To apply a commit from one branch to another in Git, you can use the `cherry-pick` command, which allows you to select a specific commit by its hash and apply its changes to the current branch.
git cherry-pick <commit-hash>
Understanding Git Commits
What Is a Git Commit?
A Git commit is a fundamental concept in version control that serves as a snapshot of your project's changes at a specific point in time. Each commit records a set of changes along with a commit message, which describes what changes were made and why. This enables you to track the history of your project, review previous work, or even revert to earlier versions if necessary.
The Structure of a Commit
Every commit consists of several components:
- Commit Hash: A unique identifier for the commit generated by Git.
- Author: The person who made the changes.
- Timestamp: The date and time when the commit was made.
- Commit Message: A brief explanation of the changes introduced in that commit.
It's crucial to write clear and descriptive messages for your commits because they serve as documentation for your project’s evolution.
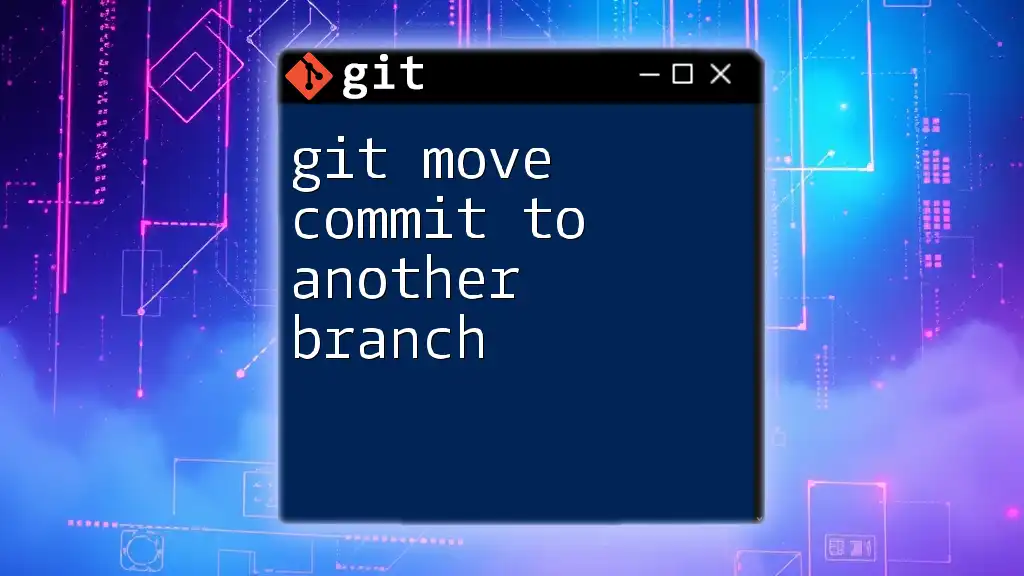
Git Branching Basics
What Are Git Branches?
Branches in Git enable you to develop features or fix bugs in isolation without affecting the main project timeline. A branch encapsulates a series of commits, allowing multiple development efforts to occur simultaneously. This way, team members can work on different features independently.
The Role of the Master Branch
Traditionally, the master branch (now often referred to as the main branch) is the default branch that contains the official project history. New features or fixes are typically developed in separate branches and then merged back into the master branch after satisfactory review and testing.
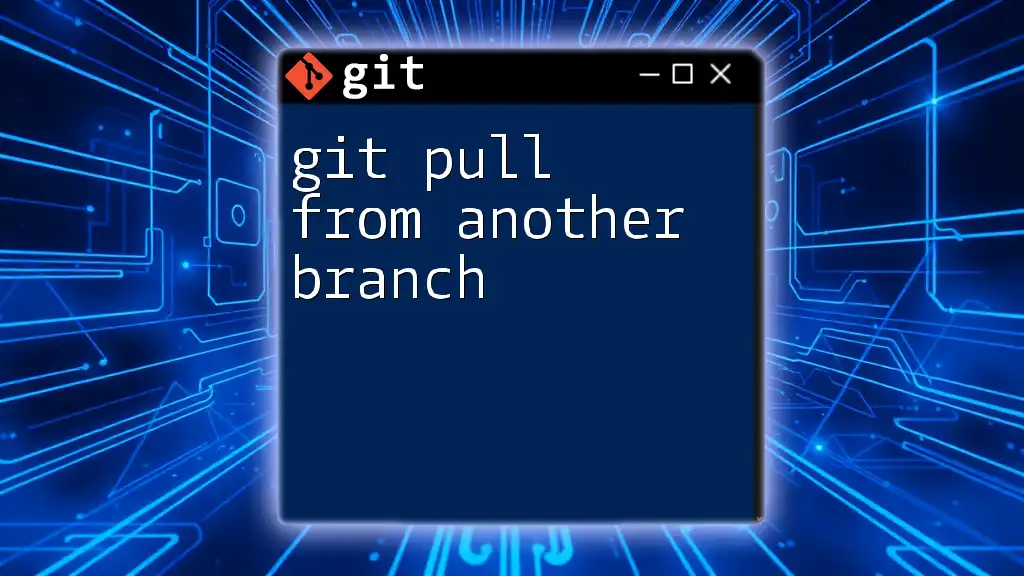
Prerequisites for Applying Commits
Recommended Knowledge
Before you can apply a commit to another branch, it's essential to have a basic understanding of some key Git commands, including:
- `git add`: To stage changes.
- `git commit`: To create commits.
- `git checkout`: To switch between branches.
Setting Up Your Environment
Make sure you have Git installed on your computer. For practice, create a sample repository to experiment with applying commits.
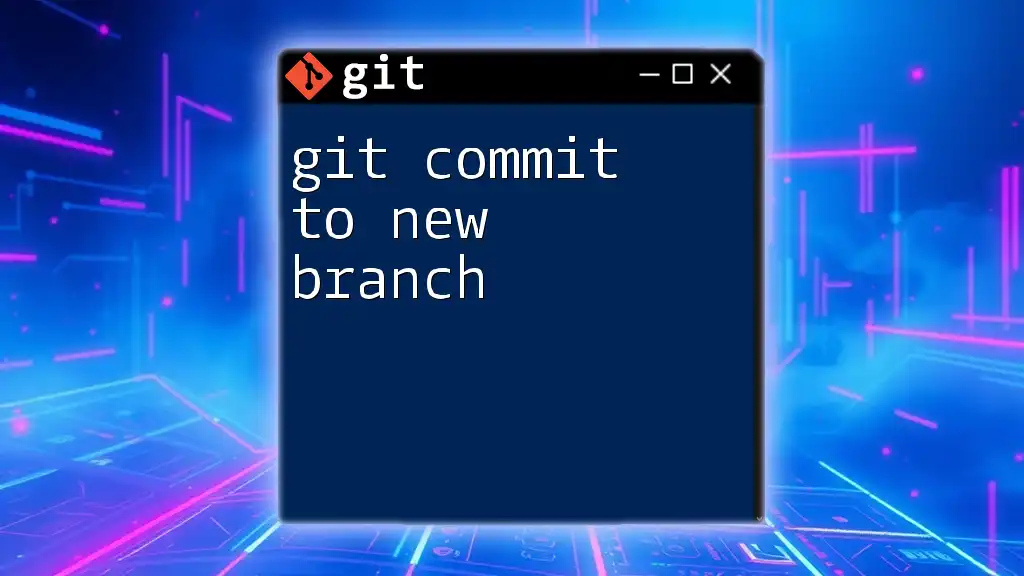
Using `git cherry-pick` to Apply Commits
What Is `git cherry-pick`?
The `git cherry-pick` command allows you to apply the changes introduced by a specific commit from one branch onto another. Unlike merging, which incorporates all the changes from a branch, cherry-picking is useful when you want only particular changes without merging the entire branch.
Step-by-Step Guide to Cherry-Picking a Commit
Step 1: Identifying the Commit Hash
To apply a commit, you first need to find its unique commit hash. You can do this by using the following command, which displays the commit history:
git log
Step 2: Checking Out the Target Branch
Before you apply the commit, ensure you’re on the branch where you want the changes to appear. Switch to the target branch using:
git checkout target-branch
Step 3: Executing the Cherry-Pick
Now, you can apply the desired commit by using its hash:
git cherry-pick <commit-hash>
Handling Conflicts During Cherry-Picking
Sometimes, cherry-picking can lead to merge conflicts if the changes in the commit conflict with existing code in your target branch.
- Identify Conflicting Files: After executing the cherry-pick command, Git will report conflicts in specific files.
- Resolve Conflicts Manually: Open the conflicting files and manually adjust the changes. Look for conflict markers (`<<<<`, `====`, `>>>>`) to find sections needing your attention.
- Mark Files as Resolved: After resolving conflicts, stage the files as follows:
git add <filename>
- Completing the Cherry-Pick Operation: Finalize the cherry-pick with:
git cherry-pick --continue
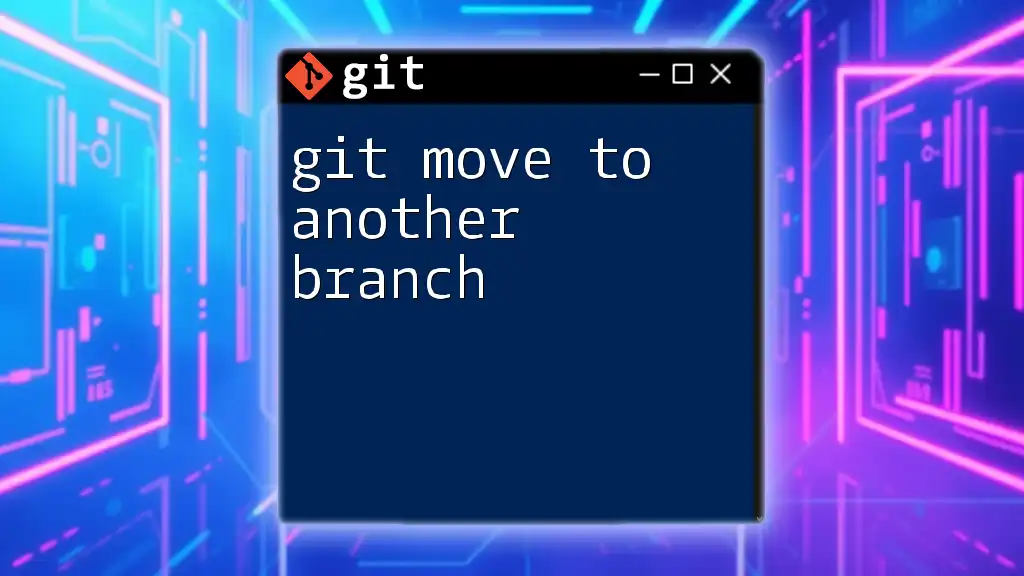
Using `git apply` for a More Complex Scenario
What Is `git apply`?
Unlike `cherry-pick`, `git apply` is primarily used to apply patch files to a branch. It is beneficial when dealing with more complex changes or when you want greater control over modifications.
Creating a Patch from a Commit
To create a patch file from a specific commit that contains the changes you want to apply elsewhere, you can use:
git format-patch -1 <commit-hash>
This command generates a `.patch` file that contains the commit's changes.
Applying a Patch to Another Branch
To apply this patch to your target branch, follow these steps:
-
Checkout the Target Branch:
git checkout target-branch
-
Applying the Patch: Use the `git apply` command with the patch file you created:
git apply <patch-file>
Verifying Applied Changes
After applying the patch, it’s essential to confirm that the changes were successfully integrated. You can check the status and see differences with the following commands:
git status
git diff
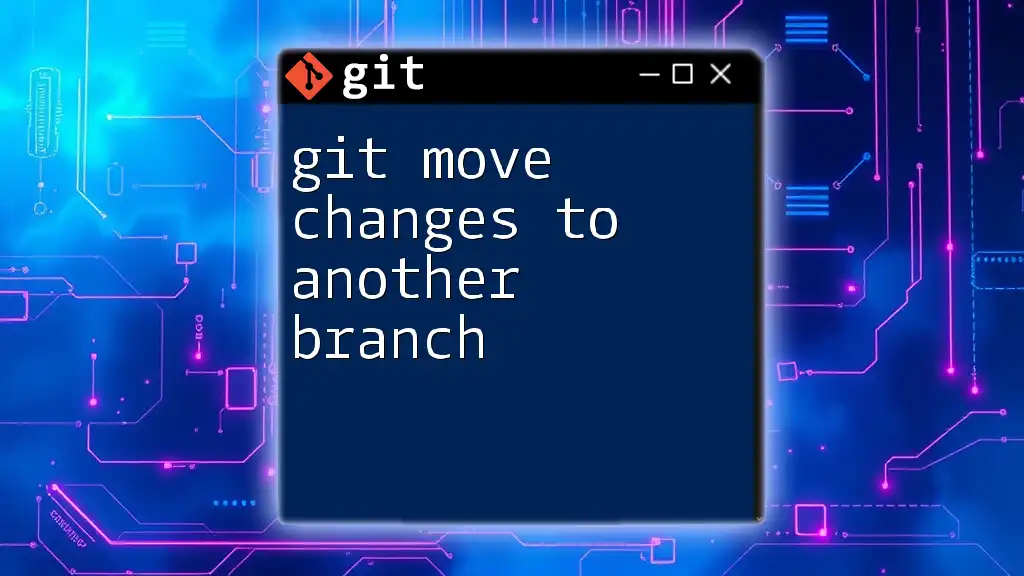
Best Practices for Applying Commits
When to Apply Commits
Applying commits should be done thoughtfully. Consider applying specific commits when you want to introduce critical fixes or features into a different line of development without merging the entire branch. This approach minimizes disruptions to your project.
Keeping Commit History Clean
A clean commit history is crucial for maintaining an understandable project timeline. To achieve this, follow these tips:
- Avoid unnecessary cherry-picks: Only apply commits that are relevant and necessary.
- Write clear messages: Each commit should tell a concise story of changes made.
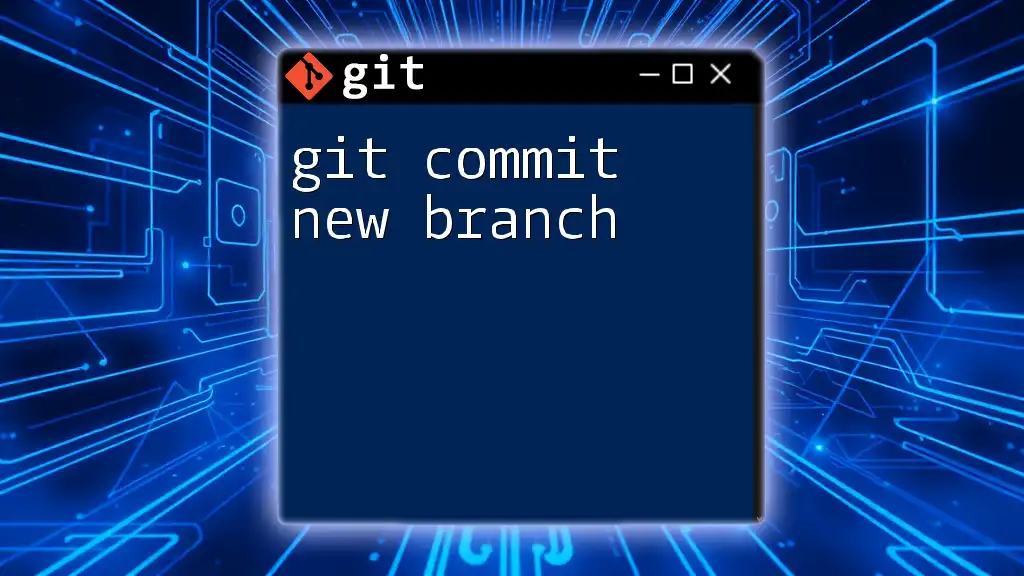
Conclusion
In this guide, you’ve learned how to use both `git cherry-pick` and `git apply` to manage commits across branches in Git. By mastering these commands, you'll gain greater flexibility in your version control practices, ensuring that you can implement changes thoughtfully and efficiently. As you practice these techniques, you'll find them invaluable in your development workflow.
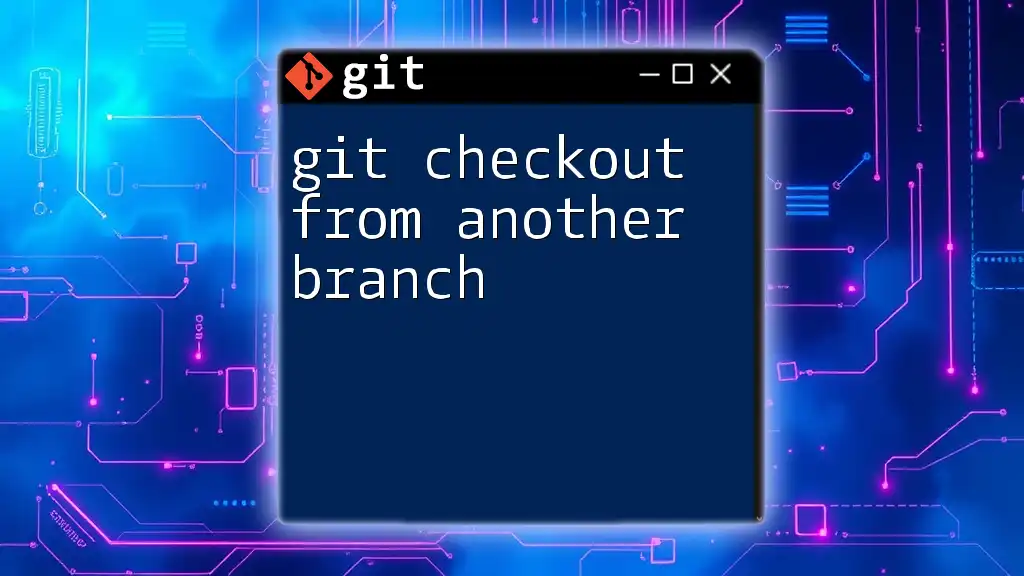
Additional Resources
- For further reference, consult the official Git documentation.
- Consider other resources such as books or online courses to deepen your understanding of Git commands and workflows.
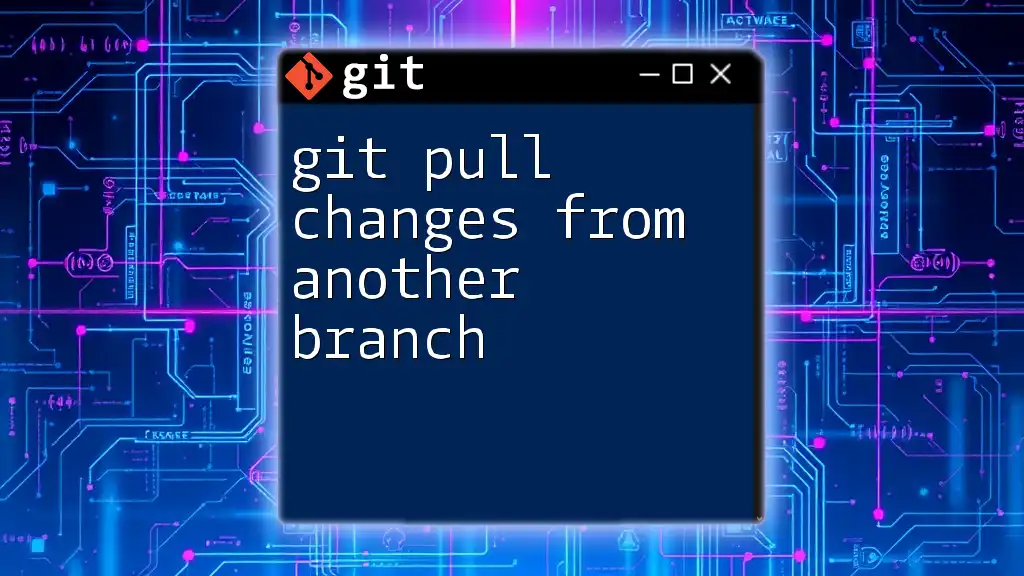
Call to Action
Practice these techniques on your projects and explore the community for more tips on using Git effectively. Don't hesitate to share this knowledge with others embarking on their Git journey!