The Git credential helper is a tool that simplifies the process of authenticating to remote repositories by storing and managing your Git credentials securely.
Here's how to set up the credential helper to cache your credentials for a specified time:
git config --global credential.helper cache
git config --global credential.helper 'cache --timeout=3600'
Understanding Git Credentials
Git credentials consist of the necessary authentication details required to access remote repositories. These can include:
- Username: Your account name on the Git hosting service.
- Password: The password associated with your account.
- SSH Keys: A more secure method that uses cryptographic keys instead of passwords.
- Tokens: Personal access tokens used in place of passwords for enhanced security, especially on platforms like GitHub, GitLab, and Bitbucket.
Common Use Cases
Effective credential management becomes essential when dealing with multiple repositories, particularly in scenarios where users frequently push to or pull from remote repositories. The convenience of not having to re-enter credentials streamlines the workflow significantly, allowing developers to focus on writing code rather than managing access.

Types of Git Credential Helpers
Git offers several built-in credential helpers, each with unique functionalities designed to match different user preferences and operating systems.
Cache Credential Helper
The cache helper stores your Git credentials temporarily in memory for a configurable duration. This means you'd only need to enter them once during that time frame, allowing you to run multiple Git operations without interruption.
To enable the cache helper, use the following command:
git config --global credential.helper cache
The default cache timeout is 15 minutes. You can modify this duration by specifying a timeout in seconds:
git config --global credential.helper 'cache --timeout=3600'
This command changes the timeout to one hour, enhancing convenience during longer coding sessions.
Store Credential Helper
The store helper provides a more permanent solution by saving your credentials unencrypted in a plain text file. While this increases convenience, it also introduces a security risk, as these credentials can be easily accessed by anyone with file-level access.
To set up the store credential helper, execute the following command:
git config --global credential.helper store
Upon the next interaction that requires authentication, Git will prompt you for your username and password and subsequently store them in `~/.git-credentials` by default. It is crucial to consider the security implications of this method and ensure that access to this file is tightly controlled.
OS-Specific Credential Helpers
Windows Credential Manager
For Windows users, the Windows Credential Manager is an effective way to manage Git credentials securely. To utilize it, run the following command:
git config --global credential.helper wincred
This configuration enables Git to use the Windows Credential Manager to save and retrieve your credentials, moving towards a more secure storage solution.
macOS Keychain
On macOS, the Keychain Access utility provides a seamless way to manage your Git credentials. To use it, issue the following command:
git config --global credential.helper osxkeychain
When using this helper, Git stores your credentials in the Keychain, requiring you to enter them only once. The system takes care of storing and retrieving them securely.
Linux Keyring
For Linux users, the Linux Keyring can be integrated with Git to manage credentials. To employ this method, configure the helper as follows:
git config --global credential.helper libsecret
This setup leverages the Linux Keyring to keep your credentials secure and easily retrievable.
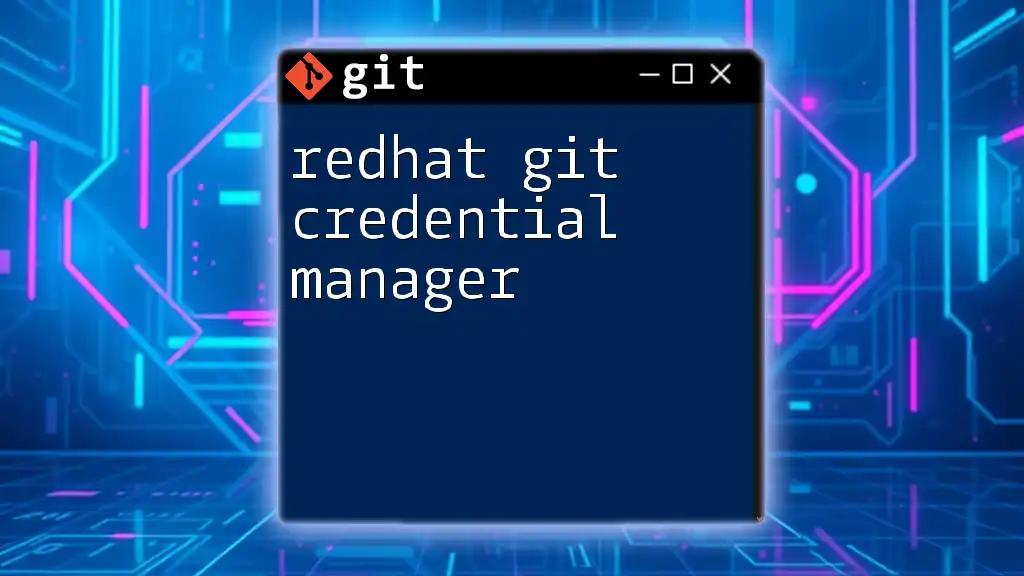
How to Set Up Git Credential Helper
Setting up the Git Credential Helper varies based on the operating system. Follow these steps tailored to your environment:
Windows Setup
- Open the Command Prompt.
- Execute the command to enable the Credential Manager:
git config --global credential.helper wincred
- You may need administrative privileges depending on your system settings.
macOS Setup
- Open the Terminal.
- Implement the following command:
git config --global credential.helper osxkeychain
- This command automatically applies your keychain settings.
Linux Setup
- Open a terminal window.
- If you haven’t already, install the required libraries to use the Keyring (consult your package manager).
- Then run:
git config --global credential.helper libsecret
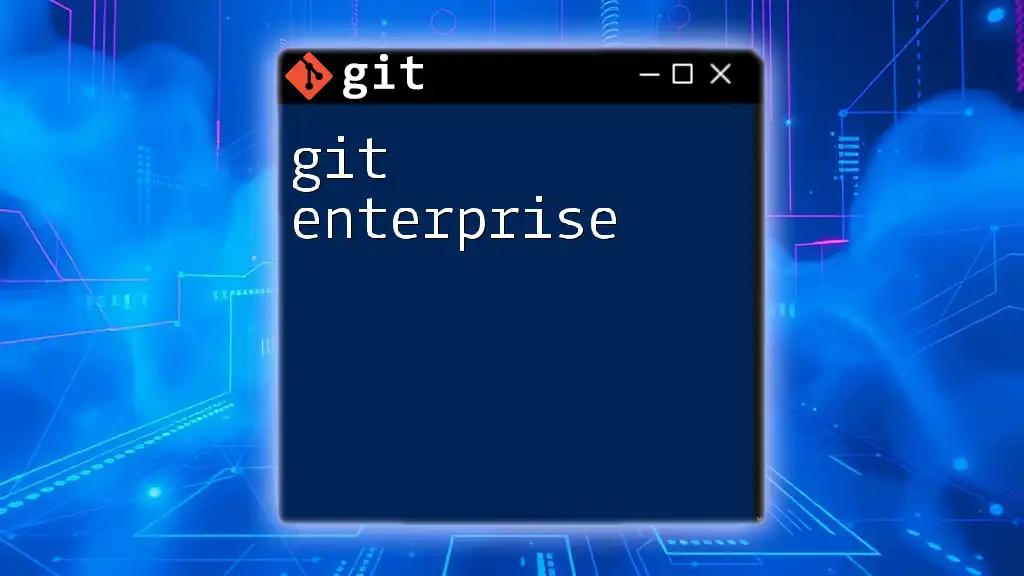
Testing Credential Helper Setup
After configuring your credential helper, it’s essential to test its functionality.
Run the following Git command:
git push
If everything is set up correctly, you should only be prompted for your credentials the first time you interact with the repository. Subsequent commands will authenticate you automatically using the stored credentials.
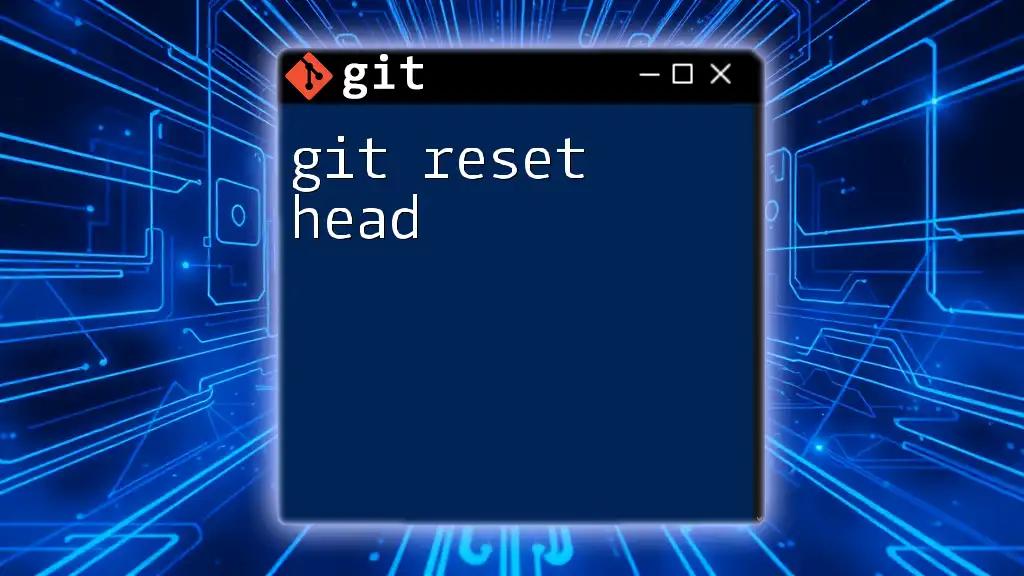
Common Issues and Troubleshooting
Even with the best intentions, configuration issues can arise. Here are some frequent hurdles users may encounter and how to address them:
- Credential Helper Not Working: If your credential helper seems ineffective, check your global configuration with:
git config --global --list
This command lists all global configurations. Ensure your credential.helper setting is present.
- Removing Stored Credentials: If you need to clear any stored credentials, use the following commands based on the helper you’ve set up:
For cache:
git credential-cache exit
For store:
Edit or remove the credentials directly from `~/.git-credentials`.
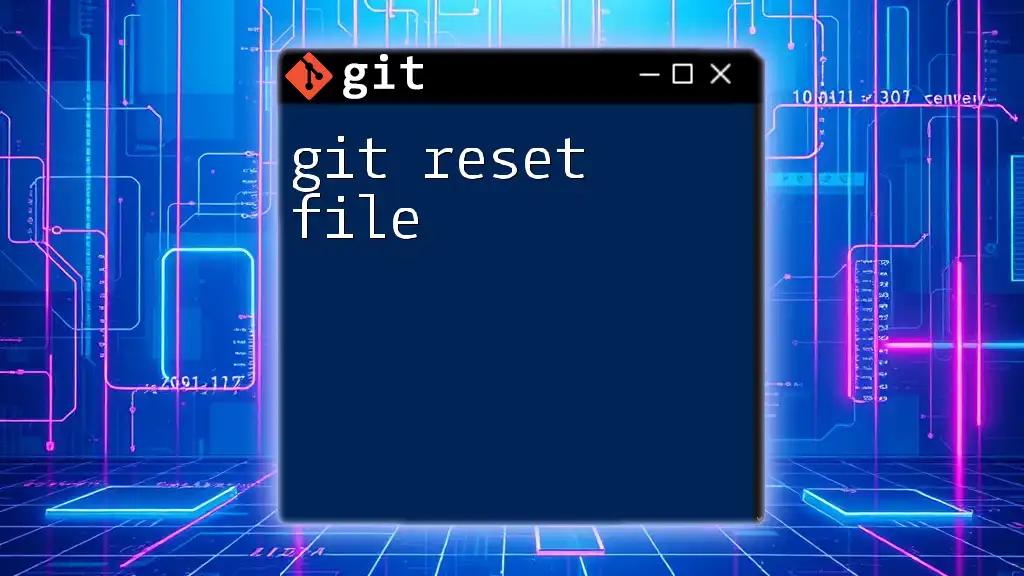
Best Practices for Managing Git Credentials
When managing Git credentials, it is essential to prioritize security. Here are some best practices:
-
Security Considerations: Always opt for encrypted storage whenever possible. Avoid using the store helper unless absolutely necessary.
-
Using Personal Access Tokens: Instead of regular passwords, opt for personal access tokens, especially when using services like GitHub or GitLab. Tokens provide a more secure alternative that can be easily revoked.
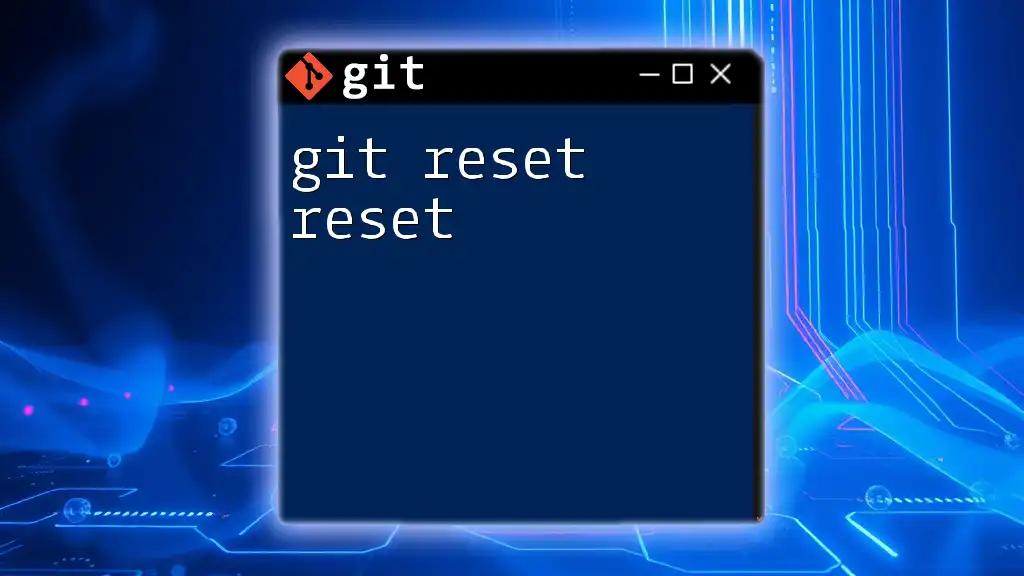
Conclusion
Utilizing a Git Credential Helper optimizes your workflow by managing authentication efficiently. By selecting the right helper based on your operating system and security needs, you can enhance your Git experience. Setting it up is easy, and the benefits far outweigh any potential downsides. Embrace the seamless convenience of Git Credential Helpers and focus on what really matters—coding!
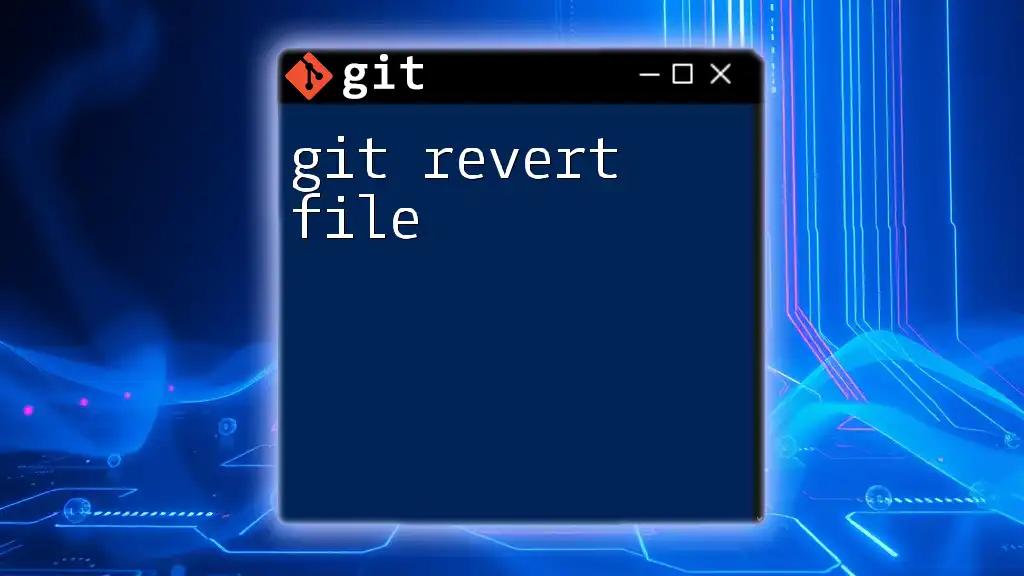
Additional Resources
For further learning and deeper insights, the [official Git documentation](https://git-scm.com/doc) serves as a valuable resource. There are also numerous tools and extensions available that can further enhance your Git workflow, so explore options that meet your specific needs.