The `git status` command displays the current state of the working directory and staging area, showing which changes have been staged, which are not, and which files aren't being tracked by Git.
git status
What is `git status`?
The command `git status` plays a fundamental role in Git's workflow. It allows you to view the state of your repository at any given point in time. Understanding what this command does is essential for effective version control, as it provides insights into the current state of your working directory, staging area, and changes to commit.
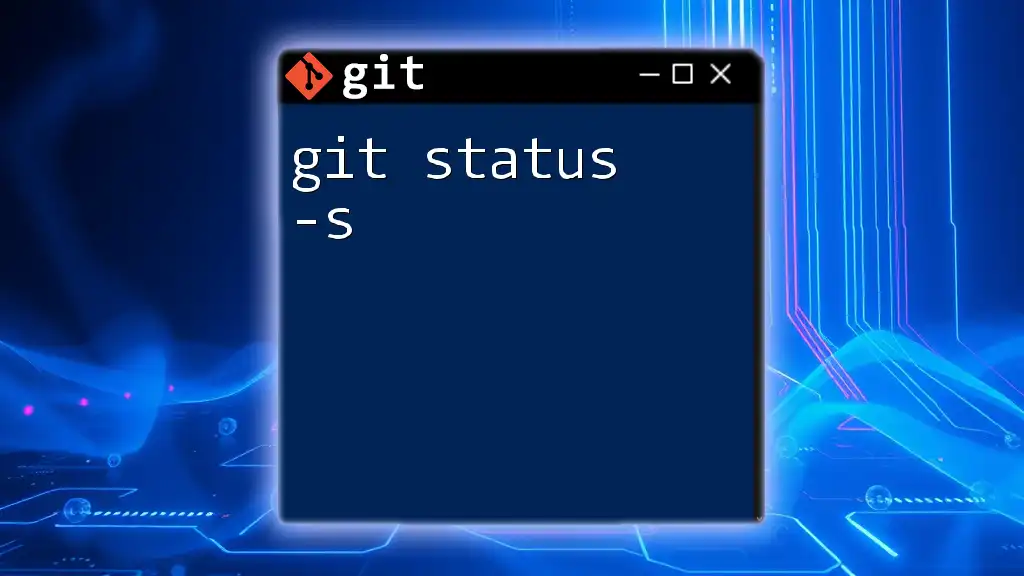
How to Use `git status`
The basic syntax to run the command is straightforward: simply type `git status` in your terminal. Upon invoking this command, Git provides a summary of the current state of your repository, informing you of any modified files, staged changes, and untracked files.
Example
To run the command, you can simply enter:
git status
The output you receive will depend on the current state of your repository.
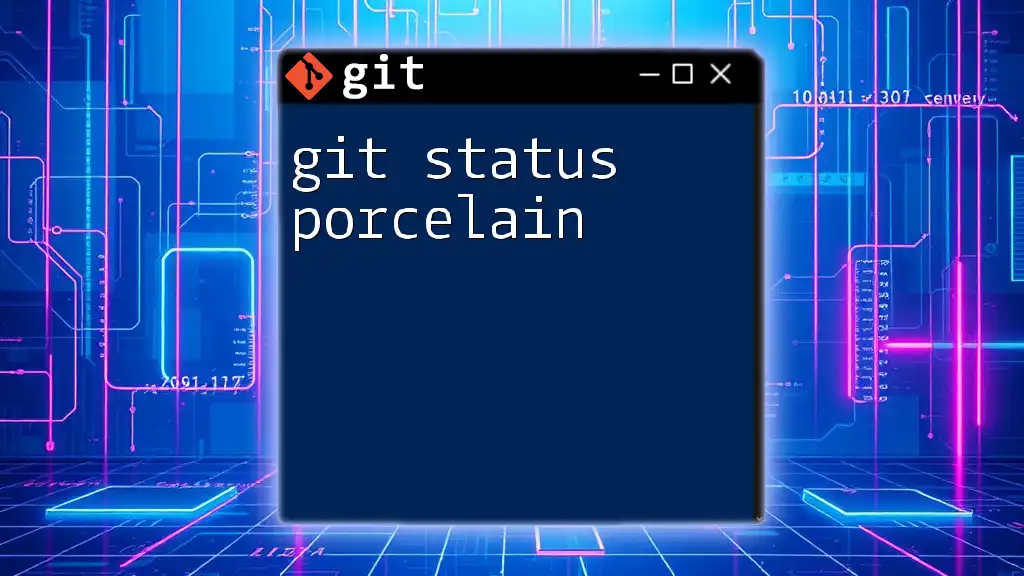
Understanding the Output
When you execute `git status`, you will encounter various sections in its output. Each section provides critical information about different aspects of your version control status.
The Working Tree
The working tree is where you make changes to your files. It represents the files you are currently working on in your repository. Modified Files indicate any files that have been changed but are not yet staged for a commit.
For instance, if you modify a file named `example.txt`, running `git status` will display this file under the section stating it has been modified.
Example of Modified Files
When you run `git status` after changing `example.txt`, you might see:
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
modified: example.txt
This output signifies that `example.txt` has been changed but is not ready to be committed.
Staging Area
The staging area is where you prepare changes to be committed. Once you have modified files and wish to include them in your next commit, you must stage them. You can stage files using the `git add` command.
Staged Files are changes that you have added to the staging area. Once files are staged, they will not appear as modified when you run `git status`.
Example of Staging Files
To stage `example.txt`, you would use:
git add example.txt
After staging, a subsequent `git status` command will output:
Changes to be committed:
(use "git reset HEAD <file>..." to unstage)
modified: example.txt
This indicates that `example.txt` is now staged and ready for committing.
Untracked Files
Untracked files are those that Git has not yet been introduced to. These could be new files you have created in the project that haven’t been added to version control.
Managing untracked files is crucial. They will show up when you run `git status` as untracked and separated from modifications.
To ignore certain untracked files, you can create a `.gitignore` file. This instructs Git to ignore specified file types or specific files entirely.
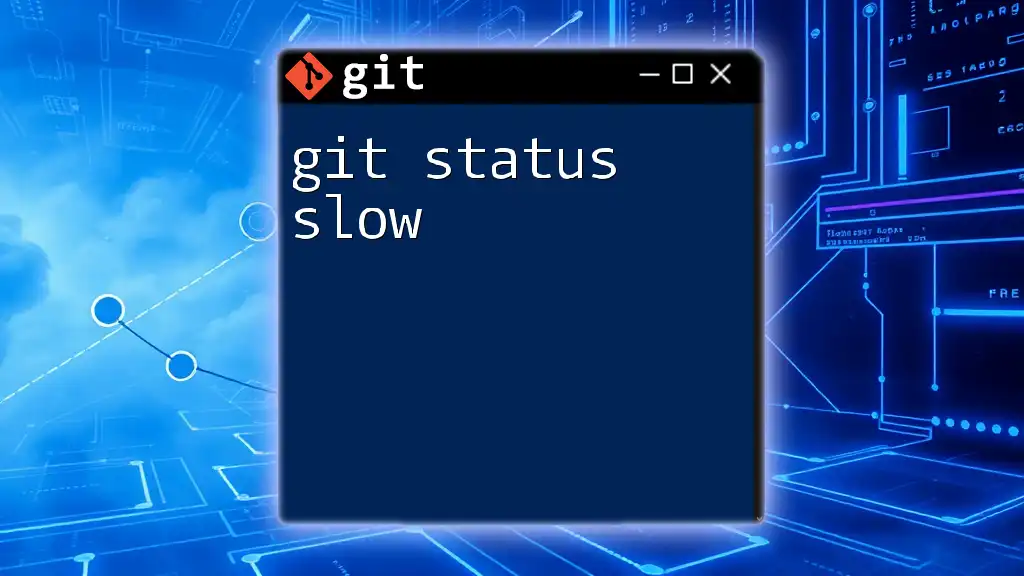
Common Scenarios
After Modifying Files
When you modify one or more files and run `git status`, the command will inform you of any changed files that have not yet been staged.
Example Output
If you modified both `example.txt` and `another_file.txt`, the output of `git status` will indicate:
Changes not staged for commit:
modified: example.txt
modified: another_file.txt
After Staging Changes
Once changes are staged, you can confirm they are in the staging area by re-running `git status`. The output will show these files as ready for commit.
Example Output with Staged Changes
git add example.txt
git status
Expected output:
Changes to be committed:
modified: example.txt
After Committing Changes
Once you have committed staged changes, running `git status` will clarify that there is nothing new to commit.
Example Output After Commit
After executing:
git commit -m "Update example.txt"
git status
You might see:
On branch main
nothing to commit, working tree clean
This indicates that your latest changes have been committed and your working directory is up to date.
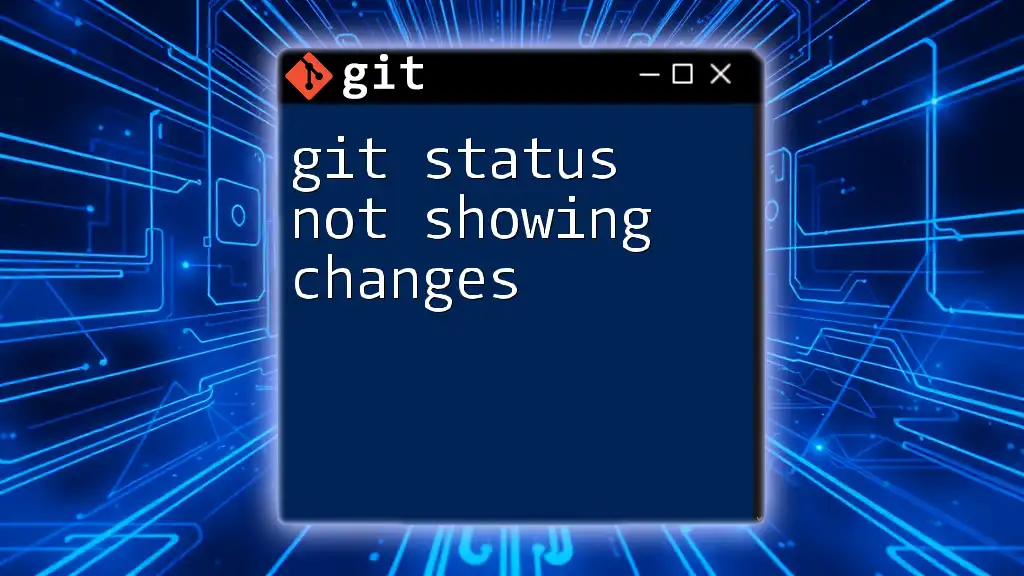
Combining `git status` with Other Git Commands
Using `git status` with `git add`
In common workflows, using `git status` alongside `git add` streamlines the process. After modifying files, you can check their status, and then stage them as needed.
Example of a Common Pattern
git status
git add <file>
git status
This sequence shows modifications, allows you to stage, and then confirm staging.
Using `git status` before a Commit
Running `git status` right before a commit is a best practice. It allows you to verify which changes are staged and which remain untracked or modified.
Example Scenario
git add example.txt
git status
Running this ensures that the output clearly indicates what will be committed before you officially commit the changes.
Checking Branch Status
`git status` also reveals the branch you are currently working on. This is crucial when managing multiple branches in a collaborative environment.
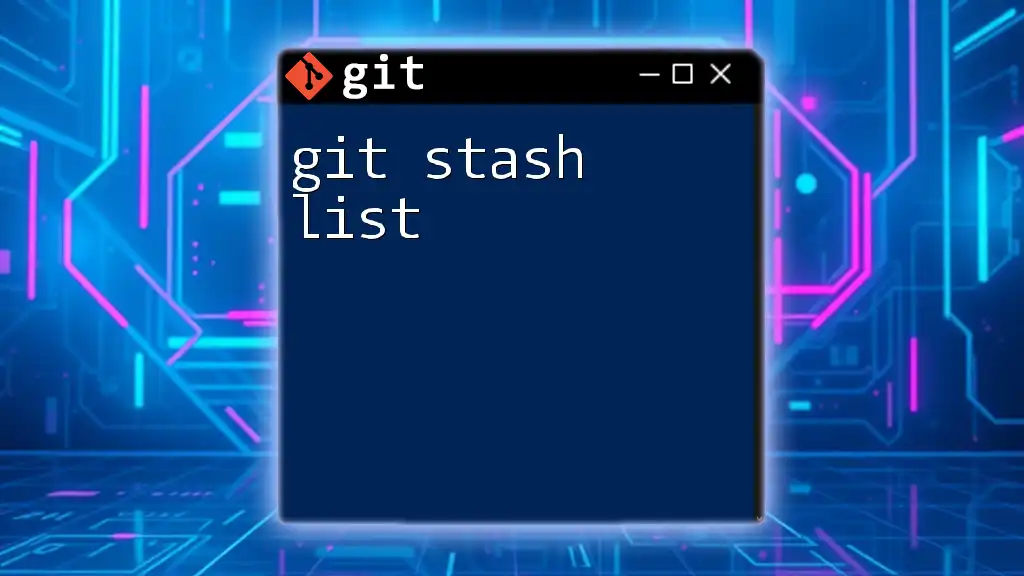
Best Practices
- Regularly check the status of your repository as you work. This helps you stay aware of modifications and staging.
- Utilize aliases for commands you frequently use. For instance, you can create a shortcut for `git status`:
git config --global alias.s status
Now, you can simply use `git s` to check the status.
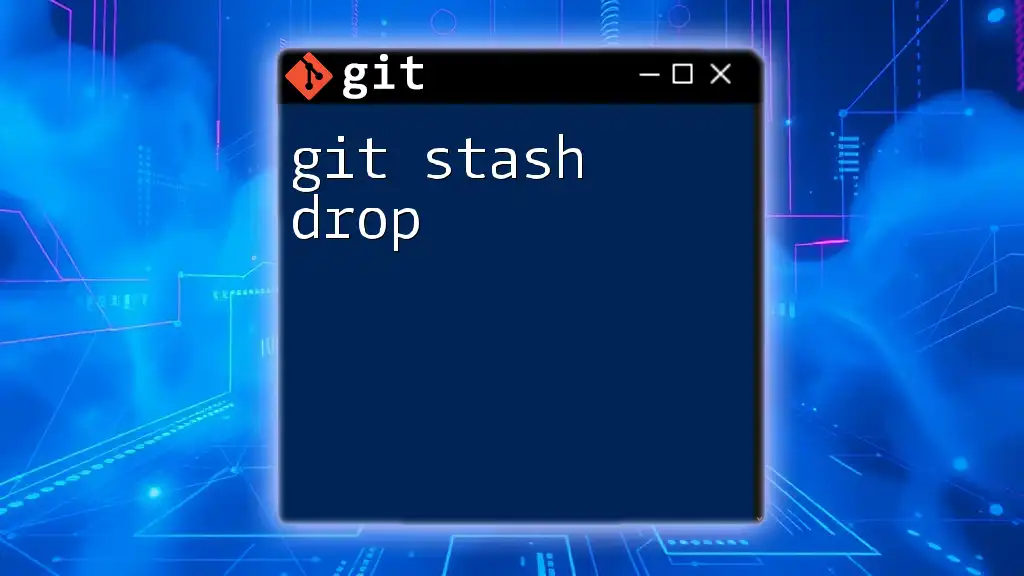
Conclusion
In summary, mastering the `git status` command is vital for anyone working with Git. This simple yet powerful command provides essential insights into the state of your repository, helping you navigate the complexities of version control. Regular use of `git status` will lead to better practices, clearer understanding, and enhanced efficiency in your development process. Whether you're new to Git or looking to refine your skills, incorporating `git status` into your routine will prove invaluable.
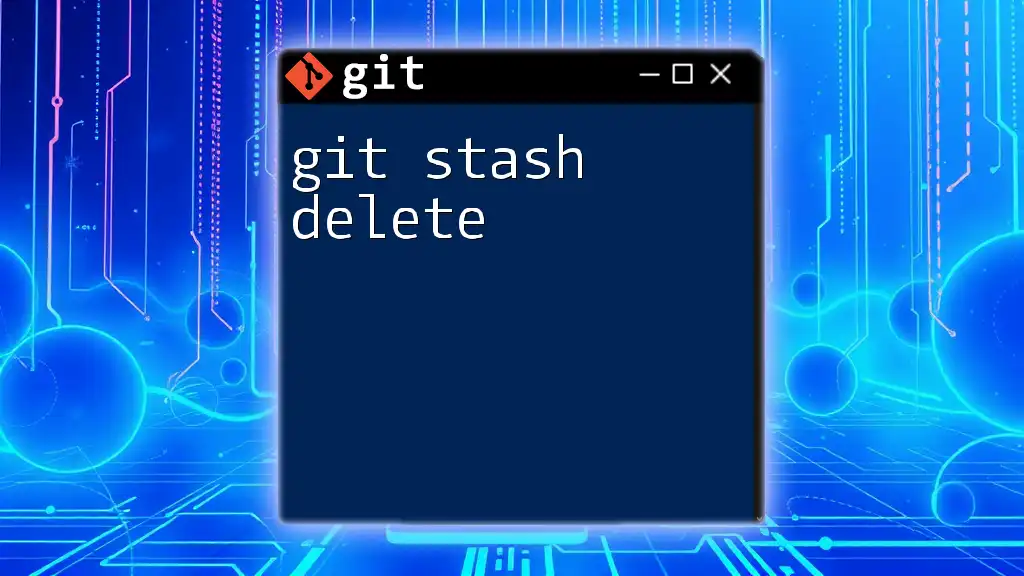
Additional Resources
For continued learning, refer to the official Git documentation for in-depth details and advanced concepts. Explore recommended books and online courses to broaden your understanding of Git and version control systems.