To create a tag in Git, use the command `git tag <tag_name>` to create a lightweight tag, or `git tag -a <tag_name> -m "<message>"` for an annotated tag, which includes a message and additional metadata about the tag.
git tag -a v1.0 -m "Version 1.0 release"
Understanding Git Tags
What are Git Tags?
Git tags are a mechanism for marking specific points in Git history. They serve as a way to create a reference to a commit, which is particularly useful when you want to indicate a significant event in the repository history—like a version release or a milestone. It’s important to understand that tags are not branches; while tags are meant to mark a specific commit, branches are used to develop features, fix bugs, or carry out experiments.
Types of Git Tags
Git provides two main types of tags: lightweight tags and annotated tags.
Lightweight Tags A lightweight tag is essentially a bookmark to a specific commit—it doesn’t contain any metadata other than the commit ID. It’s quick and simple to create, which makes it useful for transient tags.
To create a lightweight tag, use the following command:
git tag <tag-name>
Example: If you want to tag the current commit as `v1.0`, simply run:
git tag v1.0
Annotated Tags Annotated tags, on the other hand, are more robust. They include a message, the tagger's name, email, and date, making them more informative. Annotated tags are stored as full objects in the Git database, which means they can hold a lot of valuable information.
To create an annotated tag, use this command:
git tag -a <tag-name> -m "Your message here"
Example: If you want to create a tag for version release `v1.0` and enhance it with a message, you would do:
git tag -a v1.0 -m "First stable release"
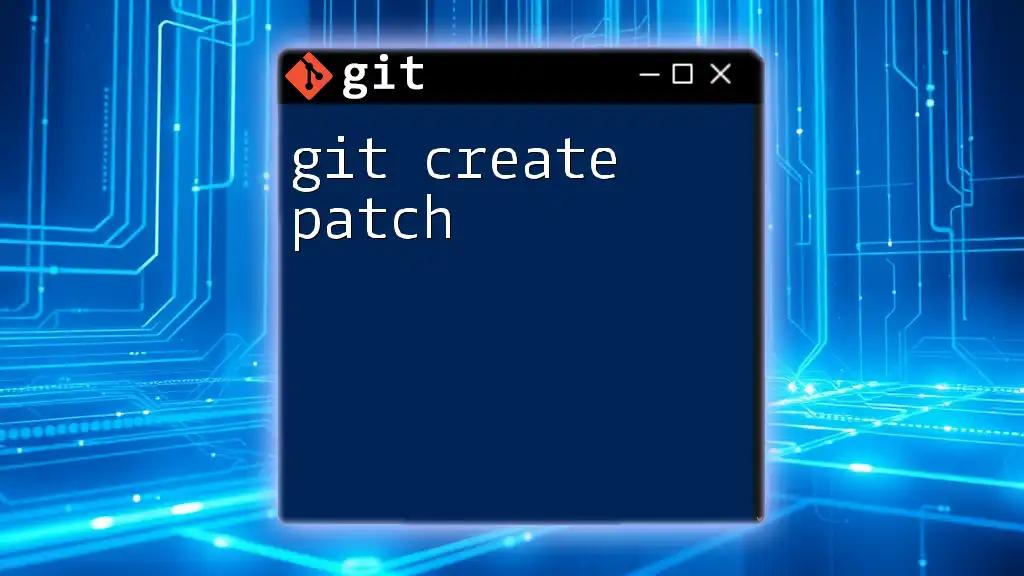
Creating a Git Tag
Prerequisites
Before diving into creating tags, ensure that you have Git installed on your machine and that you have initialized a Git repository (or cloned an existing one). If you haven't done so, you can initialize a new repository with:
git init
Creating a Lightweight Tag
Creating a lightweight tag takes just a simple command. Just remember that this tag will not have additional information beyond the reference to the commit. To create a lightweight tag, just run:
git tag <tag-name>
If you’ve just made a commit and want to tag it, you can do so immediately. For example:
git commit -m "Add new feature"
git tag lightweight-tag
This command allows you to quickly put a mark on your latest work without any overhead.
Creating an Annotated Tag
To create an annotated tag, you'll often take more time to articulate what the tag represents, making it a more professional approach in most cases. Use the following command:
git tag -a <tag-name> -m "Your message here"
For instance, this command can signify a major release:
git tag -a v1.0 -m "First major release with new features"
Creating annotated tags not only preserves the commit reference but also enhances collaboration by documenting the intent behind the tagged release or milestone.

Managing Tags
Listing Existing Tags
Once you begin tagging, you'll want to keep track of them. To list all existing tags in your repository, simply run:
git tag
This command will provide a neat list of all tags, allowing you to see what milestones you have marked. Knowing your tags is essential for managing releases or navigating your project's history.
Viewing Tag Details
If you want to dive deeper into any specific tag, you can use:
git show <tag-name>
This command displays detailed information about the tag, including the commit it points to and any accompanying message. For example:
git show v1.0
This will detail the commit associated with the `v1.0` tag, allowing you to review changes made in that release.
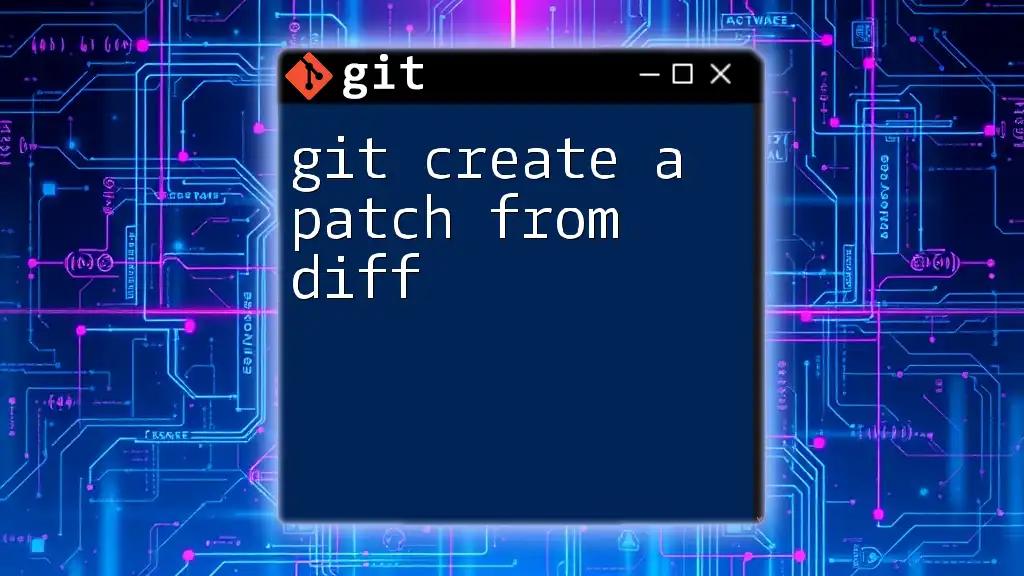
Working with Remote Tags
Pushing Tags to Remote Repository
Once you've created a tag locally, you may want to share it with your team or deploy it. To push a specific tag to your remote repository, use the following command:
git push origin <tag-name>
This command allows others to access the same historical reference. For example:
git push origin v1.0
Pushing All Tags
If you’ve created several tags that you want to share, you can push all tags at once by executing:
git push --tags
This command is particularly useful in scenarios where you’ve tagged multiple releases or significant commits in one go. It ensures that your remote repository remains in sync with your local tags.
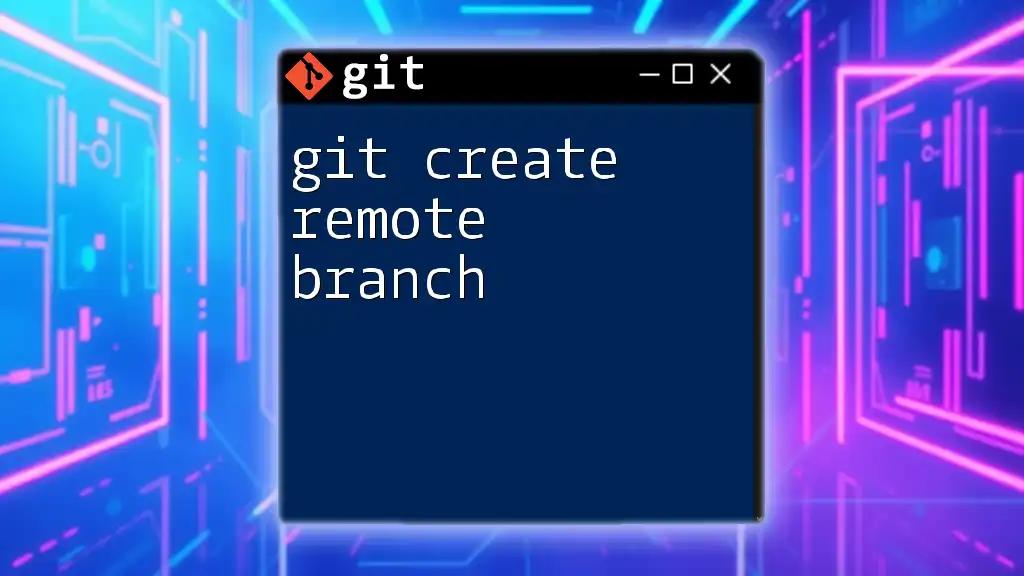
Deleting Tags
Deleting a Local Tag
Tags are not immutable, and there might be times when you need to delete a tag. If you find that a tag is no longer relevant, you can easily remove it from your local repository:
git tag -d <tag-name>
For instance, if you made an error in tagging `v1.0`, you can delete it as follows:
git tag -d v1.0
Deleting a Remote Tag
If a tag has been pushed to a remote repository, you may also want to delete it from there. Use the following command:
git push --delete origin <tag-name>
For example, to remove the `v1.0` tag from your remote repository, run:
git push --delete origin v1.0
Keep in mind that deleting tags should be done with care, especially in collaborative environments, as it may confuse other team members relying on those tags.
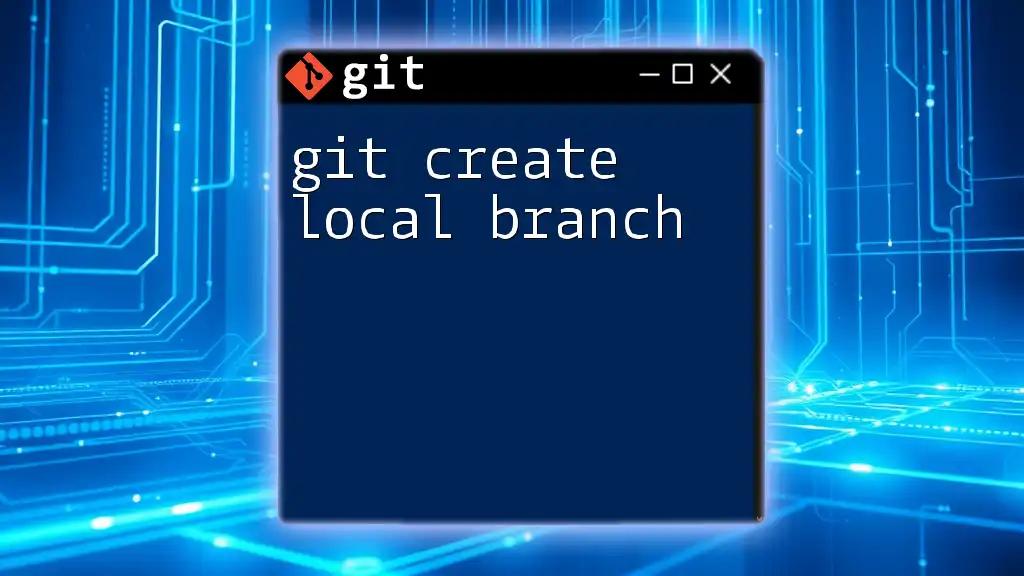
Best Practices for Tagging
Consistent Naming Conventions
When creating tags, consistency is crucial. Use clear and descriptive names that convey the purpose of the tag, such as version numbers (e.g., `v1.0`, `v2.1`) or descriptive identifiers (e.g., `feature-completed`, `bugfix-issue-123`). This makes it easier for anyone working on the project to understand the repository's history at a glance.
Tagging Releases
Tags are particularly valuable for marking software releases. They help create a clear historical reference point, allowing developers to quickly navigate to stable versions of the codebase. When deploying software, tagging corresponding commits ensures you can revert to known stable states when necessary, which enhances project management and provides peace of mind during deployment.
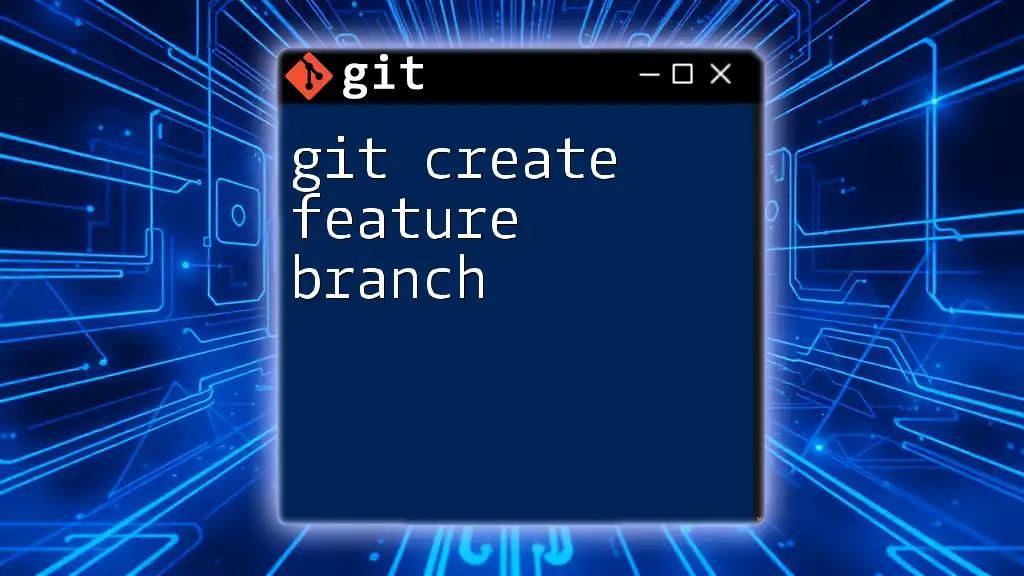
Conclusion
Tags are a powerful feature in Git that can significantly enhance your workflow by providing meaningful checkpoints in your project's history. By mastering how to create and manage tags—whether lightweight or annotated—you'll improve not only your individual productivity but also your team's collaboration and effectiveness. Embrace the power of `git create tag` in your development practices to maintain a well-structured and navigable repository.
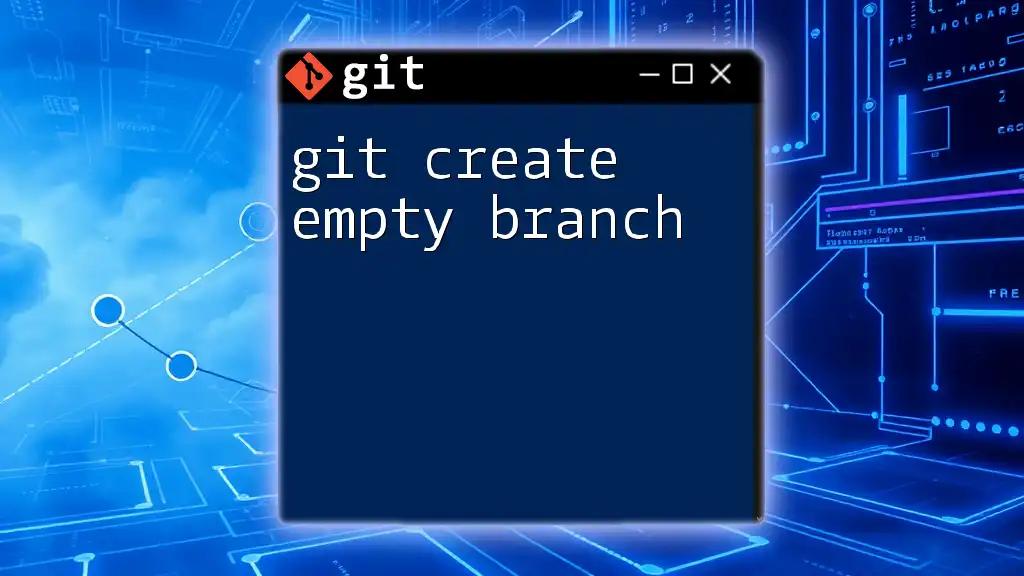
Additional Resources
To deepen your understanding of Git tags and version control, be sure to check out the official Git documentation and explore tutorials that dive deeper into Git commands. Mastering these concepts will turn you into an efficient and effective developer.
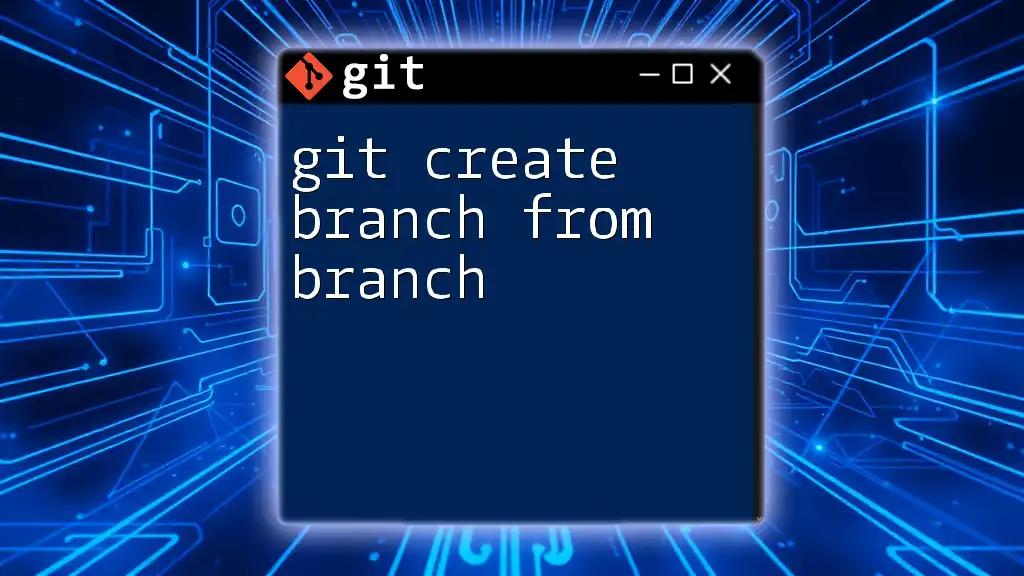
FAQs
What is the main difference between a tag and a branch in Git?
Tags mark specific points in history, while branches signify a separate line of development. Tags do not change once created; branches can evolve and diverge over time.
Can I rename a tag?
You cannot directly rename a tag in Git. Instead, you would delete the existing tag and create a new one with the correct name.
What happens to a tag when I delete the commit it points to?
Once the commit associated with a tag is deleted, the tag becomes detached but remains in the repository. You cannot use a tag if its commit is no longer in the commit history, but the tag will still exist unless explicitly deleted.