will keep your work aligned with the latest developments. As you gain confidence in using Git, make it a habit to execute these commands in your daily workflow, enhancing both your proficiency and your collaboration with others.