If your `.gitignore` file is not working, it may be due to incorrect syntax, misplaced files, or already tracked files in your repository; ensure the patterns are correctly defined and that you untrack any previously added files.
Here's an example of how to untrack a file:
git rm --cached filename.txt
Understanding .gitignore
What is .gitignore?
The `.gitignore` file is a fundamental component in Git that specifies intentionally untracked files to ignore. This is crucial for keeping your Git repository clean and efficient by excluding files that do not need to be versioned, such as build artifacts, logs, or configuration files.
Using a `.gitignore` file allows you to prevent clutter in your working directory, ensuring that only relevant files are committed to the repository. For instance, it’s generally a good practice to include files like:
- Temporary files (e.g., `.tmp`, `.log`)
- Build directories (e.g., `node_modules/`, `dist/`)
- Sensitive information (e.g., `.env` files)
Structure of .gitignore
Creating effective rules in your `.gitignore` file requires understanding its structure. Each line in the file represents a pattern that Git will check against files in your repository.
Basic syntax rules:
- Empty lines or lines starting with `#` are ignored.
- Each pattern is relative to the directory the `.gitignore` file is in unless it starts with a `/`.
- A `*` wildcard represents any number of characters, while `?` matches a single character.
- `**` matches directories recursively.
Example entries:
# Ignore all .log files
*.log
# Ignore node_modules directory
/node_modules/
# Ignore all .env files but not .env.example
*.env
!.env.example
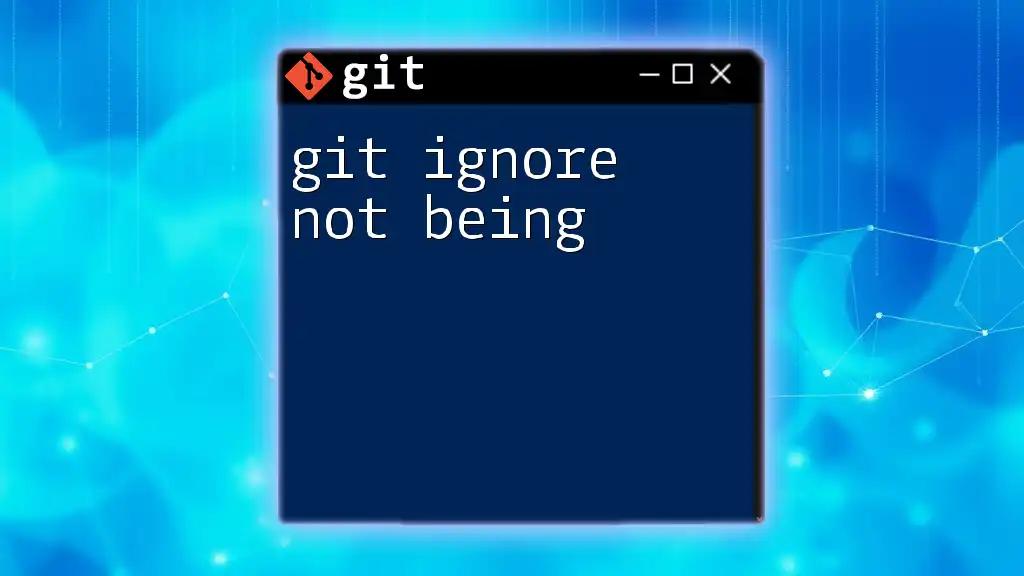
Common Reasons Why Git Ignore Might Not Work
Files Already Tracked by Git
A common mistake when facing the issue of “git ignore not working” is forgetting that Git only ignores files that aren't currently tracked. If a file was added to the repository before it was listed in `.gitignore`, Git will continue to track changes to that file.
Example: To check whether a file is being tracked, use:
git ls-files --others --exclude-standard
To stop tracking a file that should be ignored, use:
git rm --cached <file>
This command removes the file from the index (staging area) but leaves it in your working directory unchanged.
Incorrect .gitignore Syntax
A common pitfall is incorrect syntax in your `.gitignore` file. If the rules are wrongly formatted, Git won't understand which files to ignore.
Code Snippet: Correct and incorrect `.gitignore` entries:
# Correct entry
*.log # Ignores all .log files
# Incorrect entry (missing wildcard)
log # Ignores "log" but not "file.log"
Location of the .gitignore File
The location of your `.gitignore` file is critical. It should be placed in the root of your repository for the rules to apply globally. You can, however, create `.gitignore` files in subdirectories for specific rules relevant only to those directories.
Global .gitignore Settings
Git allows you to set up a global `.gitignore` that applies to all repositories. This is particularly useful for ignoring files that are common across your projects.
To configure a global `.gitignore`, use:
git config --global core.excludesfile ~/.gitignore_global
Then, add patterns to the `~/.gitignore_global` file.
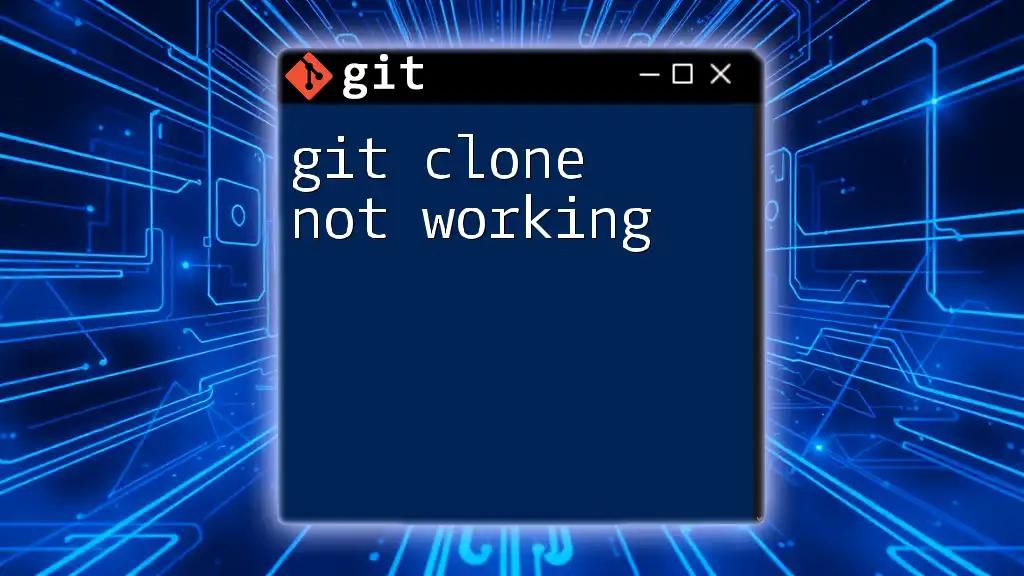
Debugging .gitignore Issues
Using Git Check-Ignore
If you still encounter issues with “git ignore not working,” Git provides the `git check-ignore` command, which helps troubleshoot and test ignore patterns directly.
Command:
git check-ignore -v <file>
This command will show which line in which `.gitignore` is affecting the file.
Verifying Ignored Files
Another effective way to check which files Git is ignoring is by using:
git status
Files listed in untracked files will not be ignored, and you can cross-reference these with your `.gitignore` to confirm why they aren’t being ignored.
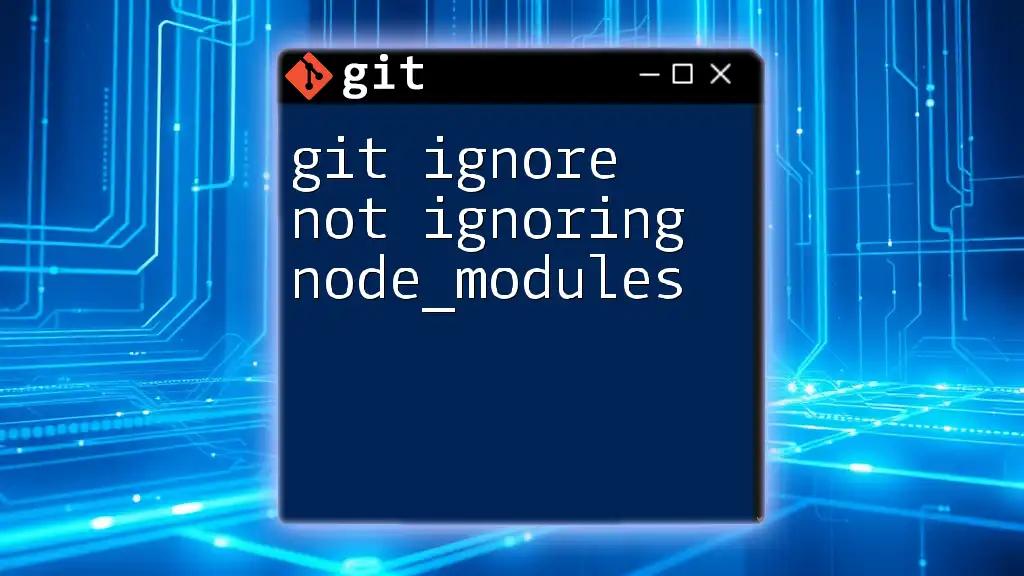
Advanced .gitignore Usage
Nested .gitignore Files
In complex projects, you might find yourself needing nested `.gitignore` files to manage specific directories. Git applies the rules in each `.gitignore` file in relative paths.
Code Snippet: In the root directory:
# Root .gitignore
*.log
In a folder called `build` inside the root:
# build/.gitignore
*.tmp
!important.tmp # Do not ignore important.tmp
.gitignore for Specific Environments
Managing different stages of your project—such as development versus production—can necessitate having environment-specific `.gitignore` files.
Example: In development, you might want to ignore all compiled files:
# .gitignore for development
*.o
*.so
While in production, you might keep these files trackable:
# .gitignore for production
# No specific ignores
Common Patterns for Popular Languages
Utilizing common patterns tailored to specific languages or frameworks can save time and ensure comprehensive coverage in your `.gitignore`. For instance, for a Node.js project, you can use:
Example:
# Node.js .gitignore
node_modules/
npm-debug.log
.env
For additional curated `.gitignore` examples, repositories like [GitHub's gitignore repository](https://github.com/github/gitignore) provide a vast collection.
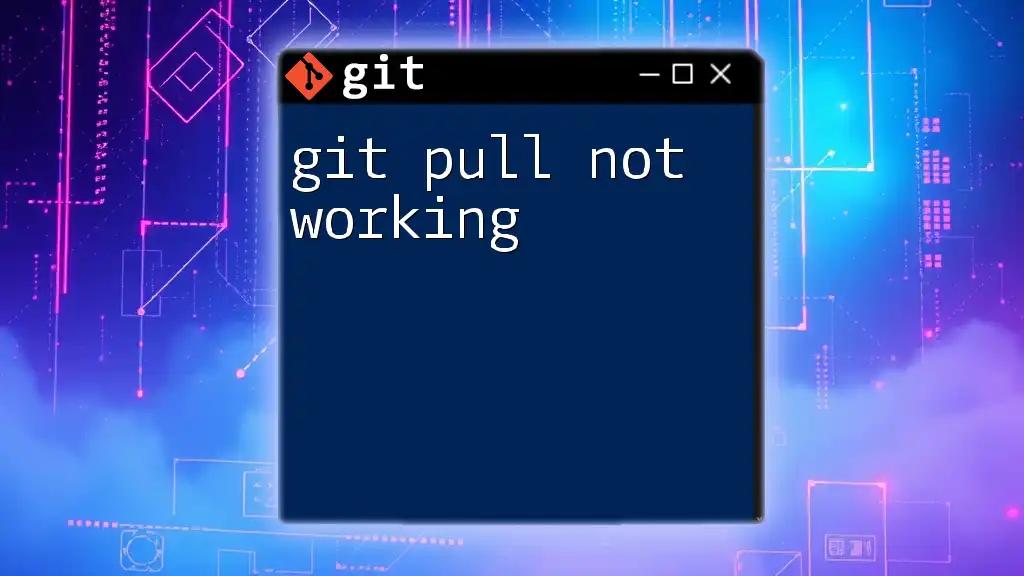
Conclusion
Understanding why “git ignore not working” can be essential for maintaining a clean repository. By recognizing how Git tracks files, learning to write correct patterns, and using global settings, you ensure that your `.gitignore` file is effective. Regular verification and adjustment of your rules based on your project’s changes will keep your Git experience efficient and manageable.
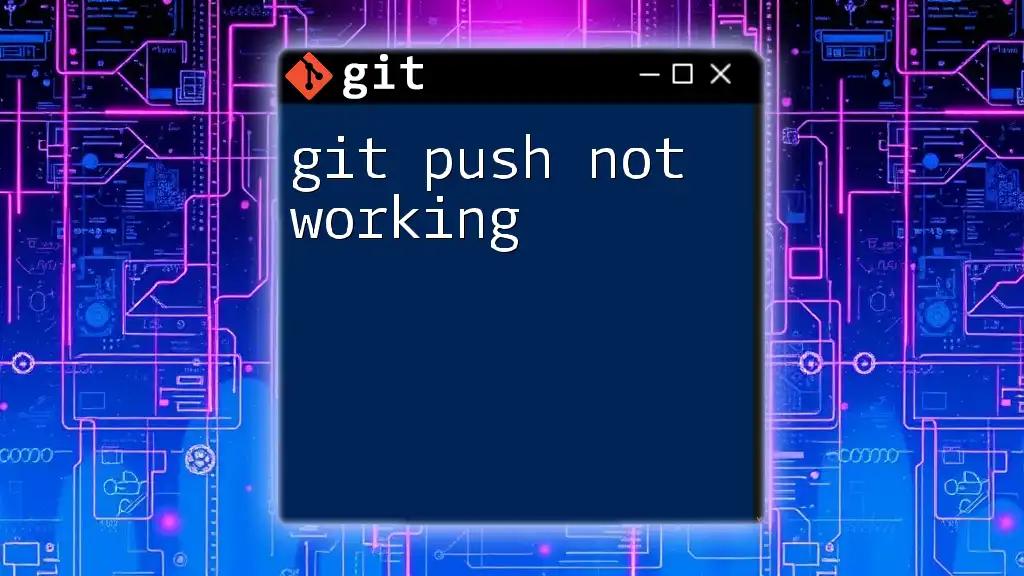
Call to Action
Have you faced challenges with your `.gitignore`? Share your experiences in the comments below! Stay tuned for more tips on mastering Git commands.
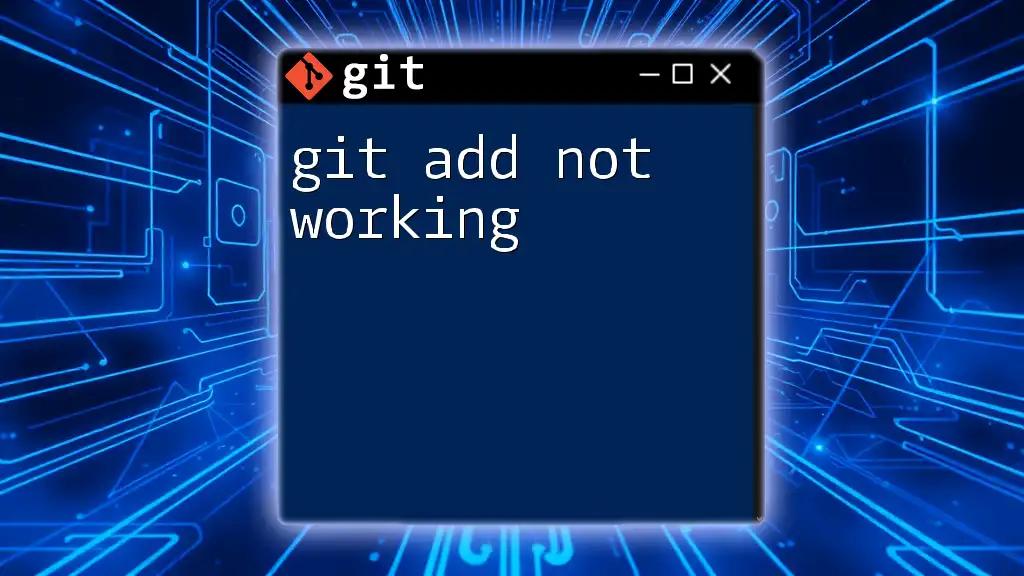
Additional Resources
For further reading, explore the [official Git documentation](https://git-scm.com/doc) on `.gitignore` and check out tools designed to assist in managing `.gitignore` files effectively.