The Git Bash prompt is a command-line interface that allows users to interact with Git and execute Git commands on their operating system, typically featuring a simple syntax for version control tasks.
Here’s a code snippet demonstrating how to create a new Git repository:
git init my-repo
Understanding Git Bash
What is Git Bash?
Git Bash is a command line interface specifically designed for Windows environments that provides a Unix-like shell, enabling users to interact with Git, the widely-used version control system. It comes packaged with Git for Windows and serves as a powerful tool for performing Git operations, allowing for a seamless experience for developers who prefer the command line over graphical user interfaces.
Why Use Git Bash?
Utilizing Git Bash comes with numerous advantages. First, it allows for quicker execution of commands compared to navigating through a GUI. Many experienced developers find command-line operations to be more efficient for repetitive tasks, as well as for scripting and automation.
Additionally, the Git Bash environment is consistent across various operating systems, making it easier to learn and deploy Git commands in different environments. When compared to other Git tools, the CLI provides unparalleled flexibility and direct access to all Git features.
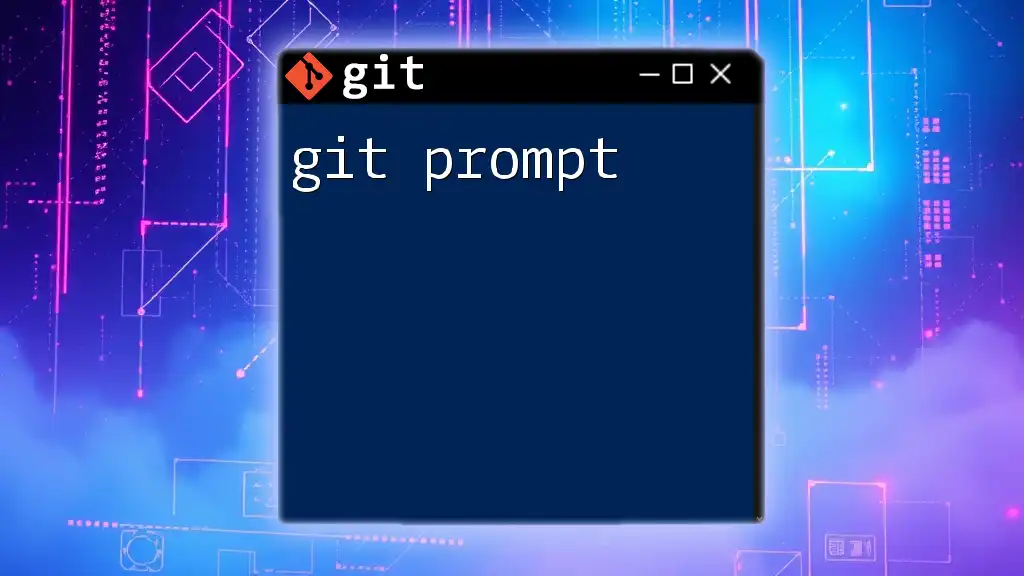
The Git Bash Prompt
What is the Git Bash Prompt?
The Git Bash prompt is the command line interface that awaits your instructions. It typically displays system information, including the username, hostname, current directory, and the Git branch you are operating on. Understanding how to navigate and utilize this prompt is crucial for efficient version control.
Anatomy of the Git Bash Prompt
When you open Git Bash, you might encounter a prompt that looks something like this:
username@hostname MINGW64 ~/path/to/directory (branch_name)
$
-
Username: This is your system's username and is displayed before the `@` symbol.
-
Host: This indicates the machine you are working on, typically represented by the hostname of your device.
-
Current Directory: The path where you currently are in your filesystem. This is crucial as it dictates where Git commands will take effect.
-
Branch Name: The currently checked out branch of your repository appears in parentheses, helping you keep track of the branch context.
Default vs. Custom Prompts
Default Prompt Configuration
The default prompt layout is functional but may not suit everyone's preferences. The default prompt typically includes all the aforementioned components but in a basic format. For example:
user@host MINGW64 ~/some/repo
$
Customizing the Git Bash Prompt
Customizing the Git Bash prompt allows you to display information that matters most to you, enhancing your productivity. The prompt is controlled by the PS1 environment variable.
To customize your prompt, you can alter the `PS1` variable in your `.bashrc` file. Here’s a simple code snippet to get you started:
export PS1="\u@\h \W (\$(git branch 2>/dev/null | grep '^*' | colrm 1 2))\$ "
- \u: Stands for username.
- \h: Represents the hostname.
- \W: Displays the current working directory.
- The Git branch name is fetched with a command that checks for the active branch.
Example of Custom Prompt Configuration
For a more personalized prompt, consider the following configuration:
export PS1="\[\e[32m\]\u@\h \[\e[34m\]\W \[\e[31m\](\$(git branch 2>/dev/null | grep '^*' | colrm 1 2))\[\e[0m\]\$ "
In this example, the prompt includes colored text for better visibility. The different escape sequences (like `\[\e[32m\]`) change the text color, making each component distinct and easier to read.
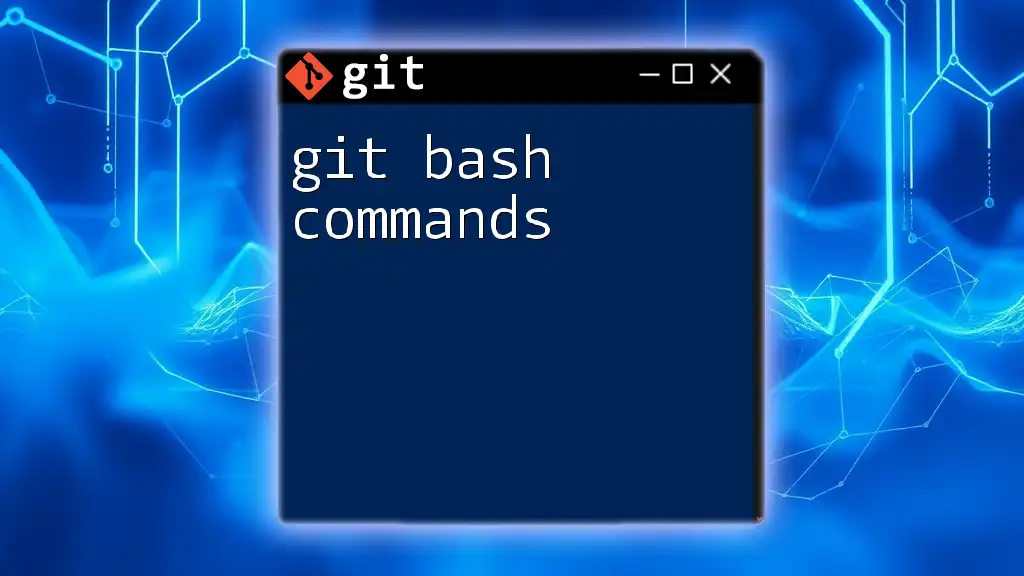
Useful Commands Using the Git Bash Prompt
Basic Git Commands from the Prompt
The Git Bash prompt serves as the interface where all Git commands can be executed. Here are a few foundational commands:
-
git status: This command checks the status of your repository, showing staged changes, unstaged changes, and untracked files.
git status
-
git add: Use this command to add files to the staging area, preparing them for the next commit.
git add filename.txt
-
git commit: This command commits your staged snapshot with a message describing the changes made.
git commit -m "Your commit message"
Advanced Git Commands Using the Prompt
Branching Commands
Understanding branching is key to mastering Git:
-
git branch: This command allows you to create and list branches in your repository.
git branch
-
git checkout: Use this command to switch to a different branch.
git checkout branch_name
Merging and Rebasing
As you grow in your Git usage, you will also need to understand merging and rebasing:
-
git merge: This merges two branches together, incorporating the changes from one branch into another.
git merge branch_name
-
git rebase: This command helps to move or combine a series of commits to a new base commit, allowing for a cleaner history.
git rebase branch_name
Navigating the Filesystem in Git Bash
Navigating the filesystem is essential for working efficiently in Git Bash:
-
Use cd to change directories:
cd ~/path/to/your/project
-
Utilize ls to list files and directories in your current location:
ls -la
These commands allow you to move around in your file system effortlessly and manage your project files directly from the terminal.
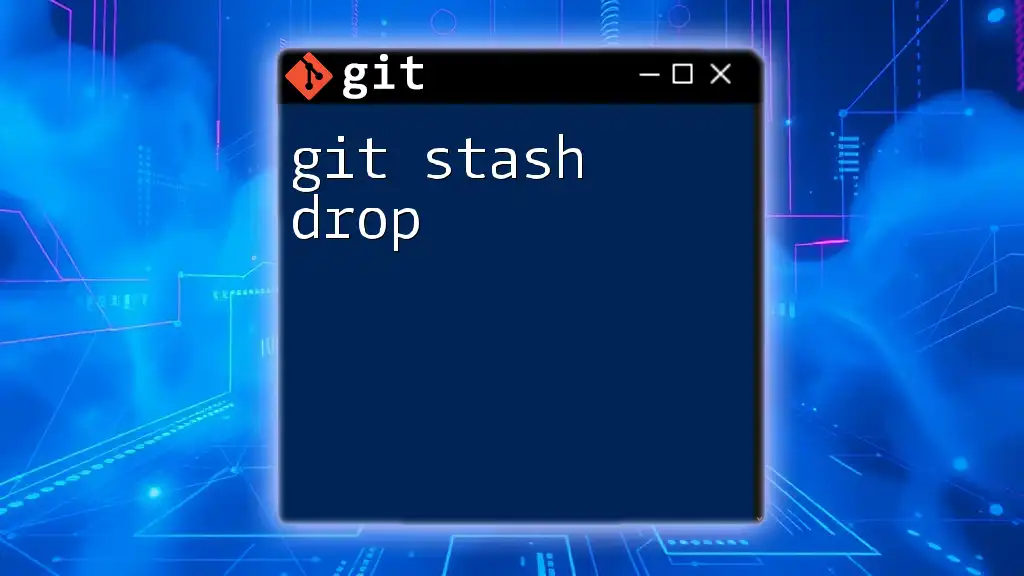
Troubleshooting Common Issues with Git Bash Prompt
Common Errors and Fixes
When using Git Bash, you may encounter errors. Common problems arise when Git Bash doesn't recognize commands, often due to misconfigured installations. If you face this issue, ensure Git is properly installed and its path is added to your system's environment variables.
Configuring Path Variables
To ensure Git is accessible via the command line, adding it to your system’s PATH variable is essential:
- Open your system environment variables.
- Locate the `Path` variable and select it.
- Click Edit and add the path to your Git installation's `bin` directory.
After adding, verify the setup by running:
git --version
If it outputs the installed version of Git, then your setup is correct.
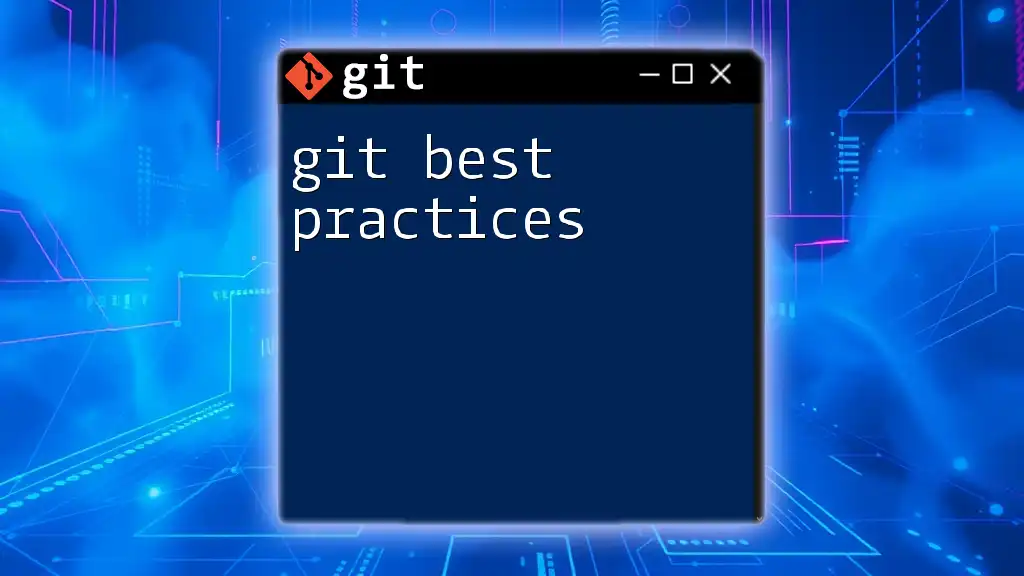
Conclusion
Understanding and utilizing the Git Bash prompt is a fundamental skill for anyone working with Git. The ability to customize and interact effectively through the prompt enhances your workflow, making version control simpler and more intuitive.
Embrace the command line, practice regularly, and participate in discussions about custom prompts and any challenges you face. As you become more comfortable, explore advanced Git features that will elevate your development process to new heights.
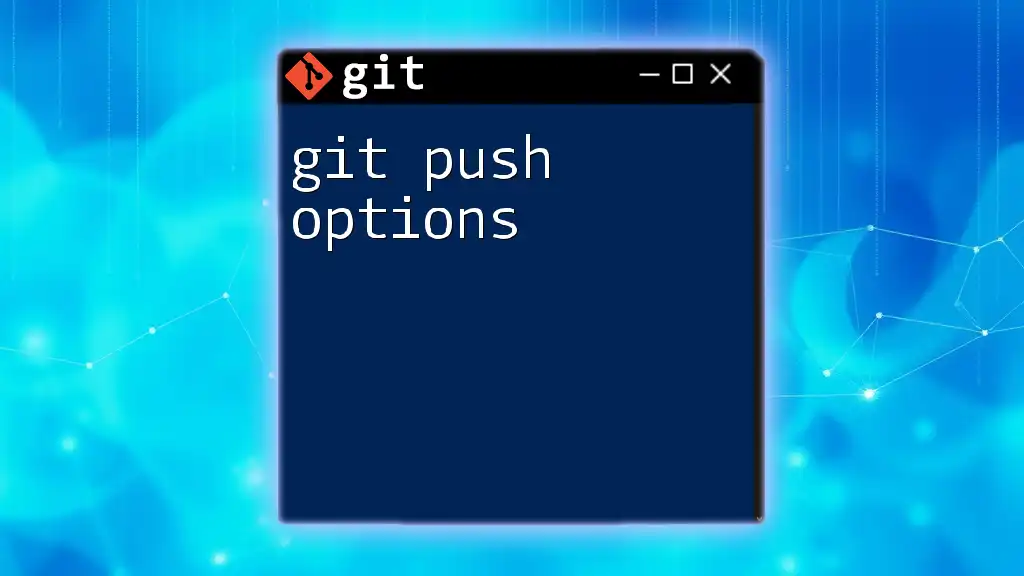
Additional Resources
Explore the official Git documentation for extensive insights and tips on using Git effectively. For those looking to deepen their understanding further, numerous tutorials and cheat sheets are available online to assist throughout your learning journey.
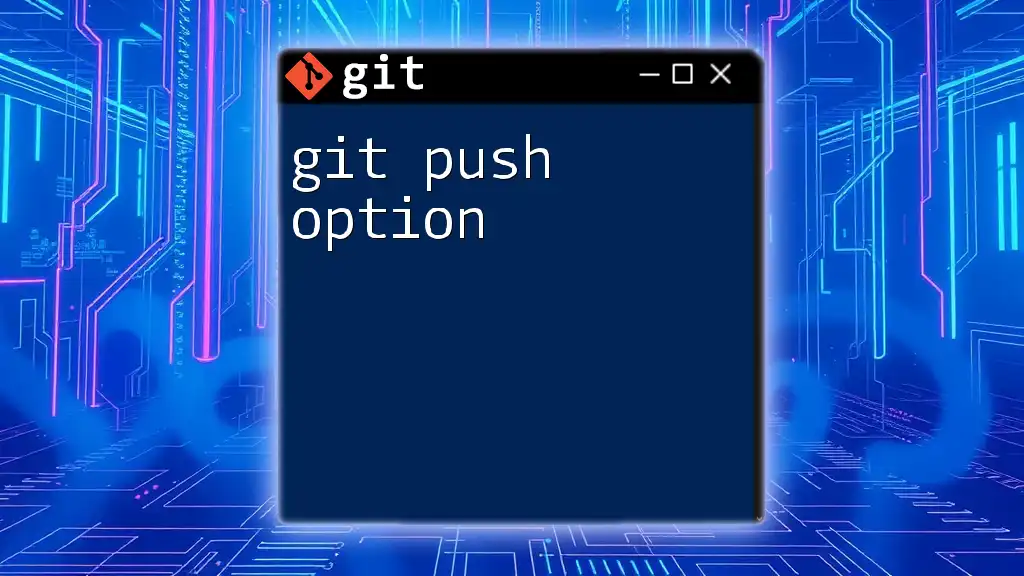
Call to Action
Join the conversation—share your unique prompt configurations or ask questions related to Git Bash. Consider signing up for upcoming workshops that delve deeper into Git essentials and productivity tips. Expand your knowledge and streamline your version control experience!