To create a new feature branch in Git, use the following command, replacing `feature-branch-name` with your desired branch name:
git checkout -b feature-branch-name
What is a Feature Branch?
A feature branch is a dedicated branch in a Git repository used to develop a new feature or functionality without affecting the main codebase. This allows developers to work in isolation, making it easier to manage changes, avoid conflicts, and maintain a clean main branch.
Using feature branches provides various benefits, including:
- Isolation of new features: Developers can work on new features without interrupting others or affecting the stable version of the code.
- Collaboration: Teams can collaborate on different features simultaneously, ensuring that each branch can be developed, tested, and reviewed independently.
- Easier debugging and testing: Any bugs or issues can be addressed within the feature branch before merging back into the main branch.
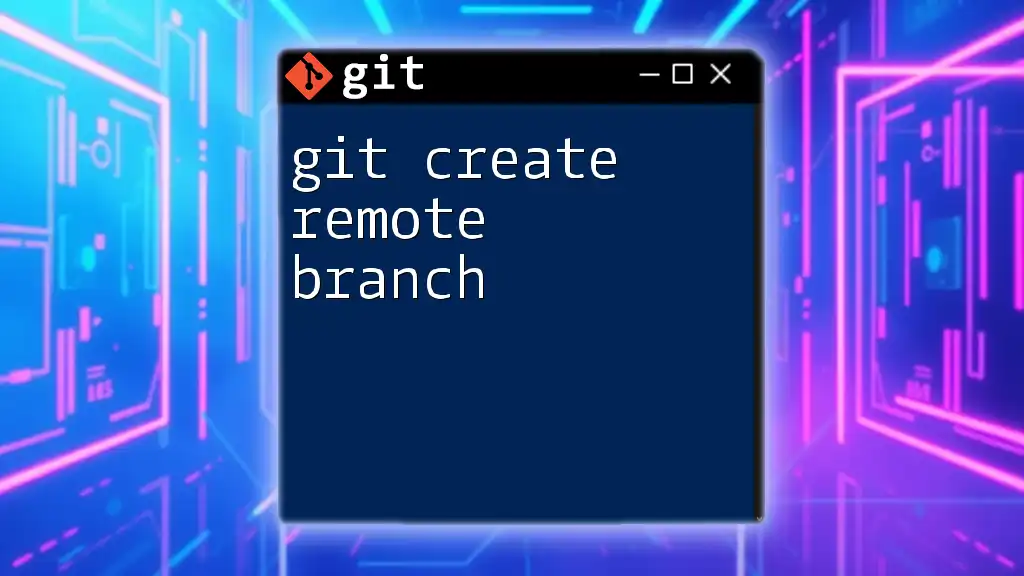
Prerequisites
Before diving into creating a feature branch, it’s essential to have a basic understanding of Git. If you're new to Git, familiarize yourself with its fundamental concepts and commands.
To get started, ensure that Git is installed on your machine. Here are quick installation steps for various operating systems:
- Windows: Download the Git installer from the [official Git website](https://git-scm.com/download/win) and follow the prompts.
- macOS: You can install Git using Homebrew with the command:
brew install git
- Linux: Use your package manager (e.g., `apt` for Ubuntu):
sudo apt-get install git
Once Git is installed, configure your environment with your name and email, which will be used for your commits:
git config --global user.name "Your Name"
git config --global user.email "your_email@example.com"
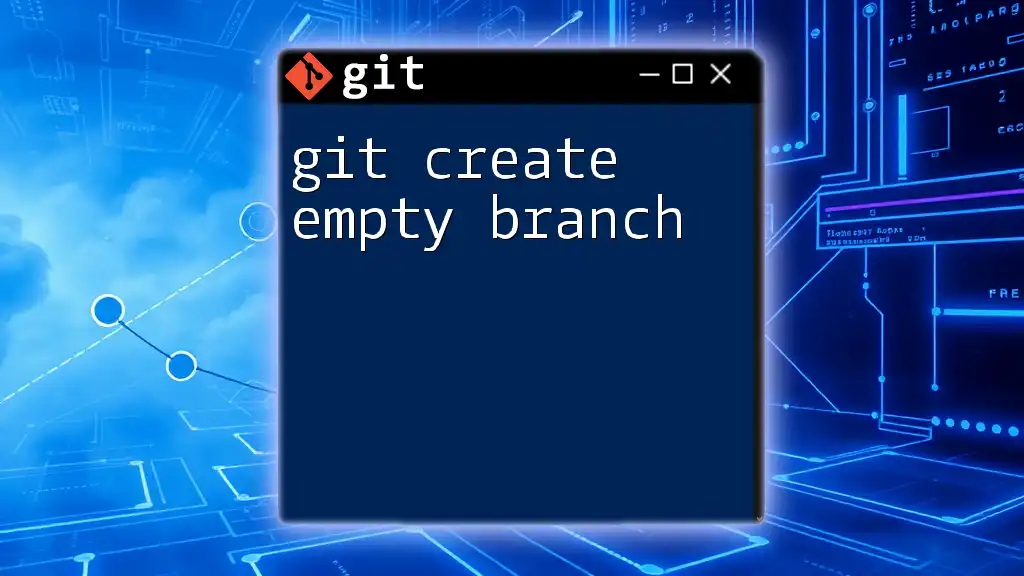
How to Create a Feature Branch
Understanding the Git Branch Workflow
Git's branching model allows for multiple divergent works within a single repository. The main branch typically holds the production-ready code, while feature branches are used to develop new features separately. This separation helps maintain stability in the main branch while developers can experiment and innovate in their feature branches.
Steps to Create a Feature Branch
Step 1: Check the Current Branch
It’s crucial to know which branch you are currently on before creating a new feature branch. Use the following command:
git branch
This will list all the branches in your repository, highlighting the one you are currently active in.
Step 2: Creating the Feature Branch
To create a new feature branch, you can use the command:
git checkout -b feature/my-new-feature
In this command, `feature/my-new-feature` is the name of your new branch. It’s a good practice to use descriptive names that explain the purpose of the feature you’re implementing.
Step 3: Verifying the Branch Creation
After creating the new branch, it's important to confirm that you've switched to it successfully. Run the following command again:
git branch
You should see your new feature branch listed and highlighted as the current branch.
Best Practices for Naming Feature Branches
When naming your feature branches, consider these guidelines for clarity:
- Be descriptive: Use names that describe the feature being developed; for example, `feature/add-login`, `feature/update-user-profile`.
- Use lowercase letters: This helps maintain consistency and avoid confusion when working on case-sensitive systems.
- Include issue numbers: If you use a project management tool, include the related issue number (e.g., `feature/123-add-login`) to facilitate tracking.
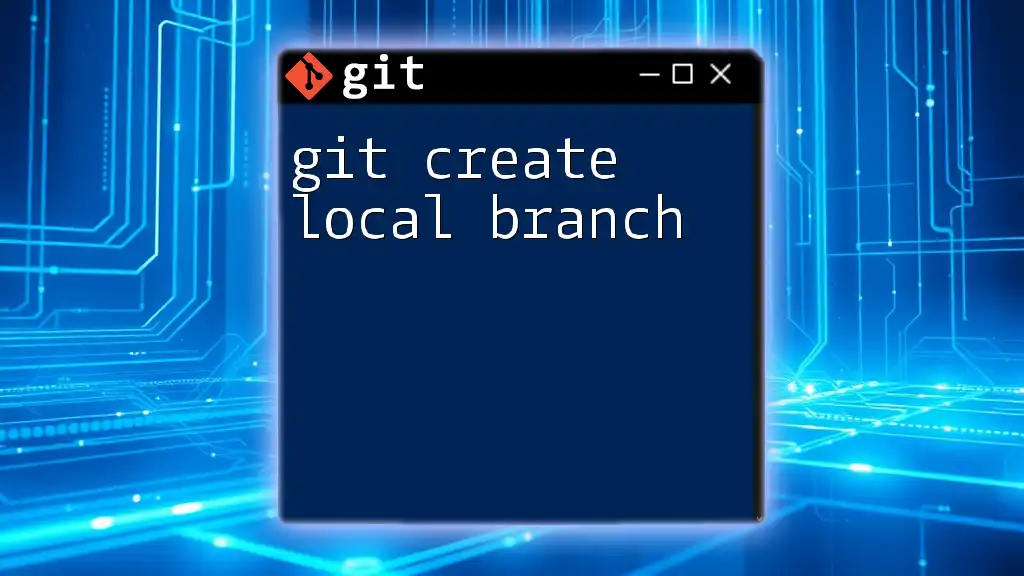
Working on the Feature Branch
Making Changes and Commits
Once your feature branch is created and you’re actively working on it, you can begin making changes to your codebase. After making your changes, you need to stage and commit them. Here’s how:
-
Stage the changes:
git add .
This command stages all modified and new files. Alternatively, you can stage specific files by replacing `.` with the file names.
-
Commit the changes:
git commit -m "Add new login feature"
The `-m` flag allows you to attach a short commit message that describes your changes.
Pushing the Feature Branch to Remote
After making and committing your changes to the feature branch, you may want to share it with your team. Use the following command to push your branch to the remote repository:
git push origin feature/my-new-feature
This uploads your feature branch to the remote repository 'origin', making it accessible for others.
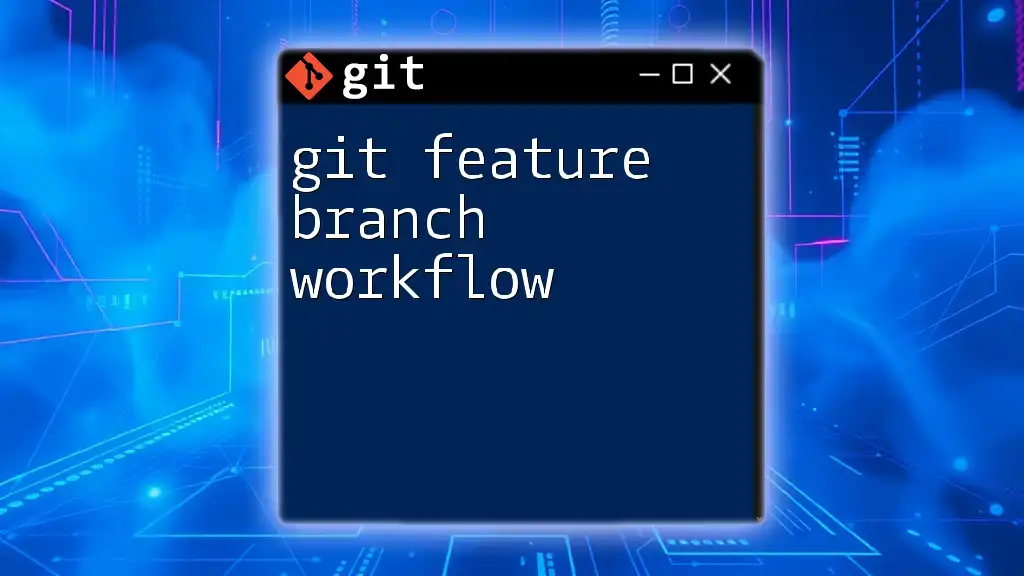
Merging the Feature Branch
Preparing for Merge
Before merging your feature branch, it’s essential to ensure your main branch is up to date with the latest changes from the remote repository. Follow these steps:
-
Switch to the main branch:
git checkout main
-
Pull the latest changes from the remote main branch:
git pull origin main
Performing the Merge
With your main branch updated, you can proceed to merge the feature branch. Use the following commands:
git merge feature/my-new-feature
This command merges the changes from `feature/my-new-feature` into your current branch (main).
Resolving Merge Conflicts
During the merging process, you may encounter merge conflicts, which occur when changes in both branches overlap. Git will mark the areas of conflict, and you'll need to manually resolve them within your code editor. After resolving the conflicts, stage and commit the changes as follows:
git add .
git commit -m "Resolve merge conflicts"
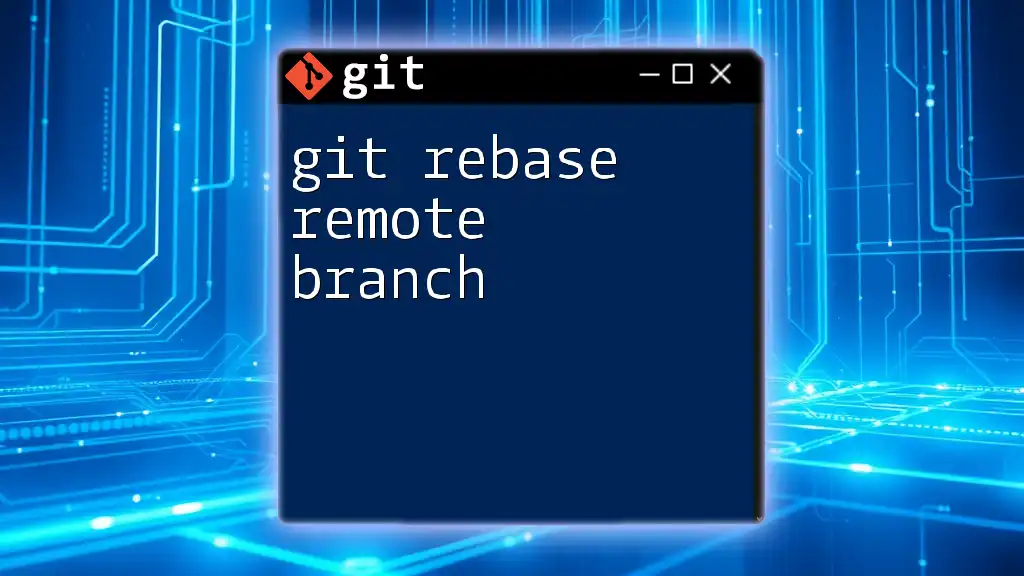
Deleting a Feature Branch
Cleanup After Merging
Once your feature has been successfully merged into the main branch, it’s a good practice to delete the feature branch to keep your repository tidy. Here’s how you can do that:
-
Delete the local branch:
git branch -d feature/my-new-feature
-
Delete the remote branch (if applicable):
git push origin --delete feature/my-new-feature
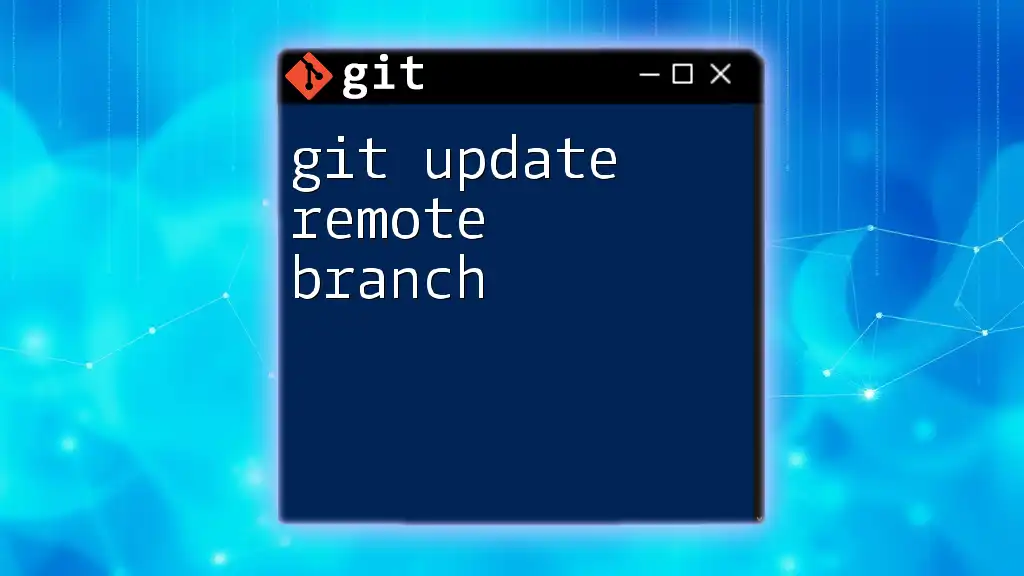
Conclusion
Creating a feature branch in Git is a fundamental practice that allows developers to work on new features independently and without disrupting the main codebase. By mastering the process of creating, managing, and merging feature branches, you can streamline your development workflow, ensure code stability, and foster better collaboration within your team.
Remember to continually practice creating feature branches and encourage your team to adopt this workflow for a more efficient and organized development process.
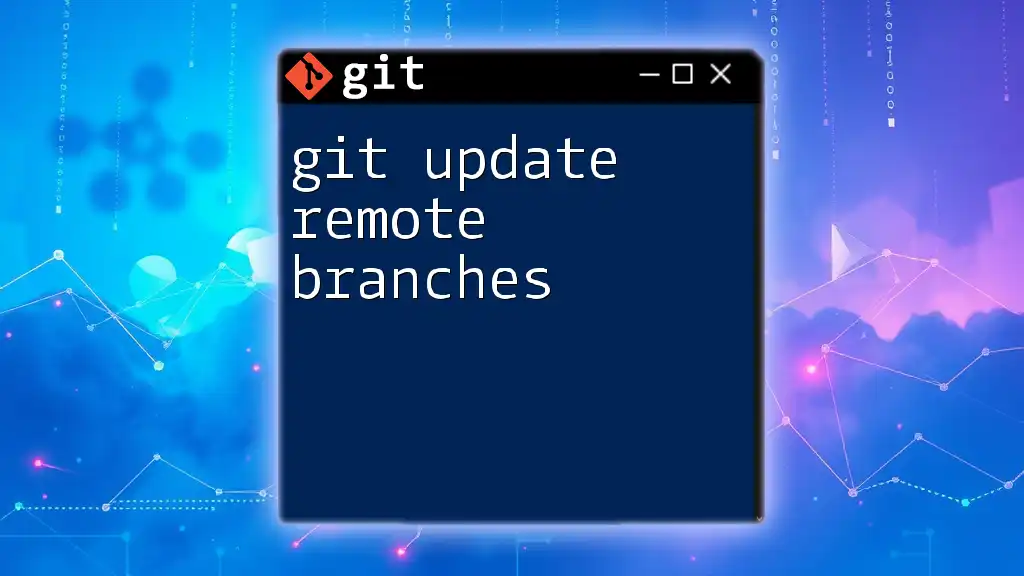
Additional Resources
For further learning, explore the official Git documentation and tutorials available online. Familiarize yourself with GitHub and GitLab integrations to enhance your development experience.
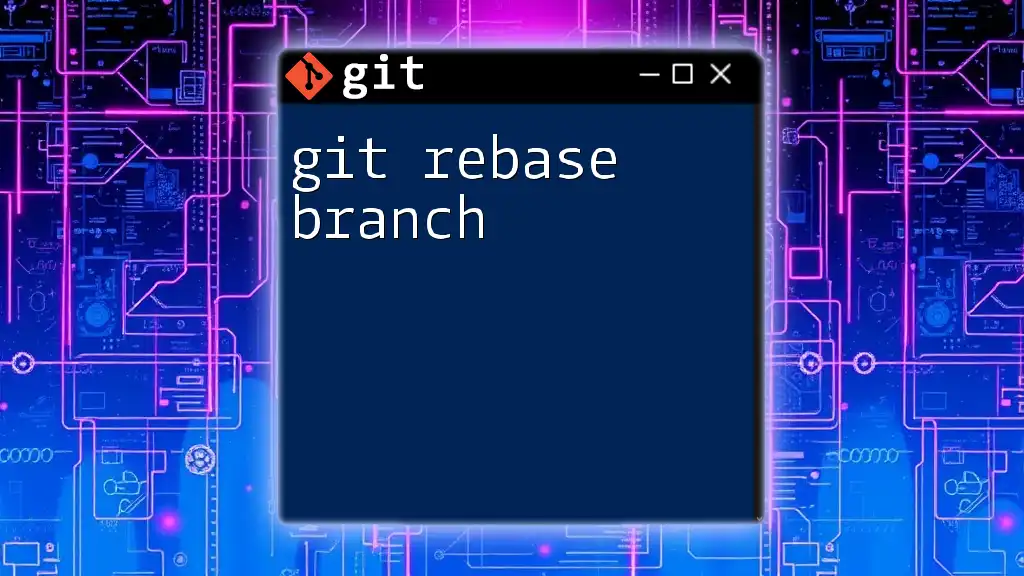
FAQs
- What are the advantages of using feature branches?
- How do I handle frequent conflicts in feature branches?
- Can I create multiple feature branches at once?
These common queries can help clarify any lingering doubts you may have regarding the use of feature branches in Git.