The command `git push origin -u` is used to push your local commits to the remote repository named "origin" and set the upstream branch for the current local branch, allowing for simpler future push and pull commands.
git push origin -u <branch-name>
What is `git push`?
`git push` is one of the fundamental commands in Git that enables you to upload local repository content to a remote repository. When collaborating with a team or deploying code, pushing your changes ensures that your work is available to others.
The basic syntax for `git push` is as follows:
git push [<repository> [<refspec>...]]
In this syntax:
- `<repository>` refers to the remote repository you wish to push to, which is often named `origin` by default.
- `<refspec>` specifies the branch or branches you are pushing.
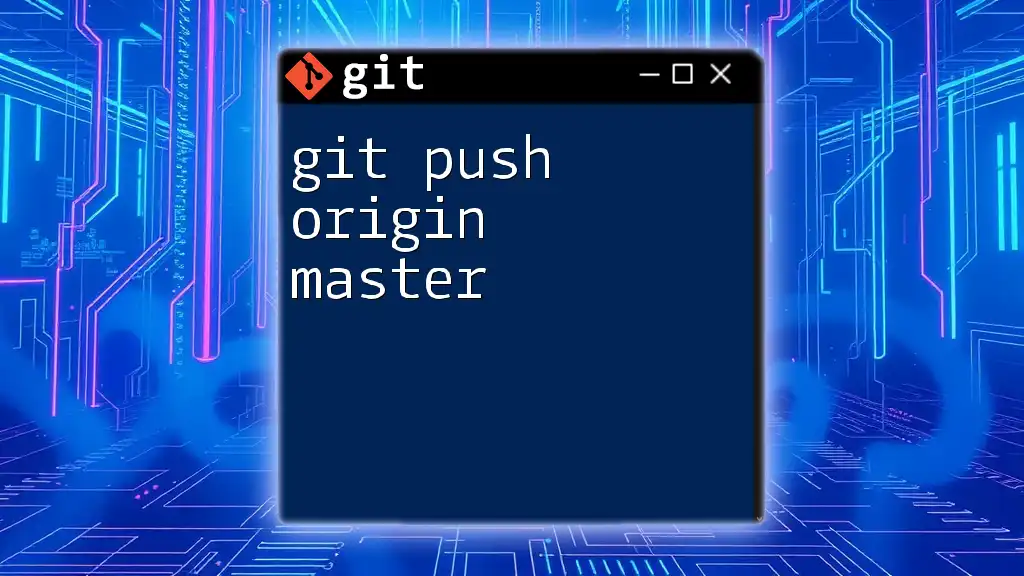
Understanding `origin`
Origin refers to the default remote repository in Git. When you clone a repository, Git automatically names the source repository `origin`. This simplifies the process of pushing and pulling changes since you don’t need to write the full URL every time.
To view your remote repositories, you can use:
git remote -v
You’ll see output displaying the remotes and their URLs, which it’s essential to set up prior to using `git push origin -u`. If you haven't set up a remote repository yet, you can do so using:
git remote add origin https://github.com/username/repo.git
This command creates a connection between your local repo and the remote repo hosted on GitHub (or any other Git hosting service).
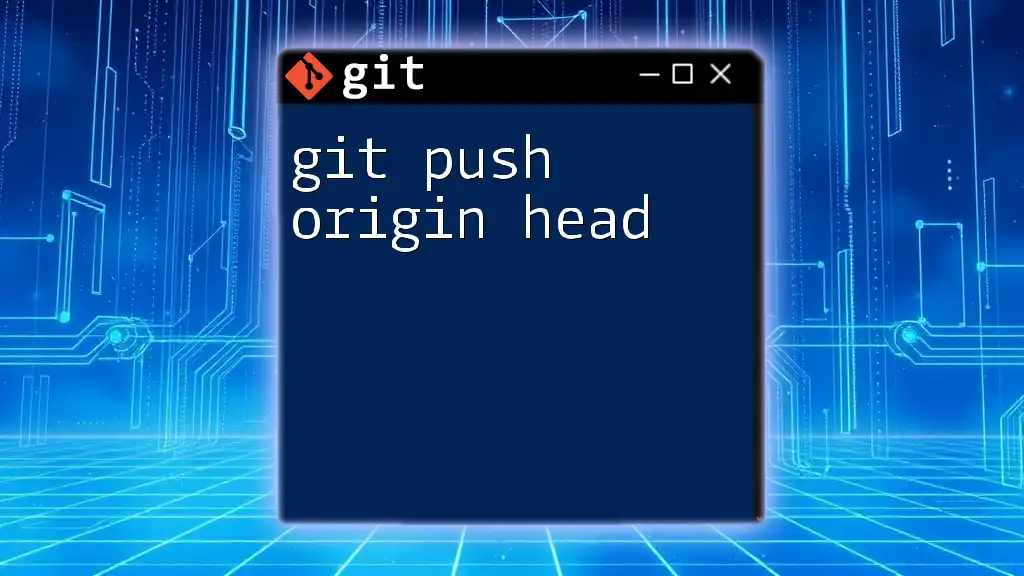
The `-u` Flag Explained
The `-u` flag in `git push origin -u` stands for `--set-upstream`. This flag tells Git to set the upstream reference for the local branch you are pushing.
By using the `-u` flag, you simplify your workflow. Future commands such as `git pull` or `git push` will know which remote branch to interact with automatically. For example, when pushing a local branch called `main`, you would run:
git push -u origin main
After executing this command, you will receive an output indicating that:
Branch 'main' set up to track remote branch 'main' from 'origin'.
This means your local `main` branch is now linked with the `main` branch on the origin remote.
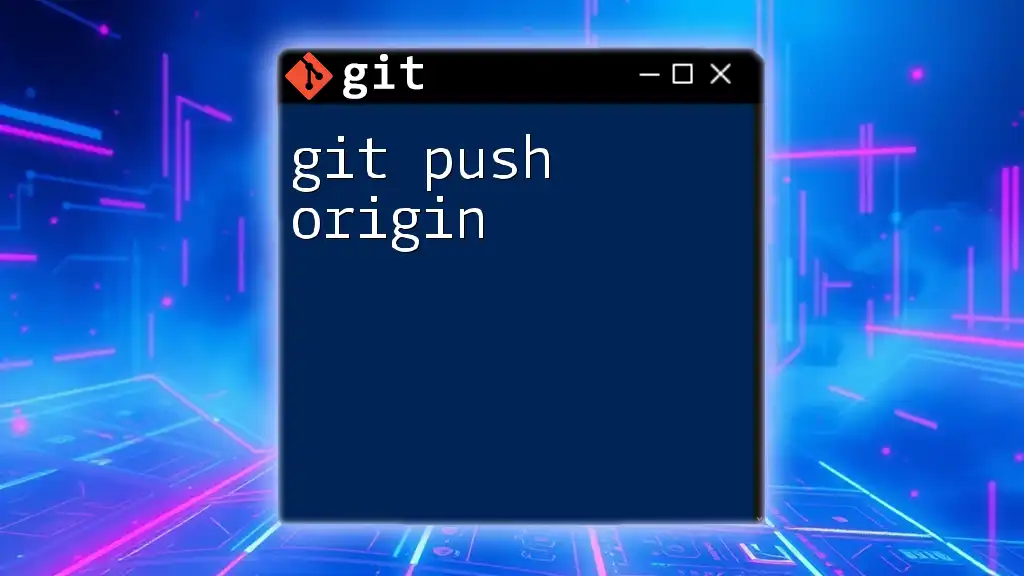
Use Cases for `git push origin -u`
One of the most common scenarios for using `git push origin -u` is during a first-time push to a remote repository. When you create a new local branch and want to share it with others, the `-u` flag is crucial. Here’s a sample process:
-
Create a new feature branch:
git checkout -b new-feature
-
Make your changes: After editing your files, stage them:
git add .
-
Commit your changes:
git commit -m "Add new feature"
-
Push the new branch to the remote repository with upstream tracking:
git push -u origin new-feature
Now, any changes made on your `new-feature` branch can be easily pushed or pulled without specifying the remote branch each time.
Another use case for `git push origin -u` is in a collaborative environment, where multiple teammates contribute to the same project. Using `-u` allows for smooth collaboration and ensures everyone is on the same page regarding the branches being worked on.
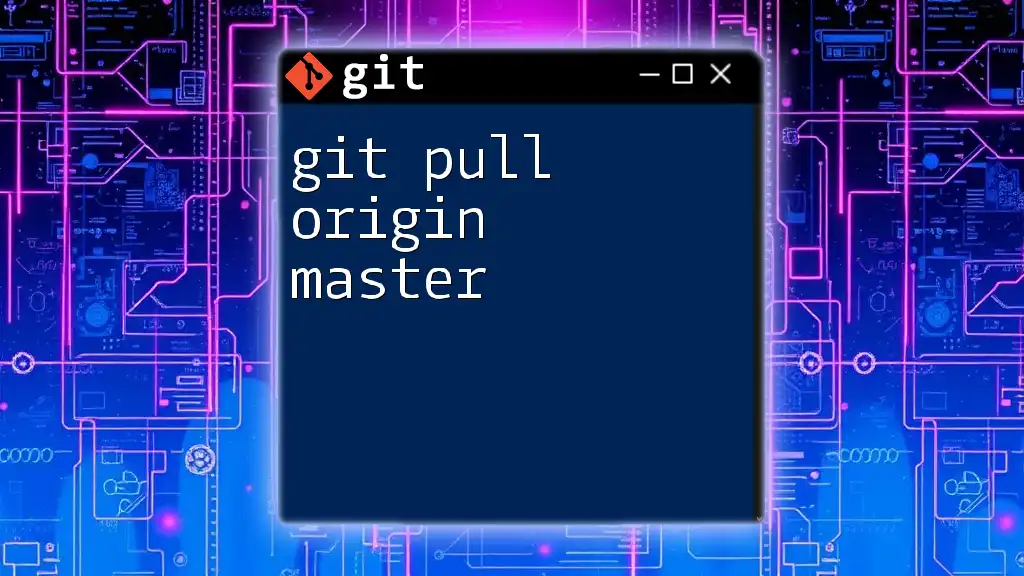
Common Errors and Troubleshooting
Even experienced users may encounter errors when using `git push origin -u`. Here are some common errors and how to troubleshoot them:
Error: "fatal: The upstream branch 'main' does not exist"
This error usually occurs when you're trying to push to a remote branch that hasn't been created yet. To resolve this, ensure that you are pushing a new branch, or check if the target branch exists on the remote. If it doesn’t, using `-u` will create it for you.
Error: "failed to push some refs"
This can happen if your local branch is out of sync with the remote branch. To resolve the issue, you can first pull the latest changes from the remote branch before pushing:
git pull origin main
After resolving any conflicts, you can then attempt to push again.
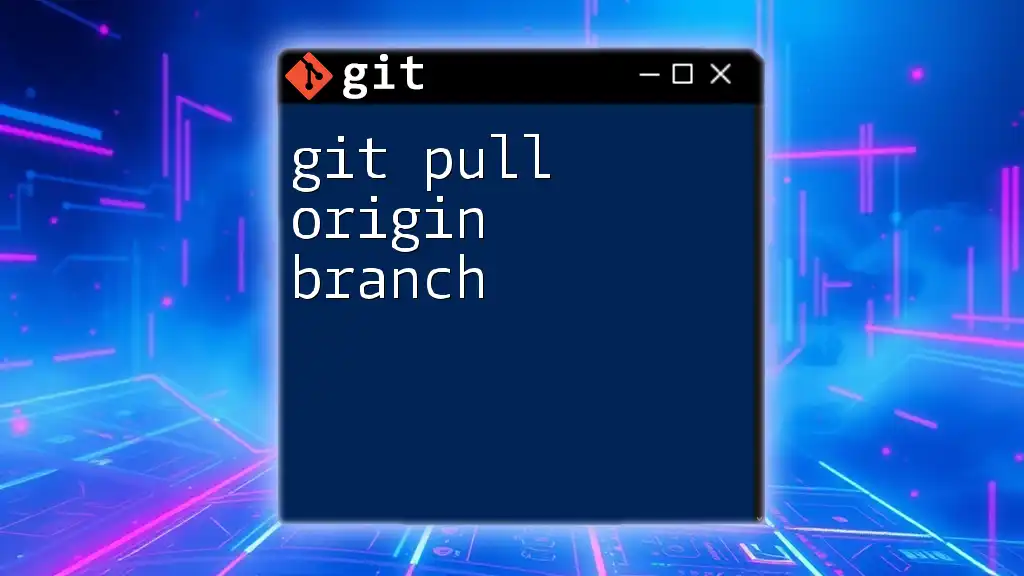
Best Practices
Knowing when to use `git push origin -u` effectively can greatly enhance your Git workflow. Here are some best practices:
-
Initial Push: Always use `-u` when you are pushing a new branch for the first time. This sets the groundwork for all future interactions with that branch.
-
Branch Naming Conventions: Adopt a clear naming convention for your branches to convey the purpose of your work, such as `feature/login-page` or `bugfix/correct-typo`. This practice helps team members quickly understand the context of your branch.
-
Regular Push Habits: Get into the routine of pushing small, frequent commits. This not only keeps your team informed but also makes it easier to manage code reviews and reverts if necessary.
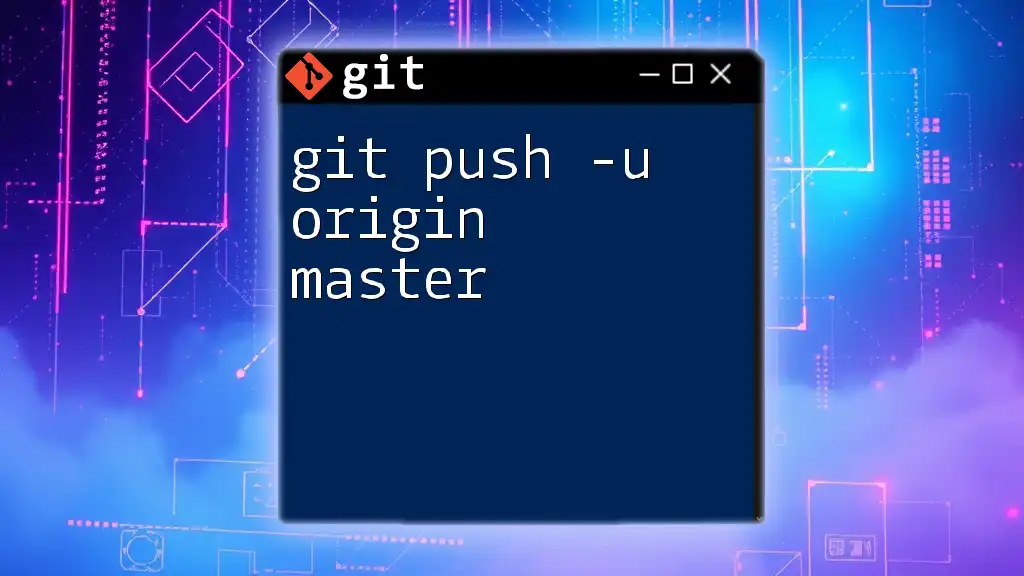
Conclusion
The `git push origin -u` command is a powerful tool in your Git arsenal, enabling smooth collaboration and efficient version control. By understanding its components and use cases, you can simplify your local and remote interactions, ensuring your work is consistently available to your team.
As you continue to explore Git, don’t hesitate to incorporate this command into your workflow and practice frequently. This habit will improve your development process and provide better transparency in team projects.
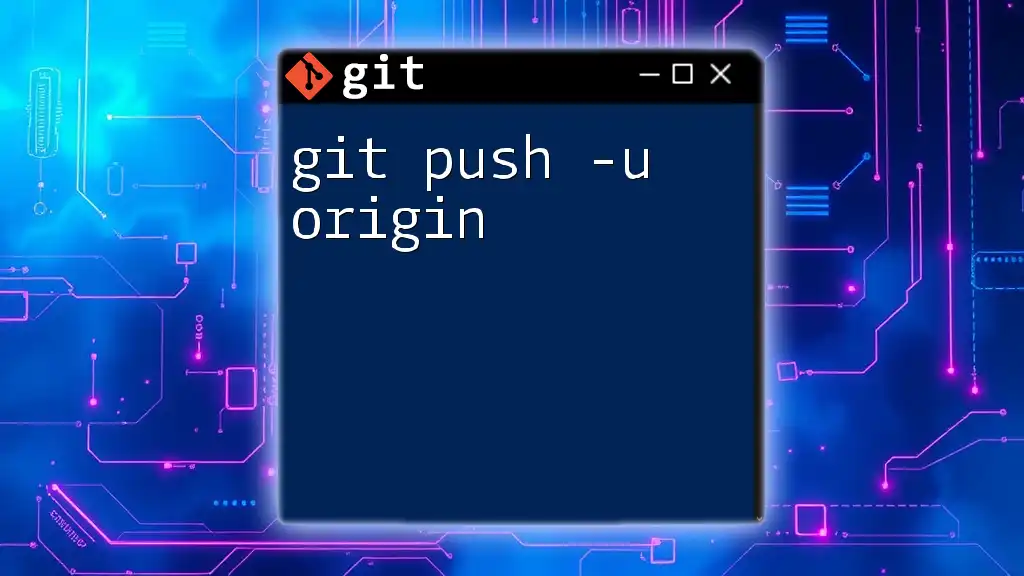
Call to Action
For more in-depth tutorials and guides on Git command usage, follow our blog and stay updated on the latest strategies for mastering Git!