To switch to another branch in Git, use the command `git checkout` followed by the branch name you want to move to. Here's the command in markdown format:
git checkout branch-name
Understanding Branches in Git
What is a Branch?
A branch in Git is essentially a pointer to a specific commit in the repository’s history. It allows developers to work on different parts of a project independently, without affecting the main codebase. By utilizing branches, you can develop features, fix bugs, or experiment with new ideas in isolation.
Why Use Branches?
Using branches is pivotal in a collaborative development environment. Here are some key reasons to branch:
- Feature Development: Create a feature branch for each new feature you're building. This makes it easy to isolate changes and integrate them later.
- Bug Fixes: Fix issues in dedicated branches to prevent instability in the main branch.
- Experimentation: Safely experiment with new ideas without affecting the main project.
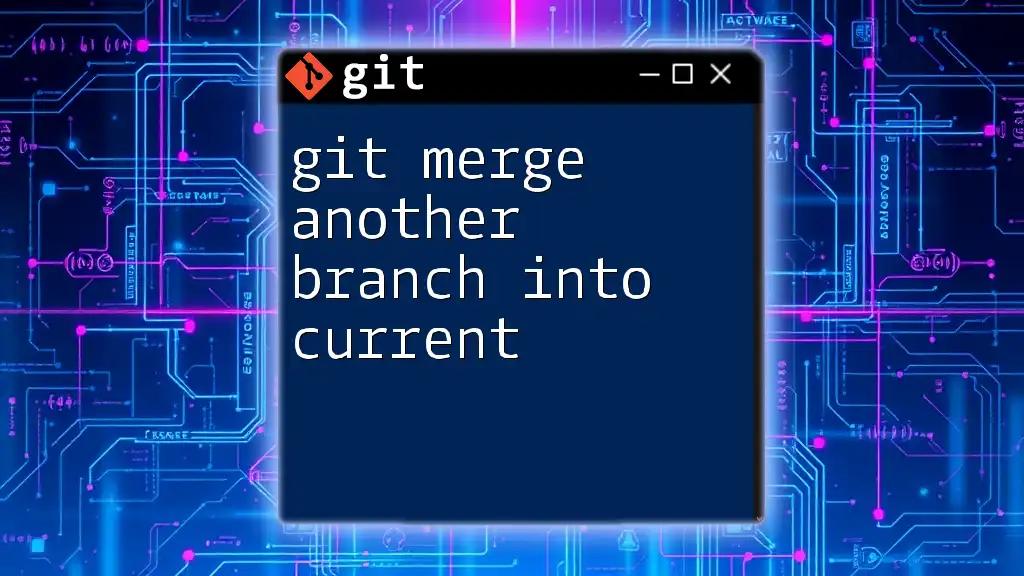
Moving to Another Branch in Git
What Does "Switching Branches" Mean?
Switching branches means changing your working directory to point to another branch's latest commit. This action allows you to continue working from where you left off on that branch, keeping your changes well-organized.
Using the `git checkout` Command
Introduction to `git checkout`
The `git checkout` command is a fundamental Git command used for switching branches and navigating your project’s history.
Basic Syntax
To switch to another branch, you can use the following syntax:
git checkout <branch-name>
Example: Switching to a Branch
If you need to switch to a branch called `my-feature-branch`, you would use:
git checkout my-feature-branch
This command effectively changes your working directory to reflect the state of `my-feature-branch`, including files and changes associated with that specific branch.
The Modern Way: Using `git switch`
Introduction to `git switch`
Introduced in later versions of Git, the `git switch` command serves a similar purpose to `git checkout`, but it is specifically designed for switching branches. This makes it clearer and easier to use, especially for newcomers.
Basic Syntax
The syntax to switch to another branch using `git switch` is straightforward:
git switch <branch-name>
Benefits Over `git checkout`
The introduction of `git switch` improves usability by eliminating potential confusion between switching branches and checking out files. It clearly delineates the actions:
- Switching branches: `git switch`
- Checking out files: `git restore`
Example: Switching to a Branch
If you want to switch to `my-feature-branch` using `git switch`, you'd run:
git switch my-feature-branch
This command works just like `git checkout`, but is framed in a way that minimizes confusion for users.
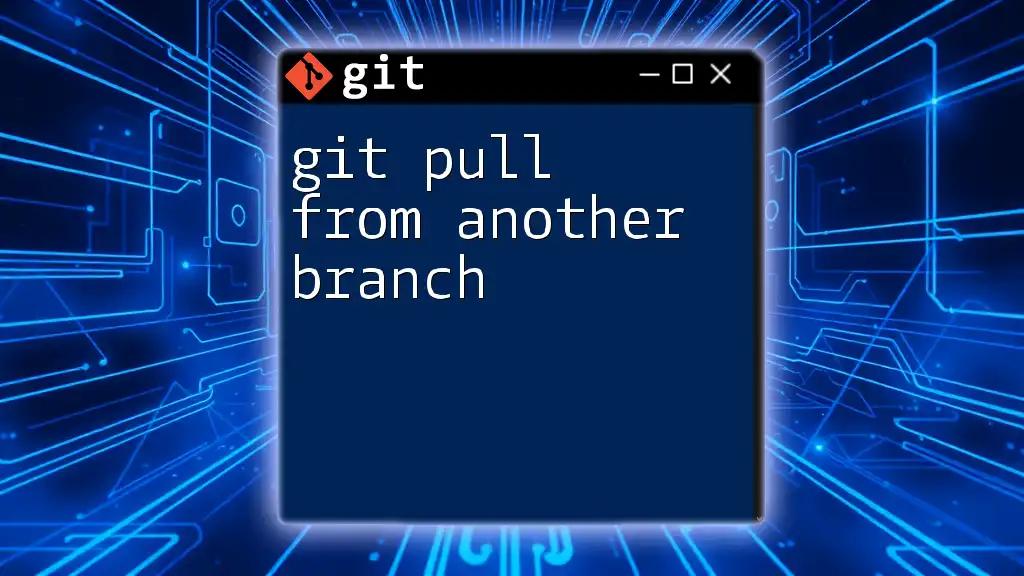
Ensuring a Safe Transition to Another Branch
Checking Your Current Changes
Before switching branches, it's essential to ensure that you have committed your changes. If there are uncommitted changes in your working directory, Git may prevent you from switching branches to avoid potential data loss.
Stashing Changes for Safety
If you have uncommitted changes and still wish to switch branches, you can temporarily store those changes using the `git stash` command.
git stash
This command saves your current changes in a stack and allows you to switch branches without losing your work. After you switch, you can retrieve those changes using:
git stash apply
This command applies your stashed changes back to your working directory, allowing you to pick up where you left off.
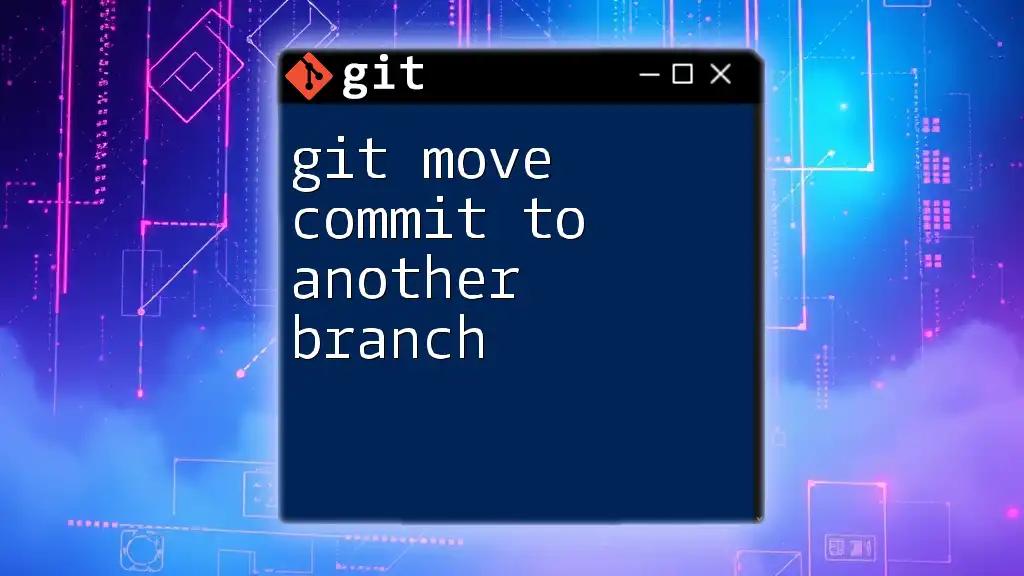
Working with Remote Branches
Fetching Remote Branches
To ensure that you have the latest branches available to your local repository, use the `git fetch` command. This command updates your local references to remote branches without merging any changes.
git fetch origin
Switching to a Remote Branch
When you need to switch to a remote branch that you haven't yet pulled locally, you can create a local branch that tracks the remote branch using:
git checkout -b my-feature-branch origin/my-feature-branch
This command allows you to create a new branch named `my-feature-branch` in your local repository, which tracks the corresponding remote branch.
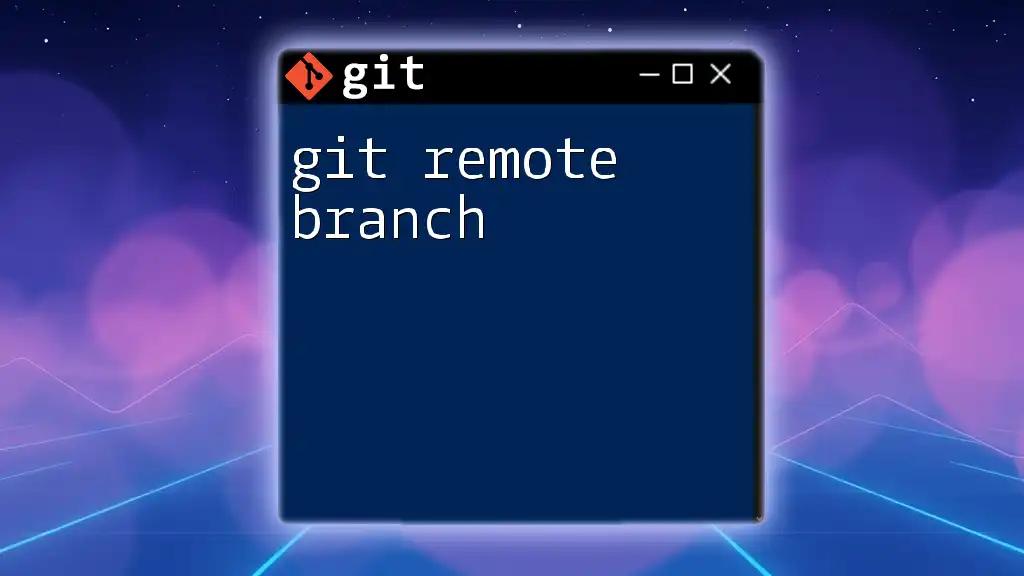
Common Issues When Switching Branches
Uncommitted Changes Warning
Attempting to switch branches with uncommitted changes may result in warnings. If you find yourself unable to switch, it’s advisable to either commit or stash those changes before proceeding.
Merge Conflicts
If you encounter merge conflicts while transitioning between branches, it's crucial to resolve these conflicts. This typically occurs when changes in the target branch overlap with your local changes.
In such cases, carefully review the conflicting files, edit them to resolve differences, and commit the resolution before you can switch branches successfully.
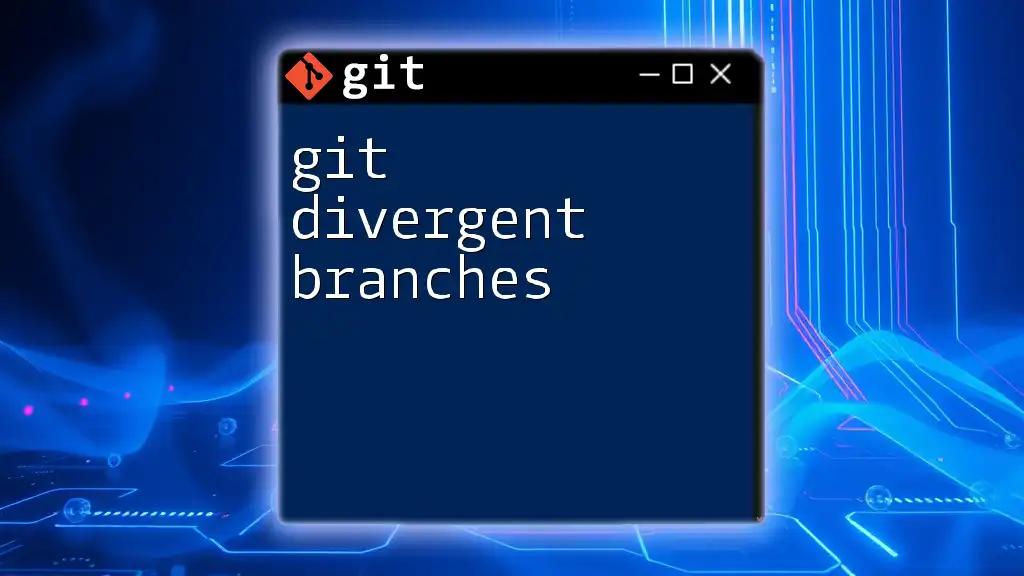
Conclusion
Understanding how to effectively git move to another branch is essential for any developer working in Git. By utilizing commands such as `git checkout` and `git switch`, you can efficiently manage your workflow while keeping your code organized. Branching allows you to tackle features and bugs separately, ensuring a smooth collaborative experience.
Don’t hesitate to put this knowledge into practice in your projects, and enhance your version control skills with Git!
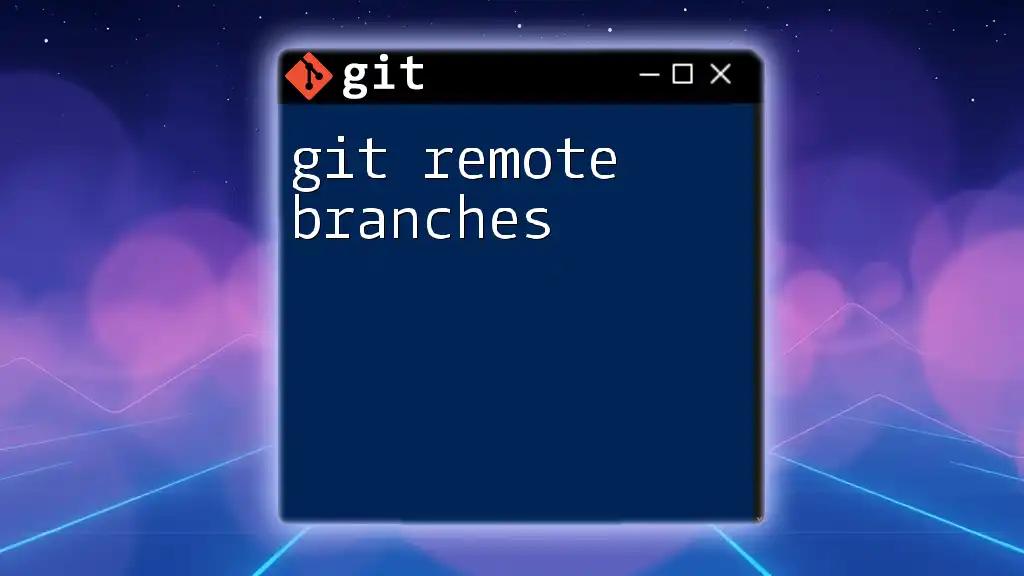
Additional Resources
For further learning, consider exploring the official Git documentation, watching video tutorials that delve deeper into branching strategies, or utilizing cheat sheets for quick command references. These resources can help reinforce your understanding and improve your proficiency with Git.