Git testing refers to the practice of using commands like `git status` and `git diff` to check the current state of your repository and to compare changes before committing them.
Here's a code snippet to illustrate this:
# Check the status of your repository
git status
# Show changes between the working directory and the index
git diff
Understanding Git Testing
What Is Git Testing?
Git testing involves the use of Git commands and workflows tailored to ensure code quality, functionality, and reliability throughout the software development lifecycle. As an integral part of development, it allows teams to manage changes, evaluate the accuracy of code, and validate new features while simultaneously tracking progress.
Why Git Testing Matters
The significance of testing cannot be overstated. It helps to identify and address bugs early in the development process, preventing issues from escalating into more significant problems later on. This proactive approach can save time, reduce costs, and enhance overall software quality. By using Git effectively, teams can collaborate more seamlessly, maintain version control, and ensure that the code base remains stable and trustworthy over time.
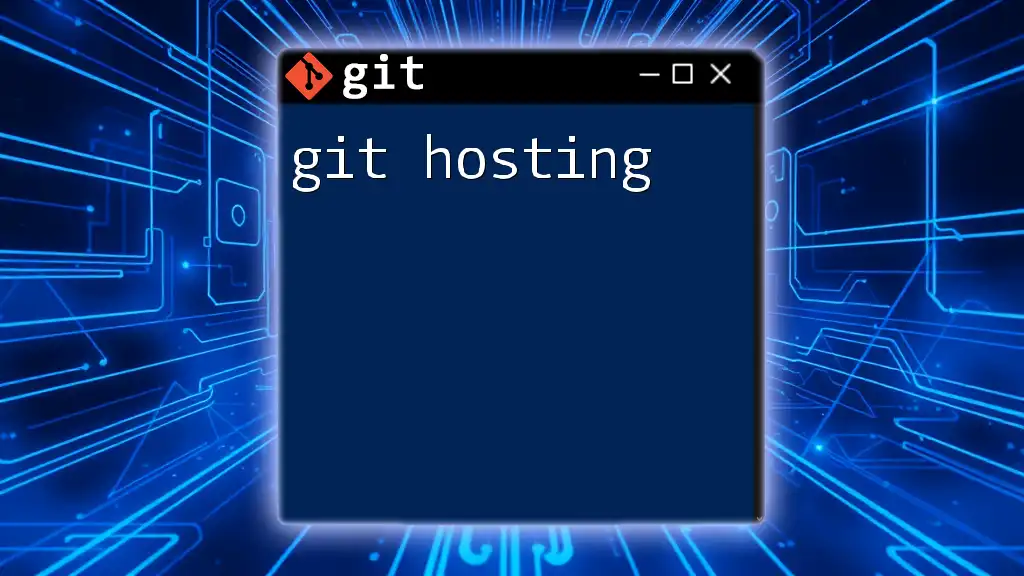
Setting Up Your Git Environment for Testing
Installing Git
To begin with Git testing, it's essential to have Git installed on your machine. Here’s how to set it up on various operating systems:
-
Windows: Download the Git installer from the official website and follow the installation instructions.
-
macOS: You can install Git using Homebrew with the command:
brew install git
-
Linux: Use your distribution’s package manager; for example, on Debian/Ubuntu:
sudo apt-get install git
Configuring Your Git Environment
Once Git is installed, configure your Git environment to ensure a smooth experience. It starts with setting up your user information, which is crucial for keeping track of who makes changes in a shared repository. You can do this with the following commands:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
This configuration ensures that all your commits are attributed correctly, enabling clearer collaboration.
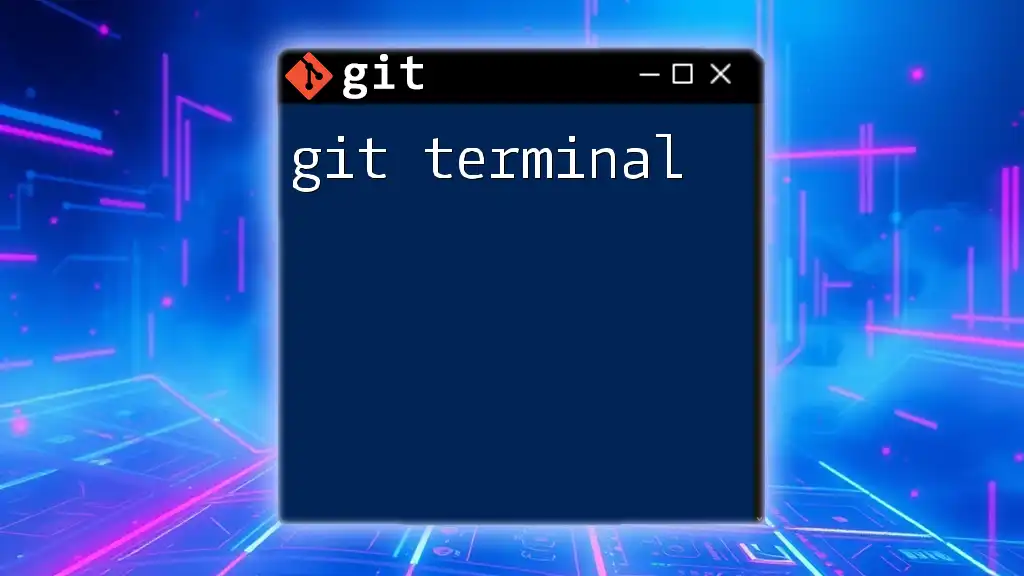
Basic Git Commands for Testing
Creating a Test Repository
The first step in Git testing is to create a test repository. This controlled environment allows you to experiment without affecting any production code. Follow these steps to initialize your repository:
mkdir test-repo
cd test-repo
git init
After running these commands, you will have a new directory named `test-repo` that is now a Git repository.
Essential Git Commands
To effectively manage and test your code changes, understanding essential Git commands is critical.
-
`git status`: This command provides an overview of the current state of your repository, indicating untracked files, staged changes, and files ready for commit. This helps you understand what needs action.
-
`git add`: Used to stage changes for commit. You can stage all your modified files with:
git add .
-
`git commit`: This command records your staged changes into the repository. Use meaningful commit messages to describe the changes you’ve made:
git commit -m "Initial commit with testing setup"
These commands form the backbone of your Git testing workflow.
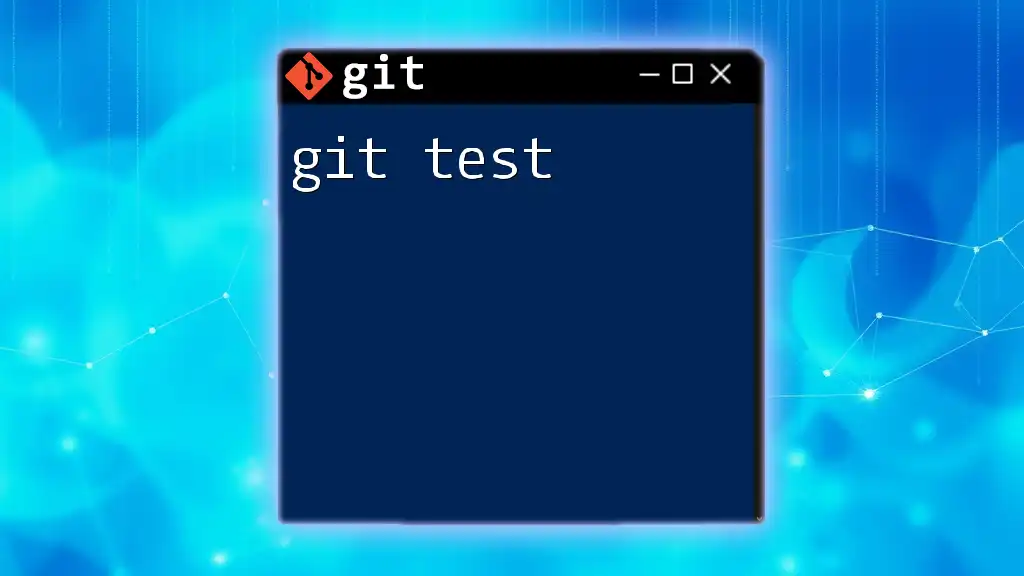
Advanced Git Testing Techniques
Branching Strategies in Testing
Employing branching strategies is essential for organized testing. Branching allows you to work on features or fixes independently without disrupting the main development flow. Two common strategies include:
-
Git Flow: A structured branching model that separates the development and production branches, with additional branches for features, releases, and hotfixes.
-
Feature Branching: Each new feature is developed in its own branch, which can then be merged back into the main branch after testing and review.
Creating a new branch is simple with Git:
git checkout -b testing-branch
This creates and switches to a new branch named “testing-branch,” allowing you to perform your tests without affecting the main codebase.
Using Tags for Versioning
Tags are useful within Git testing to mark specific points in your repository's history, often used for releases. Tagging your commits can help you easily reference important versions of your codebase. For example, to tag a released version:
git tag -a v1.0 -m "Version 1.0 release"
Tags create a snapshot of your repository at a specific time, making tracking changes and features straightforward.
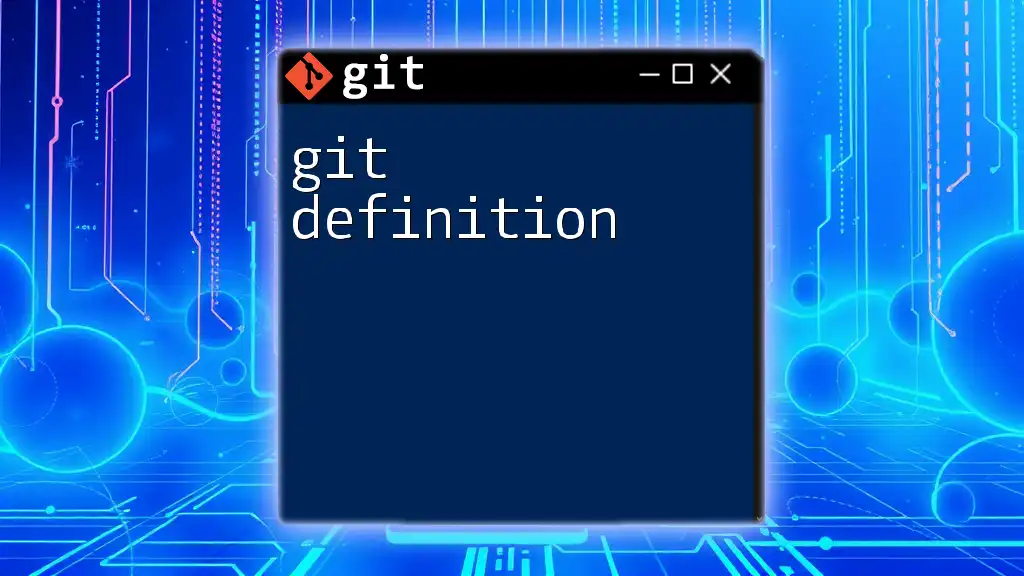
Integrating Testing Frameworks with Git
Overview of Testing Frameworks
To ensure that your code works as intended, integrate testing frameworks such as JUnit (Java), Mocha (JavaScript), or pytest (Python). These tools help automate the testing process, allowing you to identify issues efficiently.
Running Tests with Git Hooks
Git hooks are scripts that can execute actions automatically at various points in the Git workflow. For instance, you can set up a pre-commit hook to run your tests before allowing a commit. Here’s how to set up a pre-commit hook:
Create a file named `pre-commit` in your repository’s `.git/hooks/` directory with the following contents:
#!/bin/sh
npm test
This script will automatically run your tests every time you make a commit, ensuring that only code that passes the tests gets committed.
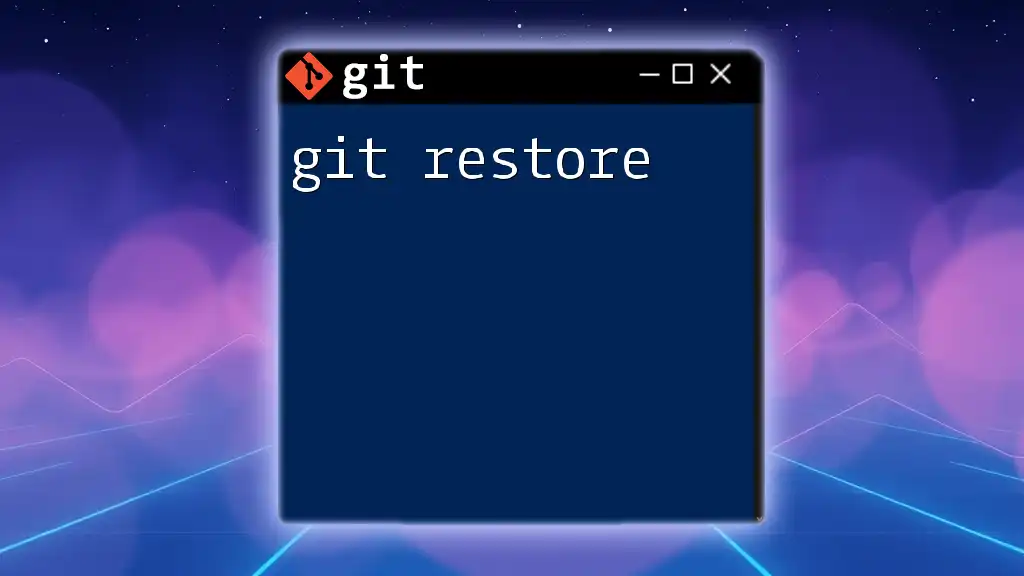
Best Practices for Git Testing
Consistent Commit Messages
Using clear and concise commit messages is vital for effective collaboration. It allows team members to understand the history and purpose of changes. A recommended format includes a subject line, a body with a detailed explanation if necessary, and any relevant issue numbers.
Regular Merging and Pulling
It's essential to keep your branch updated by regularly merging and pulling changes. This practice helps prevent merge conflicts down the line and ensures all team members are working with the most current code. Use the following command to pull the latest changes from the main branch:
git pull origin main
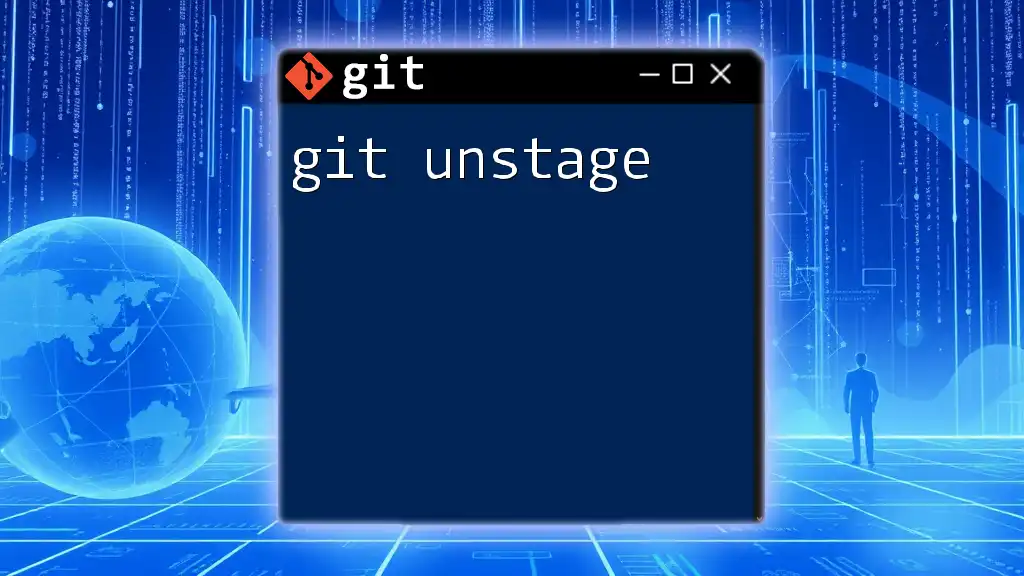
Troubleshooting Common Git Testing Issues
Resolving Merge Conflicts
While working in a collaborative environment, merge conflicts can happen when different changes are made to the same lines of code. Here’s how to effectively handle conflicts:
- Identify conflicting files listed by Git after attempting a merge.
- Open each conflicting file and look for `<<<<<<<`, `=======`, and `>>>>>>>` markers.
- Resolve the conflicts manually, and then stage and commit the resolved files.
Undoing Mistakes
Mistakes happen, and it’s crucial to know how to undo them effectively. The `git reset` and `git revert` commands allow you to remove or roll back changes safely.
Use `git revert` to create a new commit that undoes the changes made in a specific commit:
git revert HEAD
This command is safe and preserves the commit history, making it ideal for collaborative environments.
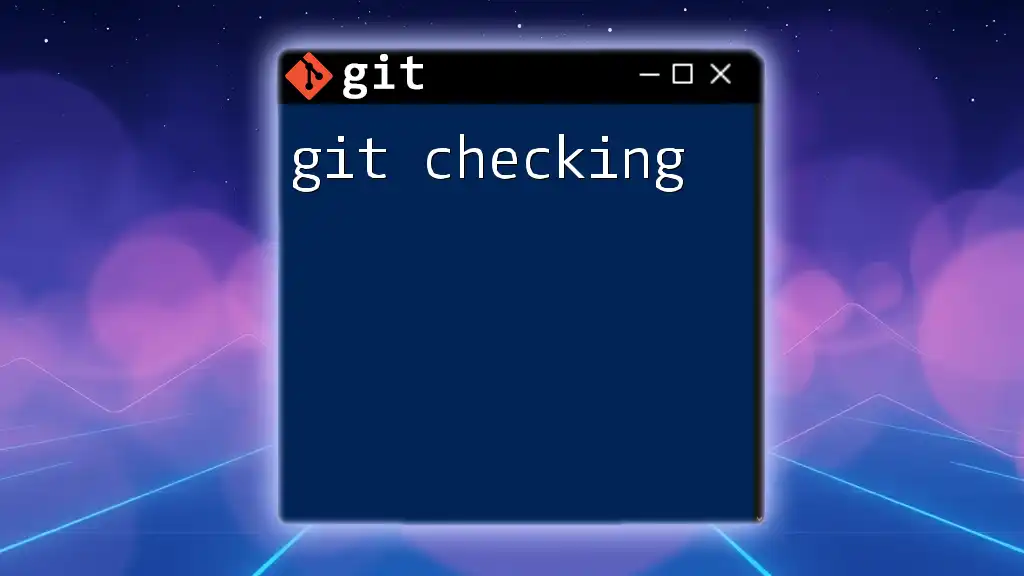
Conclusion
In summary, Git testing is a fundamental aspect of modern software development, enabling teams to maintain high-quality code. By understanding and utilizing Git's various commands and features, including branching, tagging, and integration with testing frameworks, developers can create a robust testing workflow. Remember, practice is crucial—apply what you've learned in real-world scenarios, and engage with communities to share your experiences and learn from others.