Git Jenkins is a powerful tool that integrates Git version control with Jenkins automation server, allowing developers to efficiently manage code changes and automate build processes.
Here's a simple Git command to clone a repository, which you might want to automate using Jenkins:
git clone https://github.com/yourusername/your-repo.git
Understanding Git
What is Git?
Git is a powerful distributed version control system that allows developers to track changes in their source code during software development. Unlike centralized version control systems, Git enables multiple developers to work on a project simultaneously without interfering with each other's work. This is achieved through features like branching and merging, which allow separate lines of development.
Basic Git Commands
To utilize Git effectively, you need to familiarize yourself with a few essential commands:
- `git init`: Initializes a new Git repository.
- `git clone <repository-url>`: Makes a copy of an existing repository.
- `git commit -m "commit message"`: Records changes to the repository with a descriptive message.
- `git push`: Sends committed changes to a remote repository.
- `git pull`: Fetches changes from a remote repository and merges them into your local branch.
These commands form the backbone of working with Git, enabling smooth collaboration and version control.
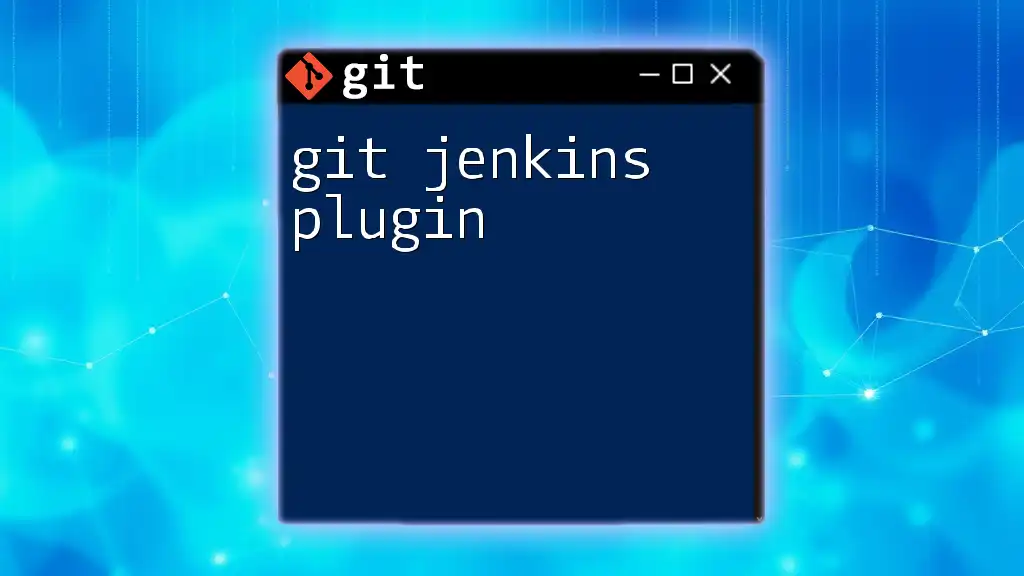
Introduction to Jenkins
What is Jenkins?
Jenkins is an open-source automation server that helps automate parts of the software development process. Its primary goal is to facilitate continuous integration and continuous delivery (CI/CD), ensuring that changes to code are automatically tested and deployed efficiently. The utilization of Jenkins in a development workflow can significantly enhance speed, reliability, and productivity.
Installing Jenkins
Before diving into using Jenkins with Git, it’s important to install it properly. Below are the steps for installing Jenkins on various platforms:
-
Installing on Windows: Download the Jenkins Windows installer from the official Jenkins site and run it. Follow the setup instructions to complete the installation.
-
Installing on macOS: Use Homebrew to install Jenkins:
brew install jenkins-lts
After installation, start Jenkins with:
brew services start jenkins-lts
-
Installing on Ubuntu/Linux: For Debian-based systems, use the following commands to install Jenkins:
wget -q -O - https://pkg.jenkins.io/debian/jenkins.io.key | sudo apt-key add - sudo sh -c 'echo deb http://pkg.jenkins.io/debian-stable binary/ > /etc/apt/sources.list.d/jenkins.list' sudo apt update sudo apt install jenkins
Configuring Jenkins
Once Jenkins is installed, it requires initial configuration. Open your browser and navigate to `http://localhost:8080` (or the respective URL if you’ve changed the default port). You'll be prompted to enter an admin password, which you can find in the installation logs.
After logging in, it’s crucial to set up security settings and create an admin user. Configure the recommended settings to secure your Jenkins environment.
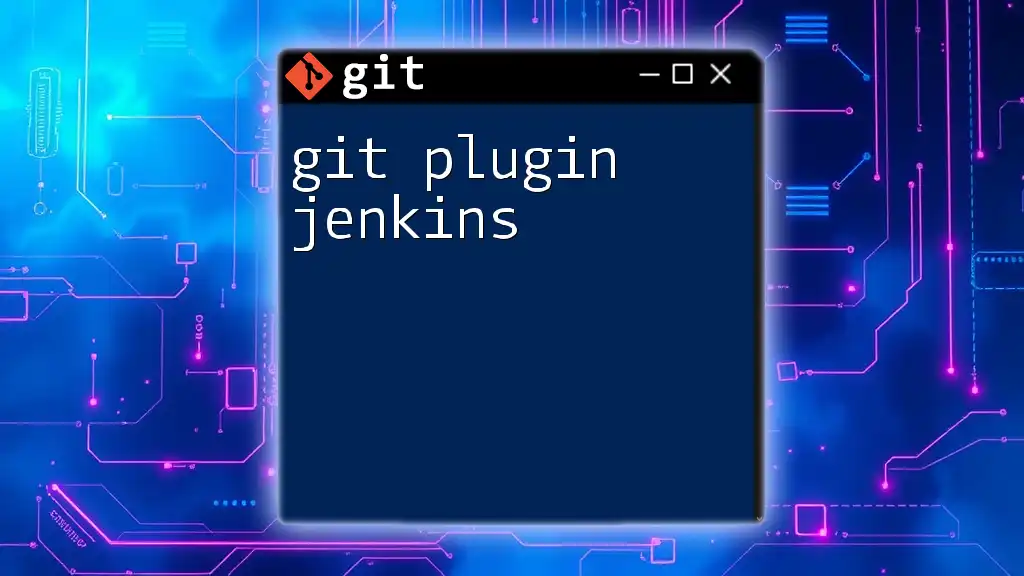
Integration of Git with Jenkins
Setting up Git in Jenkins
To integrate Git with Jenkins, it is necessary to enable Git within Jenkins. Here’s how:
- Go to Manage Jenkins → Manage Plugins and search for the Git Plugin. Ensure it is installed and up-to-date. This plugin allows Jenkins to interact with Git repositories.
Adding a Git Repository
To add a Git repository to your Jenkins job, follow these steps:
- Navigate to the Jenkins dashboard.
- Click on New Item and select a FreeStyle project.
- Name the project and click OK to proceed.
- Under the Source Code Management section, select Git.
- Enter the Git repository URL (for example, `https://github.com/user/repo.git`).
This configuration links your Jenkins job to your Git repository, allowing Jenkins to monitor changes.
Build Triggers for Git
Jenkins provides various build triggers to automate the build process. A popular method is setting up a Git webhook, which triggers a build whenever changes are pushed to the repository.
To configure this, add a webhook in your Git hosting service (e.g., GitHub or GitLab) pointing to your Jenkins server's URL ending with `/git/notifyCommit`. This setup allows Jenkins to listen for changes in real time, activating builds automatically.
Using Polling for Changes
If a webhook setup isn’t feasible, Jenkins can also poll for changes at regular intervals. You can specify this using a cron-style syntax in the Build Triggers section of your job configuration. For example:
H/5 * * * *
This configuration tells Jenkins to check for changes every 5 minutes.
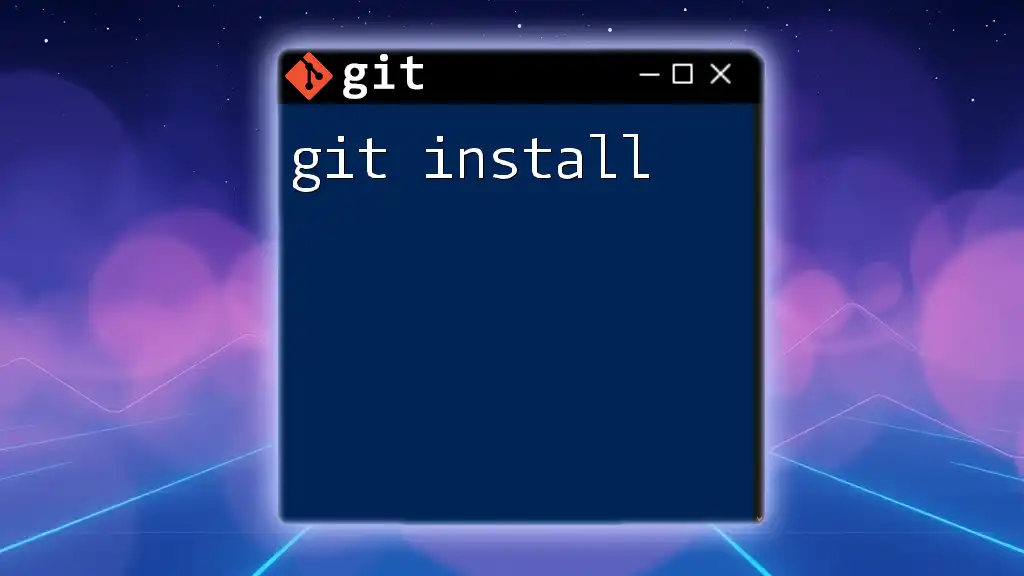
Building a Project with Jenkins and Git
Creating a Simple Build
After configuring your Git repository, proceed to create a simple build job:
- In your Jenkins project, scroll down to the Build section.
- Click on Add build step and select Execute shell or your preferred method based on your project requirements.
- Enter the build command to be executed, such as:
This command will compile and package a Maven project.mvn clean install
Executing Build Commands
Defining build steps in Jenkins is straightforward. Depending on your technology stack, you will customize the commands. For example, for a Node.js project, you might use:
npm install
npm run build
These commands install dependencies and build the application. Customizing build commands according to your project is crucial for Jenkins to execute the correct workflow.
Post-build Actions
After Jenkins completes the build, you can define post-build actions to specify what should happen next. Common actions include:
- Archiving artifacts by checking the Archive the artifacts checkbox and specifying files, such as:
target/*.jar
- Sending notifications, which can be set in the post-build actions to alert teams when a build succeeds or fails—integrating with services like email, Slack, or others.
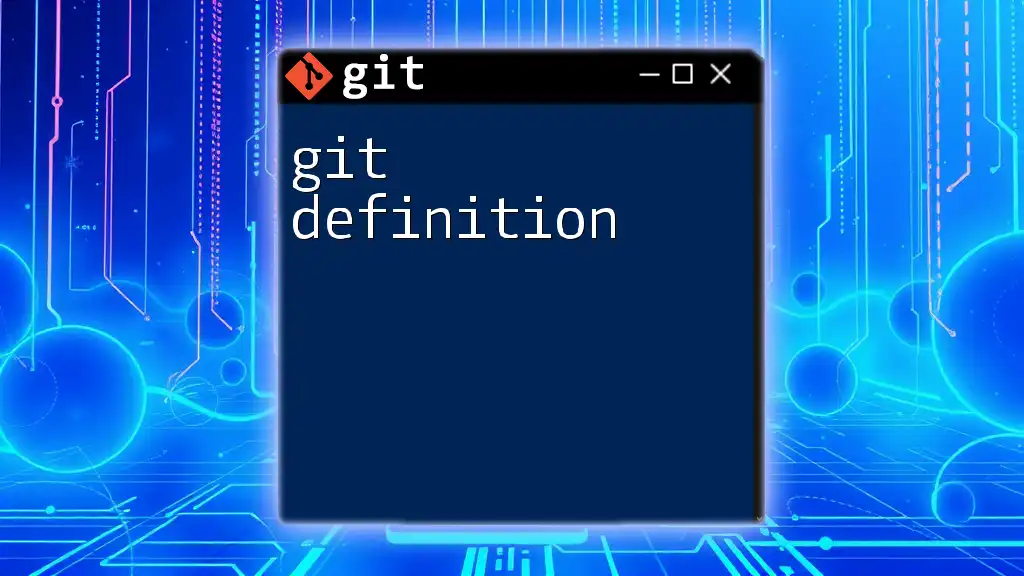
Advanced Features
Jenkins Pipeline with Git
A Jenkins Pipeline allows for more complex workflows and can be defined using a Jenkinsfile, which can reside in your Git repository.
Here’s a simple structure for a Jenkinsfile:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean install'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'deploy.sh'
}
}
}
}
This declarative syntax enables clear and structured build configurations.
Multibranch Pipeline
For projects with multiple branches, Jenkins supports a Multibranch Pipeline feature. This setup automatically creates jobs for each branch in a repository, triggering builds based on respective branch events.
To set it up, create a new item in Jenkins, select Multibranch Pipeline, and configure the branch sources. Jenkins will automatically detect branches and create jobs accordingly.
Testing and Deployment
Integrating automated tests within your Jenkins pipeline enhances quality control. You can integrate testing frameworks such as JUnit or Selenium to validate your code during the build process.
For instance, after your build step:
stage('Test') {
steps {
sh 'mvn test'
}
}
Successful builds can then trigger deployment steps to various environments directly from Jenkins, streamlining the release process.
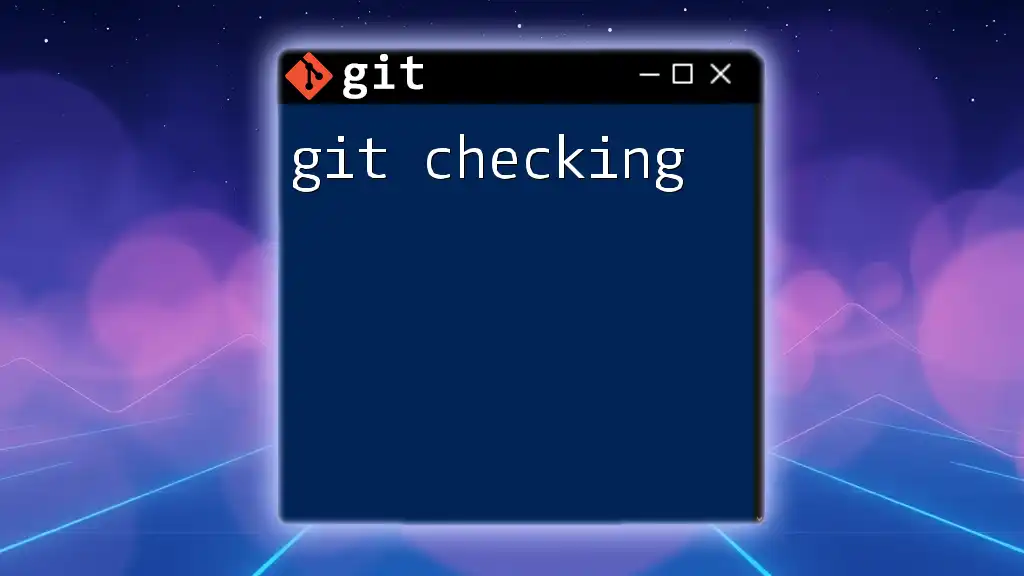
Troubleshooting Git and Jenkins Integration
Common Issues and Solutions
While working with Git and Jenkins, you may encounter common challenges, such as connectivity issues or build failures. Many of these can be resolved by checking:
- Repository URLs: Ensure the Git repository URL is correct and accessible from the Jenkins server.
- Credentials: Make sure the correct authentication credentials are configured in Jenkins.
- Network Issues: Check firewall settings that may prevent Jenkins from accessing Git servers.
Best Practices
To maintain a robust integration between Git and Jenkins, consider these best practices:
- Branch Strategy: Adopt effective branching strategies (e.g., Git Flow) to organize your commits efficiently.
- Consistent Commit Practices: Encourage developers to commit frequently with descriptive messages—this enhances traceability.
- Automated Testing: Regularly include automated tests in your pipeline to catch issues early in the development cycle.
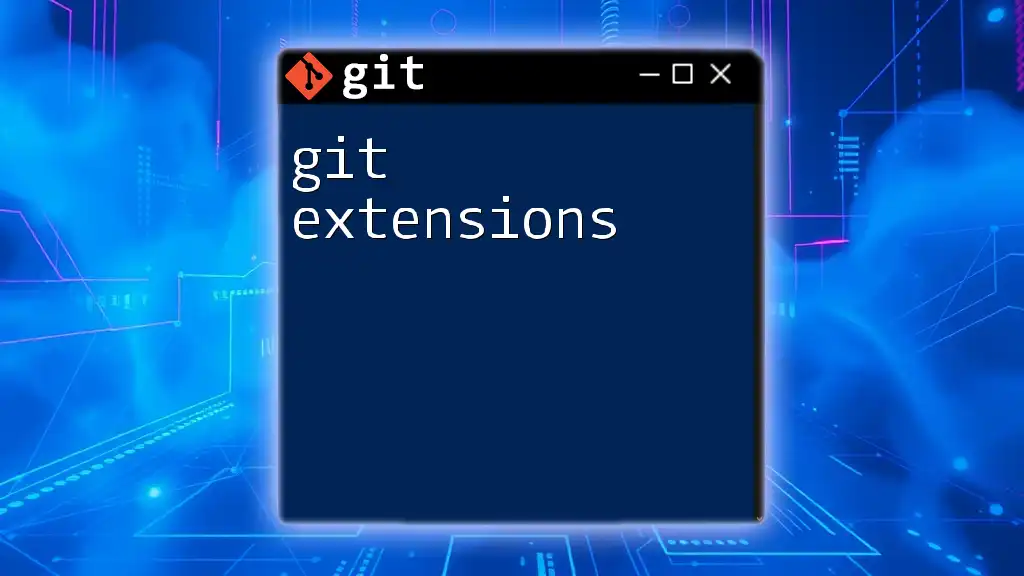
Conclusion
Mastering the integration of Git and Jenkins can transform your development workflow, allowing for faster and more reliable software delivery. By understanding and implementing the concepts outlined in this guide, you will position your team for success in a competitive software development landscape. Embrace the power of Git and Jenkins, and watch your productivity soar.
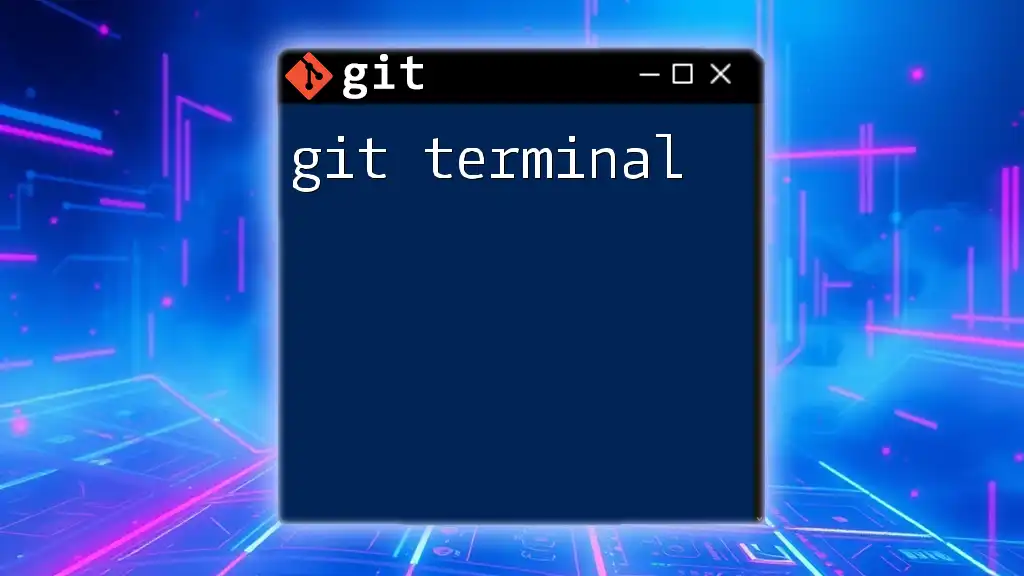
Resources and Further Learning
To deepen your knowledge of Git and Jenkins, explore the following resources:
- [Official Git Documentation](https://git-scm.com/doc)
- [Jenkins User Documentation](https://www.jenkins.io/doc/)
- Recommended tutorials and courses on platforms like Codecademy, Udemy, or freeCodeCamp for hands-on learning experiences.