To ignore Node.js specific files and directories in your Git repository, create a `.gitignore` file and add the following line:
node_modules/
Understanding .gitignore in Git
Importance of .gitignore
The `.gitignore` file is a vital component in any Git-based project. It instructs Git which files and directories to ignore, helping to keep your repository clean and focused. Including a proper `.gitignore` file is essential for maintaining a seamless workflow, especially in collaborative environments. By excluding unnecessary files, you can avoid cluttering your version control history and prevent sensitive information from being committed.
Basic Syntax of .gitignore
The syntax of a `.gitignore` file is straightforward yet powerful. Each line in the file represents a pattern that Git will use to determine which files to ignore. Here are some key components:
- Wildcards: Use `` to represent zero or more characters. For example, `.log` will ignore all log files.
- Slashes: A leading slash `/` indicates the root directory. For instance, `/build` targets the build folder at the root level.
- Negation: Use `!` to negate a pattern. This allows you to specify exceptions, such as keeping an important file even when other similar files are ignored.
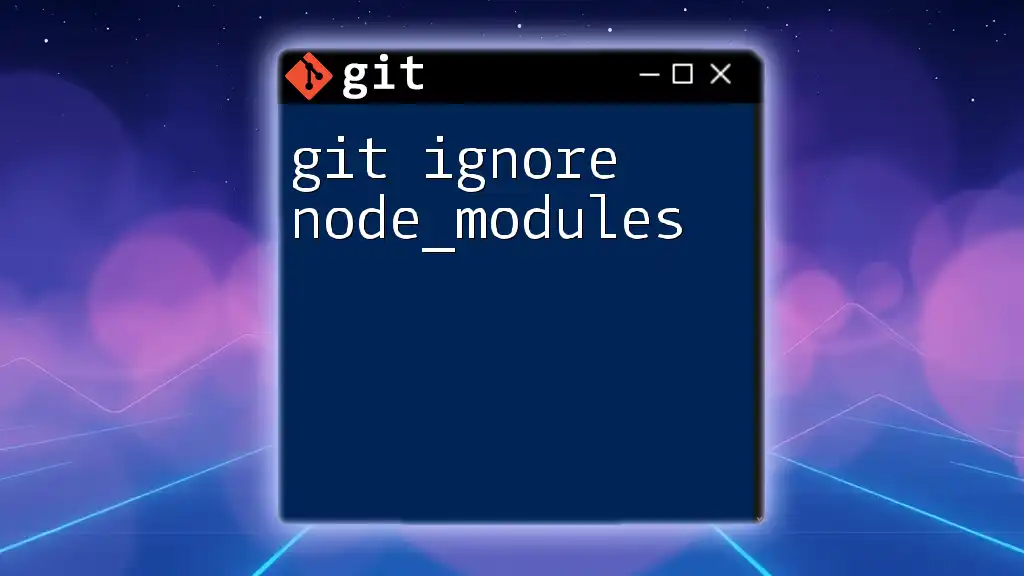
Setting Up a .gitignore File for Node.js
Creating a .gitignore File
To set up a `.gitignore` file for your Node.js project, you simply need to create a text file named `.gitignore` in the root of your project directory. You can execute the following command in your terminal:
touch .gitignore
Recommended Entries for Node.js Projects
When it comes to Node.js, certain files and directories should be ignored to keep your repository clean. Here are some common entries you should include in your `.gitignore` file.
Common Directories to Ignore
-
`node_modules/`
The `node_modules/` directory is where npm installs packages, and it can grow very large. Since everyone on your team can install their own dependencies, there's no need to include this directory in Git. You would add this line to your `.gitignore` file:node_modules/
-
Logs and Environmental Files
Log files can quickly clutter your repository and contain runtime information that shouldn't be tracked. Common files to ignore include:npm-debug.log* .env
-
Development Artifacts
Other directories that might contain build artifacts include `dist/`, `.build/`, and similar. Including these lines in your `.gitignore` helps ensure that only relevant source files are managed by version control:.build/ dist/
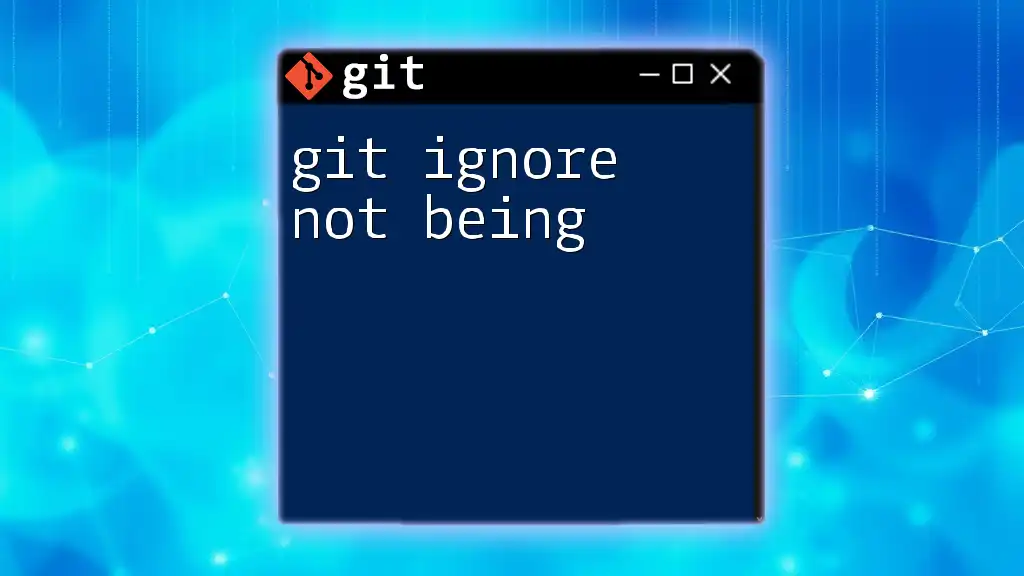
Advanced .gitignore Techniques
Using Wildcards and Patterns
Understanding how to use wildcards effectively can significantly enhance your `.gitignore` configurations. For instance, if you want to ignore all log files, regardless of their names, you can use:
*.log
This command will exclude any files ending with `.log`, such as `error.log` or `access.log`.
Examples of Patterns
If you want to ignore all test files ending with `.test.js`, you can add:
*.test.js
Negating Patterns
Sometimes you might want to ignore a broad set of files while keeping a specific one. For example, if you want to ignore all log files except `important.log`, you would do the following:
*.log
!important.log
This makes it easy to manage files that are crucial for your project's operations while keeping the rest clean.
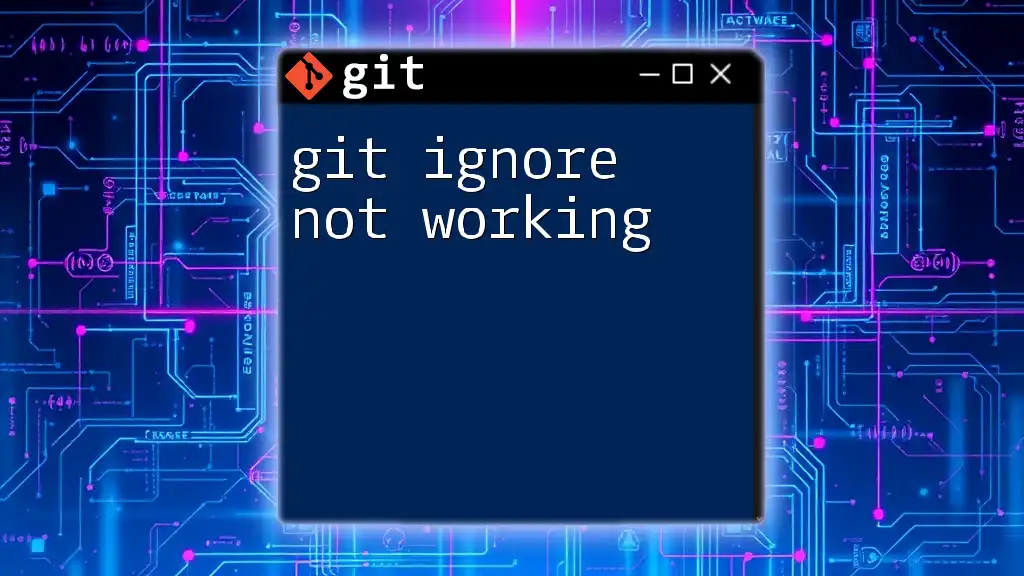
Testing Your .gitignore Configuration
Verifying Ignored Files
After configuring your `.gitignore`, it's essential to confirm which files are being ignored. You can do this by running:
git check-ignore -v *
This command will show you which files are ignored based on your current configuration, ensuring you're only tracking what you intend to.
Common Pitfalls and Fixes
Ignoring files in Git can sometimes be tricky. A common pitfall occurs when developers add files to `.gitignore` after they’ve already been tracked. In this case, you must untrack those files with:
git rm --cached <file>
Remember that the above command only removes the file from tracking; it will still exist in your working directory.
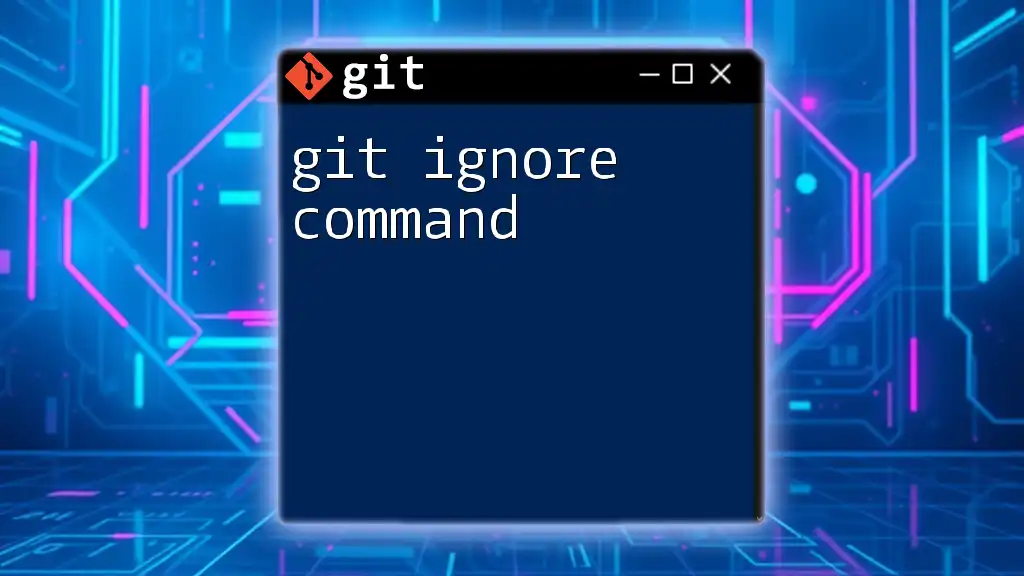
Best Practices for Managing .gitignore in Node.js Projects
Keep it Simple
It’s best to keep your `.gitignore` file simple and straightforward. Avoid overcomplicating it with unnecessary entries. Only ignore files and folders that are genuinely not needed for version control.
Versioning Your .gitignore
Your `.gitignore` file itself should be versioned and treated as any other important configuration file. Whenever you make updates or changes to this file, make sure to commit those changes:
git add .gitignore
git commit -m "Update .gitignore for Node.js"
Doing so ensures that your team is always aligned with what should be ignored, minimizing confusion and discrepancies.
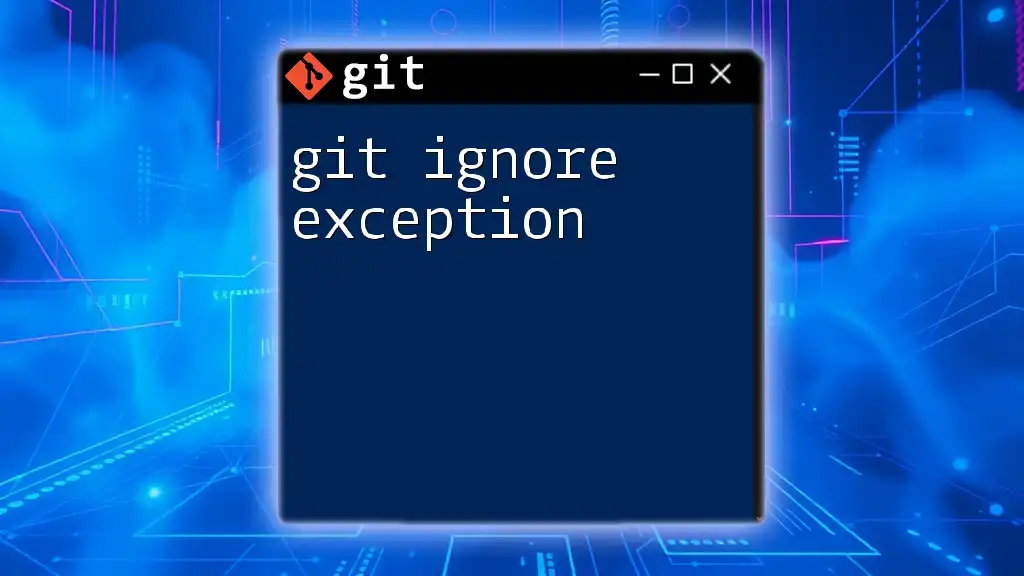
Conclusion
A well-configured `.gitignore` file is essential for any Node.js project. By following best practices and utilizing the proper entries, you can maintain a clean and efficient repository. Always remember to keep your `.gitignore` file updated and verify its performance regularly to ensure smooth collaboration. Embrace the power of `.gitignore` in managing your Node.js projects and enhancing your Git workflow.
Resources for Further Learning
For more comprehensive information regarding `.gitignore`, consider reviewing the official [Git documentation](https://git-scm.com/docs/gitignore). Additionally, keep learning about Node.js project structures to refine your understanding of best practices in using Git effectively.
Call to Action
Now that you have the tools to manage your `.gitignore` for Node.js projects efficiently, we encourage you to implement these strategies. Share your experiences or any questions you may have in the comments below. Happy coding!