Certainly! The difference between `git stash pop` and `git stash apply` is that while both restore stashed changes, `pop` also removes the stash entry from the stash list, whereas `apply` keeps the stash entry intact for future use.
# Pop the most recent stash and remove it from the stash list
git stash pop
# Apply the most recent stash without removing it
git stash apply
Understanding Git Stash
What is Git Stash?
Git stash is a powerful command in the Git version control system that allows you to temporarily save changes in your working directory that you aren’t ready to commit yet. Think of it as setting aside unfinished work until you are ready to come back to it. Common use cases include:
- Switching branches without committing incomplete changes.
- Collaborating with team members while wanting to pause your current work.
- Experimenting with new ideas without polluting the commit history.
Git stash can hold not only uncommitted changes to tracked files but also untracked files and changes to files ignored by Git.
How Git Stash Works
When you run `git stash`, it saves your current changes and provides a clean slate, allowing you to switch contexts. These changes are stored in a special stack called the stash stack, which you can think of as a series of layers that you can apply to your working directory later.
The command `git stash list` shows you all the stashes available, with each entry labeled sequentially, like `stash@{0}`, `stash@{1}`, etc.
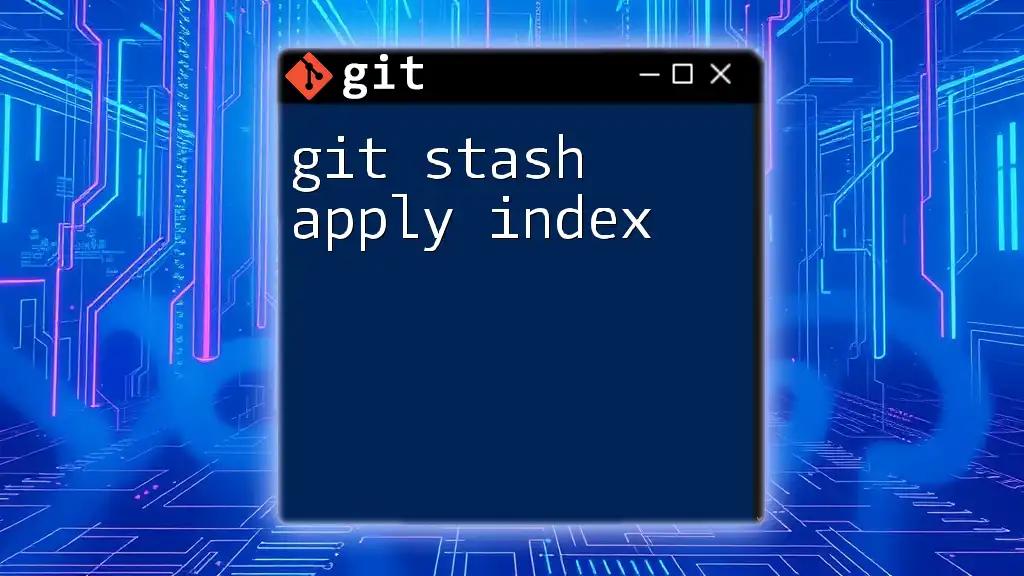
Git Stash Pop vs Git Stash Apply
Introducing Git Stash Pop and Apply
Both `git stash pop` and `git stash apply` are used to reapply changes that you have stashed away. However, their behavior differs significantly:
- `git stash pop`: This command retrieves the latest stash and removes it from the stash stack after applying it to the current working directory.
- `git stash apply`: This command reintroduces the changes from the stash into your working directory but keeps the stash in the stack for future use.
Key Differences Between Git Stash Pop and Apply
Behavioral Differences
The key difference lies in what happens after executing each command. When you use `pop`, the stash is applied and immediately deleted from the stash stack:
git stash pop
Conversely, when you use `apply`, you are merely applying the stash without removing it:
git stash apply stash@{0}
This means that if you use `apply`, the stash remains available for later use.
Use Case Scenarios
Choose `git stash pop` when you’re confident that you want to remove the changes from the stash after applying them. This is ideal when you are sure you won’t need those changes again.
Use `git stash apply` when you might want to reuse the stashed changes in the future. For instance, if you're still experimenting or are unsure whether the changes work, `apply` is the way to go.
Syntax and Examples
Using Git Stash Pop
The basic syntax for `git stash pop` is:
git stash pop [<stash>]
You can run this command without specifying a stash, in which case it will pop the most recent one—`stash@{0}`.
For example:
git stash pop
This command applies the changes stored in the most recent stash and removes that entry from the stash list.
To illustrate, if you stashed changes made to `file1.txt` and `file2.txt`, running this command reintroduces those changes and cleans up your stash.
Using Git Stash Apply
The basic syntax for `git stash apply` is similar:
git stash apply [<stash>]
For example:
git stash apply stash@{0}
This command applies the changes from the specified stash but does not remove it from the stash stack, allowing you to run it again later if needed.
If you only need a specific stash, you can specify it explicitly.
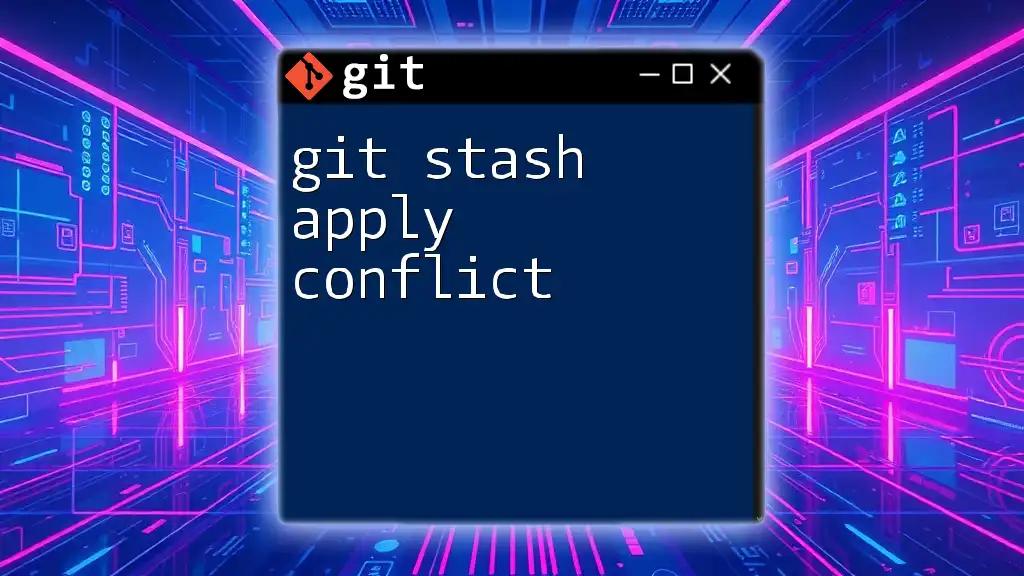
Conflict Resolution
Handling Conflicts with Pop and Apply
Both commands can lead to conflicts if the stashed changes overlap with changes made in the working directory after you stashed them. If conflicts occur, Git will notify you, detailing the conflicts in the terminal.
Using `pop` will generally stop execution at the first conflict encountered, while `apply` will continue applying changes until it hits an issue. Both methods require conflict resolution before moving forward.
Example of Resolving a Conflict
When a conflict arises after using either command, you may see error messages indicating which files are in conflict. You will need to manually resolve these conflicts by editing the affected files and then marking them as resolved with:
git add <conflicted-file>
git commit
Both commands can lead to the necessity for resolving conflicts, but the approach depends on whether you're using `pop` or `apply`.
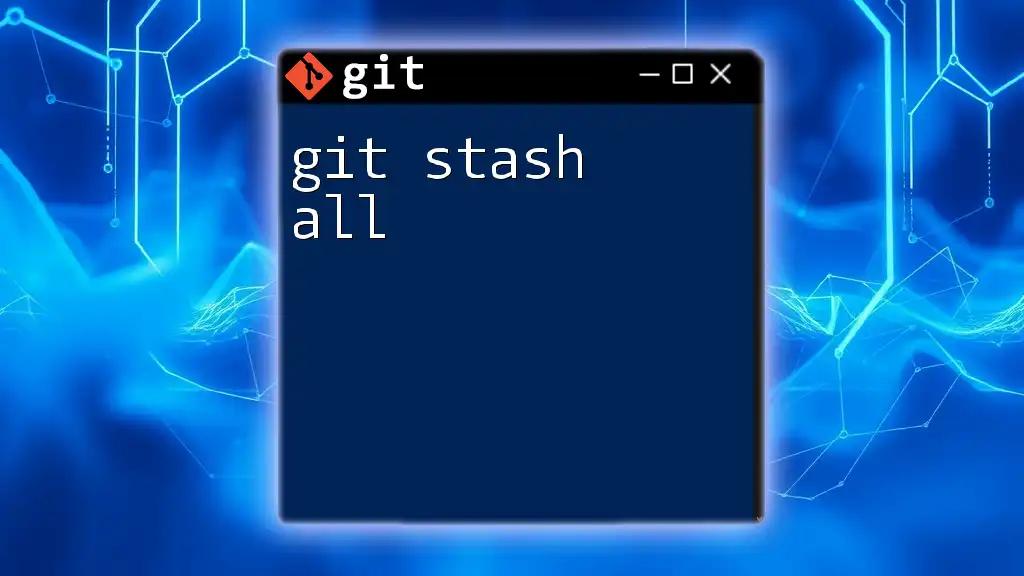
Practical Tips and Best Practices
When to Use Pop
Use `git stash pop` when you are sure that after applying the stash you have no further need of it. This is ideal for rapid iterations where you are confident that the stash either worked or you won’t need to revert back.
When to Use Apply
Leverage `git stash apply` when you think you may need to access the stash later or want to keep it for troubleshooting. This is especially useful in team environments or complex feature developments where multiple iterations may be required.
Performance Considerations
Managing stashes effectively is essential, particularly in projects with multiple collaborators. Keeping track of your stash using `git stash list` can help maintain an organized workflow. Always consider whether you need to pop or apply based on your current workflow and goals.
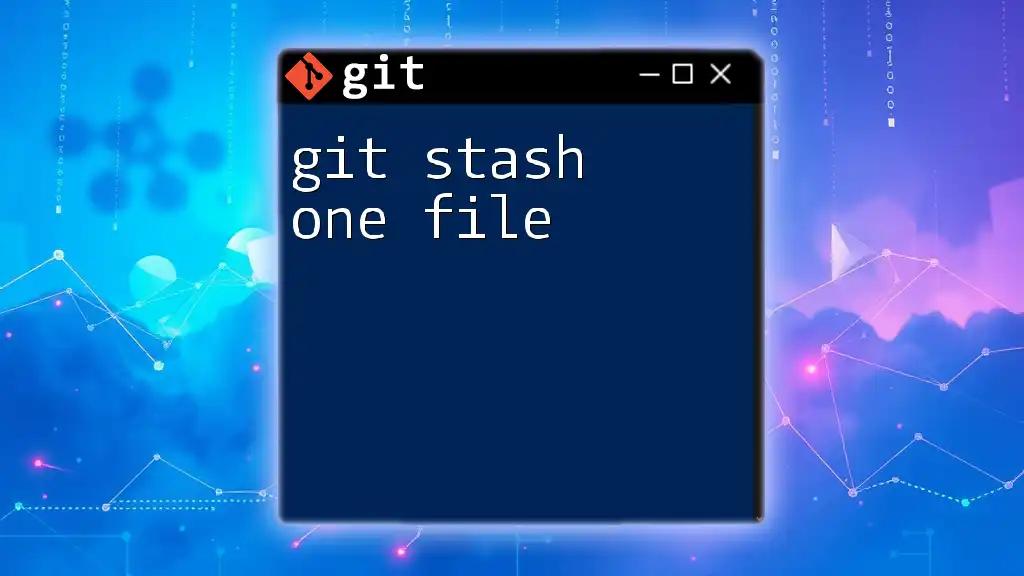
Conclusion
Understanding the differences between git stash pop and git stash apply can greatly enhance your version control workflow. Both commands serve valuable purposes in managing code changes efficiently. By mastering these tools, you will improve your ability to navigate complex project requirements while minimizing disruptions to your development process.
Further Reading and Resources
For a deeper understanding, it is highly recommended to refer back to the official Git documentation and explore further tutorials that will guide you in mastering advanced Git techniques. Happy stashing!