The `git switch` command is a simplified way to switch branches in a Git repository.
git switch <branch-name>
Understanding Branches in Git
The Role of Branches
Branches are a fundamental concept in Git, allowing developers to create independent lines of development. This functionality is crucial for various aspects of software development, including feature development, experimentation, and collaboration. By using branches, developers can isolate changes, making it easier to experiment with new features or fix bugs without affecting the main codebase.
How Branching Works in Git
In Git, every branch is essentially a pointer to a specific commit in the history of a repository. When you create a new branch, Git does not create a complete copy of your project; instead, it creates a new pointer that you can move forward or backward through changes. This lightweight nature of branches enables quick context switching and minimizes the overhead associated with traditional version control systems.
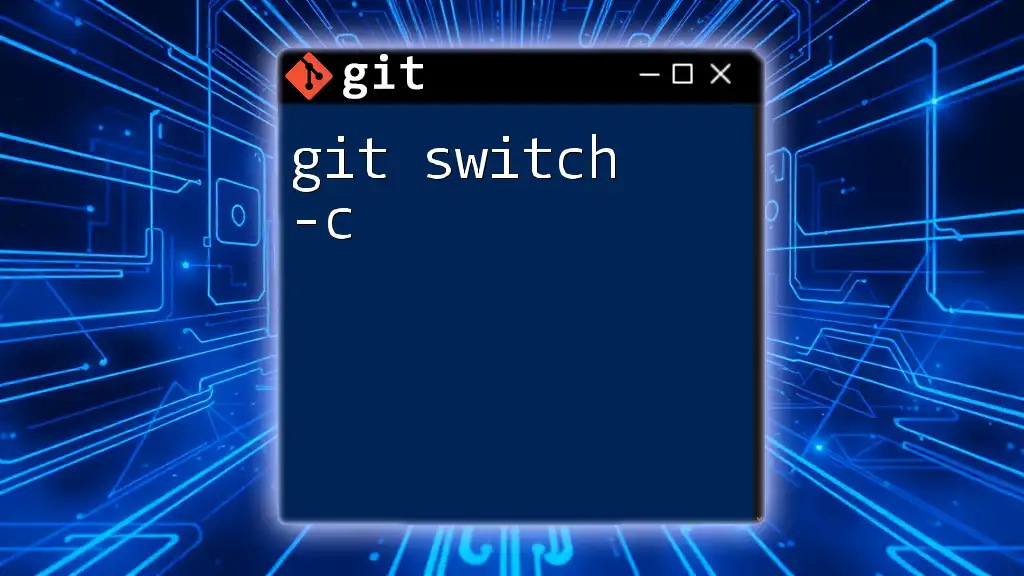
Getting Started with `git switch`
Basic Syntax and Usage
The `git switch` command was introduced to simplify the process of switching between branches and creating new ones. The basic syntax for switching to an existing branch is:
git switch <branch-name>
When you run this command, Git performs the following actions:
- Updates the working directory to match the state of the target branch.
- Moves the HEAD pointer to the specified branch.
Examples of Basic Usage
To switch to an existing branch, you would use:
git switch feature-branch
After executing the command, your working directory will reflect the state of `feature-branch`.
If you want to switch back to the main branch (often named `main` or `master`), you can run:
git switch main
This action is straightforward yet powerful, allowing for seamless transitions between different areas of your project.
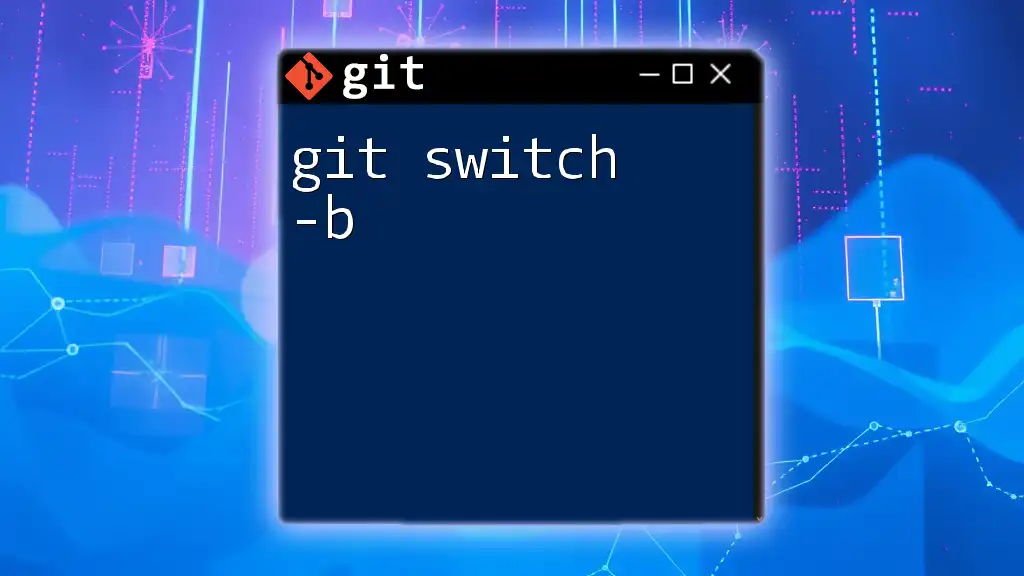
Common Scenarios for Using `git switch`
Switching Between Branches
One of the most common use cases for `git switch` is switching between branches. Developers frequently navigate between branches as they work on different features or address various issues. It's essential to ensure that you are working with the latest commits of each branch, and using `git switch` makes this process intuitive and efficient.
Creating a New Branch and Switching to It
The `-b` option allows you to create a new branch and switch to it in a single command. This method is particularly useful when starting a new feature or bug fix. For example, you could create a new branch called `new-feature` and switch to it using:
git switch -b new-feature
This command encapsulates both creating the branch and checking it out, streamlining your workflow and reducing the number of steps needed to get started on new work.
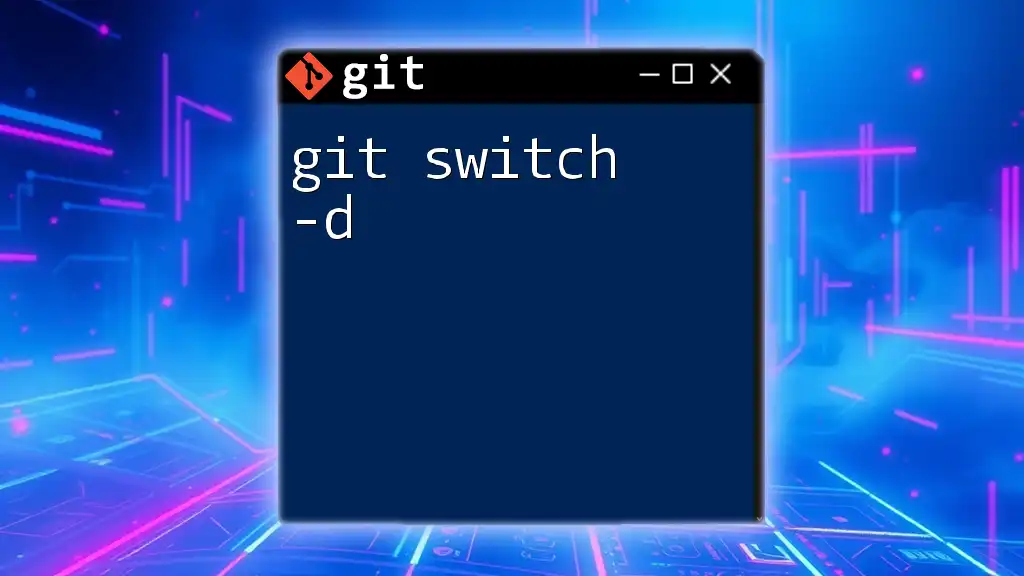
Advanced Usage of `git switch`
Switching with Uncommitted Changes
When working in Git, you may have uncommitted changes in your working directory. The `git switch` command can help you manage this situation effectively. By default, Git will not allow you to switch branches if doing so would lose uncommitted changes. However, if you're certain that you want to discard those changes, you can use the `--discard-changes` option:
git switch --discard-changes feature-branch
This command will switch to the specified branch and discard any uncommitted changes in your current working directory. Caution: This action is irreversible, so ensure that you truly wish to discard those changes.
Switching Using the `--merge` Option
In some cases, you might want to switch branches while merging uncommitted changes from your current branch into the target branch. You can achieve this by using the `--merge` option:
git switch --merge <branch-name>
This command enables you to transition to another branch while integrating your current changes. It's particularly useful when you want to work on a related task without losing unsaved progress.
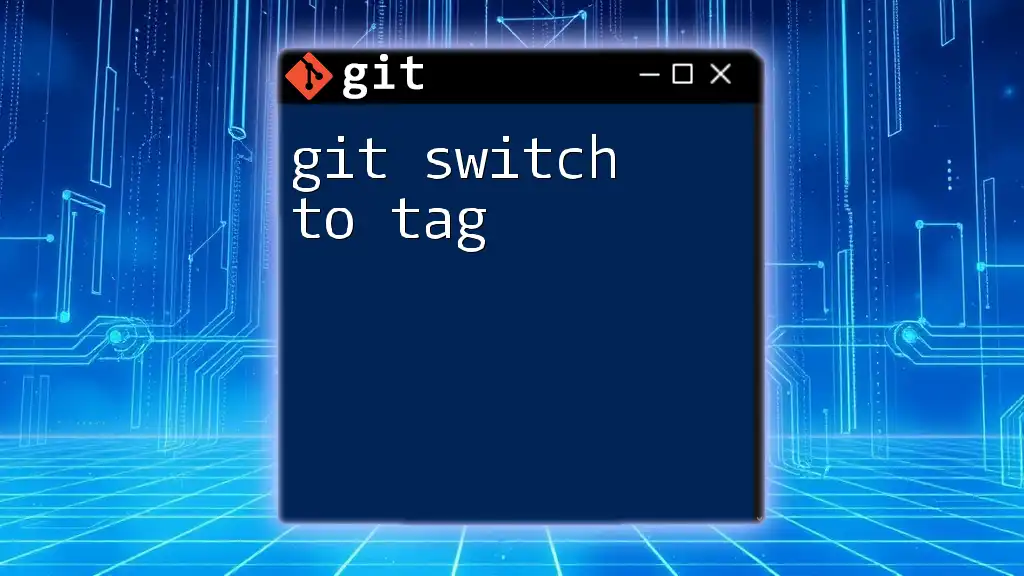
Troubleshooting Common Issues with `git switch`
Conflicts When Switching Branches
While switching branches, you may encounter conflicts if uncommitted changes conflict with the target branch. These conflicts often arise when two branches modify the same line of code in different ways. To resolve conflicts when they arise:
- Review the messages from Git to understand which files are in conflict.
- Use visual tools or command-line editors to merge changes carefully.
- After resolving the conflicts, remember to commit your changes.
Dealing with Detached HEAD States
A detached HEAD state occurs when you check out a specific commit directly rather than a branch. In this situation, if you create new commits, they will not belong to any branch, making them hard to reference later. You can recover from a detached HEAD state using the `git switch` command. To restore your previous branch:
git switch main
This command redirects you back to the main branch, preserving your work associated with it. Always be proactive to avoid losing commits made during a detached state by following a standard workflow.
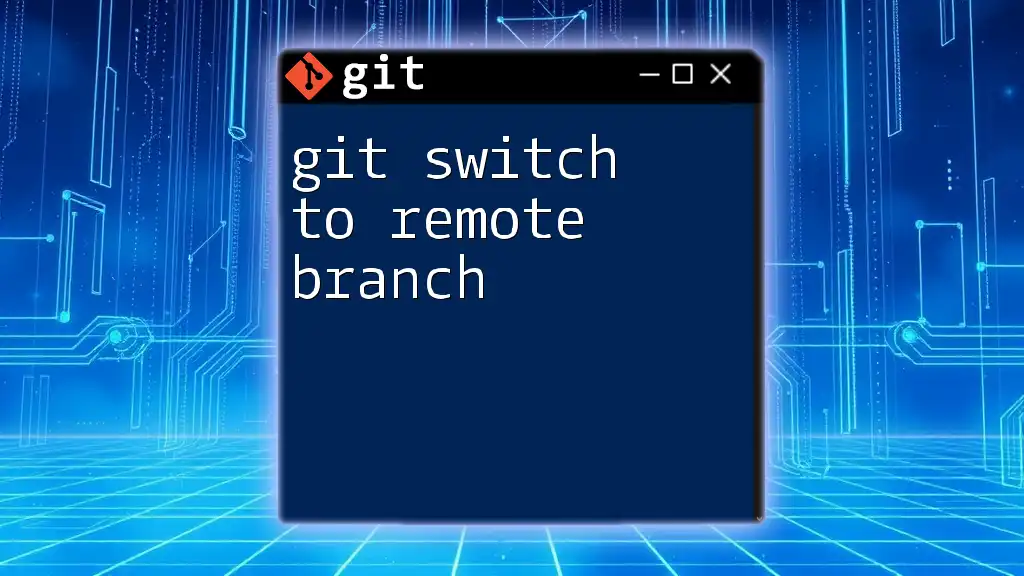
Best Practices for Using `git switch`
Know Your Branches
Being aware of your existing branches is essential for efficient branching. Use the `git branch` command to list all available branches in your repository. This understanding will help you choose the correct branch to switch to and ensure you are working with the necessary features.
Frequent Committing and Switching
To keep your branches organized and maintain a clean working directory, it’s recommended to commit your work before switching branches. Regular commits not only preserve your progress but also reflect the history of your contributions. The shift between branches becomes smoother when you do not have uncommitted changes.
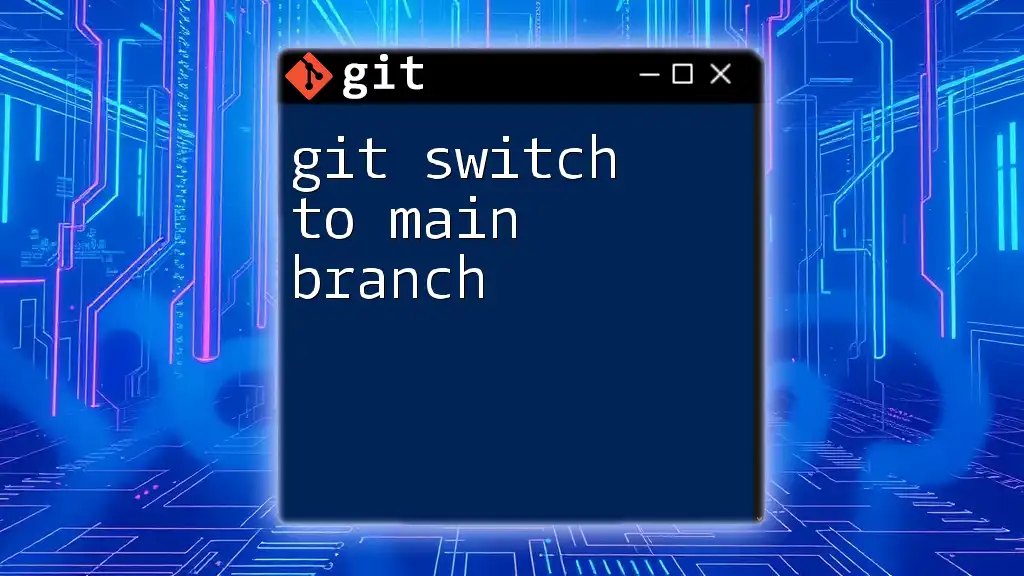
Conclusion
In this guide, we've explored the powerful `git switch` command, which steers developers towards efficient branch management. Mastery of this command enables smooth transitions between various stages of your projects and enhances collaborative development efforts.
Call to Action
I encourage you to practice using `git switch` regularly in your projects. Familiarizing yourself with branching and how to manipulate it effectively will undoubtedly enhance your development skills.
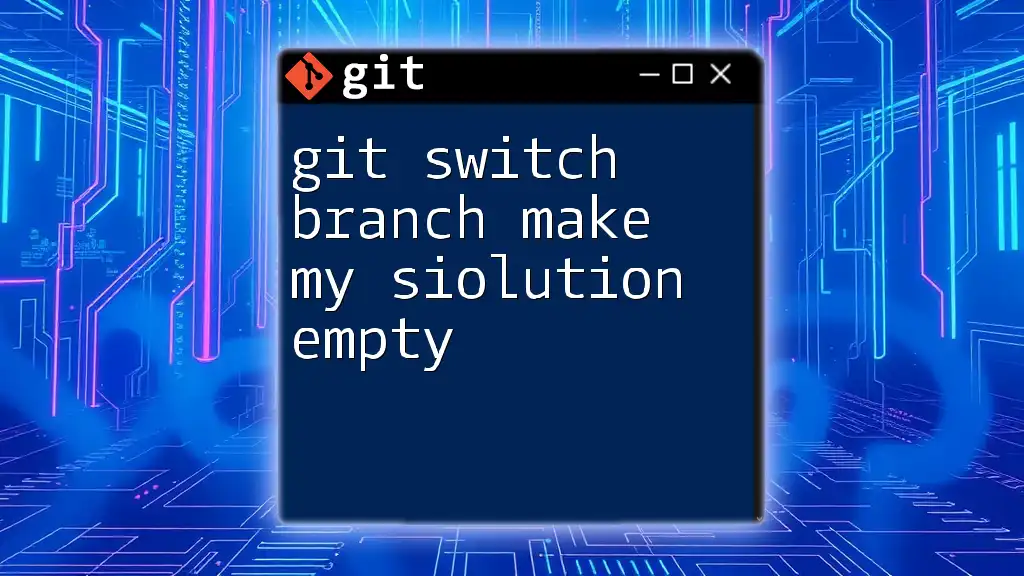
Additional Resources
Links to Further Reading
Look for articles and tutorials specializing in Git branching strategies and advanced Git commands.
Community and Support
Don't hesitate to seek assistance from Git communities, forums, or platforms like Stack Overflow whenever you encounter challenges. Engaging with others can provide valuable insights and solutions.