When you encounter a "git patch does not apply" error, it usually means that the changes in the patch file cannot be applied cleanly to the current branch, often due to conflicts with existing changes or differences in file context.
Here's a basic command to check the differences before applying a patch:
git apply --check your_patch_file.patch
Understanding Git Patches
What is a Git Patch?
A Git patch is essentially a file that contains a list of differences (or "diffs") between two sets of files, which is generated to represent changes in a repository. Patches are particularly useful for sharing changes with others, applying fixes across branches, and performing code reviews without needing direct access to a repository. When you create a patch, you're encapsulating your changes in a format that can easily be transmitted, emailed, or stored.
Common Use Cases for Git Patches
-
Sharing patches between developers: When you need to collaborate with team members who may not have direct access to your repository, a patch allows you to share modifications easily.
-
Applying changes across different branches or repositories: Patches can bridge the gap between various branches or even separate repositories, allowing for smoother integration of changes.
-
Staging changes for review before committing: By creating a patch, you can review your modifications and share them for input without immediately altering the main codebase.
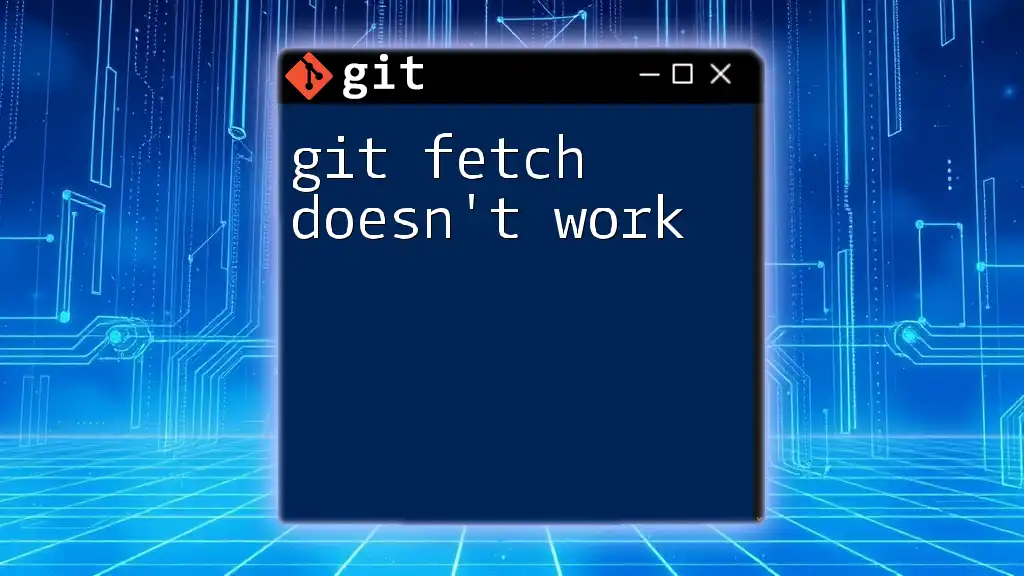
Causes of "Git Patch Does Not Apply"
File Conflicts
A primary reason for the error message "git patch does not apply" is file conflicts. Conflicts happen when the actual content of the files in the target branch has diverged from those referenced in the patch, making the patch's changes impossible to apply cleanly. For instance, if you attempt to apply a patch from a feature branch onto a main branch that has seen substantial alterations, Git will struggle to reconcile the differences, resulting in errors.
Context Errors
Context errors arise when the lines surrounding the changes specified in the patch do not match those in the target files. When creating a patch, Git captures contextual lines to ensure accuracy, and any alterations in this area can prevent the patch from being successfully applied.
For example, imagine your patch adds a new feature, but those lines have been deleted or altered in the target branch. You might encounter a context error displayed as:
error: patch failed: path/to/file:xyz
error: path/to/file: patch does not apply
Differences in Line Endings
Another common issue is the difference in line endings, particularly between Windows (CRLF) and Unix (LF). If your patch was created on a system supporting one line ending but applied to a system with a different setting, you may see the error “git patch does not apply.” This variance can create confusion, as the same files may appear different to the Git engine due to these line-ending discrepancies.
Changes in File Structure
If there were renames, moves, or deletions of files after the patch was created, it may lead to unsuccessful patch applications. If a file is renamed in the target branch but referenced in a patch by its old name, Git won’t find it, which would produce an error.
Consider the following example: If your patch modifies `src/fileA.py`, but in the target branch, `fileA.py` has been renamed to `src/fileB.py`, you will encounter issues applying the patch.
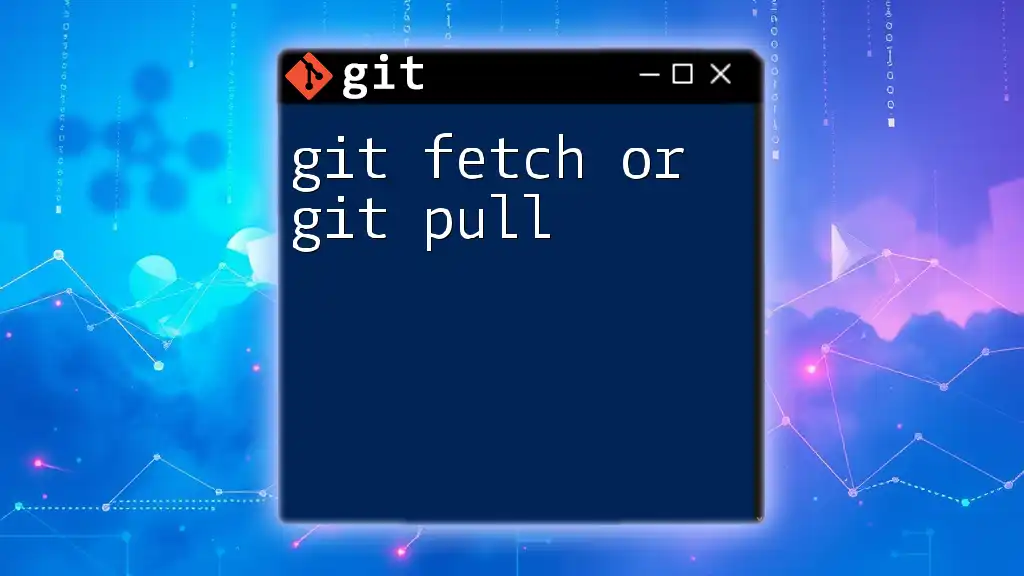
Troubleshooting "Git Patch Does Not Apply"
Checking Patch Contents
When faced with a patch application issue, the first step is to examine the contents of the patch file. Run:
git apply --check path/to/your.patch
This command checks the patch file against the current state of the repository, helping identify potential problems before attempting to apply the patch. It can provide clear insights into what went wrong, giving you a better understanding of what to fix.
Using the '--reject' Option
If you're encountering persistent issues, consider utilizing the `--reject` option. This option allows you to apply portions of the patch that can be applied cleanly while creating `.rej` files for the hunks that were rejected. Use the command like this:
git apply --reject path/to/your.patch
This way, you can manually resolve the rejected chunks, allowing your other changes to be applied seamlessly.
Manual Application of Patches
If automated methods fail, you can resort to manual application of the patch. This process may be time-consuming but sometimes is the only solution. Review the patch's diffs in the patch file and make the corresponding changes directly in your files.
You can view the patch using:
cat path/to/your.patch
Make the changes line-by-line, carefully adhering to what the patch specifies. After incorporating the changes, use `git add` to stage them before committing.
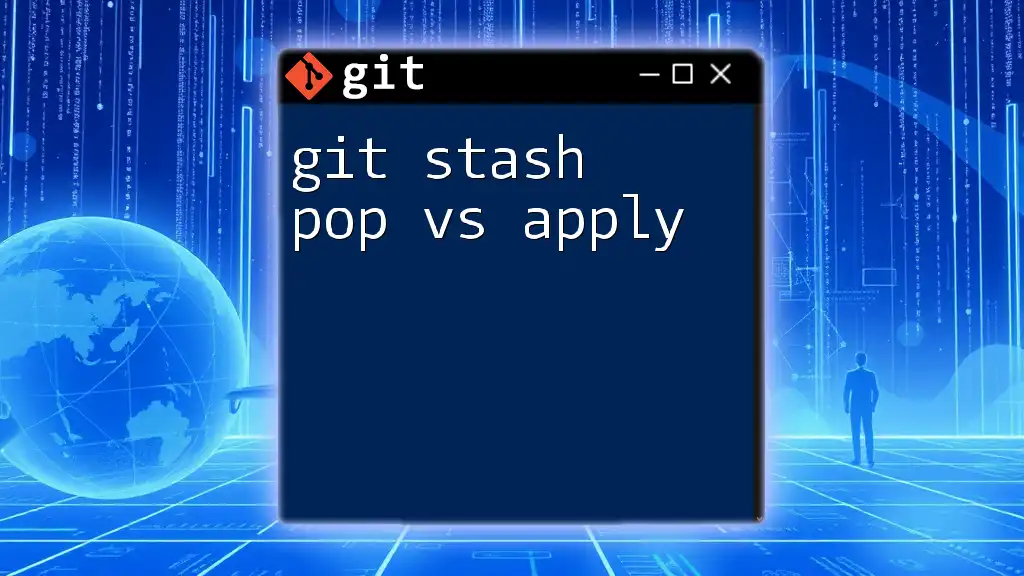
Preventing Patch Application Issues
Keeping Repositories Updated
Keeping repositories updated is crucial to minimize the chances of patch application problems. Frequently pull changes from the upstream repository to ensure you are working with the latest version. This habit helps prevent the divergence of file content that can lead to conflicts.
Collaboration Best Practices
Fostering effective collaboration reduces the risk of patch issues. Encourage team members to communicate about changes early and often. Implementing feature branches and using pull requests can streamline this process by stabilizing the main codebase while allowing developers to work on their individual contributions.
Commit Message Standards
Clear commit messages play a pivotal role in creating effective patches. Ensure that commit messages describe the changes accurately and how they relate to the overall project. For instance:
Improve error handling in user authentication process
Communicating what a patch does makes it easier for others to understand any overlaps or potential issues before applying the patch.
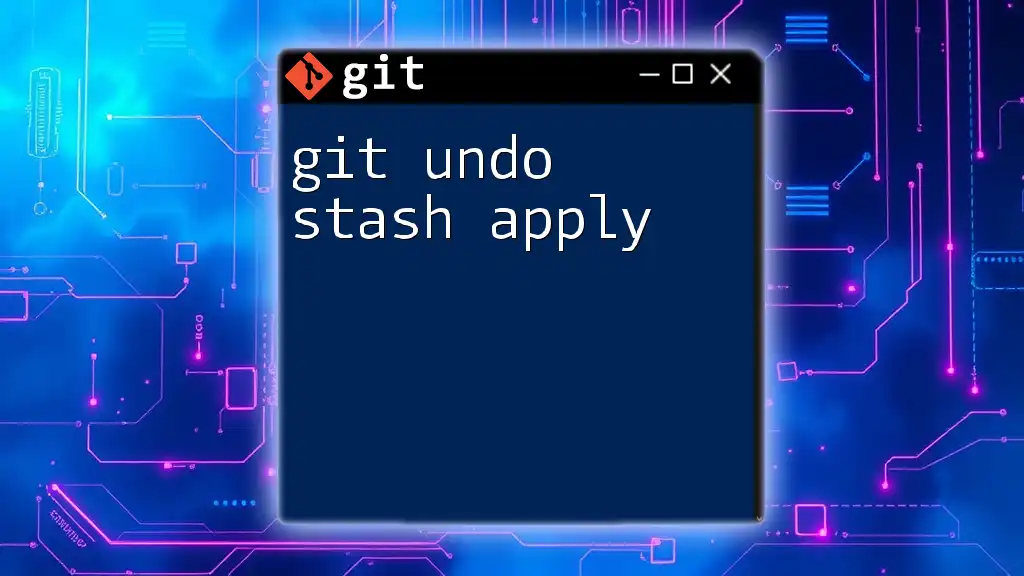
Advanced Techniques for Patch Management
Using `git-format-patch`
To create patches suitable for email or dissemination, consider using `git-format-patch`. This command converts commits into patches that retain a better context for review.
Here’s how to create a patch for the last commit:
git format-patch HEAD~1
This technique allows you to keep a detailed history of your changes while ensuring that recipients can easily apply them without confusion.
Interactive Rebasing and Patch Application
Another advanced technique is interactive rebasing, which allows for more nuanced control over your commit history. By rebasing interactively, you can modify patches before applying them, ensuring that potential conflicts are addressed beforehand.
Utilizing Other Tools
Last but not least, consider using graphical tools to assist with managing patches. Tools like GitKraken or SourceTree provide intuitive interfaces that make it easier to visualize changes, manage patches, and resolve conflicts more effectively. These tools can save time and make the patch application process more user-friendly.
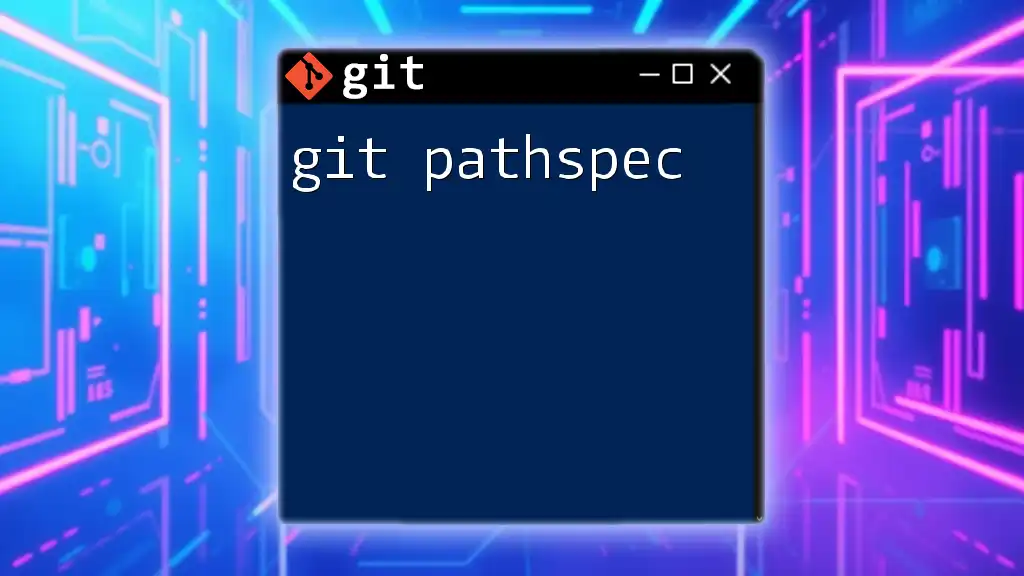
Conclusion
Understanding the reasons behind the "git patch does not apply" message can significantly enhance your ability to manage patches effectively. By familiarizing yourself with common causes and troubleshooting techniques, you can ensure a smoother development experience. A proactive approach to patch management minimizes conflicts and enhances collaboration, ultimately leading to a more efficient development cycle. Don't hesitate to reach out and explore more through your company’s Git training sessions for mastering these essential skills.
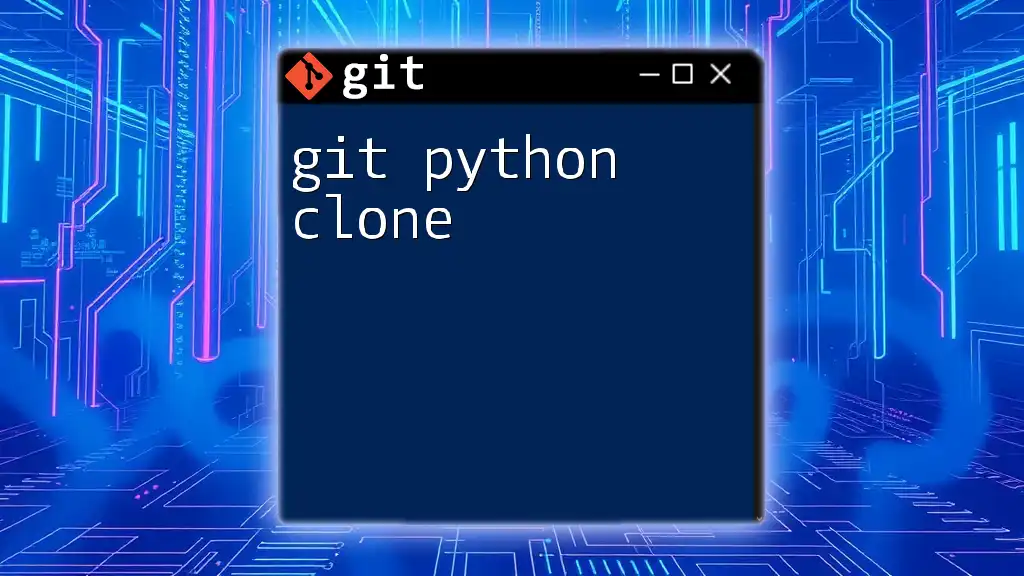
Frequently Asked Questions (FAQ)
What should I do if a patch fails to apply?
Start by running `git apply --check [patch_file]` to understand the root of the problem. Resolve any conflicts or errors identified, and try to apply the patch again.
Can I revert a patch if it causes issues?
Yes, you can revert a patch using the `git apply -R` command. This command will undo the changes introduced by the patch if they were successfully applied.
How can I ensure my patches are compatible with others' branches?
Regularly sync with the upstream changes and communicate with your team about changes being made. Using feature branches and holding departures from the main codebase until they are ready to merge also helps maintain compatibility.