To prevent your Python virtual environment directory (typically named `venv`) from being tracked by Git, you can add it to your `.gitignore` file using the following command:
echo "venv/" >> .gitignore
What is a .gitignore File?
A .gitignore file is a simple text file placed in your Git repository that tells Git which files—or groups of files—it should ignore. This is especially useful for preventing unnecessary clutter in your version control history. By ignoring certain files and directories, you keep your repository cleaner, more manageable, and focused on the files that truly matter for your project's development.
Common Use Cases
Commonly ignored files include:
- Operating system files: macOS tends to generate `.DS_Store` files, while Windows might create `Thumbs.db`.
- Compiled code: Files generated during the build process, like `.class` files for Java or `.o` files for C/C++.
- Dependencies and libraries: Such as the `node_modules` directory in JavaScript and the `venv` folder in Python projects.
Ignoring these files prevents them from cluttering your Git logs and reduces the overall size of the repository.
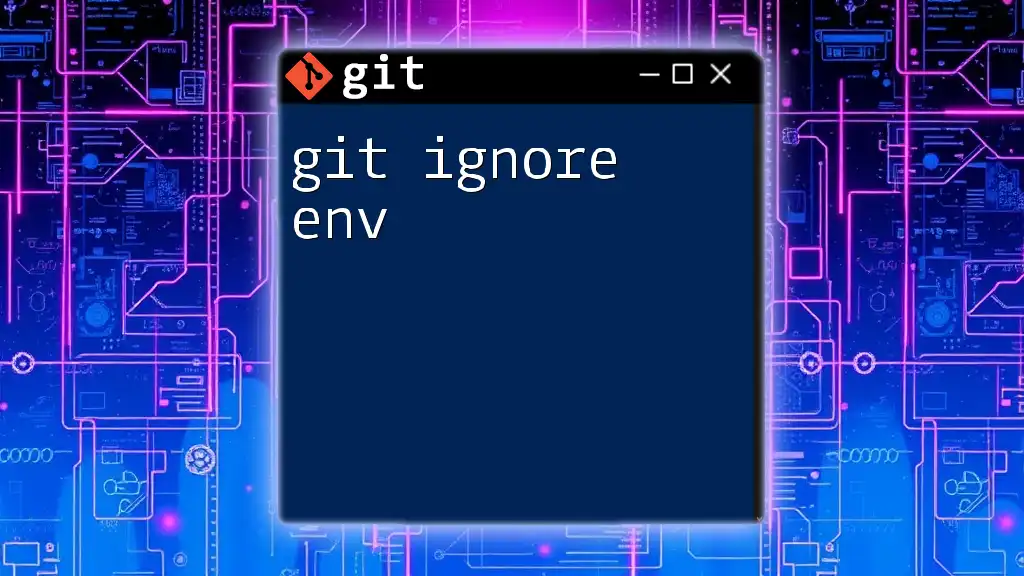
Understanding Virtual Environments (venv)
What are Virtual Environments?
Virtual environments in Python are isolated environments that allow developers to manage dependencies for different projects separately. This ensures that each project can have its own dependencies, regardless of what dependencies every other project has.
Creating a Virtual Environment
Creating a virtual environment is straightforward. You can do this using the following command:
python3 -m venv venv
This command creates a new directory named `venv` in your project folder. Inside `venv`, you’ll find directories for Python binaries and a place to install packages, effectively isolating these from your global Python environment.
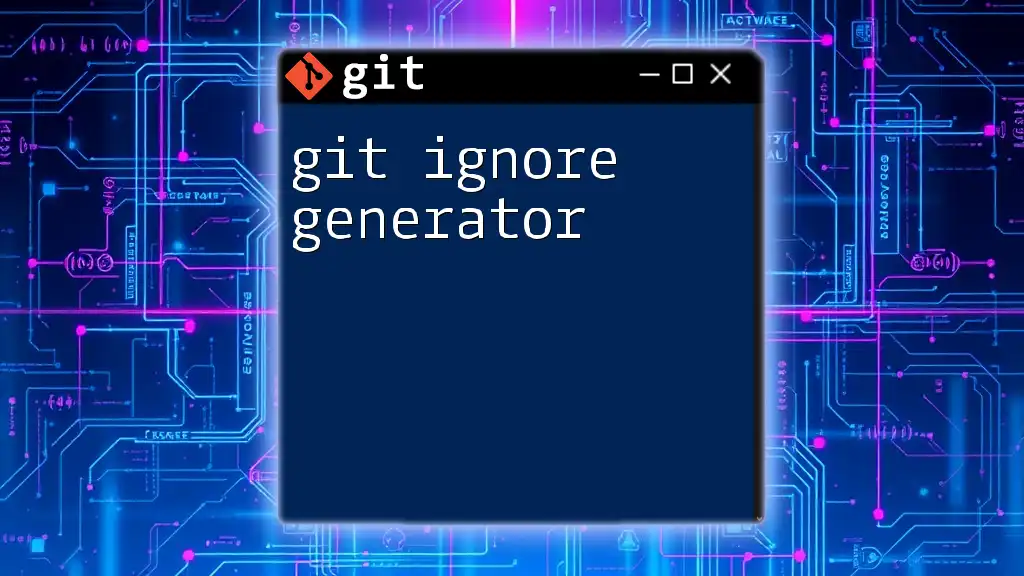
Why Ignore venv in Git Repositories?
Reducing Clutter in Your Repo
Including the `venv` directory in your Git repository can significantly bloat your project files. The `venv` directory can contain hundreds of files and take up a large amount of disk space. By ignoring it, your repository stays cleaner and more focused on the version-controlled files that are essential for collaboration and deployment.
Avoiding Dependency Conflicts
When you track the virtual environment files, you risk introducing dependency conflicts. Each developer might have different packages or versions installed in their `venv`, leading to discrepancies when the code is run on different machines. Instead of tracking these files, rely on a file like `requirements.txt` to manage your project's dependencies effectively.
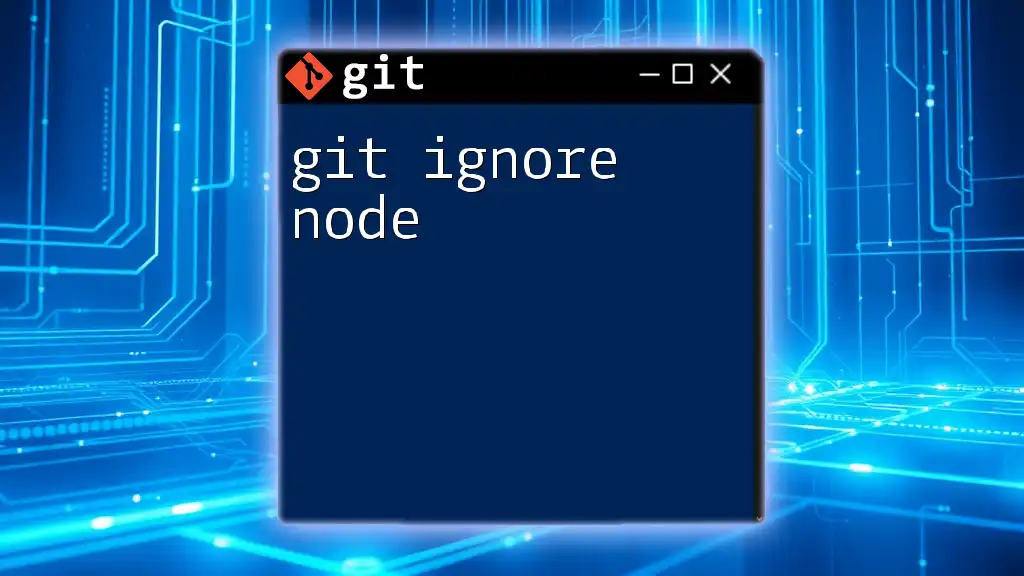
How to Ignore venv in Git
Creating a .gitignore File
To avoid committing the `venv` folder, you need to create a `.gitignore` file in the root of your Git project. You can do this manually, or by using the `touch` command:
touch .gitignore
Adding venv to .gitignore
Once you have your `.gitignore` file, you need to add the `venv` folder to it. Open the file in a text editor and insert the following line:
venv/
This instructs Git to ignore the `venv` directory specifically. The trailing slash denotes that it’s a directory and ensures that all files within will be ignored.
Checking If venv is Ignored
To confirm that Git is ignoring the `venv` folder properly, you can check the status of your repository. Run the following command:
git status
If everything is set up correctly, you should see that the `venv` directory is not listed among the untracked files.
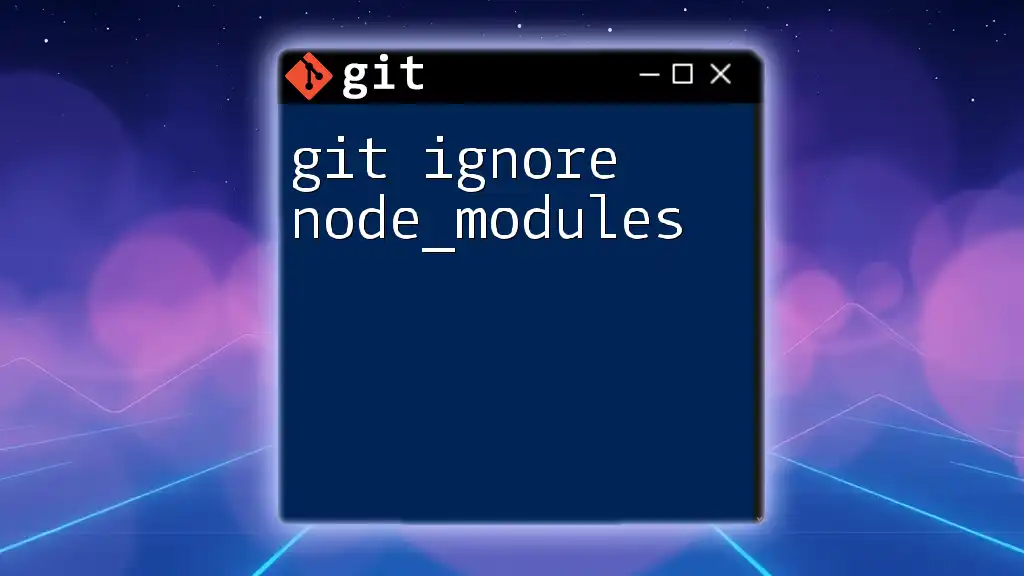
Best Practices for Managing Git and Virtual Environments
Keeping Your Project Organized
Maintaining an organized project directory is essential for efficiency. Use a standard project layout. Typically, a Python project might look like this:
my_project/
│
├── venv/ # This folder will be ignored
├── .gitignore # Contains venv/
├── requirements.txt # Lists your dependencies
├── main.py # Your main application code
└── README.md # Project documentation
Versioning Dependencies
While the `venv` directory should be ignored, it is vital to track your project’s dependencies. Use a `requirements.txt` file to document the packages needed for your project. After installing packages using pip, run:
pip freeze > requirements.txt
This command writes all installed packages, along with their versions, into `requirements.txt`. You can later recreate the same environment on another machine using:
pip install -r requirements.txt
Consistent Development Environment Across Teams
In team environments, it is crucial to ensure all members work in the same way even though individual setups may vary. Document the environment setup in your `README.md` file, and consider using tools like pipenv or poetry for defining and managing project dependencies. These tools facilitate a more streamlined and consistent approach for everyone on the team.
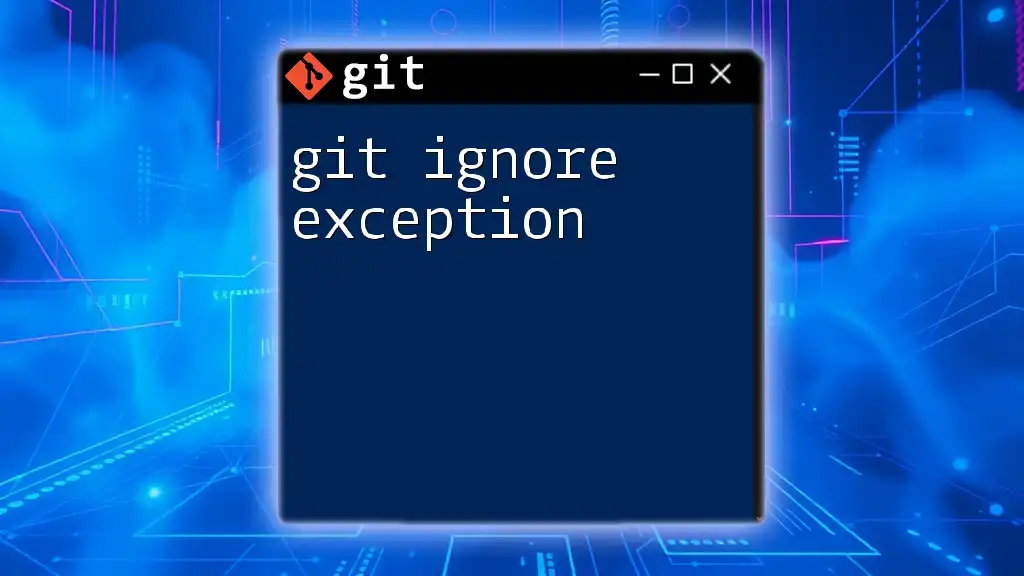
Conclusion
Incorporating a `.gitignore` file to ignore your `venv` directory is a small yet impactful best practice in Git version control. It keeps your repository clean and manageable while avoiding potential conflicts in development. Focus on tracking what matters most—your source code and documentation—and rely on files like `requirements.txt` for dependency management. By following these practices, you enhance collaboration and maintain a professional development workflow.
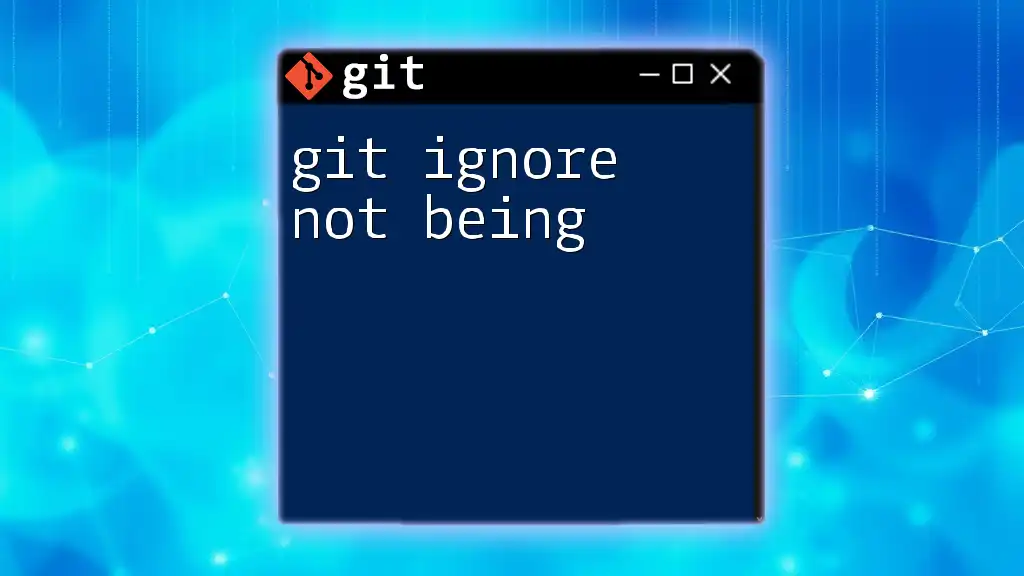
Additional Resources
For further reading on managing Git repositories and virtual environments in Python, consider checking out the official Git documentation and tutorials on virtual environments. Additionally, familiarize yourself with popular tools to facilitate a streamlined development experience, such as `pipenv` or `poetry`.