If `git status` is not showing changes, it may indicate that you've either staged the changes (which could be viewed with `git diff --cached`), or you're in a directory that is not under version control.
Here's how you can check staged changes:
git diff --cached
Understanding `git status`
What is `git status`?
The `git status` command is fundamental in Git's version control system. It serves as a snapshot of your project's state, helping you identify changes in your working directory and staging area. When you run `git status`, the output informs you of three main aspects:
- What files have been modified (but not staged).
- What files are staged and ready to be committed.
- What untracked files exist in the directory.
By comprehensively understanding this command, you can effectively manage your changes and avoid confusion during your workflow.
Common Outputs of `git status`
When you execute `git status`, Git will categorize the files in different states:
- Modified: Files that have been changed but not yet staged.
- Staged: Files that are ready to be included in your next commit.
- Untracked: New files that Git is not currently tracking.
Understanding these outputs is crucial for effective version control and ensuring that no changes go unnoticed.
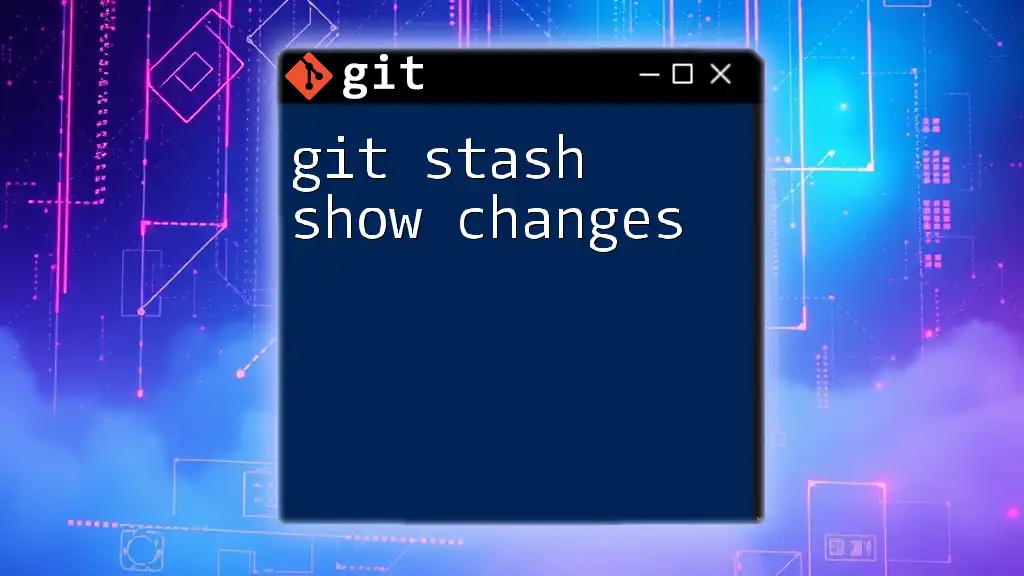
Reasons Why `git status` Might Not Show Changes
Files Not Saved
One of the most common reasons for `git status not showing changes` is the failure to save changes in your text editor. If you've modified a file but don't save it, Git will not recognize these modifications. Always ensure that your changes are saved before checking the status.
Example: If you're working on a file called `example.py`, make sure to save the file in your editor before running:
git status
Changes in Ignored Files
Files specified in your `.gitignore` file will not be tracked by Git, and as a result, they won't appear in the output of `git status`. The `.gitignore` file tells Git which files or directories to ignore, generally those that should not be included in the version control (like log files or temporary files).
Example: Consider a `.gitignore` that looks like this:
*.log
temp/
In this case, any file ending in `.log`, as well as the entire `temp` directory, won't show any output from `git status`. Therefore, ensure you check the contents of your `.gitignore` if you suspect changes are not appearing.
Incorrect Repository Path
Running `git status` in a directory that is not initialized as a Git repository will result in no information regarding changes. If you're unsure of your current directory, you can use the command:
pwd
This will print the working directory. Always verify that you are within the intended Git repository.
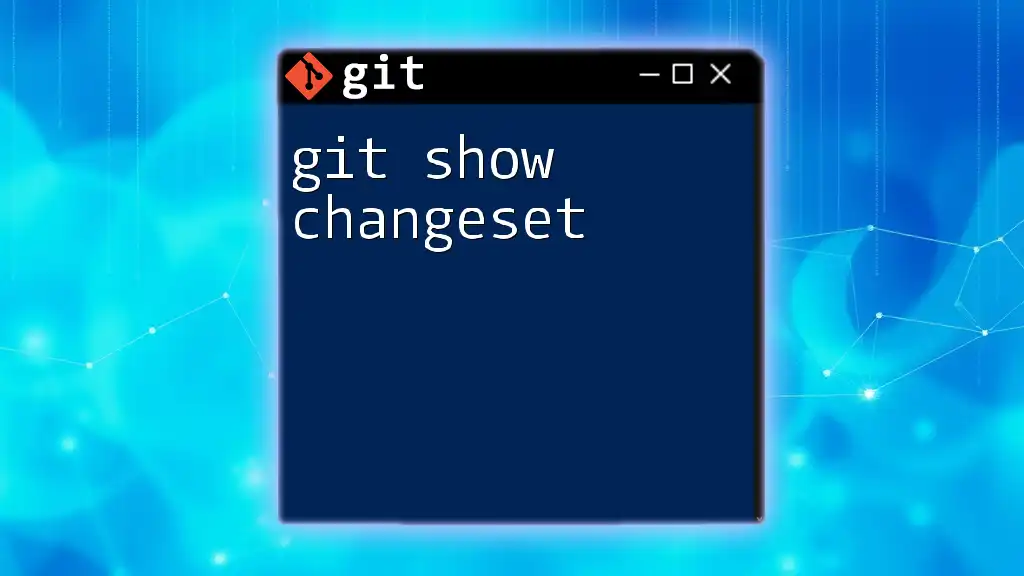
Troubleshooting Steps
Step 1: Ensure Files Are Saved
If you suspect that `git status` is not reflecting your recent changes, the first step is to verify that you have indeed saved the latest version of your files in your text editor.
Step 2: Verify `.gitignore` Settings
Next, check your `.gitignore` file. Display its contents to confirm if any unintended files are being ignored. You can view the ignored files with the following command:
git check-ignore -v *
This command will help you identify which files are ignored and why.
Step 3: Check the Current Directory
As mentioned, ensuring you're in the correct directory is essential. If you navigate to another directory that is not a part of your repository, `git status` will show no relevant information. Always use `pwd` to verify your current location.
Step 4: Stash or Commit Changes
If you have local changes but want to temporarily set them aside or commit them, you can use `git stash` or `git commit`. Using `git stash` will save your changes temporarily while allowing you to work on something else:
git stash
Alternatively, if you are ready to save your progress, commit the changes with:
git commit -m "Your commit message"
This will move your staged changes to your repository, making them part of the project’s history.
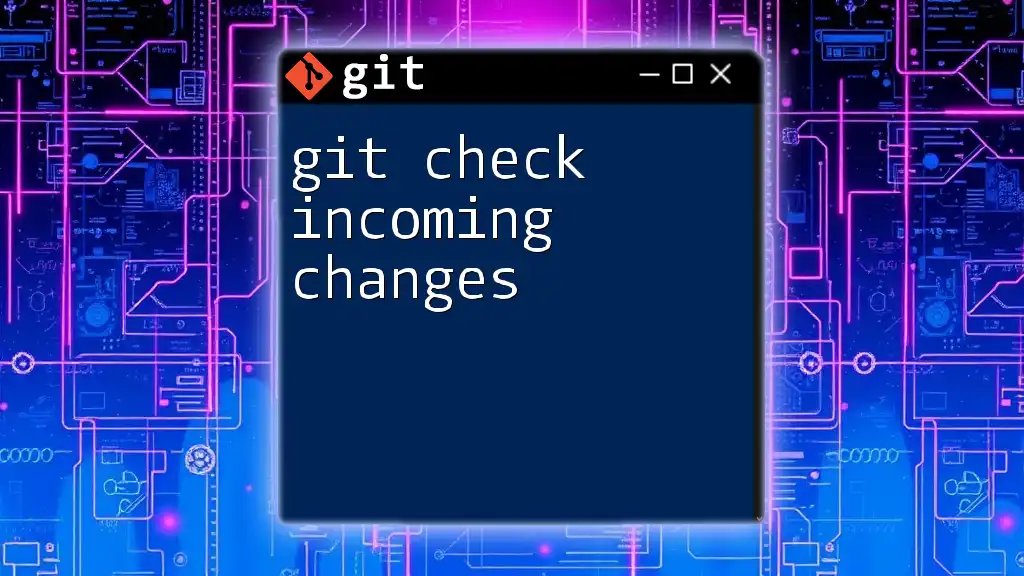
Additional Considerations
Working with Submodules
If your repository contains submodules, they may complicate your understanding of `git status`. Changes made within submodules will not be reflected in the main repository’s status. You will need to navigate to the submodule directory and run `git status` there to see its state.
Permissions and Access Issues
File permission errors can sometimes lead to confusion when using `git status`. If Git cannot read the file due to permission restrictions, it may not display expected outputs. You can check permissions with:
ls -l filename
This command will help you identify any permission-related issues that might be causing your changes to go unnoticed.
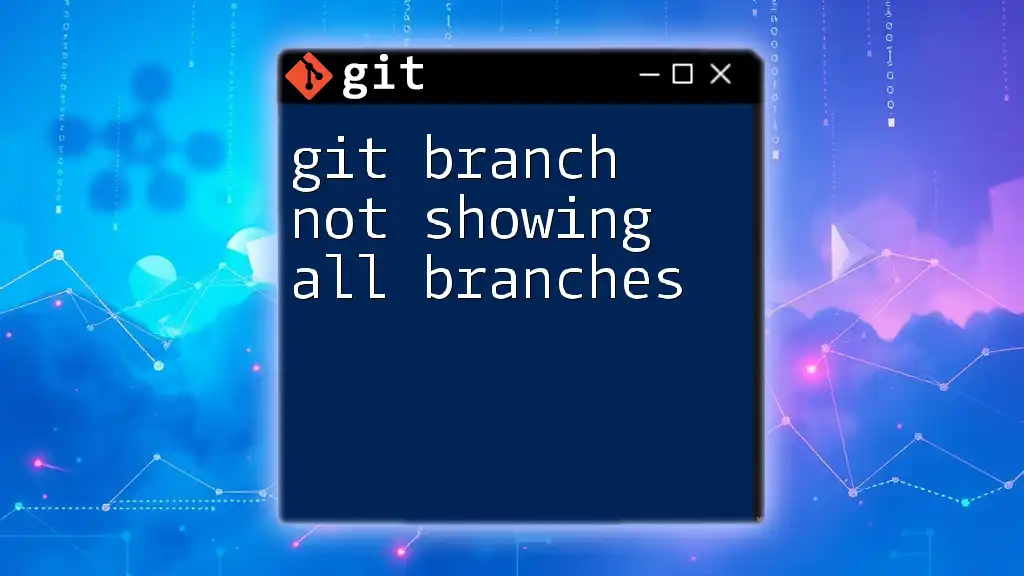
Conclusion
If you encounter situations where `git status not showing changes`, remember to check for these common issues. Ensure your files are saved, verify your `.gitignore` settings, confirm your directory alignment, and use Git commands effectively. By following these tips, you’ll be able to troubleshoot any problems confidently and maintain a smooth Git workflow.
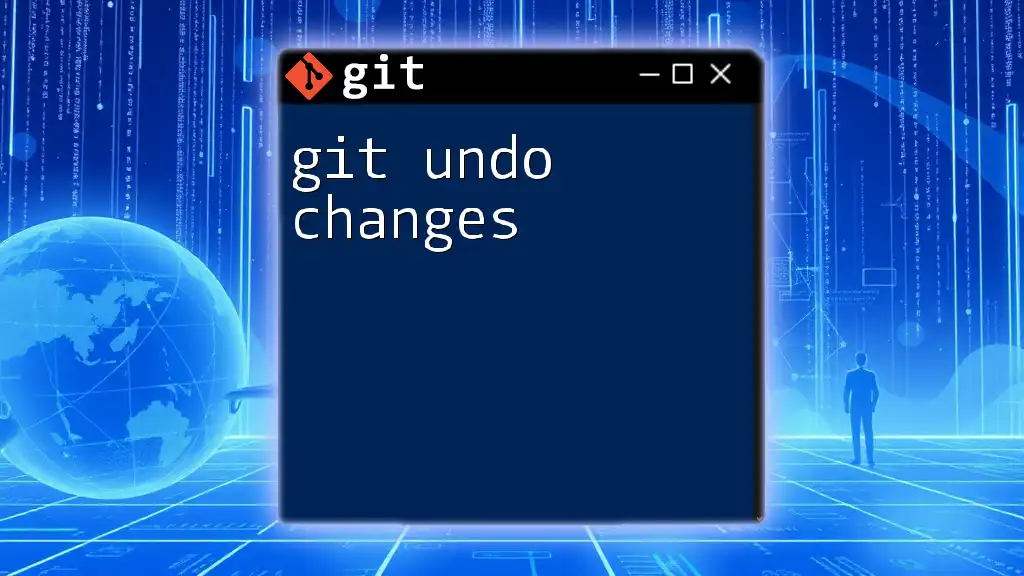
Call to Action
Have you experienced issues with `git status` not showing changes? Share your experiences and solutions in the comments! If you’re eager to learn more concise Git tutorials and tips, subscribe to stay updated!
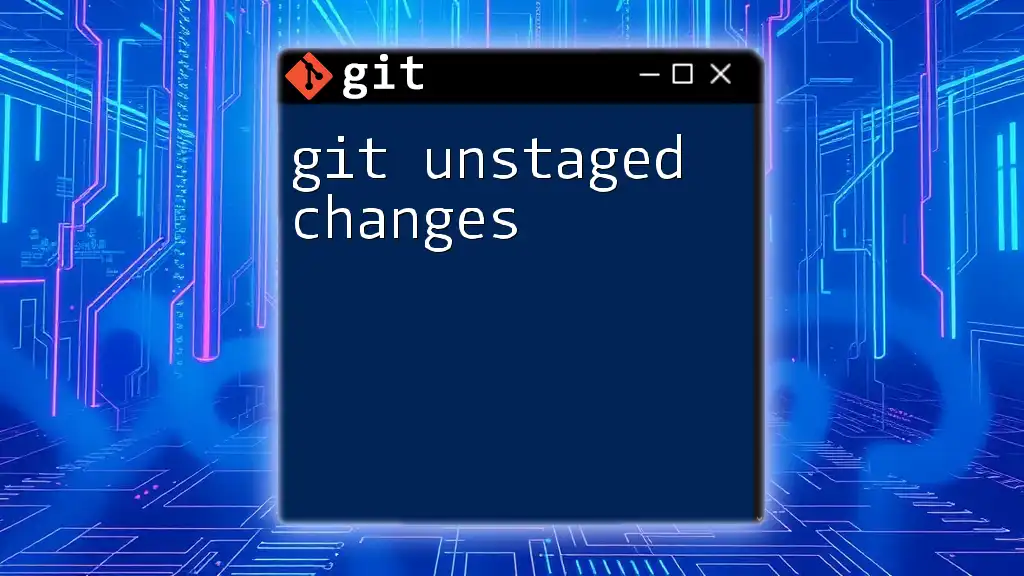
Additional Resources
Recommended Further Reading
- [Git Documentation](https://git-scm.com/doc)
- [Comprehensive Git Tutorials](https://www.atlassian.com/git/tutorials)
FAQs Section
- What should I do if `git status` still doesn't show expected files?
- How can I recover lost changes in Git?