In this Git Bash tutorial, you'll learn how to quickly navigate your repository and execute essential commands to manage your version control effectively.
Here’s a simple code snippet to clone a repository:
git clone https://github.com/username/repo.git
Introduction to Git Bash
What is Git Bash?
Git Bash is a command-line interface that combines Git with the Bash shell, allowing users to manage version control efficiently. It is primarily used on Windows but can be installed on Mac and Linux as well. Git Bash enables developers to execute Git commands seamlessly in a UNIX-like environment, which is essential for collaborating on projects and tracking changes in code over time.
Why Use Git Bash?
Using Git Bash offers several advantages over graphical interfaces. It allows for greater flexibility and control when managing repositories. Command-line interactions can be faster and more powerful, especially for users who are familiar with the commands. Moreover, many advanced Git features are only available through the command line. For instance, complex tasks such as rebasing, merging, or resolving conflicts can be executed more swiftly using Git Bash.
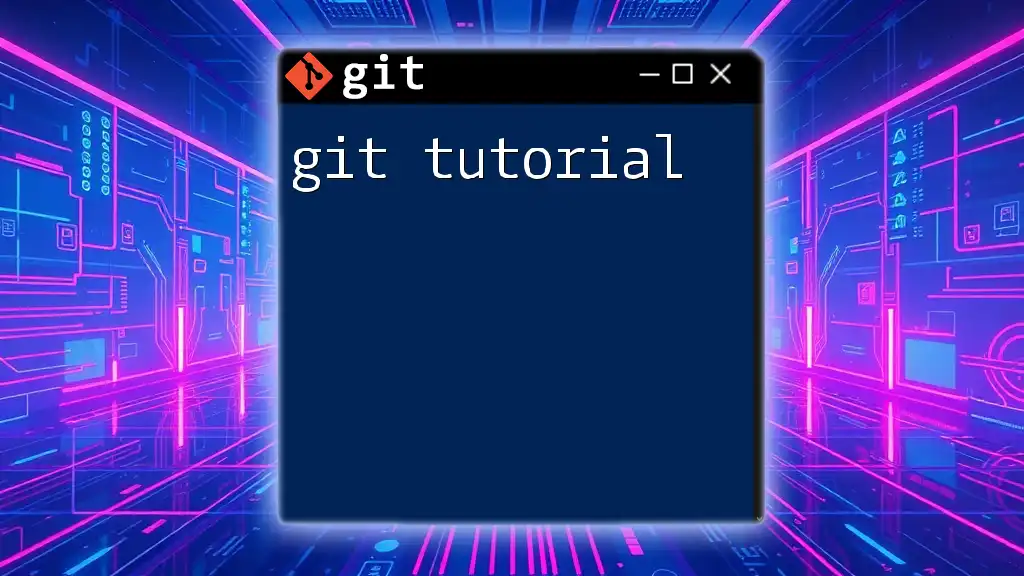
Setting Up Git Bash
Installing Git Bash on Your System
To begin using Git Bash, the first step is to install it on your machine. Here’s a brief installation guide:
-
Windows: You can download the Git installer from [git-scm.com](https://git-scm.com/). Follow the installation prompts, and make sure to check the option to include Git Bash in the context menu during installation.
-
Mac: Install Git Bash using Homebrew with the command:
brew install git
-
Linux: Use your package manager to install git. For Debian-based systems, run:
sudo apt-get install git
Configuring Git Bash
After installation, configuring Git Bash for your needs is crucial. You can customize your terminal appearance by changing colors and fonts in the terminal settings. Setting up your identity is also a critical step. Use the following commands to configure your name and email, which will be attached to your commits:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
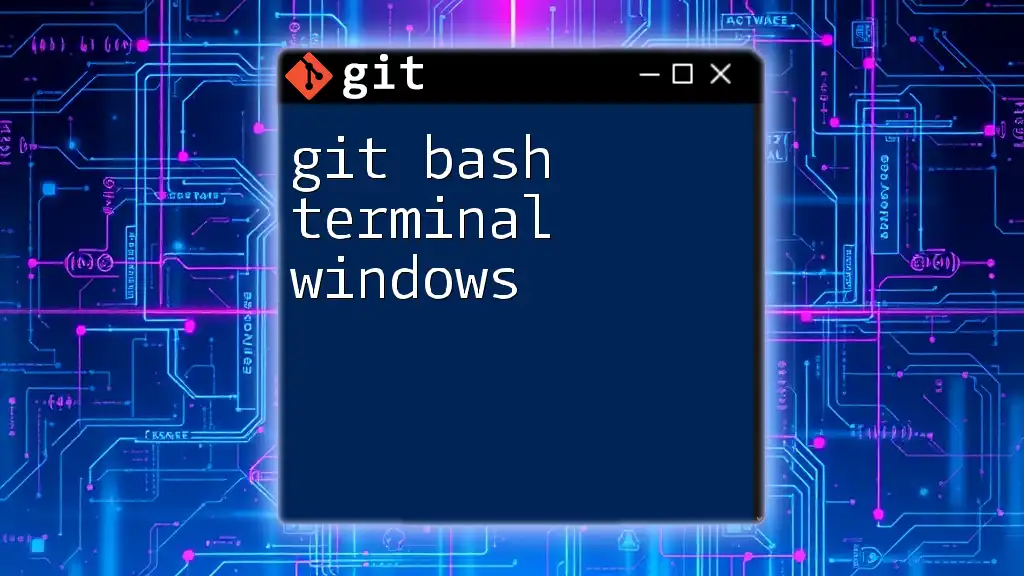
The Basics of Git Commands
Navigating the Filesystem in Git Bash
Learning to navigate your filesystem with Git Bash is foundational. Start with the basic commands:
- Changing Directory: Use `cd` to navigate to your project's directory.
- Listing Files: You can see the files in your current directory with `ls`, and to view detailed information, use `ls -la`.
- Getting Current Directory: To know your current path, simply type `pwd`.
Example usage:
cd /path/to/your/repo
ls -la
pwd
Creating and Managing Repositories
Creating a Git repository or managing existing ones is straightforward.
-
Initializing a New Git Repository: To create a new repository in your current directory, run:
git init
-
Cloning an Existing Repository: If you want to work on an existing project, you can clone it from a remote source, such as GitHub, using:
git clone https://github.com/username/repo.git
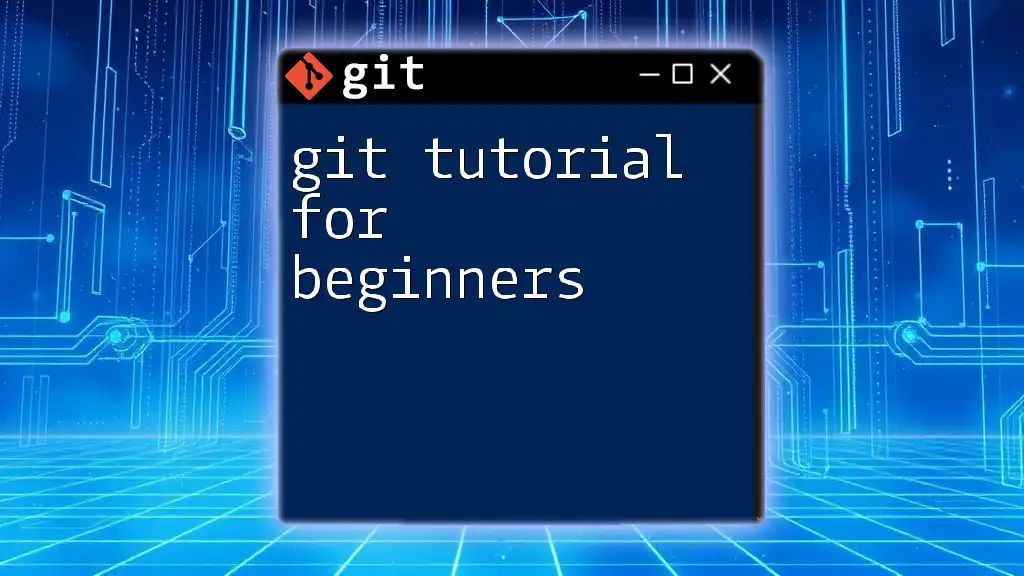
Core Git Commands in Action
Tracking Changes
One of the most powerful features of Git is its ability to track changes.
-
Checking Status of Your Repository: Use `git status` to see the state of your current working directory and staging area. It informs you about modified files, untracked files, and more.
-
Adding and Committing Changes: After modifying files, you need to stage and commit them. Use `git add` to stage files and `git commit` to commit:
git add file.txt git commit -m "Initial commit"
Managing Branches
Branches are fundamental for working on features or bug fixes without affecting the main codebase.
-
Creating a New Branch: To create and switch to a new branch simultaneously, use:
git checkout -b new-branch
-
Merging Branches: When you’re ready to integrate changes from your feature branch back into the main branch, first switch to the main branch and then merge:
git checkout main git merge new-branch
Pushing and Pulling Changes
Syncing your changes with a remote repository is essential for collaboration.
-
Pushing Changes to Remote: To send your commits to the remote repository, use:
git push origin main
-
Pulling Changes from Remote: To update your local repository with the latest changes from the remote, use:
git pull origin main
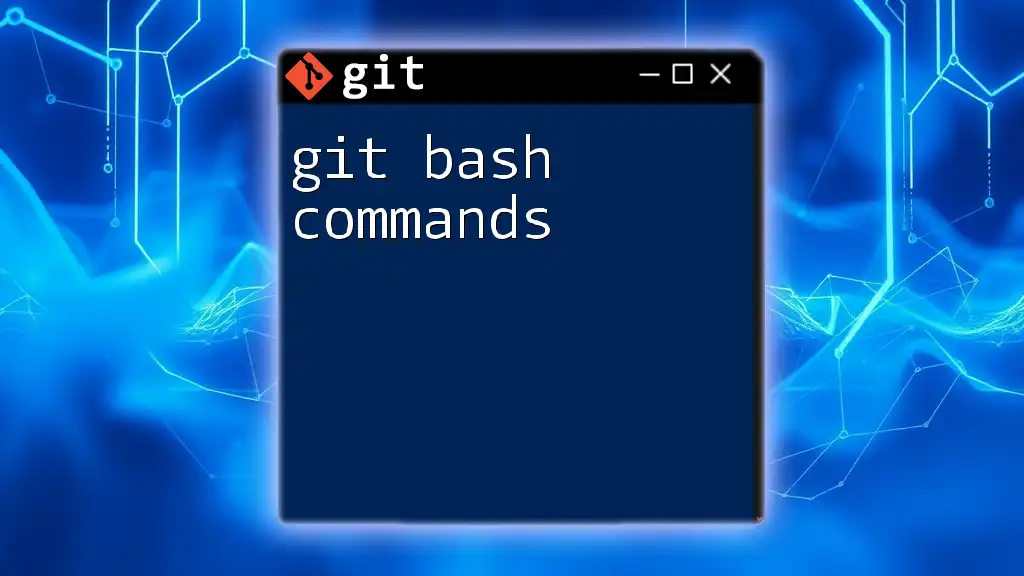
Advanced Git Bash Commands
Viewing Commit History
Understanding your commit history is vital. You can view logs using:
git log
To present it more succinctly, use:
git log --oneline --graph
This gives you an overview of your commit history in a compact format.
Stashing Changes
Sometimes, you may need to save your work temporarily without committing. This is where `git stash` comes in handy. To stash your changes, simply run:
git stash
When you’re ready to retrieve those changes, use:
git stash pop
Resolving Merge Conflicts
Merge conflicts may occur when changes from different branches overlap. To resolve them:
- Identify conflicts using `git status`.
- Open the conflicting file and edit it to reconcile changes.
- After resolving, stage the changes and commit:
git add file.txt git commit -m "Resolved merge conflict"
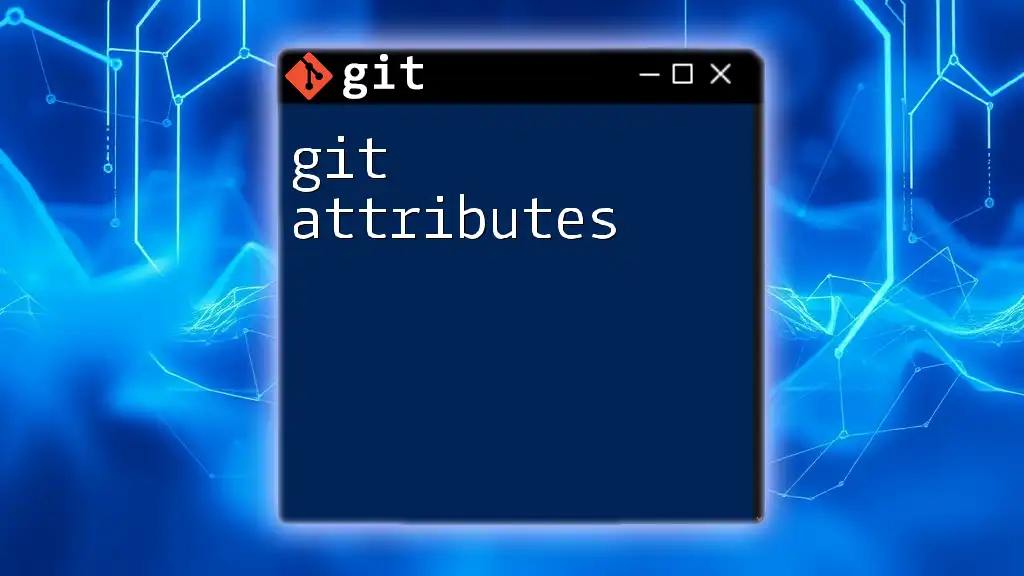
Customizing Git Bash Usage
Shortcuts and Aliases
To improve your efficiency, you can create aliases for frequently used commands. For example, if you often check out branches, you can set an alias:
git config --global alias.co checkout
Now, instead of typing `git checkout`, you can simply type `git co`.
Utilizing Git Bash with Other Tools
Integrating Git Bash with code editors like Visual Studio Code can streamline your workflow. Using the built-in terminal in VS Code allows you to run Git commands directly while coding.
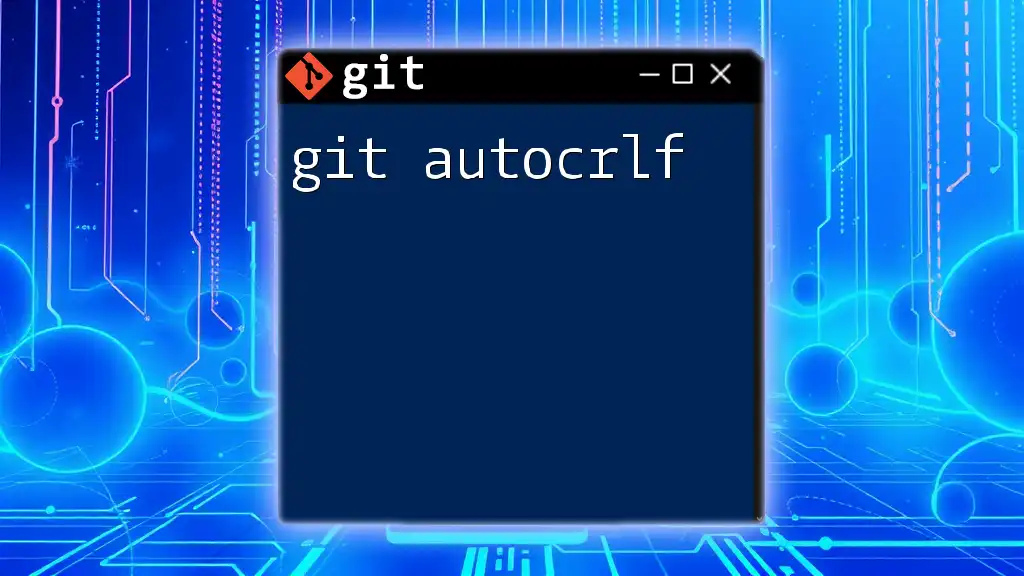
Troubleshooting Common Issues
Handling Common Errors in Git Bash
While working with Git Bash, you might encounter several common errors, such as merge conflicts or authentication issues. Understanding these errors and how to resolve them is crucial for maintaining efficiency. Make sure to check online resources or the Git documentation for guidance on specific errors you face.
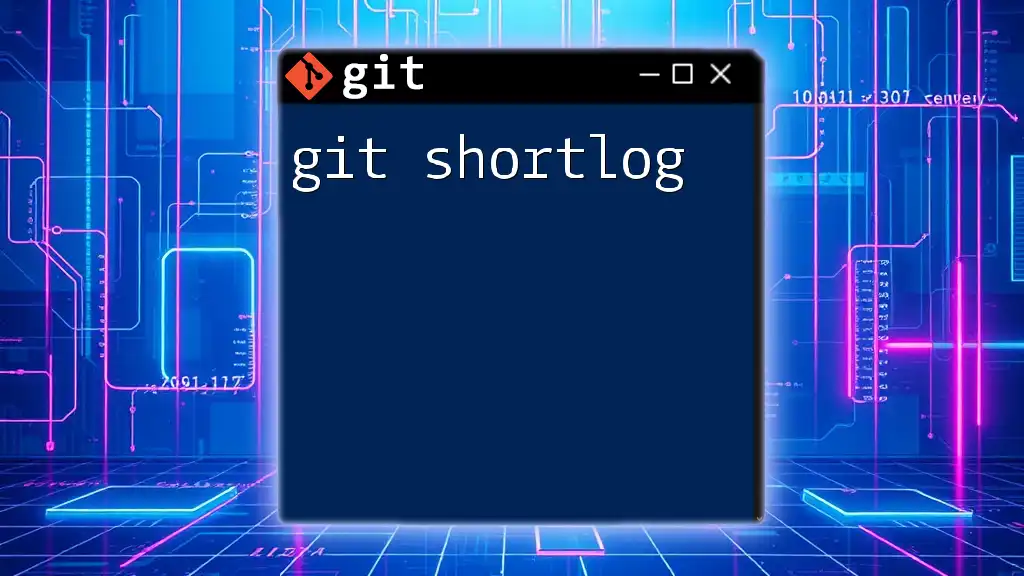
Conclusion
In this Git Bash tutorial, we’ve covered the fundamentals of setting up and using Git Bash effectively. From navigating your filesystem to managing repositories and resolving conflicts, these skills are essential for any developer looking to harness the full power of Git.
As you continue your journey with Git, practice using these commands regularly to become more comfortable and proficient. The command line may seem daunting at first, but with time and experience, it will enhance your productivity and elevate your coding skills.
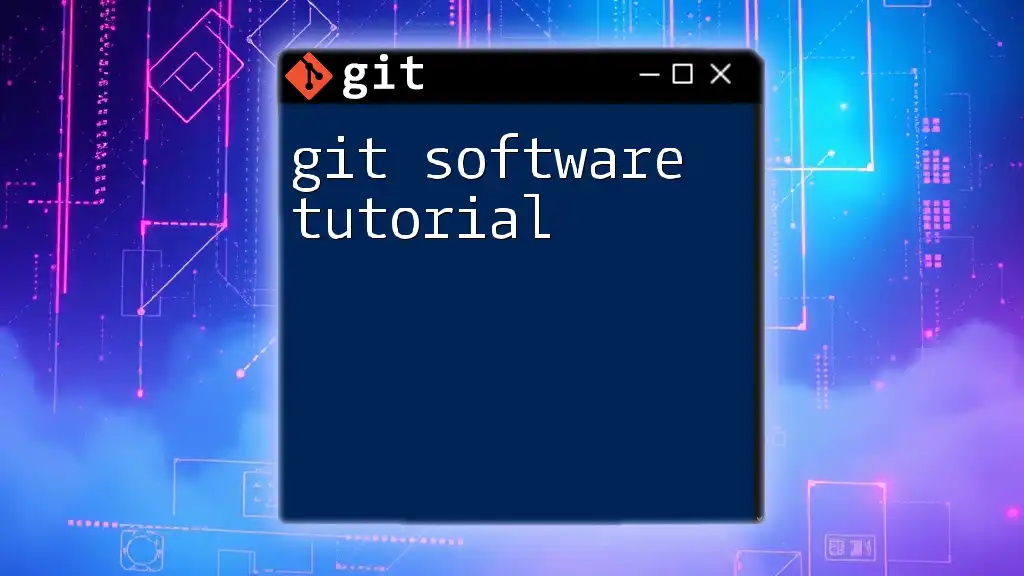
Additional Resources
For ongoing learning and support, explore Git documentation, cheat sheets, and community forums. Engaging with these resources will help you deepen your understanding and make you a more effective Git user.