If your `git commit` is not working, it may be due to untracked files, unstaged changes, or a missing commit message; ensure everything is staged and provide a message with the command shown below:
git add . && git commit -m "Your commit message here"
Understanding `git commit`
What is `git commit`?
The `git commit` command plays a crucial role in version control. It captures the state of the project at a specific moment, allowing developers to create a historical record of changes. Each commit includes a unique identifier and a commit message that describes the changes made. This record is essential for collaboration and tracking progress in your project.
Basic Syntax of `git commit`
The basic syntax for using the `git commit` command is straightforward:
git commit -m "Your commit message here"
The `-m` flag allows you to provide a short commit message in-line without launching an editor, streamlining your workflow and ensuring that you can document your changes swiftly.
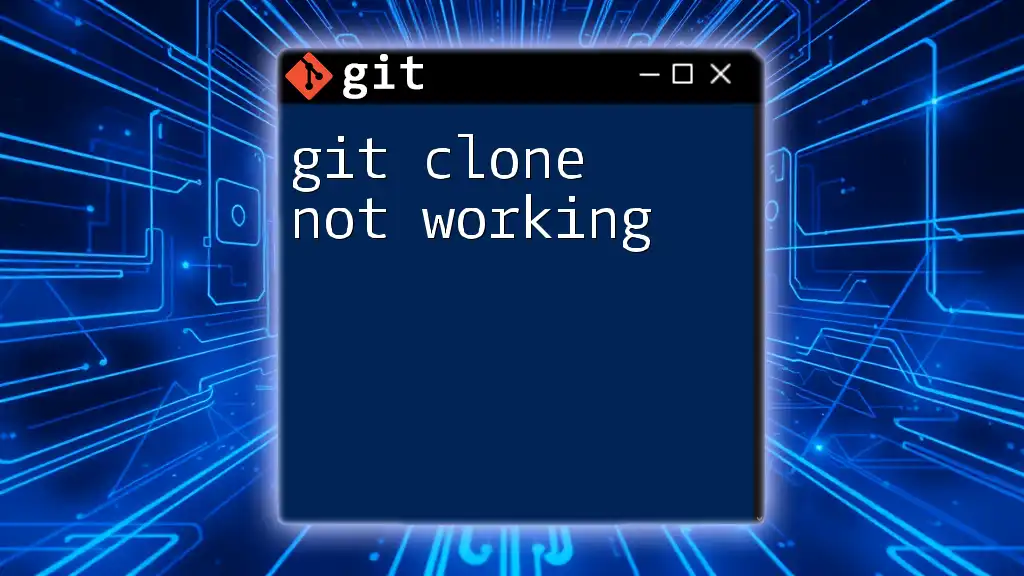
Common Reasons Why `git commit` Might Not Work
No Changes to Commit
One of the most common reasons for the error “nothing to commit, working tree clean” arises when there are no changes staged for commit. Before executing `git commit`, ensure that you have made changes to your files. Use the following command to check the status of your repository:
git status
If you see output indicating no changes, you need to modify files and stage them using the `git add` command:
git add filename.txt
Once your changes are staged, you can successfully commit them.
Changes Not Staged
In Git, modifications need to be staged before they can be committed. If you forgot to stage your changes, the `git commit` command will not find anything to commit. Again, use `git status` to verify which files have been modified but not staged.
After confirming your changes, stage the changes:
git add filename.txt
Then, proceed with your commit:
git commit -m "Add updates to filename"
This ensures that your changes are captured in the commit history.
Untracked Files
Another issue can arise when untracked files exist in your working directory. Untracked files are those that have not been staged for commit. Check these files by using:
git status
If you see untracked files listed, you can track them with:
git add untracked_filename.txt
Following this, you can commit your changes normally, as these files will now be included.
Merge Conflicts
Merge conflicts can prevent you from committing changes if you're working on a branch and attempting to merge it with another branch. When conflicts arise, Git will indicate this with an error message.
To resolve merge conflicts, use the `git status` command to identify which files are in conflict and require resolution. You can fix conflicts manually in the affected files, highlight the differences, and follow with:
git add filename_with_conflicts.txt
Following this step, complete the merge with:
git commit -m "Resolve merge conflicts"
This ensures that all changes from both sources are appropriately consolidated.
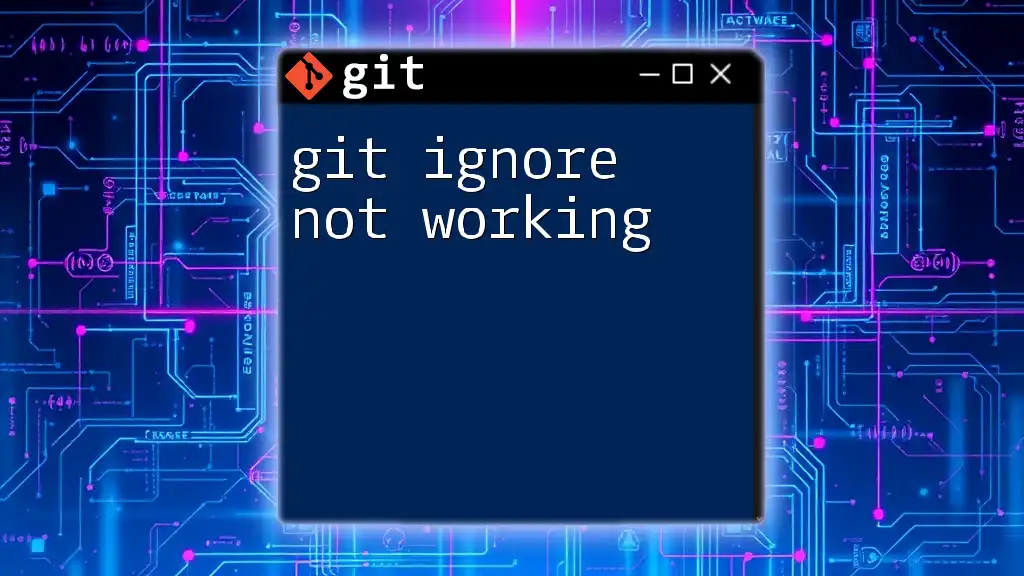
Error Messages and Troubleshooting
Common Error Messages
Error: “nothing to commit, working tree clean”
This message means there are no changes staged. If you’re seeing this, double-check your modifications, stage them, and try committing again.
Error: “You have unmerged paths”
This error indicates that there are unresolved merge conflicts. You’ll need to resolve these conflicts before you can make any commits.
Error: “Your branch is ahead of ...”
This message suggests that your branch has commits that are not present in the remote branch. Consider pushing your changes or using `git pull` to synchronize your branches before committing any new changes.
Solutions to Common Errors
How to Force Commit
If you realize you’ve made a mistake in your last commit and want to amend it, you can use the `--amend` flag:
git commit --amend -m "Updated commit message"
Note: Be cautious when using this command, particularly in shared branches, as it rewrites commit history.
Permissions Issues
Sometimes `git commit not working` can be traced back to file permission issues. If you're getting errors related to permissions, you can check the permissions using:
ls -l filename.txt
If necessary, adjust the permissions to ensure that your user has write access:
chmod +w filename.txt
This command will grant write permissions for the specified file, allowing you to proceed with your commit.
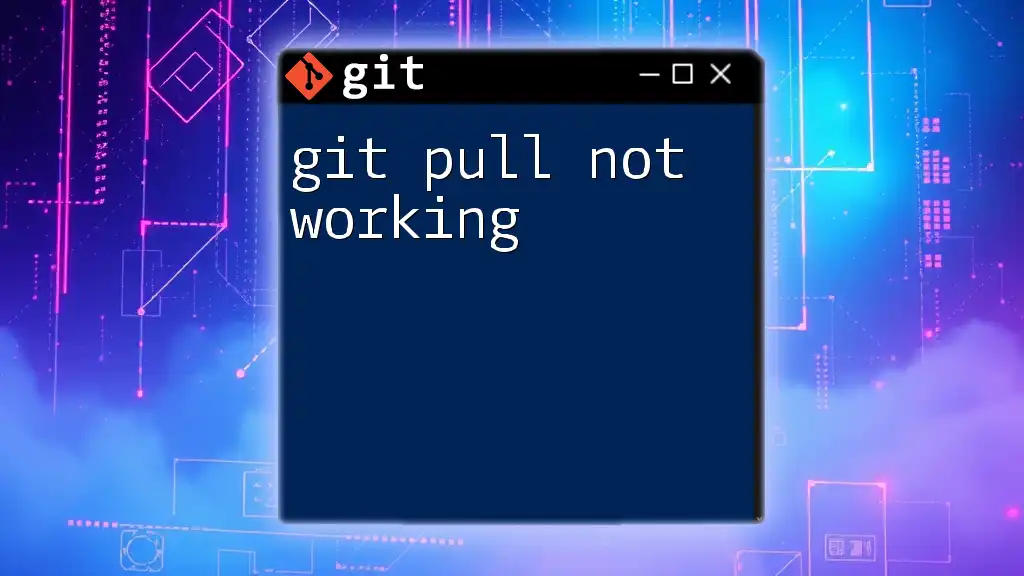
Best Practices for Using `git commit`
Writing Good Commit Messages
Writing clear and informative commit messages is essential for effective project management. A good commit message should convey the what, why, and how of the changes made. For instance, a weak commit message like “Updated file” lacks context. In contrast, a strong message like “Fix issue with filename handling in the parser” provides clear insight into what the commit entails.
Frequent Commits Over Large Ones
Regularly committing smaller chunks of work rather than one large commit has distinct advantages. It keeps your commit history clean, helps in isolating bugs, and facilitates easier collaboration among team members. Aim to commit often, particularly after completing a specific feature, fixing a bug, or implementing a change.
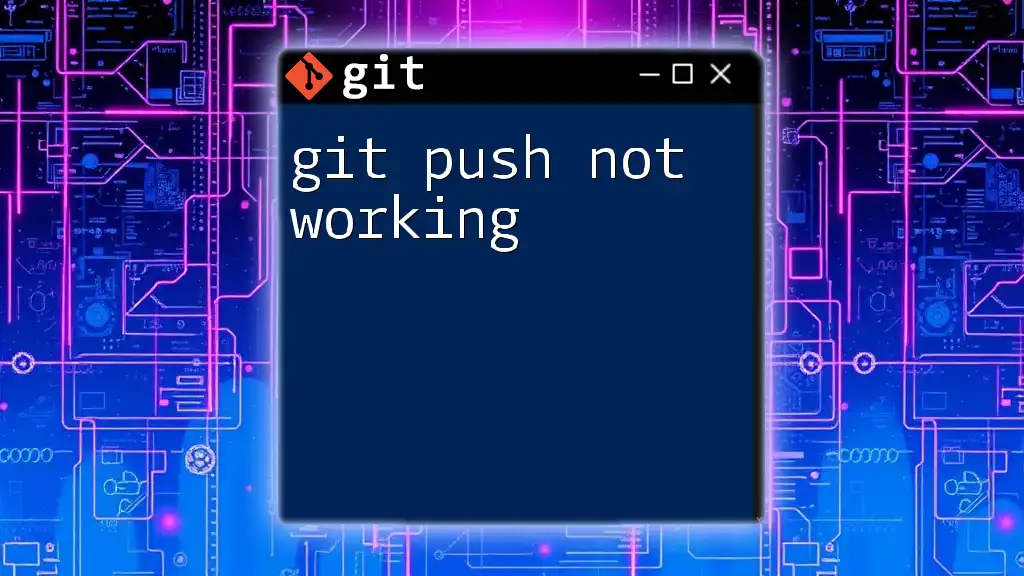
Conclusion
Understanding the reasons why `git commit not working` is essential for effective version control. By familiarizing yourself with common issues and their solutions, you will enhance your productivity and collaboration with others on projects. Embrace the power of the `git commit` process and share your thoughts or inquire about further assistance. Your Git journey just got more manageable!
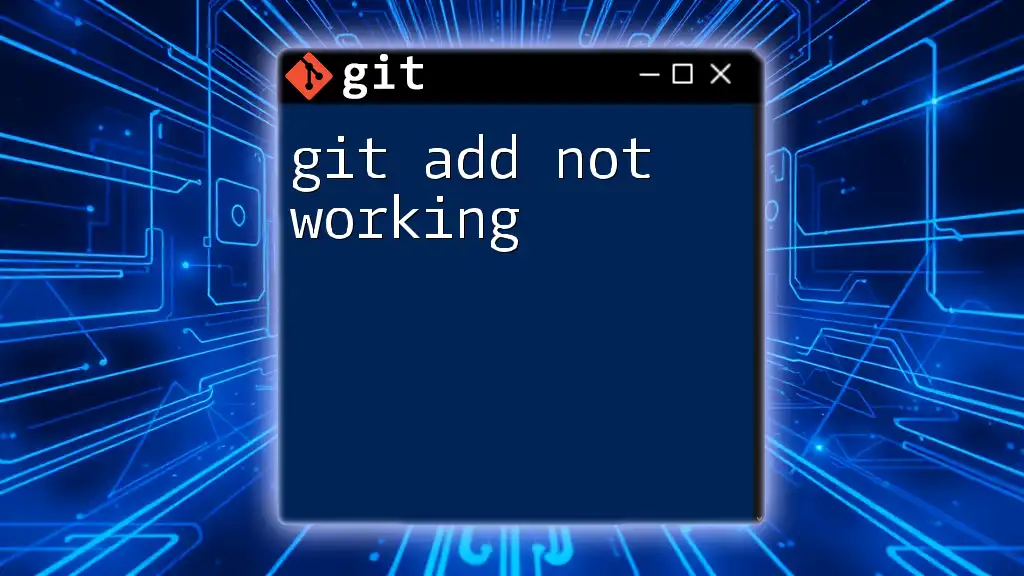
Additional Resources
For further learning, consider exploring the official Git documentation and look for online tutorials and courses that delve deeper into Git commands. Additionally, check out our upcoming workshops where you can improve your command line skills and become a Git expert!