To update a Git tag, you can delete the old tag locally, recreate it with the desired commit, and then push the updated tag to the remote repository.
git tag -d <tag_name> # Delete the old tag locally
git tag <tag_name> <commit> # Create a new tag pointing to a specific commit
git push origin <tag_name> # Push the updated tag to the remote repository
Understanding Git Tags
What are Git Tags?
Git tags are a mechanism designed to mark specific points in a repository's history as important. Essentially, a tag acts as a snapshot of your project at a given time, much like a bookmark. While branches can change as new commits are added, tags are fixed references to specific commits, making them ideal for versioning.
Importance of Using Tags
Tags provide several benefits in version control, especially when managing releases and significant project milestones.
-
Semantic Versioning: Tags play a crucial role in marking stable releases. For instance, tags follow the pattern of `v1.0`, `v2.1.5`, and so on. These semantic versions convey information about the nature of changes made between releases.
-
Easier Navigation: Tags simplify the navigation of project history. With tags, developers can quickly switch between releases and easily reference the state of a project at specific points in time.
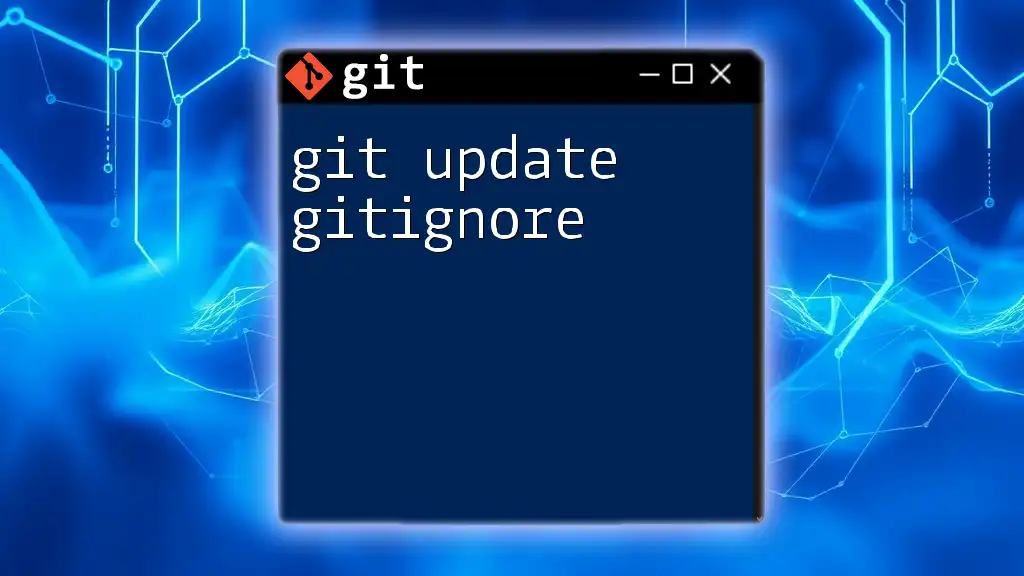
Common Use Cases for Tags
Release Management
Tags are frequently used in release management to identify stable versions of a project. For instance, a development team may tag their release version `v1.0` to denote the completion and readiness of their software for distribution. This way, anyone can access the codebase at that particular state.
Marking Significant Milestones
Tags also serve the purpose of marking key milestones in a project timeline. For example, it may be beneficial to tag a release as `beta` if you've reached a phase where user testing is essential. This tagged version makes it clear to collaborators and users that it is a significant step in the development lifecycle.
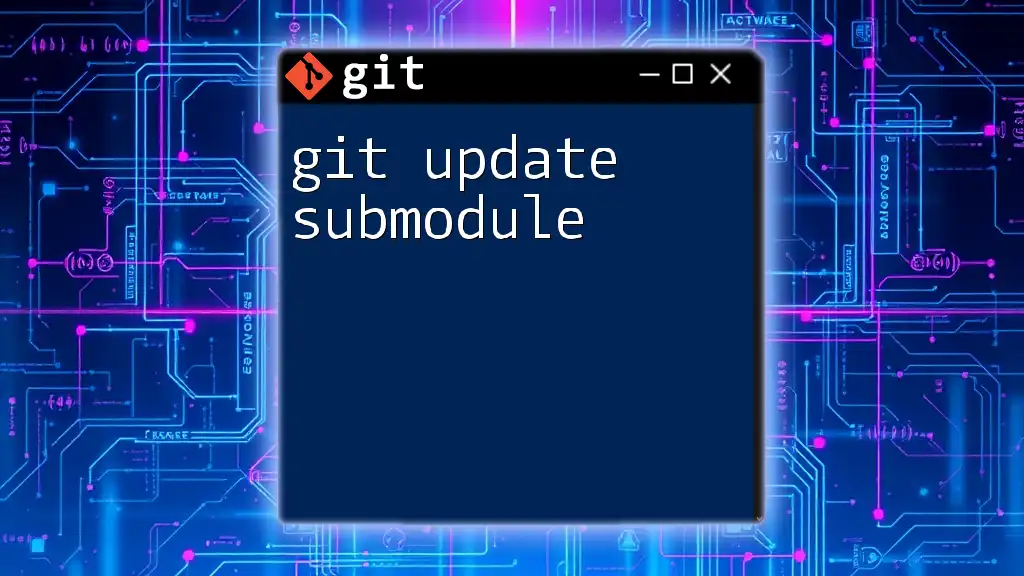
How to Create a Tag
Basic Tag Creation
Creating tags in Git is straightforward. There are two main types of tags:
-
Lightweight Tags: These are simple and serve as pointers to a commit.
To create a lightweight tag, you would use the command:
git tag <tagname>
-
Annotated Tags: More useful and recommended, annotated tags include additional metadata such as the tagger's name, email, and date.
To create an annotated tag, you can use:
git tag -a <tagname> -m "Tag message"
Tagging a Specific Commit
Sometimes, you'll want to tag a specific commit rather than the latest commit (HEAD). This can be easily accomplished by using the commit hash. Here’s how:
git tag -a <tagname> <commit_hash> -m "Tag message"
In this case, replace `<commit_hash>` with the actual ID of the commit you want to tag. Tagging specific commits can be essential for keeping track of important revisions within a large project.
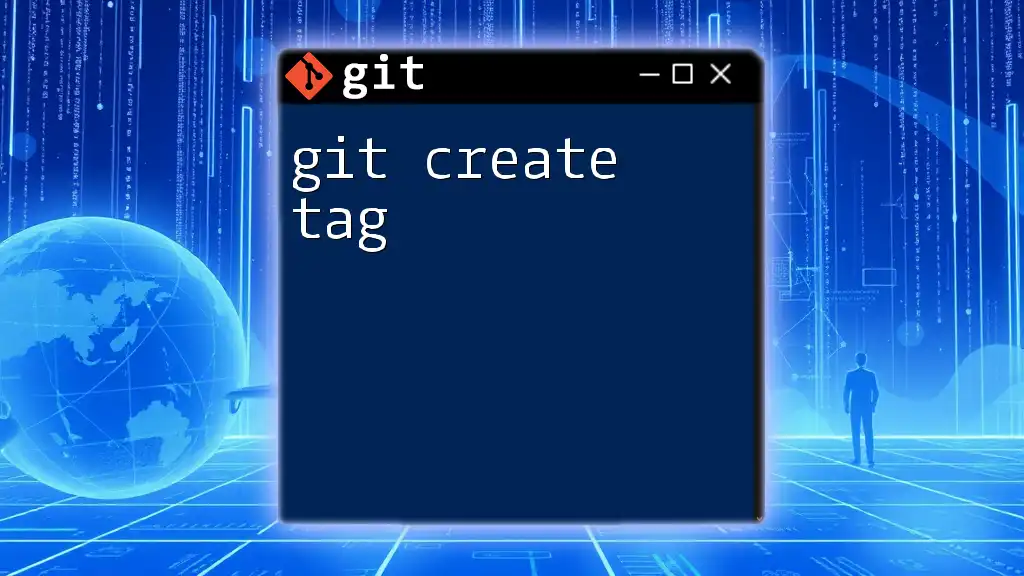
Updating an Existing Tag
Why You Might Need to Update a Tag
Occasionally, you may realize that a tag needs to be amended. This could be due to an error in the original tag or a change in the project that warrants a tag update. It’s essential to differentiate between changing an existing tag and creating a new one; updating a tag should only be done if it’s appropriate for the context.
How to Delete a Tag Locally
If you need to update a tag, the first step is to delete the current tag locally. You can achieve this with:
git tag -d <tagname>
Recreating and Updating the Tag
To update a tag, follow these steps:
-
Delete the existing tag:
git tag -d <tagname>
-
Create the tag again with any updates or corrections:
git tag -a <tagname> -m "Updated tag message"
-
Push the updated tag to the remote repository:
git push origin <tagname> --force
This process ensures that your changes are reflected both locally and in any shared repositories. It's also a good practice to inform your team when tags have been updated.
Best Practices for Tag Management
When managing tags, use the `--force` option with care. Force-pushing can overwrite the history that other collaborators might rely on, so ensure that everyone is aware of the change. Maintaining a clean tagging structure will also facilitate easier navigation and understanding of project progression and version history.
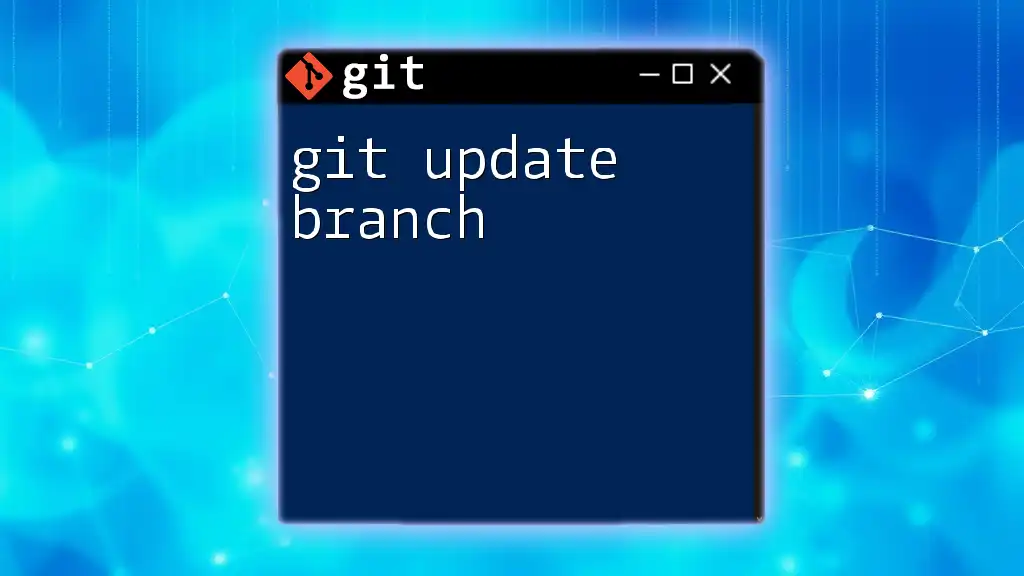
Viewing Tags
Listing All Tags
To see all tags currently available in your repository, use the command:
git tag
This will list all the tags in an alphabetical order and can help you quickly find on which versions of the project you are working.
Viewing Tag Details
If you want to view details about a specific tag, you can use:
git show <tagname>
This command provides not just the tag message but also includes the commit information it points to. Understanding the context of a tag can help you make better management decisions.
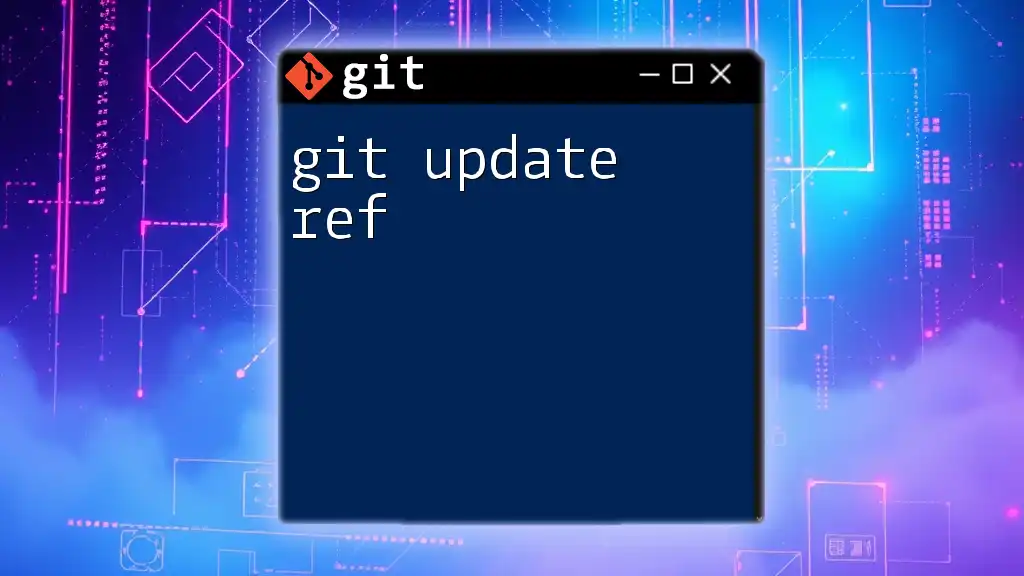
Pushing and Deleting Tags Remotely
How to Push Tags to a Remote Repository
After tagging your commits locally, you might want to share these tags with your collaborators. To do this, push all tags to your remote repository with:
git push origin --tags
This command ensures that all tags you’ve created locally are pushed to the remote repository, allowing your team to see and access them.
Deleting Remote Tags
If you need to remove a tag from the remote Git repository, you can do so using:
git push --delete origin <tagname>
This will permanently delete the specified tag from the remote, but make sure you’ve communicated the reason for this to your collaborators to avoid confusion.
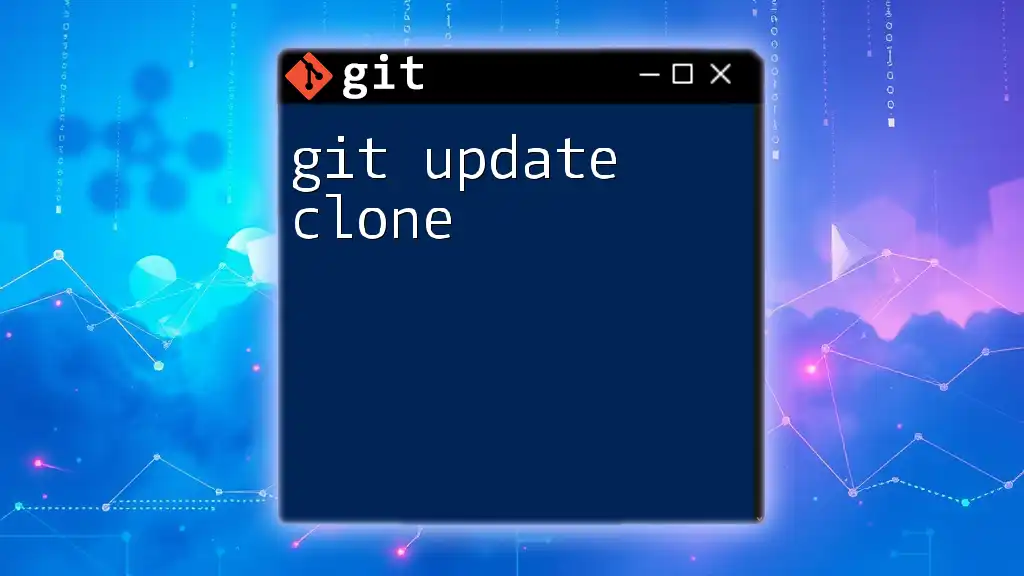
Conclusion
Summary of Key Points
Tags are integral to effective version control, especially for marking releases and significant project milestones. Knowing how to properly create and manage tags, including updating them when necessary, is an essential skill for any developer using Git.
Encouragement to Practice
We encourage you to apply these tagging techniques in your next project. Incorporating tags into your workflow will make your development process more organized and accessible, benefiting both you and your collaborators.
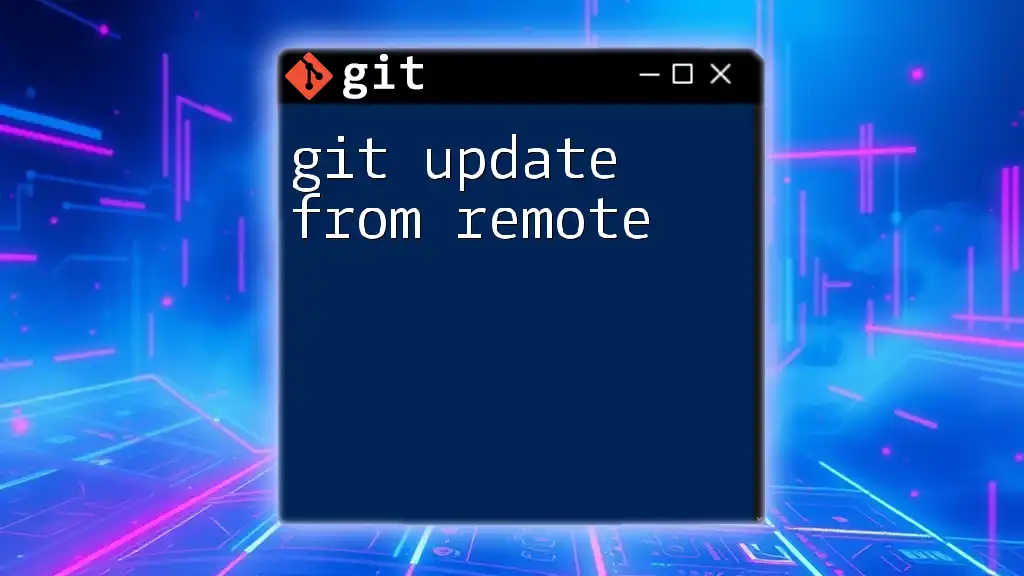
Additional Resources
For more in-depth information, check out the official Git documentation on tags. Additionally, consider looking for video tutorials and courses that can help enhance your Git skills and deepen your understanding of version control management.