Git squash commits on a branch allows you to combine multiple commits into a single, more manageable commit, streamlining your commit history.
git rebase -i HEAD~n
Replace `n` with the number of commits you want to squash, then change `pick` to `squash` for the commits you want to combine in the interactive rebase editor.
What is Git Squashing?
Git squashing is the process of combining multiple commits into a single commit. This technique helps maintain a cleaner and more meaningful commit history, making it easier for collaborators to understand the evolution of a project. By squashing and consolidating commits, developers can present a summary of changes instead of a cluttered record of every small modification made.
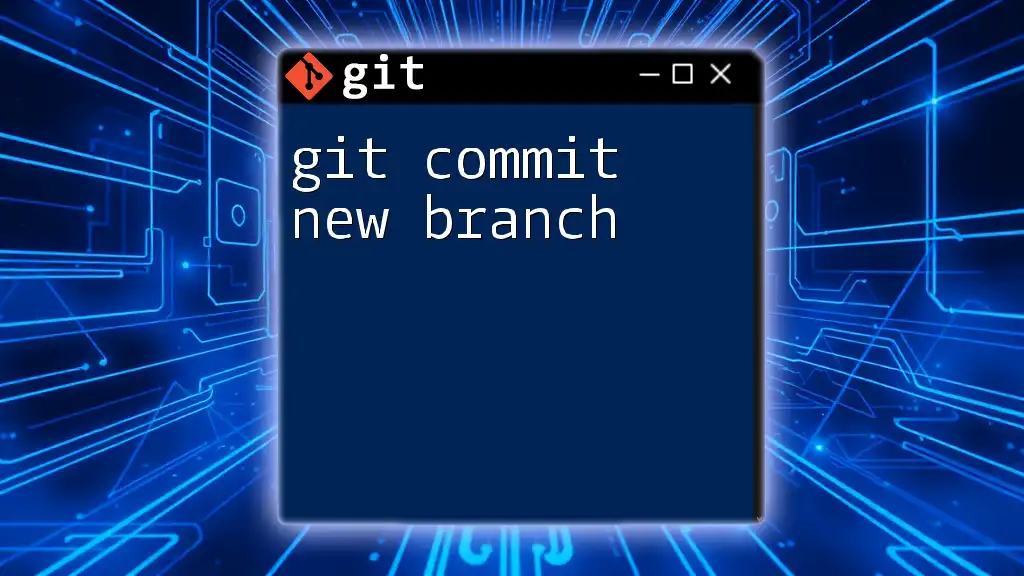
When to Use Git Squashing
Squashing commits is especially beneficial in scenarios where you've made numerous small commits while working on a feature or fix. Here are some key situations when squashing is recommended:
- When developing a feature that ends up with multiple commits as you refine or iterate on your work.
- Before submitting a pull request, to ensure the commit history is clear and concise for the reviewers.
- When collaborating in a team environment that values a clean and professional commit history.
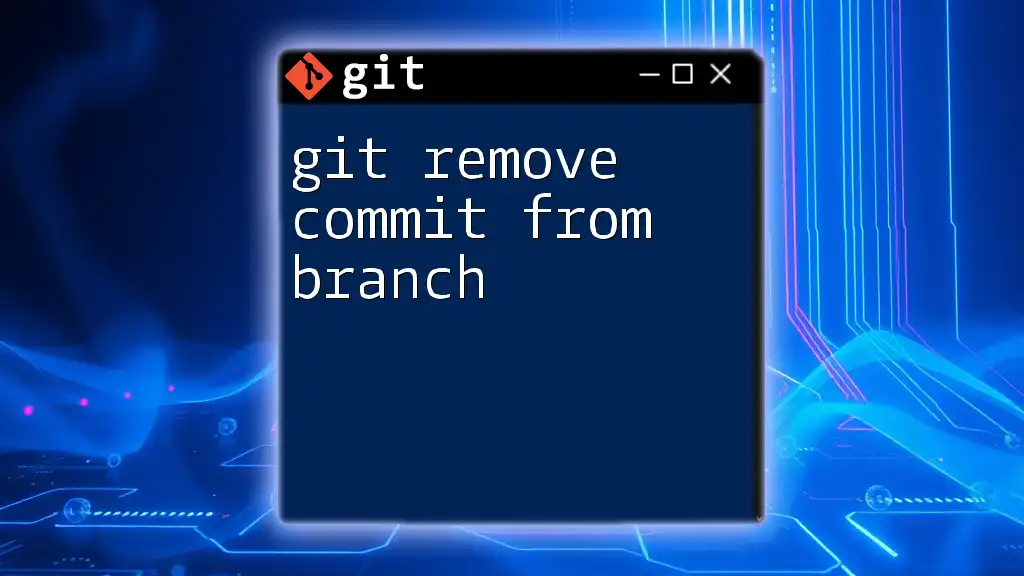
Understanding Git Commits
What Are Git Commits?
A Git commit is a snapshot of your project's changes. Commits hold meaningful information:
- Commit Message: Describes the changes made, providing context for future developers.
- Author Information: Shows who made the changes.
- Timestamp: Indicates when the changes were made.
Creating meaningful commits is essential for a collaborative workflow, as they serve as the building blocks of code history.
How Commits Work in Branches
Git allows you to create branches, which are separate lines of development within a project. Each branch can have its own commits, allowing for experimentation without affecting the main codebase. Commits in branches can later be merged into the main branch, forming a coherent development process.
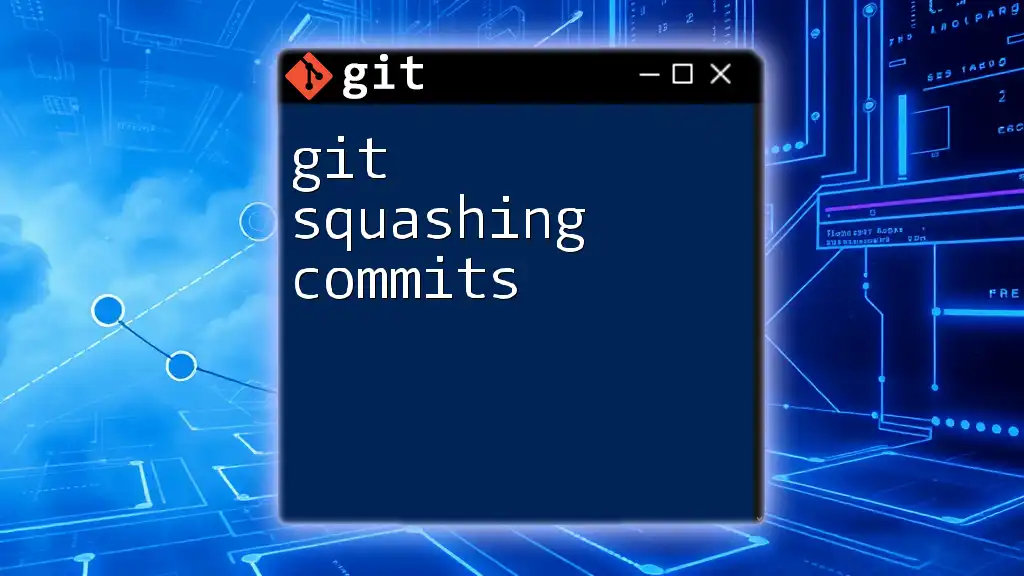
The Benefits of Squashing Commits
Cleaner Project History
A clean project history is both visually appealing and enhances comprehension among team members. Multiple small commits clutter the repository's history, making it challenging to understand the overall changes. By contrast, a squashed commit presents a single, cohesive change that encapsulates all relevant modifications.
For instance, consider the difference between the following commit histories:
-
Messy History:
Fix typo Add initial structure Update README Refactor code
-
Squashed History:
Implement feature X with README updates
Easier Code Review
Squashing commits simplifies the code review process. Reviewers can focus on a compact set of changes, rather than navigating through numerous incremental updates. This enables a more thorough review of the significant evolution of feature development, rather than getting lost in the minutiae.
Improved Traceability
By squashing commits, you can maintain meaningful commit messages that summarize key changes. This is essential for traceability, making it easier to understand the context behind modifications when reviewing the project history. For instance, a single commit titled "Revamp feature X to enhance user experience" is more insightful than several vague commits that do not provide such context.
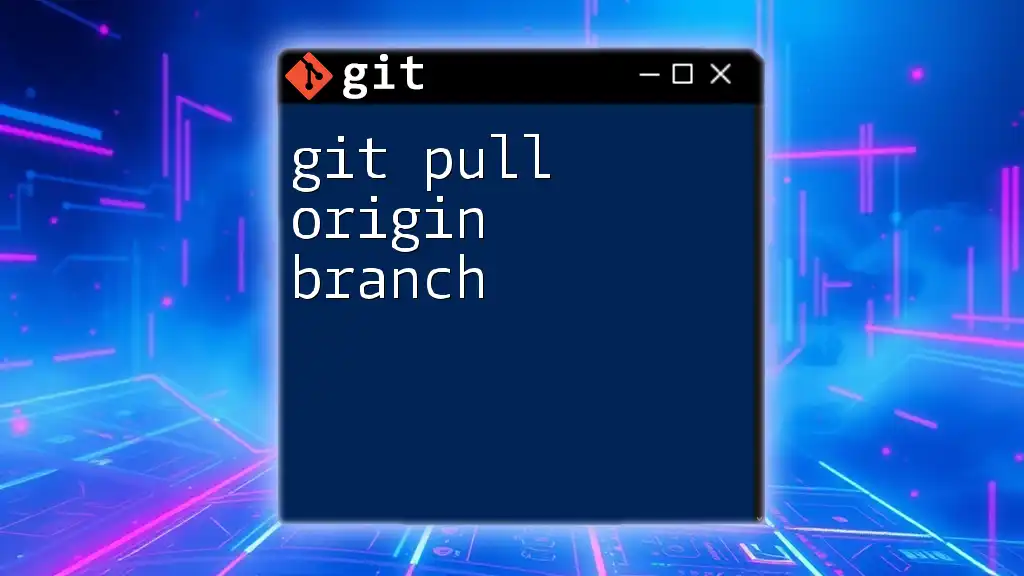
Preparing to Squash Commits
Setting Up Your Environment
Before you proceed with squashing commits, ensure your Git environment is ready:
- Use a clean working directory: Ensure all changes are committed or stashed before performing a rebase.
- Backup your branch: You may want to create a temporary branch to save your original work, allowing you to revert if needed.
Identifying Commits to Squash
To identify the commits you want to squash, you can view the commit history with the following command:
git log --oneline --graph
This command provides a compact view of your commits, showcasing the commit messages along with their related commit hashes. You can identify which commits are most suitable for squashing based on a chronological understanding of your changes.
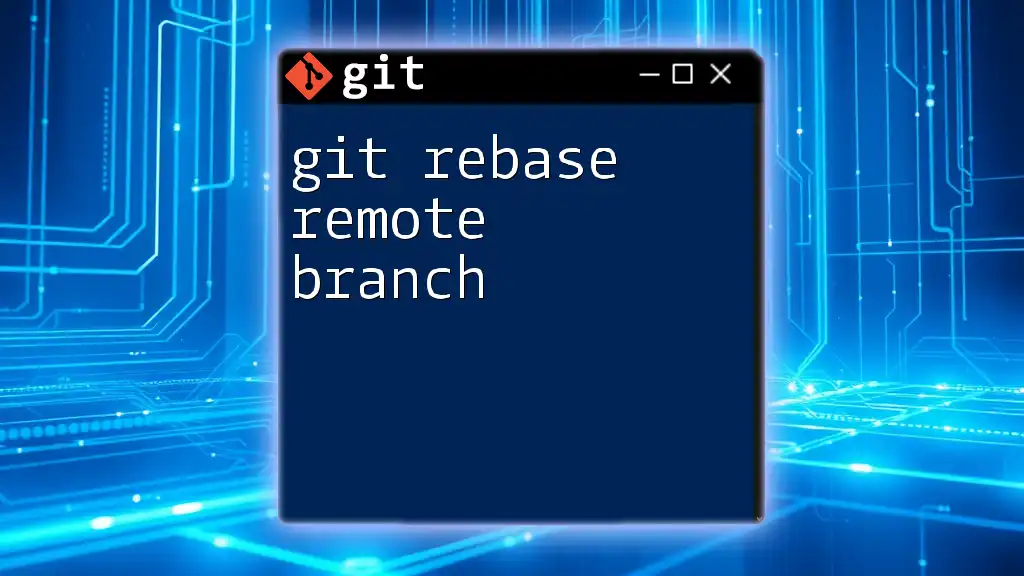
Squashing Commits in Git
Using Git Rebase for Squash
The most common way to squash commits is through an interactive rebase. This involves rewriting the commit history to combine changes. The basic command for squashing using rebase is:
git rebase -i HEAD~n
In this command, replace `n` with the number of commits you want to view and potentially squash. Here's a step-by-step guide:
- Run the Rebase Command: Execute the command to enter the interactive rebase mode.
- Choose Commits to Squash: An editor will open listing your commits. Change `pick` to `squash` (or `s` for short) for the commits you want to combine with the previous commit.
- Modify Commit Messages: After confirming the squashes, you can edit your commit message to reflect the changes in a meaningful way. This new message will represent the combined commit.
Using the Squash Option in Merge
You can also squash commits directly while merging a branch. To do this, you would use:
git merge --squash branch-name
This command takes all changes from the specified branch and squashes them into the current branch without creating a separate commit for the merges. This is especially useful when integrating a completed feature branch into the main branch.
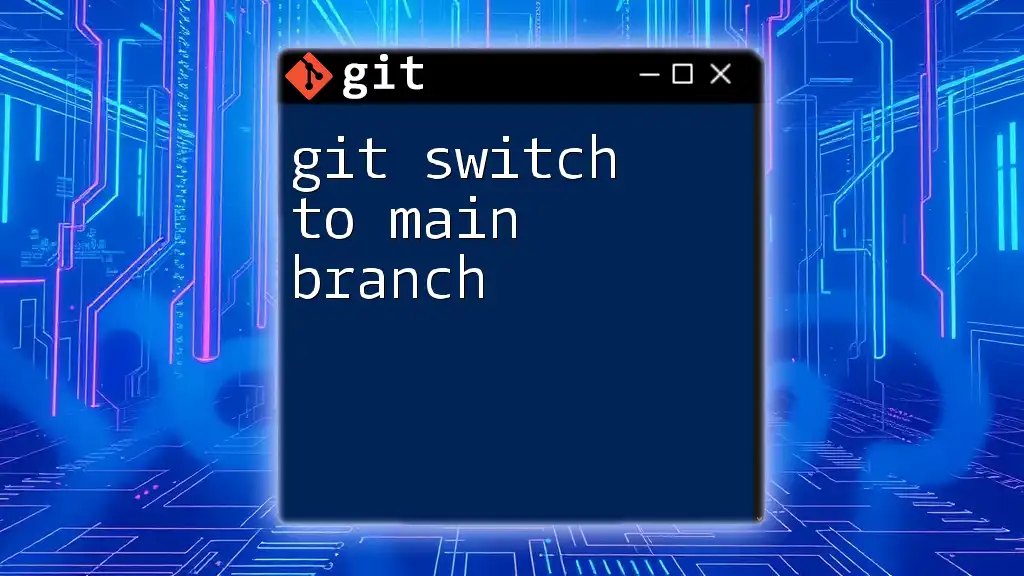
Resolving Conflicts During Squashing
Common Conflict Scenarios
When squashing commits, conflicts may arise, particularly if multiple changes overlap in the same files. This means Git is unable to automatically merge changes, requiring manual intervention.
Steps to Resolve Conflicts
If you encounter a conflict during a squash, follow these steps:
-
Check the Status: Use `git status` to see the list of files that have conflicts.
-
Open a Merge Tool: You can use a visual merge tool by running:
git mergetool
-
Resolve Conflicts Manually: Edit the affected files to resolve the conflicts, keeping the desired changes and discarding or modifying conflicting lines.
-
Complete the Rebase or Merge: After resolving conflicts, continue the rebase with:
git rebase --continue
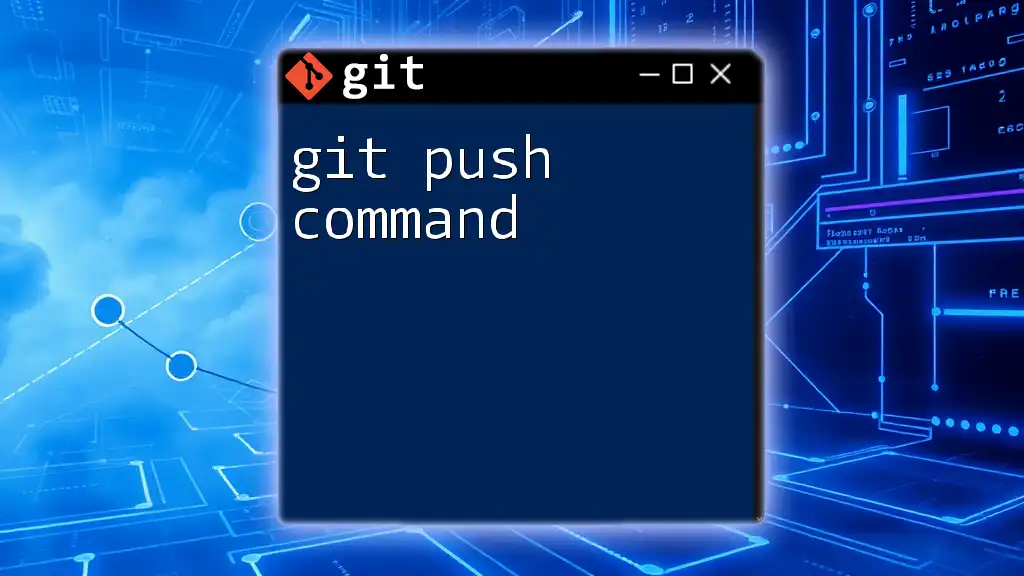
Finalizing Your Squash
Committing the Squashed Changes
Once you've squashed the commits and resolved any conflicts, it's time to commit the changes. Ensure that your commit message is descriptive and conveys the essence of the combined changes, such as:
Implement feature X and update documentation
Pushing Your Changes
After squashing and committing, you will need to push the changes to the remote repository. If you have rewritten history, you must use the `--force` option:
git push origin branch-name --force
This command updates the remote branch with your squashed commits, ensuring that the history remains clean and fresh for collaboration.
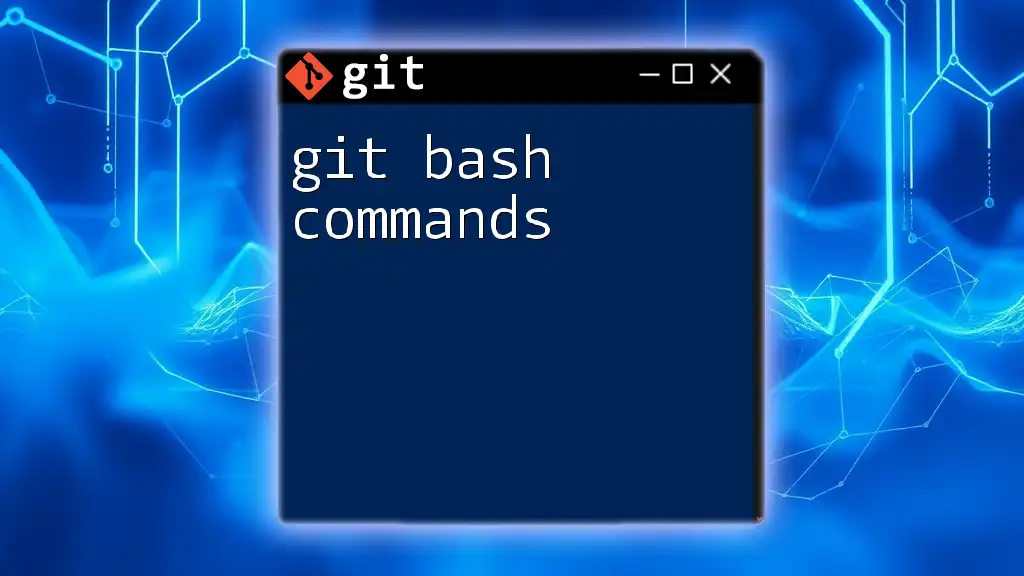
Best Practices for Squashing Commits
Commit Message Guidelines
Make it a habit to write clear and precise commit messages. Good practices include:
- Use the imperative mood (e.g., "Fix bug" instead of "Fixed bug")
- Keep it concise but descriptive enough for clarity
- Avoid jargon, and ensure it's understandable to future collaborators
Maintaining a Clean History
Regularly squash commits as part of your development process. Encourage your team to follow a policy of squashing commits before merging feature branches to the main branch. This maintains a clear and professional commit history.
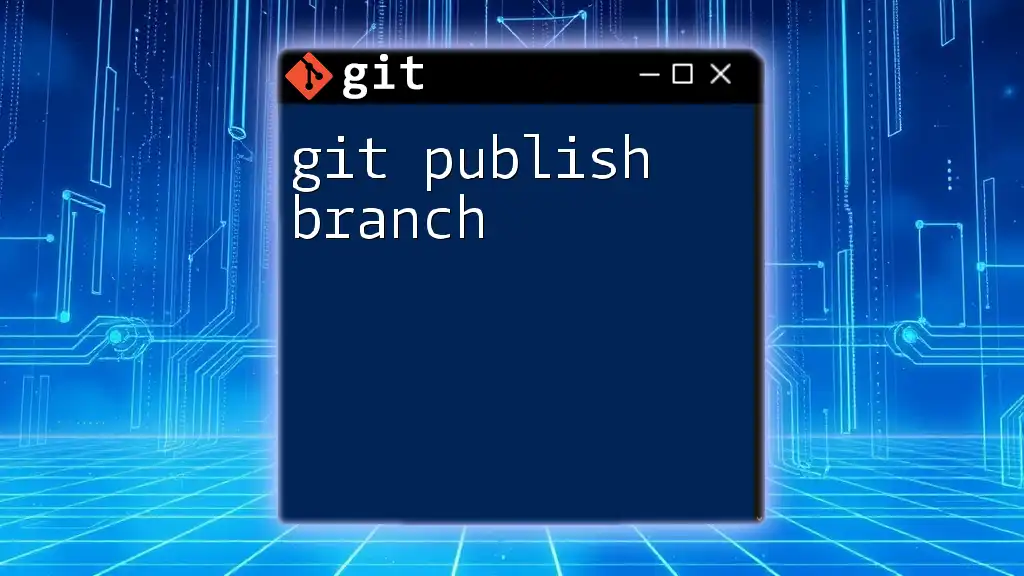
Conclusion
In conclusion, squashing commits on branches is a powerful technique for improving the clarity and professionalism of your Git commit history. It simplifies code reviews, enhances traceability, and helps maintain a cleaner project history. By following the outlined steps and best practices, you ensure that your project remains easy to navigate and comprehend for all contributors.
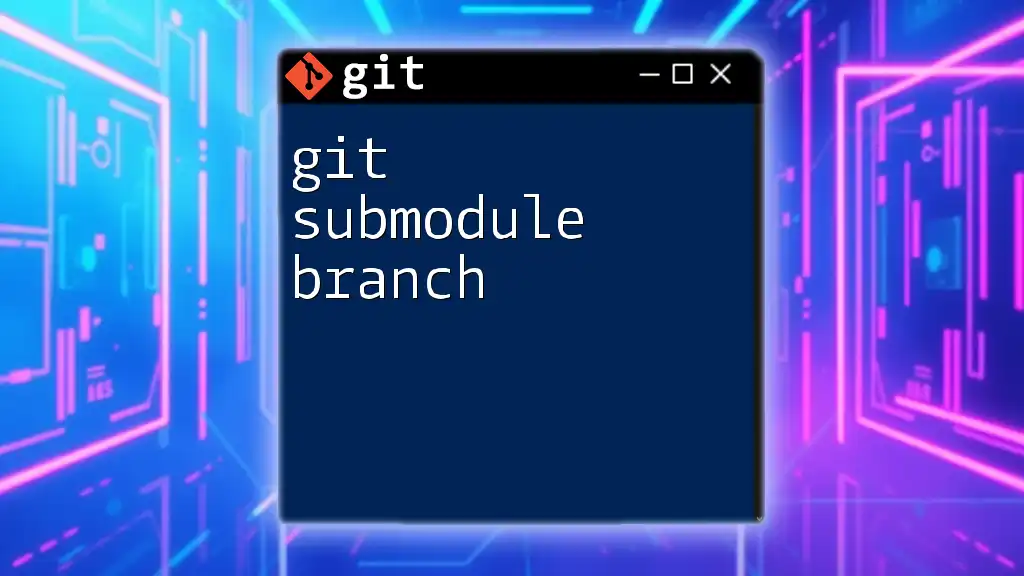
Additional Resources
For further reading, check out trusted Git resources and tutorials to deepen your understanding and skills. Additionally, consider exploring GUI tools that simplify commit management, ensuring an efficient workflow.
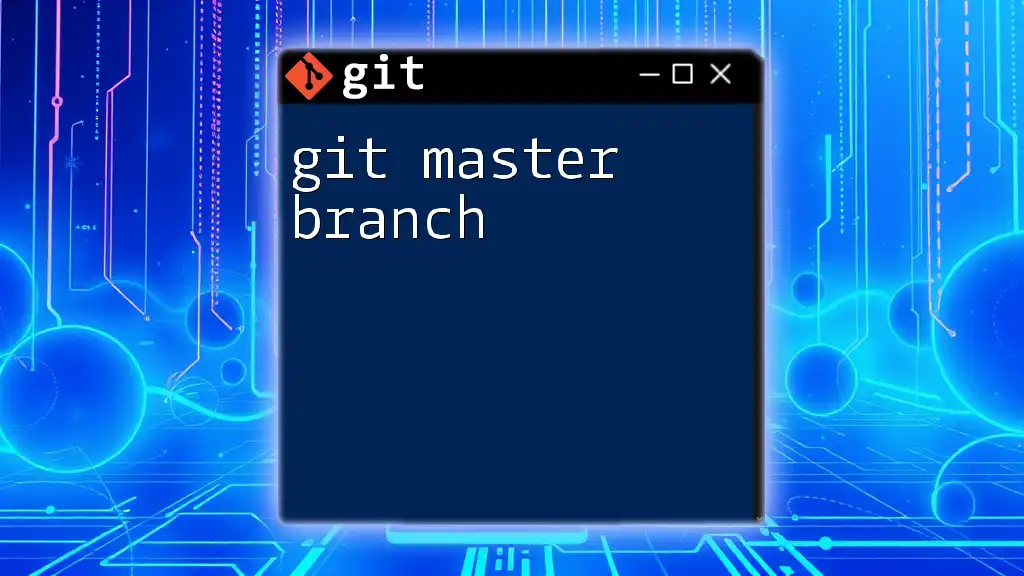
Call to Action
We invite you to join our Git training sessions to gain hands-on experience and mastery over essential Git commands, including squashing commits, to enhance your workflow and collaboration.