If `git fetch` is not working, it could be due to issues with your remote repository configuration or network connectivity problems.
git remote -v # Check the configured remote repositories
git fetch origin # Attempt to fetch from the remote named 'origin'
Understanding the Basics of Git Fetch
What is `git fetch`?
`git fetch` is a command that downloads updates from a remote repository into your local repository without affecting your current working directory or branch. This operation gathers the data about new commits, branches, and tags from the remote but does not merge any of these changes into your local branches. It’s a safe way to review changes before you integrate them into your own work.
One critical distinction to remember is that `git fetch` only downloads the changes, while `git pull` fetches and merges the changes. This makes `git fetch` essential for collaborative environments where frequent updates are made by multiple developers.
Common Use Cases for `git fetch`
Developers often use `git fetch` to keep their local branches updated with the remote repository. Some common scenarios include:
- Updating Local Branches: It helps you stay informed about what’s happening in the project, especially in fast-paced development environments.
- Synchronizing with Remote Repositories: Team members might push updates that you need to be aware of before starting your own work.
- Fetching Changes Without Merging: This allows you to review code and changes made by others before integrating them into your work, adding an element of caution to your workflow.
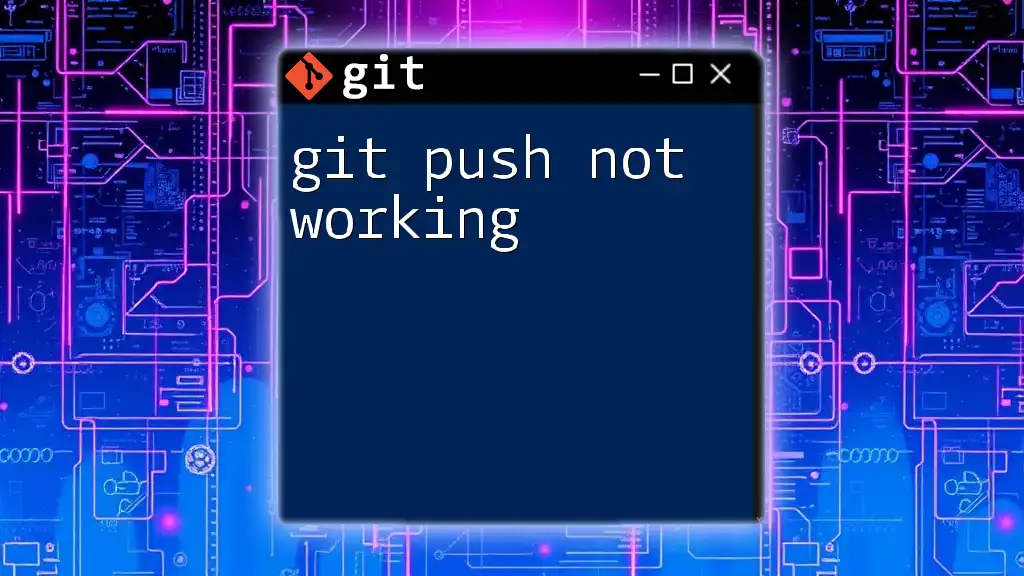
Troubleshooting `git fetch`
Common Error Messages
When running `git fetch`, you may encounter several error messages. Below are some common errors and their resolutions.
Could not resolve hostname
This error typically indicates that Git is unable to connect to the remote repository. This could happen for several reasons:
- Network issues: Ensure your internet connection is stable.
- DNS configuration: Check if your DNS settings are correctly configured to resolve the hostname of the remote repository.
You can resolve this issue by testing your connection with simple network commands. For instance, you can use `ping` to check the hostname:
ping <repository-host>
fatal: 'origin' does not appear to be a git repository
This error suggests that Git cannot find the specified remote repository named 'origin'. To rectify this:
- Confirm that you have configured your remotes correctly:
git remote -v
- If there’s an issue, you can update the remote URL using:
git remote set-url origin <new-url>
Diagnosing Connection Issues
Checking Internet Connectivity
If you suspect your connection is the issue, you can perform basic connectivity tests. Check if you can access the remote repository using `curl`:
curl -I <repository-url>
If the command returns an error, it hints at a potential Internet or repository server issue.
SSH vs. HTTPS Issues
Understanding whether you are using SSH or HTTPS to connect to your repository can help resolve connection difficulties. If you face persistent issues with one method, switching to another may help.
- SSH Connections: Ensure your SSH key is configured correctly in your SSH agent and added to your Git hosting service (e.g., GitHub).
- HTTPS Connections: Make sure to double-check your username and password, and verify any required tokens or credentials.
To set up SSH keys properly, follow these commands to generate and add a new key:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
eval "$(ssh-agent -s)"
ssh-add ~/.ssh/id_rsa
Remote Repositories & Configuration
Checking Remote Repository Configuration
To troubleshoot issues with remote configurations effectively, you must first check the current remote settings:
git remote -v
This command lists all remote URLs. If 'origin' appears incorrect, you must set or update it accordingly.
Permissions and Access Rights
Sometimes, permission issues can also prevent `git fetch` from working. You could encounter 403 Forbidden or 401 Unauthorized errors. To resolve permission issues:
- Ensure you have appropriate access rights for the remote repository.
- Collaborate with your repository owner to confirm your permissions.
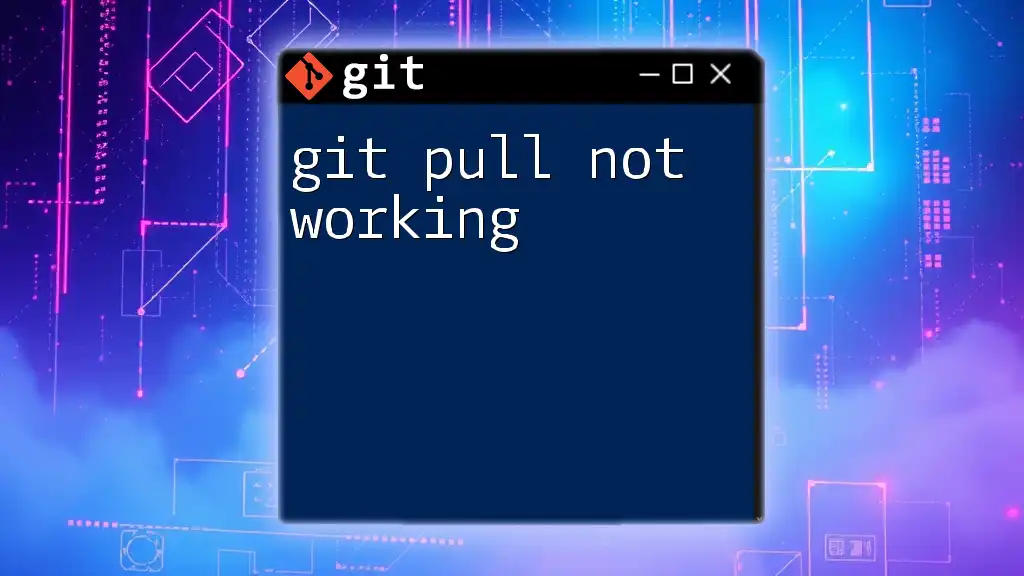
Advanced Troubleshooting
Understanding Git's Internal Mechanics
What Happens During `git fetch`
When you execute `git fetch`, several internal processes kick in. Git communicates with the remote server, retrieves data about commits, branches, and tags that exist remotely but not locally, and stores them in your local repository without altering your current branches. This operation is fundamental for maintaining a clean working environment while keeping up with non-disruptive updates.
Using Verbose Mode for Debugging
If you’re struggling to understand why `git fetch` is not working, using the verbose mode can provide critical insights. Running the command with verbose output will show detailed information on what Git is attempting to do:
git fetch --verbose
Interpreting this output can give clues about connectivity issues, authentication failures, and configuration problems.
Connectivity and Firewall Issues
Sometimes, local firewalls or proxy settings might block Git's access to the remote repositories. If you're on a corporate or secured network, check to ensure Git is allowed through the firewall, or consider using a Virtual Private Network (VPN) to bypass these restrictions.
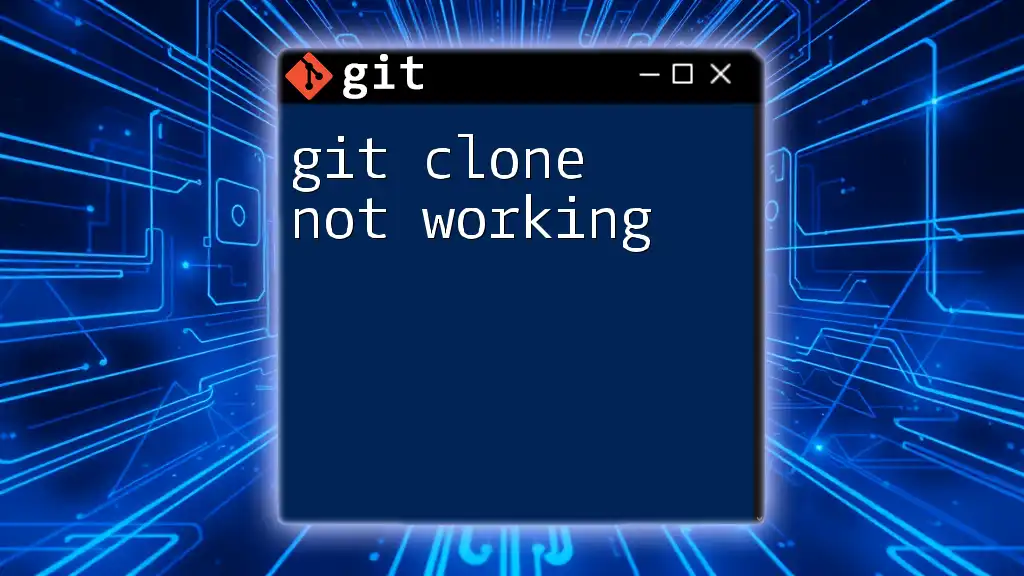
Best Practices for Using `git fetch`
Regularly Fetching Changes
Getting into the habit of regularly fetching changes keeps you informed and minimizes surprises when you start development. It’s recommended to fetch changes at the start of your working session. A simple command will suffice:
git fetch origin
Implementing Branch Tracking
By setting up branch tracking, you can simplify the fetching process. When you create a new branch that tracks a remote branch, Git will know from where to fetch changes:
git branch --track <branch-name> origin/<branch-name>
Review and Merge Strategies Post-Fetch
After Fetching: What Next?
After fetching, it’s vital to review the changes. Use `git log` to view commits fetched from the remote:
git log origin/<branch-name> --oneline
Decide on the appropriate strategy to integrate the changes. Depending on your project and team practices, you might prefer merging or rebasing.
Keeping Your Local Repository Clean
Over time, unused remote branches accumulate in your repository. Keeping your environment clean is essential, and you can do this by periodically running cleanup commands:
git remote prune origin
This command deletes references to remote branches that no longer exist.
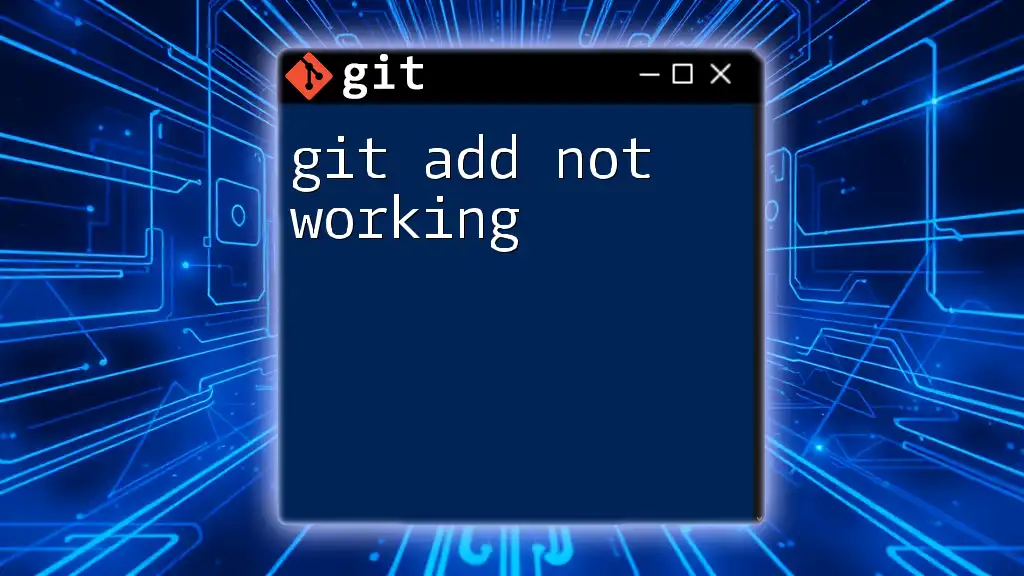
Conclusion
Recap of Key Points
In conclusion, mastering the `git fetch` command is crucial for collaborating efficiently in teams. Understanding its function, recognizing common errors, and employing best practices will set you up for success in your Git workflows.
Encouragement to Experiment
Don’t hesitate to explore various Git commands and functionalities beyond `git fetch`. The more you experiment, the more proficient you will become, equipping yourself with the knowledge needed to tackle real-world projects effectively. As you grow your skills, don’t forget to leverage additional resources and tutorials available online to deepen your Git knowledge.