To instruct Git to ignore specific files or directories on a Mac, you can create or modify a `.gitignore` file in your repository with the patterns of the files you want to exclude. Here’s an example:
echo "*.log" >> .gitignore
Understanding Git and `.gitignore`
What is Git?
Git is a distributed version control system that helps developers collaborate on software projects efficiently. It allows multiple users to work on the same code without interfering with each other. Each change can be tracked, reverted, and managed easily, making it an essential tool in modern development workflows.
The Role of `.gitignore`
A `.gitignore` file tells Git which files or directories to ignore in a project. When you have files that do not need to be tracked—such as temporary files, configuration files, or system files—adding them to a `.gitignore` makes your repository cleaner and helps prevent sensitive data from being accidentally shared.
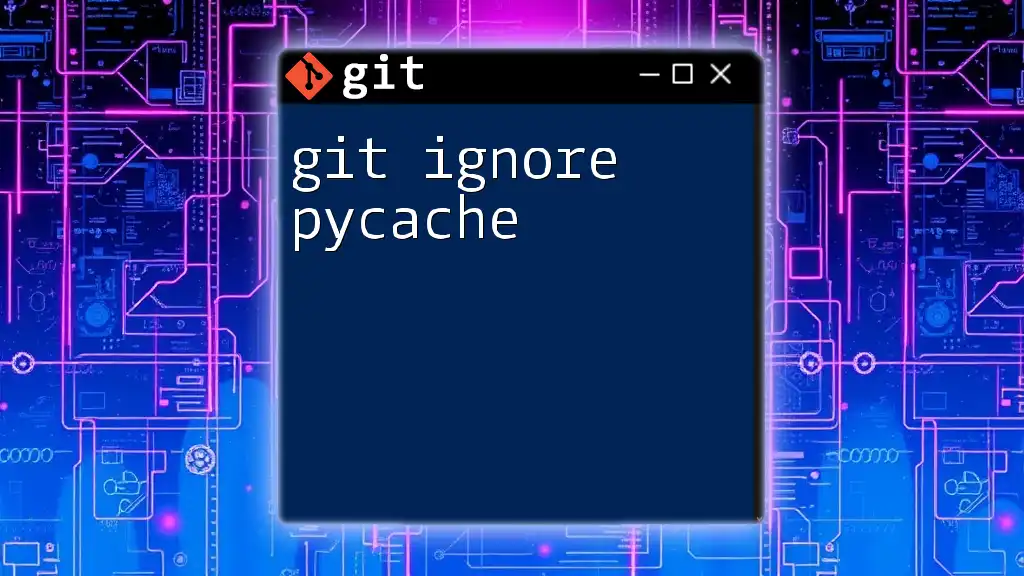
Setting Up the `.gitignore` File on macOS
Creating Your `.gitignore` File
To set up a `.gitignore` file on macOS, you first need to navigate to your project directory using Terminal. You can create the file by entering the following command:
cd /path/to/your/project
touch .gitignore
This command creates a new `.gitignore` file in your project directory.
Location of the `.gitignore` File
The placement of your `.gitignore` file is crucial. It is generally best practice to place it in the root directory of your project. However, if you have subdirectories that require different ignore settings, you may also create additional `.gitignore` files in those specific directories.
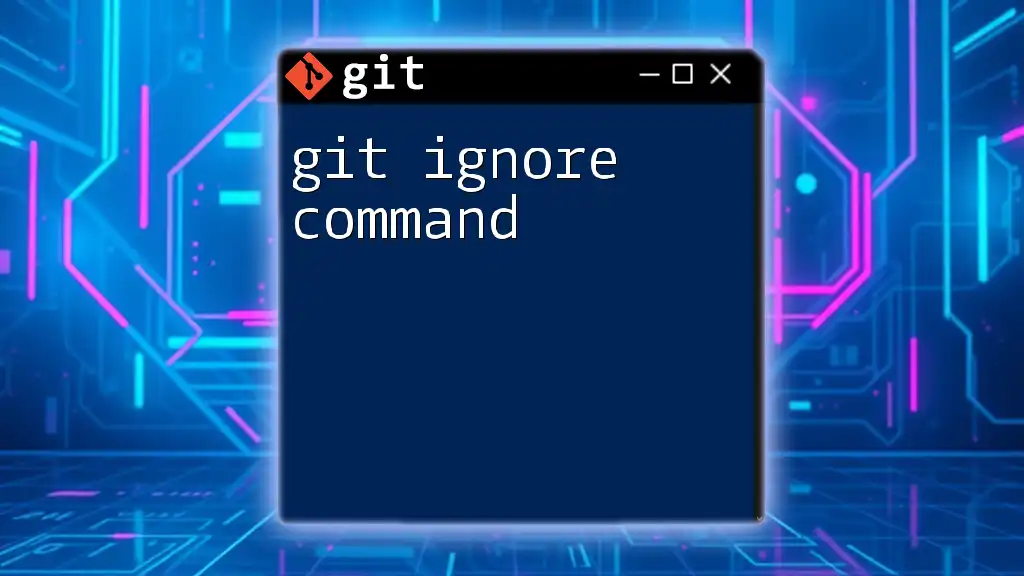
Configuring Your `.gitignore` File
Basic Syntax of `.gitignore`
The syntax of a `.gitignore` file is straightforward. You can specify which files or directories Git should ignore using patterns. Here are some important aspects of the syntax:
- Wildcards: Use characters like `*` to match any number of characters and `?` for a single character.
- Comments: Anything following a `#` will be treated as a comment and ignored.
Patterns and Examples
When configuring your `.gitignore` file, you can include patterns for files and directories that should be ignored. Here are a few common examples:
-
To ignore all log files:
*.log
-
To ignore temporary files:
*.tmp
-
To ignore the `node_modules` directory, which is common in JavaScript projects:
node_modules/
Conditional Ignoring
In some cases, you might want to ignore certain files while keeping others. You can utilize negation patterns for this purpose. For example, if you want to ignore all log files except for `error.log`, you would write:
*.log
!error.log
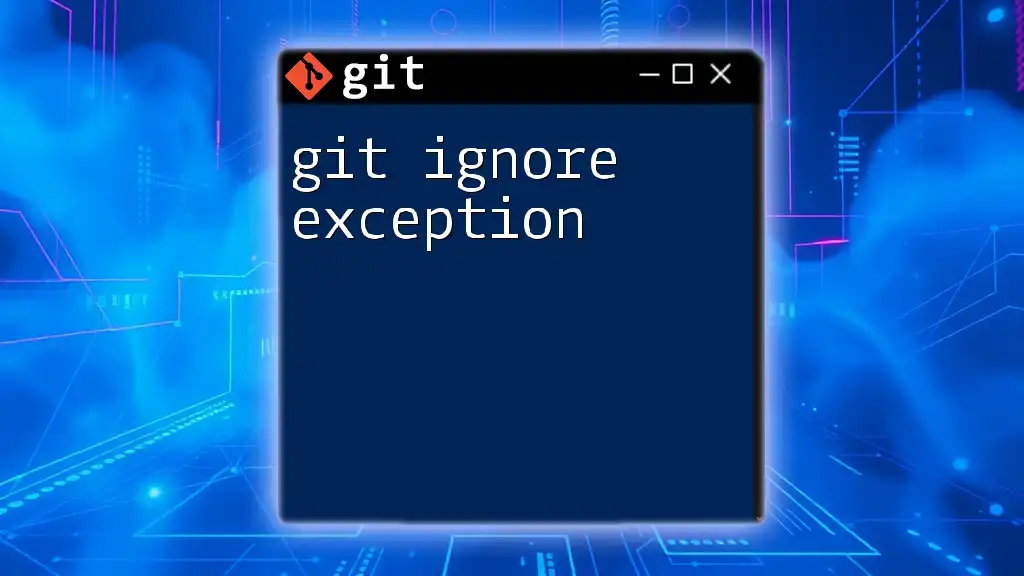
Common Use Cases for `.gitignore` on macOS
Ignoring macOS System Files
On macOS, certain system files are automatically generated but are not needed in your repository. One of the most common files to ignore is `.DS_Store`. This file is created by the Finder to store custom attributes of a folder. To ignore it, add the following line to your `.gitignore`:
.DS_Store
Ignoring Configuration Files
Most Integrated Development Environments (IDEs) or code editors generate configuration files that you may not want to include in your version control. Here are a few examples of such files:
-
To ignore configuration files from JetBrains IDEs:
.idea/
-
To ignore Sublime Text workspace files:
*.sublime-project
Temporary Files
Build artifacts and caches can also clutter your repository. Here are examples of directories that are often ignored to keep your version control clean:
dist/
build/
*.tmp
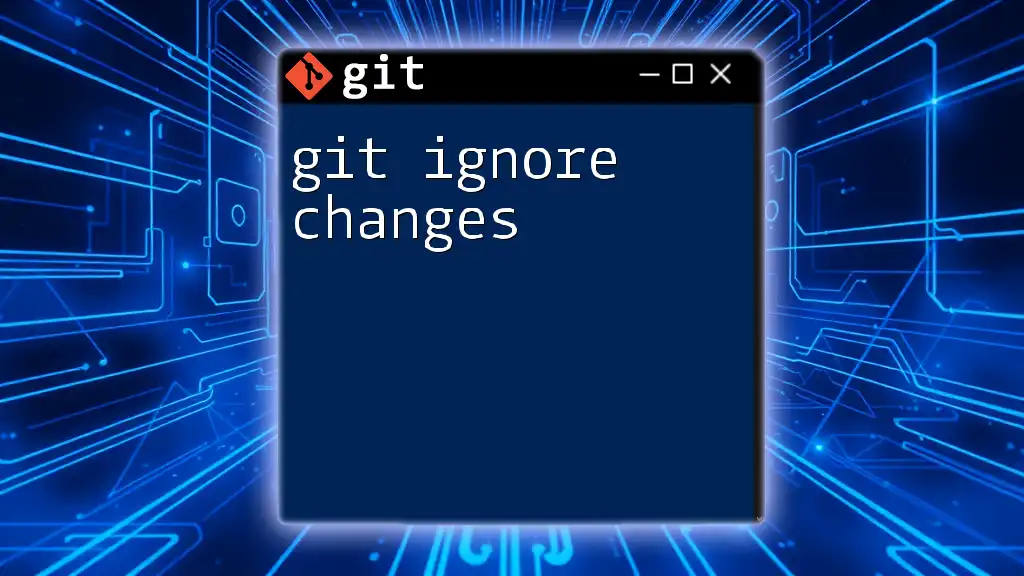
Mac-Specific Tips for `.gitignore`
Utilizing Global `.gitignore`
If you want to ignore certain files across all your projects on macOS, you can set up a global `.gitignore` file. This can be particularly useful for system files that are not relevant to any of your projects. Here’s how to create a global ignore file:
First, create the global ignore file:
touch ~/.gitignore_global
Next, configure Git to use this global ignore file:
git config --global core.excludesfile ~/.gitignore_global
Finally, add common entries for files you want to ignore globally:
.DS_Store
*.log
Using `.gitignore` with Homebrew Projects
If you are working with Homebrew projects, you may want to manage the packages and their configurations effectively. Often, package directories and cache files can be ignored. For instance, you can add:
Cellar/
This pattern helps to prevent Homebrew installation paths from cluttering your repositories.
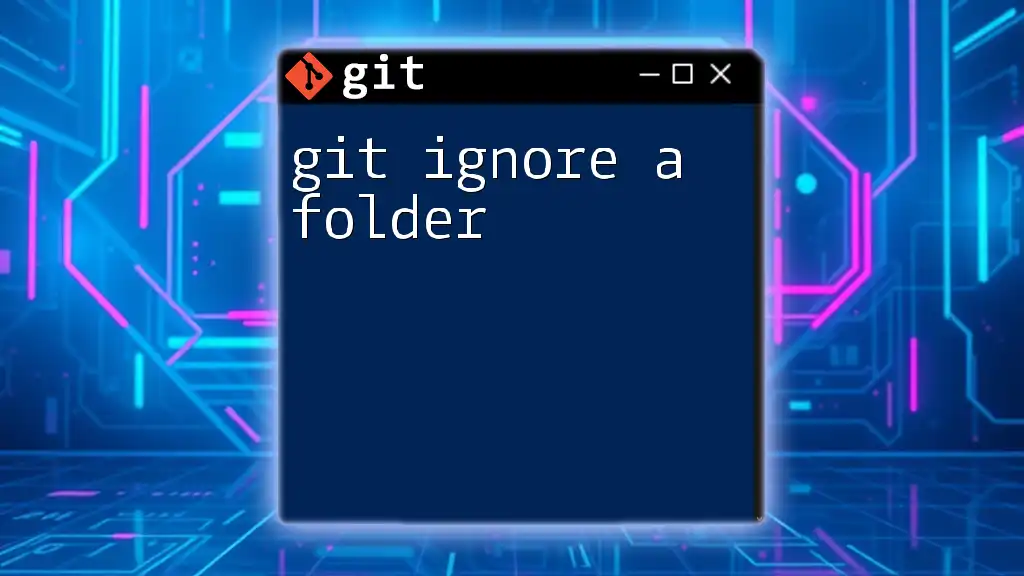
Testing Your `.gitignore`
Verifying Ignored Files
Once you’ve configured your `.gitignore`, it’s important to verify that it’s working as intended. You can do this by using the `git check-ignore` command to inspect specific files:
git check-ignore -v <file>
This command gives you information about why a specific file is ignored.
Troubleshooting Common Issues
Sometimes, files may still appear in your Git status even after being added to `.gitignore`. This can happen if the files were already tracked by Git before you added them to your `.gitignore`. To resolve this, you’ll need to remove the files from tracking but keep them locally using:
git rm --cached <file>
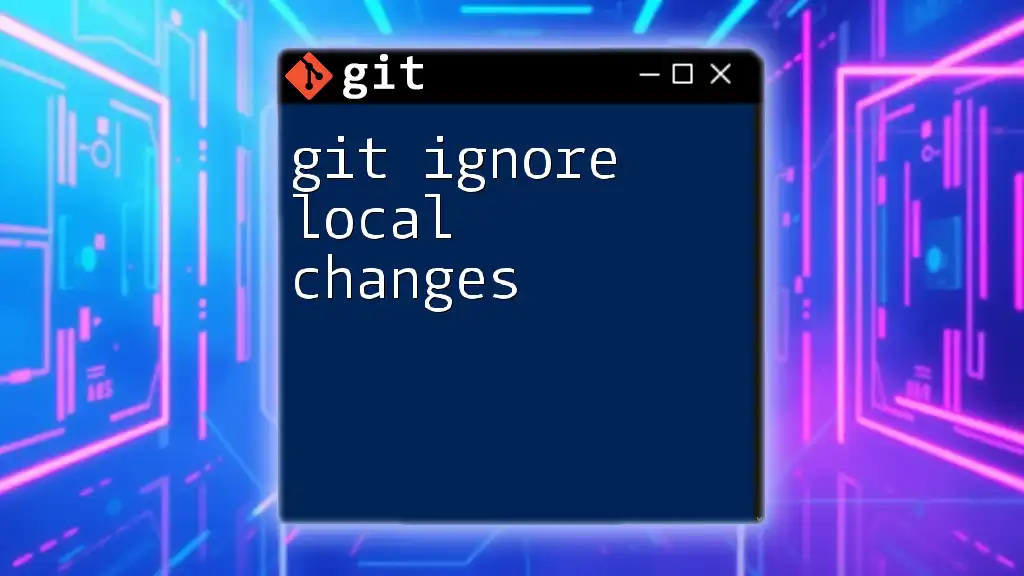
Conclusion
Using a `.gitignore` file effectively is essential for maintaining a clean and organized repository. It helps prevent unnecessary files from being tracked, which allows for easier collaboration and version management. By following the guidelines outlined in this article, you can ensure that your Git projects on macOS are free from clutter, making your development experience smoother and more efficient.
Additional Resources
For further reading, refer to the official Git documentation or community forums dedicated to Git support. These resources are invaluable as you deepen your understanding of Git and refine your usage of `.gitignore`.