To create a patch file from the differences between the working directory and the last commit in Git, you can use the `git diff` command followed by output redirection to save the differences in a `.patch` file.
git diff > changes.patch
Understanding `diff`
What is `diff`?
The `diff` utility is a powerful tool that calculates the differences between two files or directories. It outputs the lines that differ, helping developers to quickly identify changes in source code or other text files. Understanding how to read and utilize `diff` output is essential for anyone working in collaborative environments or involved in code review processes.
Basic `diff` Usage
To use the `diff` command, you simply specify which two files you want to compare:
diff [options] file1 file2
For example, if you have two text files named `file1.txt` and `file2.txt`, you would run:
diff file1.txt file2.txt
This command will show you the lines that differ between the two files, making it easier to pinpoint changes.
Understanding Diff Output
The output of the `diff` command includes several common symbols:
- `+`: Indicates a line that has been added in the second file.
- `-`: Indicates a line that has been removed from the first file.
- `c`: Represents a change between the two files.
Familiarity with this output will enhance your ability to track and review changes efficiently.
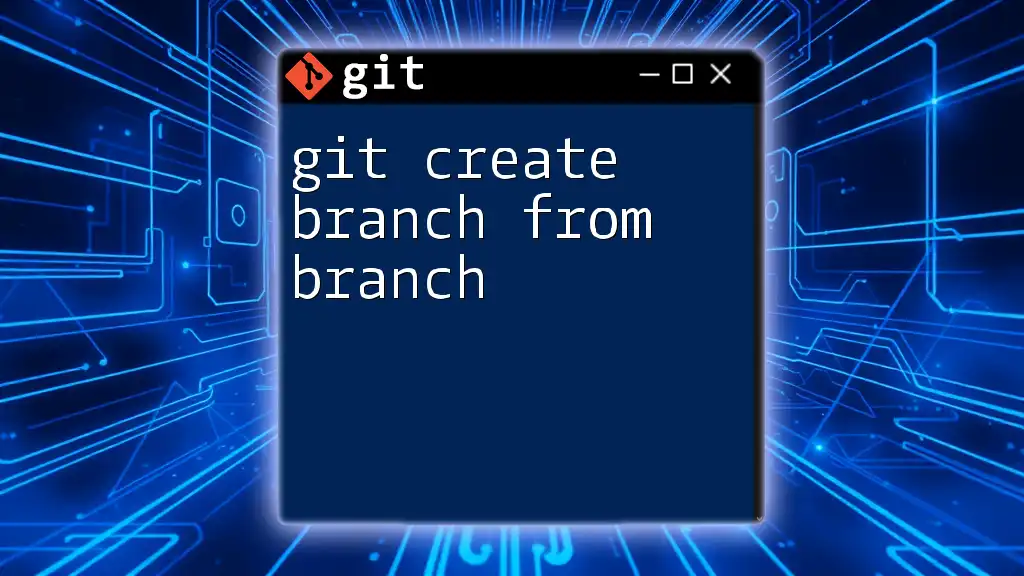
Creating a Git Patch from Diff
What is a Git Patch?
A Git patch is a file that contains a minimal set of changes made to the source code. This format is particularly valuable when you want to share your modifications with others or apply them in a different branch or repository. Git patches can greatly facilitate collaboration, as they provide a clear snapshot of the changes, complete with context.
Generating a Patch in Git
Using `git diff`
You can create a patch from your uncommitted changes using the `git diff` command. Here's how the command syntax works:
git diff [options]
For instance, if you want to capture all the changes you’ve made but haven’t committed yet, you can run:
git diff > my_changes.patch
This command exports the current uncommitted changes to a file named `my_changes.patch`. It’s essential to understand that this file will include all modifications from your working directory.
Producing a Patch from Commits
You can also create patches based on specific commits. The `git diff` command allows you to specify two commits to compare:
git diff commit1 commit2
For example, to generate a patch for the last commit compared to the one before it, you would execute:
git diff HEAD~1 HEAD > my_patch.patch
In this command, `HEAD~1` refers to the previous commit, and `HEAD` refers to the most recent commit. This approach helps create targeted patches for the exact changes you want to share or apply.
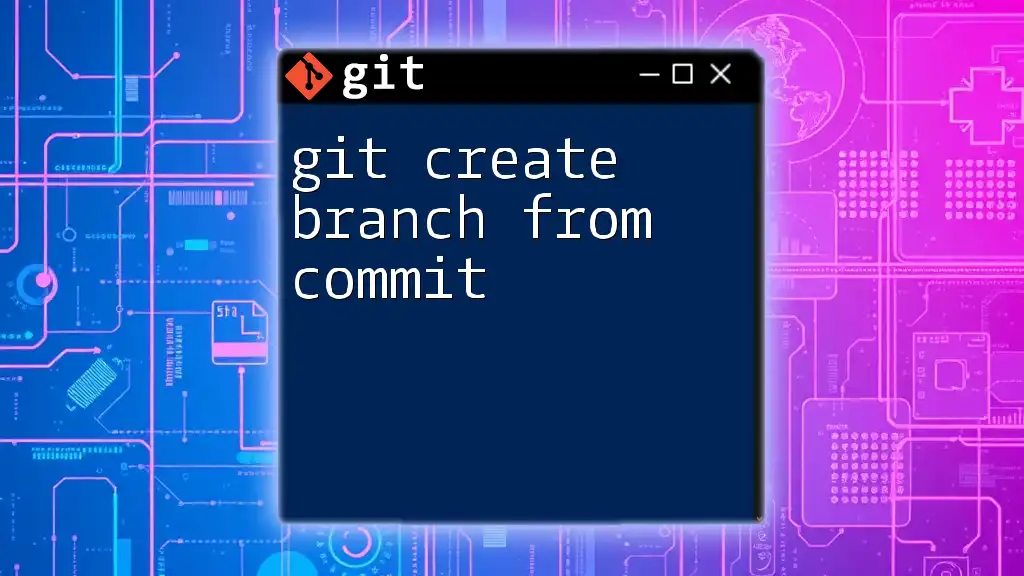
Customizing Output with Diff Options
Common `diff` Options
Unified Diff Format
Creating a unified diff format can improve readability. The command allows you to visualize changes in a more condensed way:
git diff --unified
This command will format the output to include context around the changes, making it easier for reviewers to understand the modifications in context.
Creating Patched Files with Context
Moreover, the `-U` flag lets you define how many context lines to show. For instance:
git diff -U5 > detailed_patch.patch
This command will create a patch that includes five lines of context before and after the changes, providing valuable insight into the surrounding code.
Redirecting Output to a Patch File
Understanding how to redirect output to a specific file is crucial. You can easily save the output of a `git diff` command to a given file:
git diff commit1 commit2 > my_specific_patch.patch
This method helps in generating specific patches without cluttering your terminal with output.
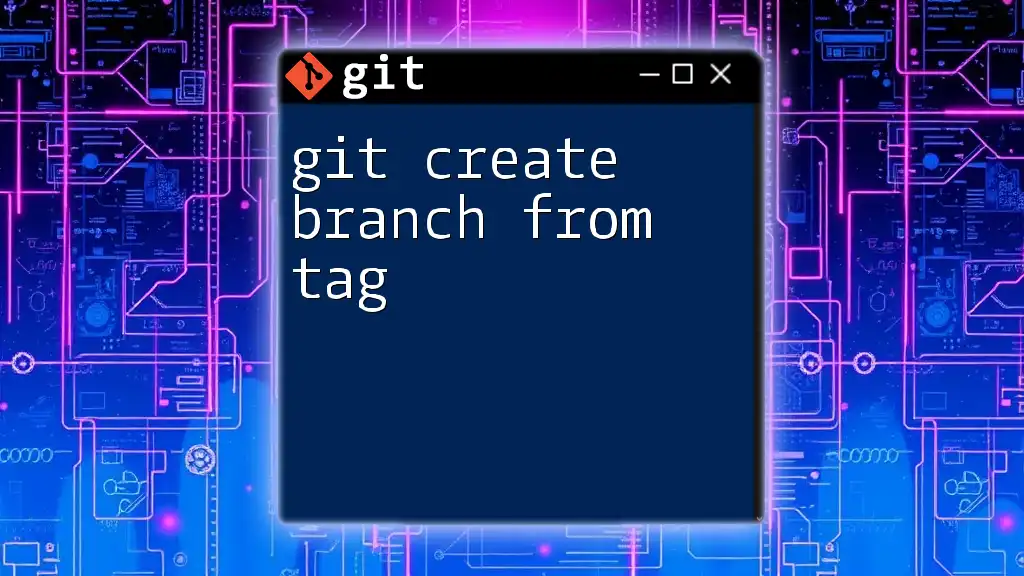
Applying a Patch
Using `git apply`
Once you have a patch file, applying it is straightforward with the `git apply` command. This command applies changes from a patch file to your working directory:
git apply [options] <patchfile>
For instance, if you want to apply the patch saved in `my_changes.patch`, you’d simply execute:
git apply my_changes.patch
This will integrate the changes represented in the patch into your current working directory.
Checking Patch Before Application
Before applying changes, it’s wise to check the patch compatibility using the `--check` option. This prevents potential issues:
git apply --check my_changes.patch
Running this command will help you verify that applying the patch will not result in merge conflicts or errors, thus saving you time and trouble.
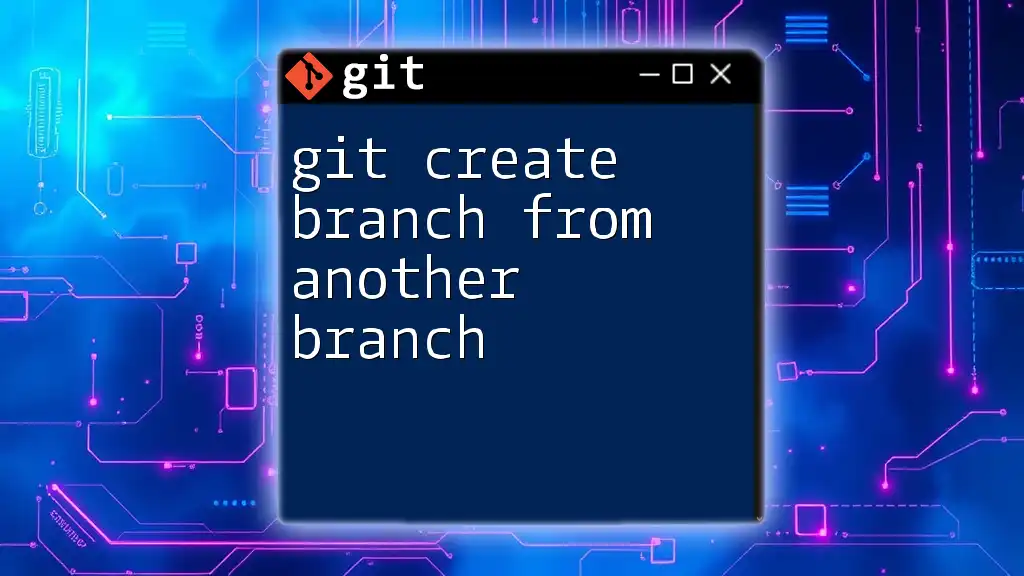
Real-World Use Cases
Code Review and Collaboration
Patches are invaluable in code review processes. When sending modifications for review, a developer can generate a patch file that reviewers can easily apply and test. This method keeps discussions focused and clear, as the contributions are solidly documented.
Bug Fixes and Feature Additions
Patches can also facilitate the submission of bug fixes or feature additions in open-source projects. Developers can create a patch for their changes and submit it to the project maintainers, who can then review and merge it as necessary. This process streamlines contributions and enhances collaboration across development teams.
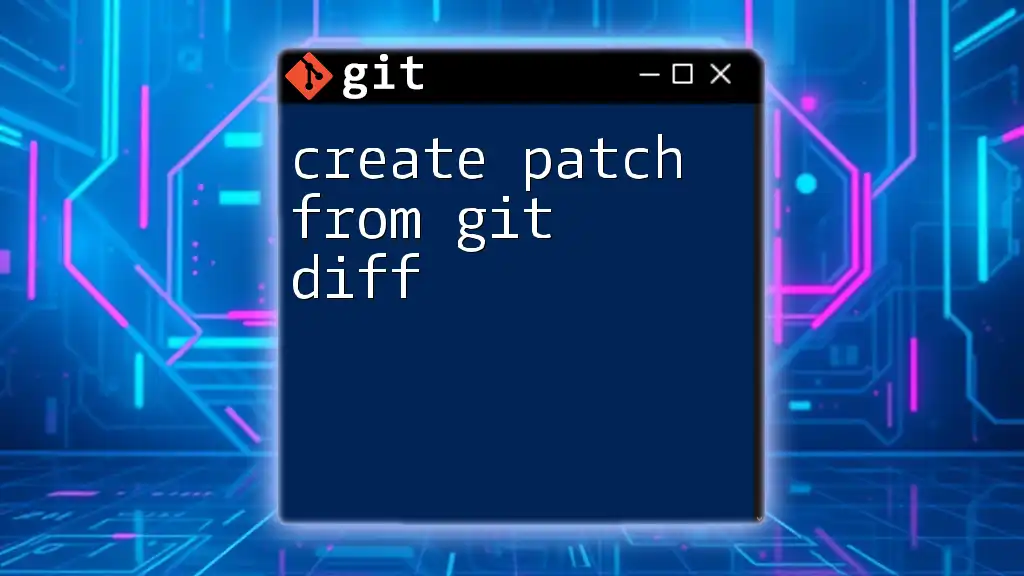
Conclusion
Creating and applying patches using the `git create a patch from diff` methodology streamlines collaboration and enhances communication within development teams. Understanding how to generate patches through committed changes and uncommitted modifications equips developers with essential tools for efficient version control.
As you integrate these techniques into your workflow, you will find that they not only improve your productivity but also enhance your readiness for collaborative development. Embrace these practices today and become a more effective collaborator in the world of Git.
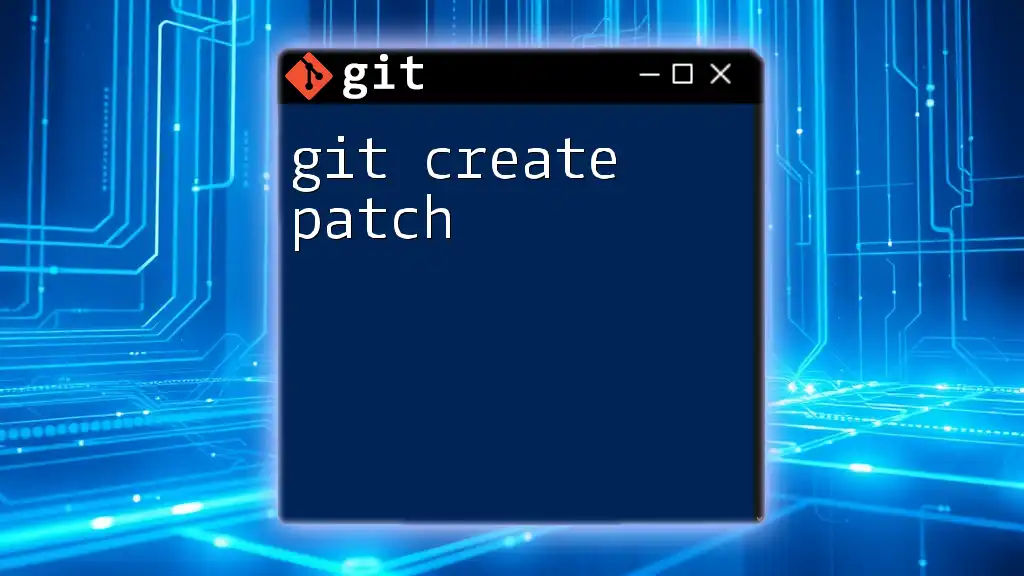
Additional Resources
For further exploration of Git patches and related commands, consider diving into the official Git documentation or seeking online courses tailored for Git and version control best practices. These resources will provide you with additional insights and techniques to improve your development skills.
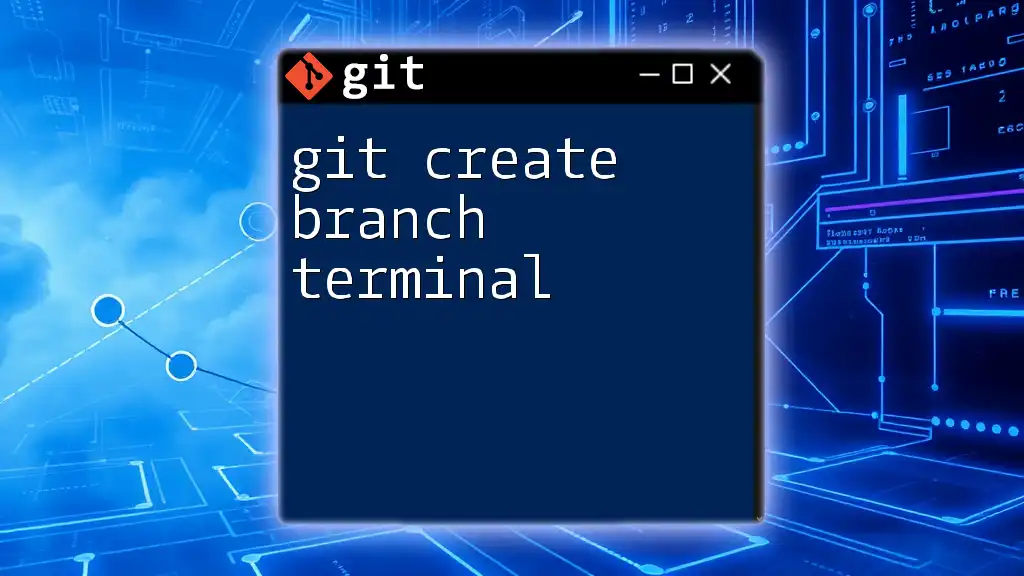
Call to Action
Take the next step in mastering Git commands and consider signing up for our workshops, where you can gain hands-on experience with Git and learn to utilize these techniques effectively for your projects.