Git is a distributed version control system that helps manage code changes locally, while GitHub is a cloud-based platform that hosts Git repositories and provides collaboration tools for developers.
Here's a basic example of initializing a Git repository:
git init my-repo
Understanding Git
What is Version Control?
Version control is a system that allows developers to keep track of changes made to files over time. It serves several key purposes:
- History Tracking: Version control keeps a detailed history of changes, enabling easy access to previous versions of code.
- Collaboration: Teams can work on the same codebase simultaneously without overwriting each other's work.
- Backup: If errors are made, the ability to revert to previous versions provides a safety net.
Core Features of Git
Git is a distributed version control system that offers a variety of features that improve the development workflow:
-
Distributed Architecture: Unlike centralized version control systems, every developer has a full copy of the repository, which promotes collaboration and redundancy.
-
Branching and Merging: Git allows users to create separate branches for individual features or fixes. Changes can be merged back into the main branch once they are complete and tested, providing a clean and manageable codebase.
-
Staging Area: Git introduces the concept of a staging area that allows developers to prepare changes before committing them. This ensures that only the intended changes are recorded.
-
Local and Remote Repositories: Developers can work locally and sync their changes with a remote repository whenever necessary. This decoupling of local and remote work enhances flexibility.
Basic Git Commands
Getting started with Git involves understanding some fundamental commands. These commands form the basis for managing a Git repository and include:
- `git init`: Initializes a new Git repository in the current directory.
- `git add`: Stages changes for the next commit.
- `git commit`: Records changes to the local repository with a message describing the changes.
- `git branch`: Manages branches in your repository, allowing you to create, delete, or view branches.
- `git merge`: Combines changes from one branch into another.
Here are some code examples to illustrate these commands:
git init
git add README.md
git commit -m "Initial commit"
git branch feature-branch
git merge feature-branch
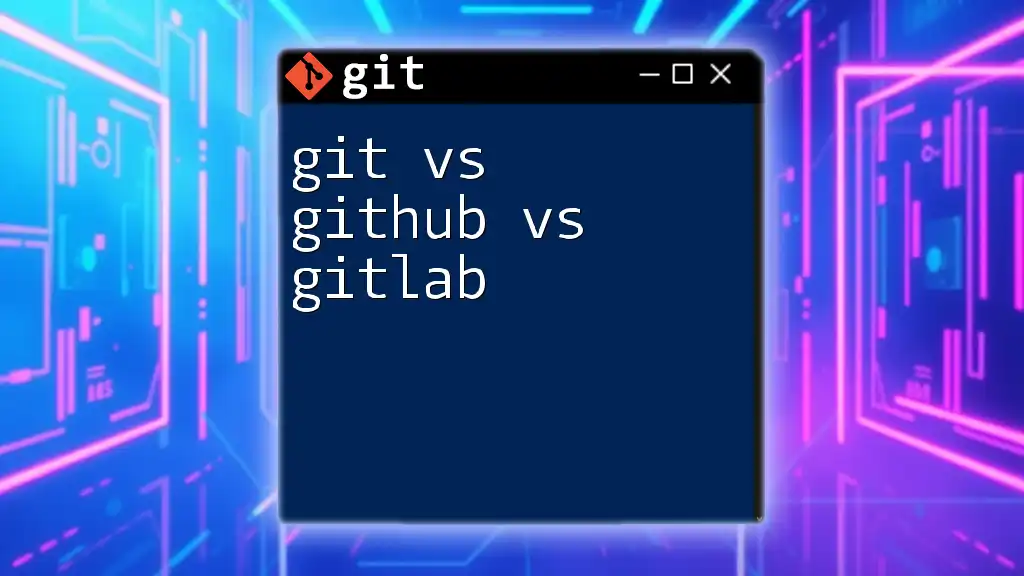
Understanding GitHub
What is GitHub?
GitHub is an online platform built around Git that provides remote hosting, collaboration tools, and social networking features. It is widely used by developers to share and collaborate on projects.
How GitHub Works with Git
GitHub enhances the functionality of Git by introducing a user-friendly interface and numerous collaborative features. When you interact with GitHub, you work with remote repositories. Here are some important commands for managing interactions with GitHub:
- `git clone`: Creates a local copy of a remote repository.
- `git push`: Uploads local changes to a remote repository.
- `git pull`: Fetches and integrates changes from a remote repository into your local branch.
Example code snippets for interacting with GitHub include:
git clone https://github.com/username/repo.git
git push origin main
git pull origin main
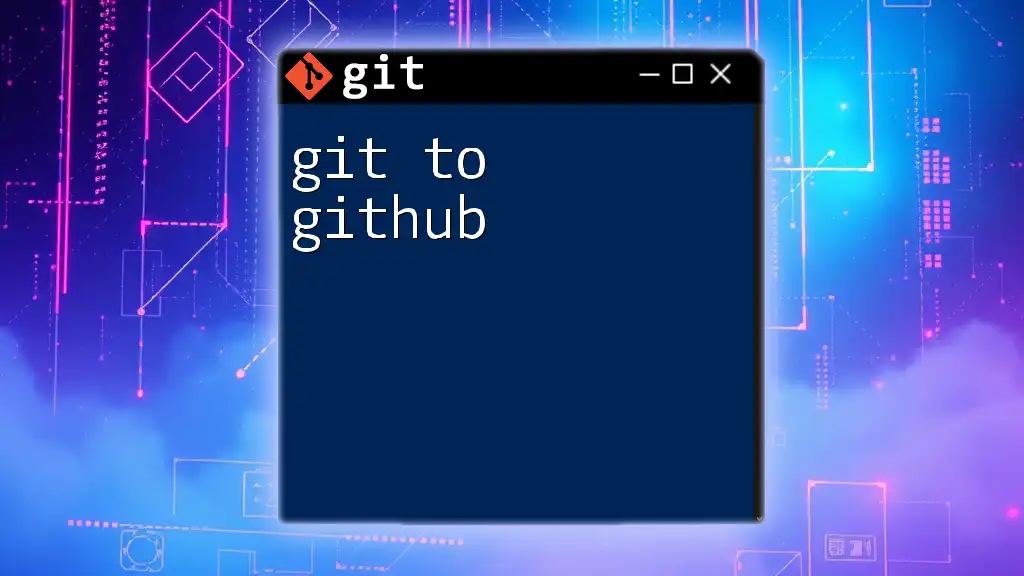
Key Differences Between Git and GitHub
Nature of Tools
Git serves as a command-line tool for version control. It allows you to track changes in your projects locally but lacks any integrated environment for collaboration. On the other hand, GitHub is a web-based platform designed to facilitate teamwork, code sharing, and repository management.
How They Interact
Git and GitHub work together seamlessly. You use Git to manage files locally, while GitHub helps you upload, share, and manage that work in a collaborative setting. Connecting locally developed projects to remote repositories is essential for teamwork, and understanding this workflow is crucial for modern software development.
Use Cases
Using Git alone is ideal for personal projects where collaboration is unnecessary, while GitHub shines in scenarios requiring multiple contributors. For instance, you might use Git to develop a feature alone, but once you're ready to share your work, you can push it to GitHub to collaborate with others.
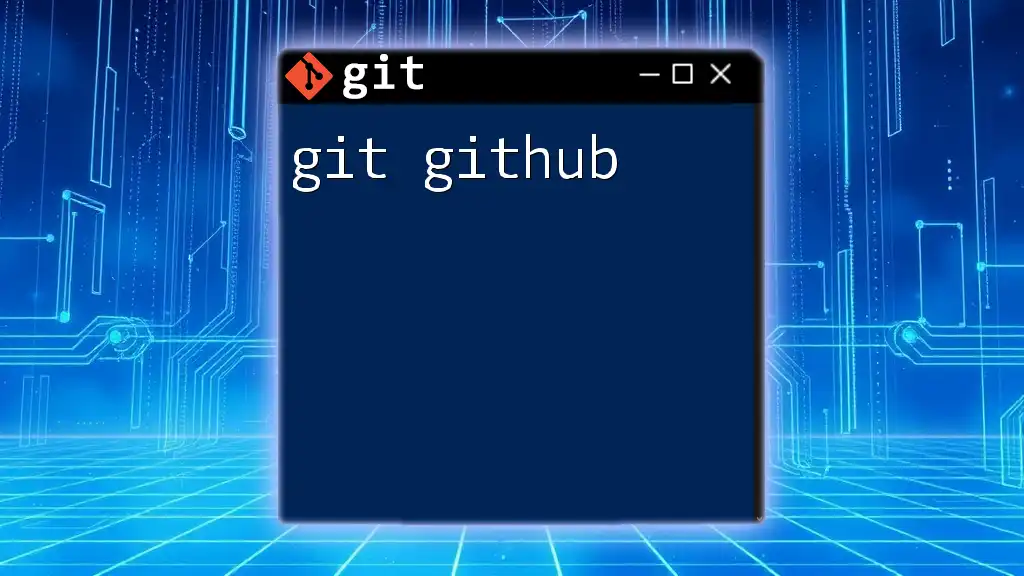
When to Use Git Alone
Local Development Scenarios
Git is sufficient for local development, especially when you are experimenting or working on personal projects. You can create branches to test new ideas without affecting the main codebase. Here’s an example of how you might manage branches locally:
git checkout -b experimental-feature
git commit -m "Experiment with new logic"
By using Git alone in such scenarios, you can organize your code and track changes effectively while maintaining control over your workflows.
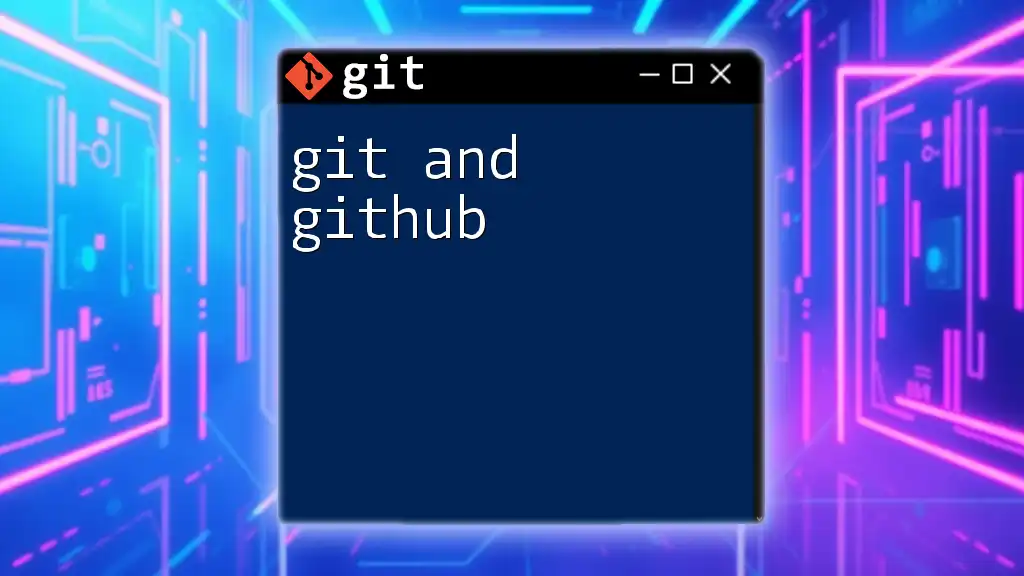
When to Use GitHub
Collaboration and Sharing
GitHub is the go-to solution when it comes to working with multiple developers. It offers a set of tools that allow teams to collaborate effectively. For example, you can create pull requests to propose changes to a project, initiate discussions, and undergo code review.
Public vs Private Repositories
GitHub offers both public and private repository options, catering to various needs in project development. Public repositories allow anyone to see your code, promoting open-source projects and community engagement. In contrast, private repositories keep your work confidential, which is beneficial for proprietary projects.
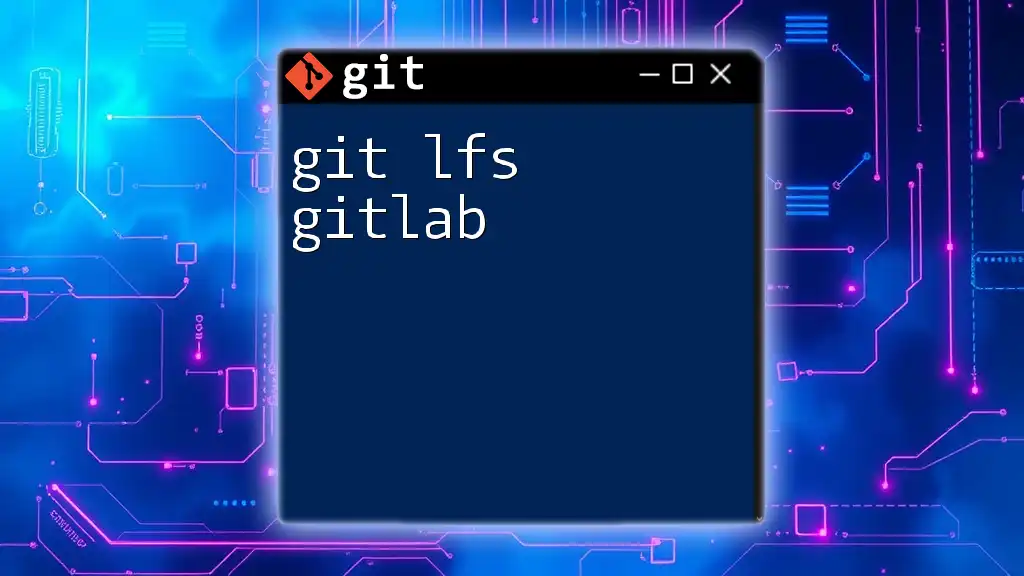
Common Misconceptions
Git vs GitHub Misunderstandings
It is crucial to clarify that Git and GitHub are separate entities. Git is a version control tool that you can use independently, whereas GitHub is a service that provides an environment to host and share Git repositories. Many people mistakenly believe that Git is tied exclusively to GitHub, which is not the case; Git can also work with other platforms such as Bitbucket and GitLab.
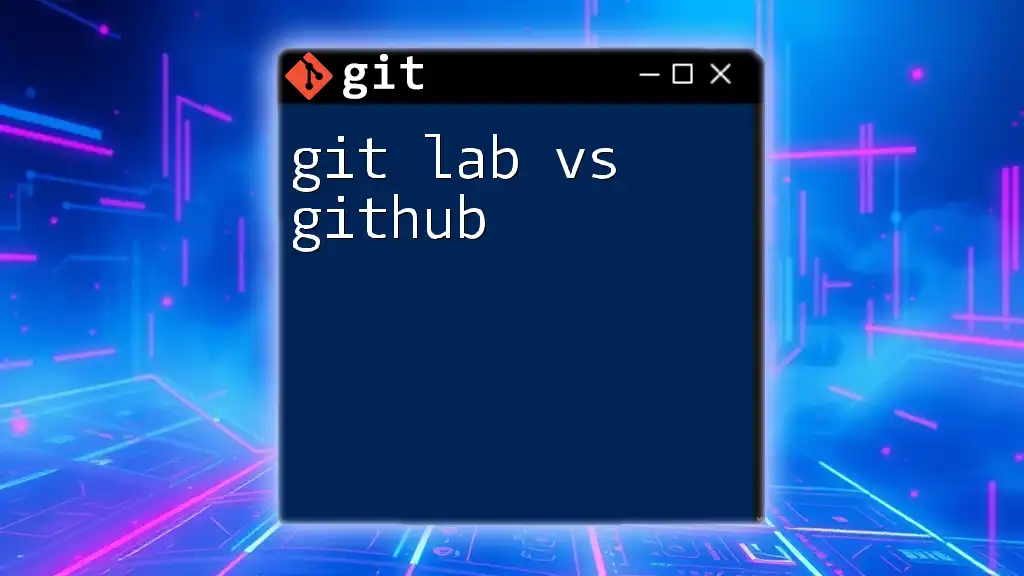
Conclusion
Understanding the key differences between Git and GitHub is essential for anyone stepping into the world of software development. While Git serves as a powerful version control tool for tracking changes, GitHub enhances collaboration and sharing among developers. Familiarizing yourself with both tools will vastly improve your workflow and teamwork ability in coding projects.
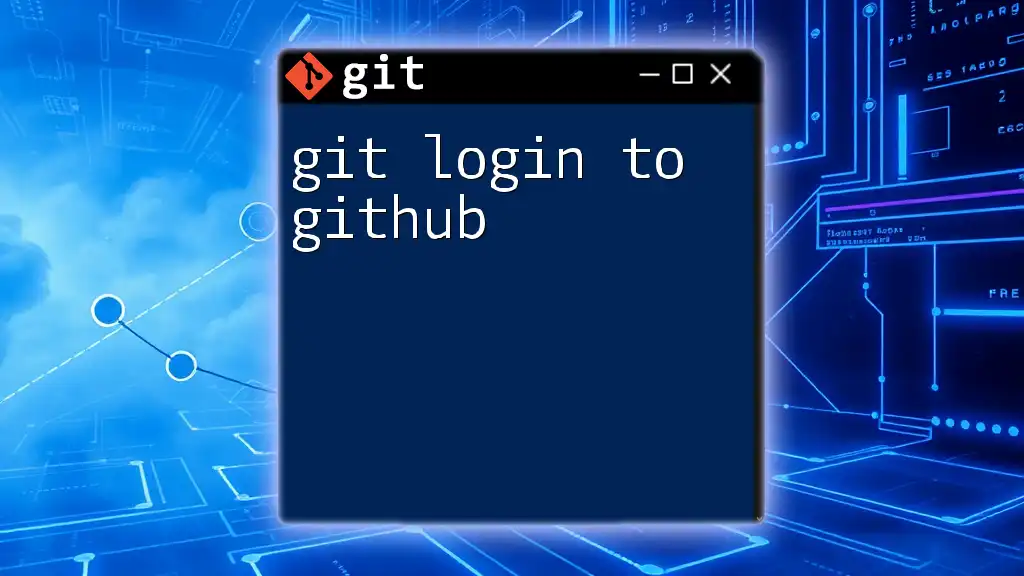
Additional Resources
To further expand your understanding of Git and GitHub, consider exploring official documentation and tutorials available online.
Tools and Integrations
Additionally, tools like GitHub Desktop and GitKraken can facilitate your experience with Git and GitHub, providing a more visual approach to version control and repository management.