The Jenkins Git plugin enables Jenkins to access and manage Git repositories, allowing seamless integration of version control within continuous integration and deployment pipelines.
Here's a simple code snippet to demonstrate how to use Git in a Jenkins pipeline:
pipeline {
agent any
stages {
stage('Clone Repository') {
steps {
git 'https://github.com/username/repository.git'
}
}
stage('Build') {
steps {
sh 'make' # Replace with your build command
}
}
}
}
What is the Jenkins Git Plugin?
The Jenkins Git Plugin is an essential tool that integrates Git version control directly into Jenkins, the popular open-source automation server known for continuous integration and continuous delivery (CI/CD). By leveraging the Jenkins Git Plugin, developers can automate the process of retrieving source code from their Git repositories, enabling a smooth workflow in software development.
Key Features
The Jenkins Git Plugin offers several valuable features, which include:
- Support for Multiple Repositories: It allows users to connect to multiple Git repositories, making it versatile for various projects.
- Polling and Webhooks: Users can set up notifications for changes in their repositories, triggering builds whenever a new commit is made.
- Integration with Popular Services: This plugin works seamlessly with Git hosting platforms such as GitHub, GitLab, and Bitbucket, allowing users to maintain their workflows without interruption.
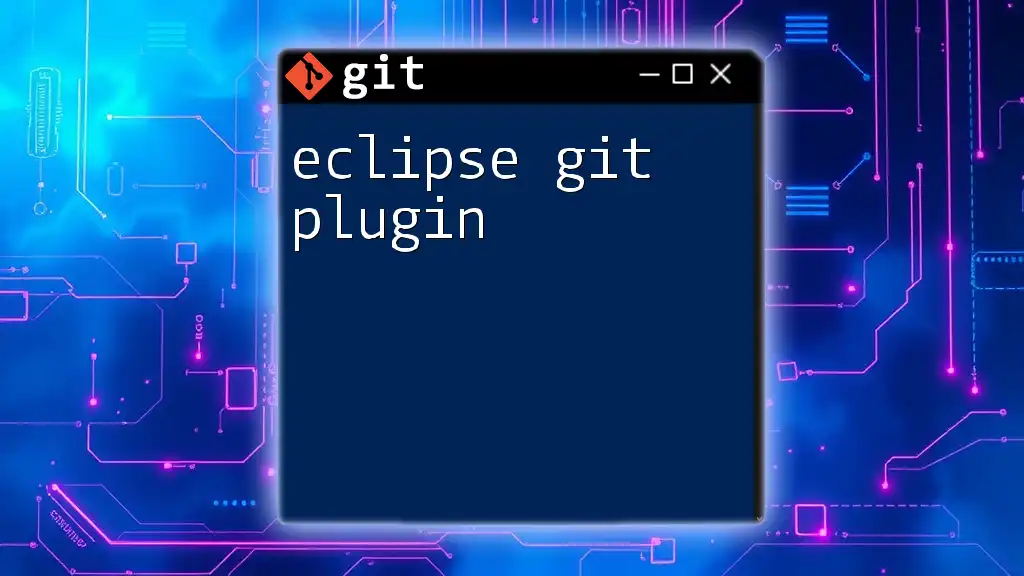
Installing the Jenkins Git Plugin
Prerequisites
Before installing the Jenkins Git Plugin, ensure you have Jenkins installed and running on your server. Additionally, you should have the necessary permissions to manage plugins.
Installation Steps
To install the Jenkins Git Plugin, follow these steps:
- Navigate to Jenkins: Go to your Jenkins dashboard.
- Manage Plugins: Click on "Manage Jenkins," then choose "Manage Plugins."
- Search for the Git Plugin: Within the "Available" tab, type "Git Plugin" in the search bar.
- Install the Plugin: Check the box next to the Jenkins Git Plugin and click on "Install without restart."
After installation, you will see a confirmation message indicating that the plugin has been successfully added.
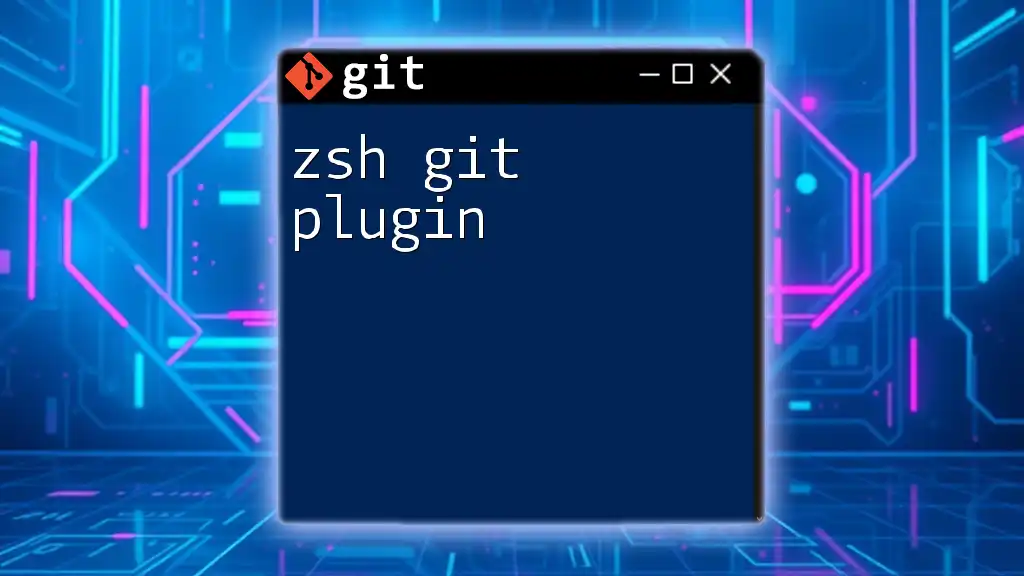
Configuring the Jenkins Git Plugin
Global Configuration Settings
Setting up the Jenkins Git Plugin involves configuring several global settings that ensure it behaves as expected.
- Git Executable Path: Make sure the path to the Git executable is correctly set. This configuration is found under "Manage Jenkins" > "Global Tool Configuration."
- Default Git Behaviors: Adjust various settings related to clone timeout, remote branch tracking, and other Git behaviors as per your project's needs.
Job Configuration
Once the global settings are in place, you can define a new Jenkins job to utilize the Git integration.
-
Create a New Job: On the Jenkins dashboard, click "New Item" and select a job type (e.g., Freestyle project).
-
Source Code Management (SCM): In the job configuration, select "Git" as your SCM type.
-
Repository URL: Insert your Git repository URL. For example:
https://github.com/username/repository.git
-
Credentials Management: Utilize Jenkins' built-in credentials management to securely store your Git credentials, enabling seamless access to private repositories.
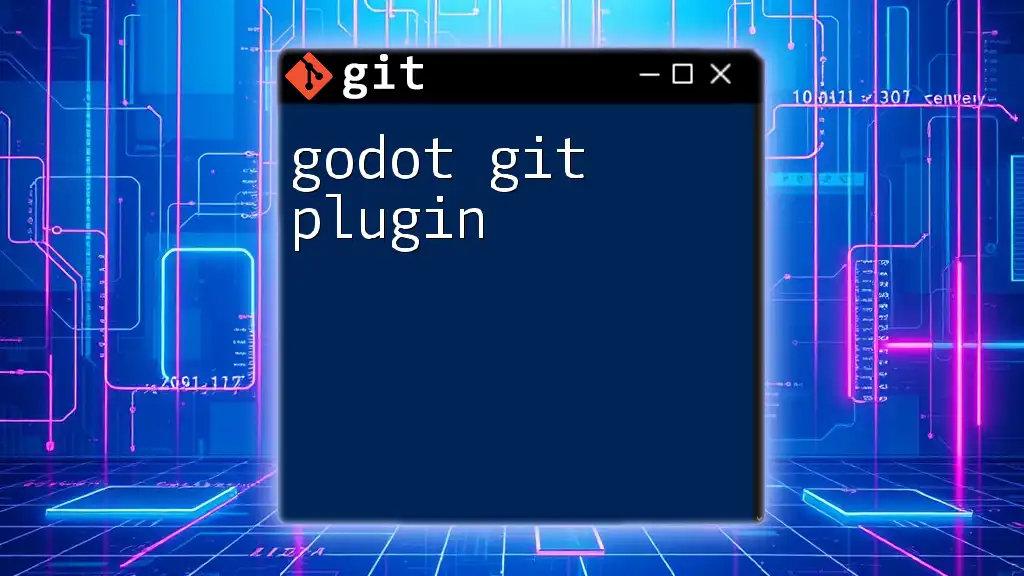
Using the Jenkins Git Plugin in Your CI/CD Pipeline
With the Jenkins Git Plugin configured, you can start automating your pipeline.
Triggering Builds
Two primary methods for triggering builds when changes occur are:
-
Polling: Configure Jenkins to periodically check for changes in your Git repository. You can set this up in your job configuration under Build Triggers by enabling the "Poll SCM" option, adding a schedule like `H/5 * * * *` to poll every five minutes.
-
Webhooks: Many Git hosting services support webhooks, allowing you to configure an HTTP POST request to your Jenkins instance whenever a change occurs. This method is more efficient because it avoids unnecessary polling.
Example Pipelines with Git
You can define a Jenkinsfile to specify your CI/CD pipeline, incorporating Git commands to streamline your processes. Here is an example Jenkinsfile:
pipeline {
agent any
stages {
stage('Checkout') {
steps {
git url: 'https://github.com/username/repository.git', branch: 'main'
}
}
stage('Build') {
steps {
// Your build commands here, e.g., executing a script
sh 'echo Building project...'
}
}
stage('Test') {
steps {
// Running tests
sh 'echo Testing project...'
}
}
stage('Deploy') {
steps {
// Deploying code
sh 'echo Deploying project...'
}
}
}
}
In this example, Jenkins checks out the code from the repository and proceeds through the build, test, and deploy stages, all integrated with Git.
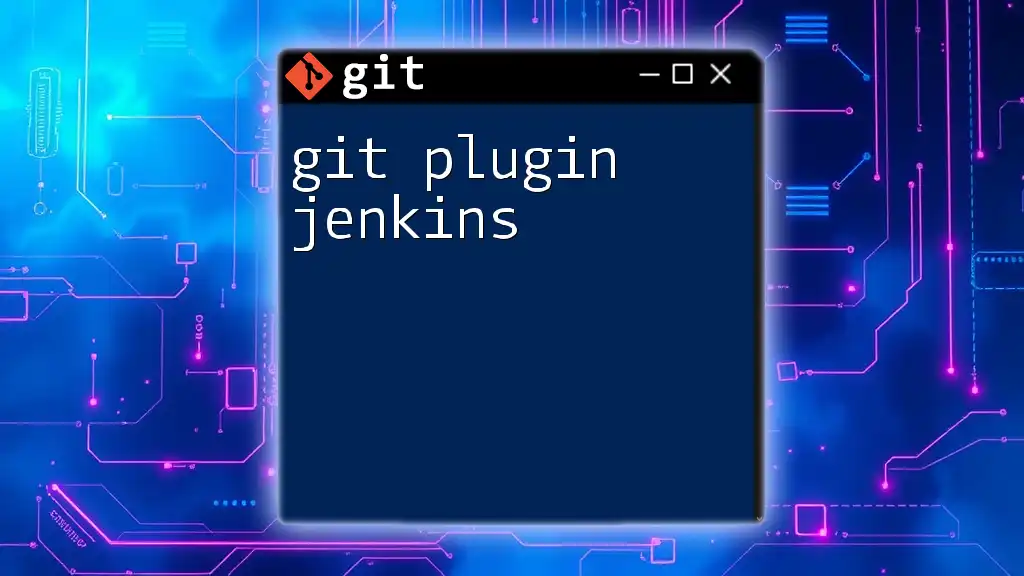
Common Commands and Scenarios
Basic Git Commands in Jenkins
The Jenkins Git Plugin enables several essential Git commands:
- Pulling Code: Retrieves the latest changes from the repository.
- Cloning Repositories: Allows Jenkins to create a local copy of the repository during the build.
- Fetching Updates: Checks for changes in the remote repository without merging them.
Handling Merge Conflicts
When a build fails due to a merge conflict, Jenkins will provide logs that detail the conflict. To manage this:
- Review the build logs to understand which files are conflicting.
- Resolve the conflicts locally and push the resolved code back to the repository.
- Rerun the Jenkins job after resolving the conflict.
Testing Pull Requests
Integrating pull request testing is crucial for maintaining code quality. You can create a job that triggers when a pull request is opened or updated by configuring GitHub or GitLab webhooks.
Using declarative pipelines, you can easily set this up:
pipeline {
agent any
stages {
stage('Pull Request Check') {
steps {
script {
if (env.BRANCH_NAME == 'PR-1') {
// Execute tests specifically for the pull request
sh 'echo Running tests for Pull Request...'
}
}
}
}
}
}
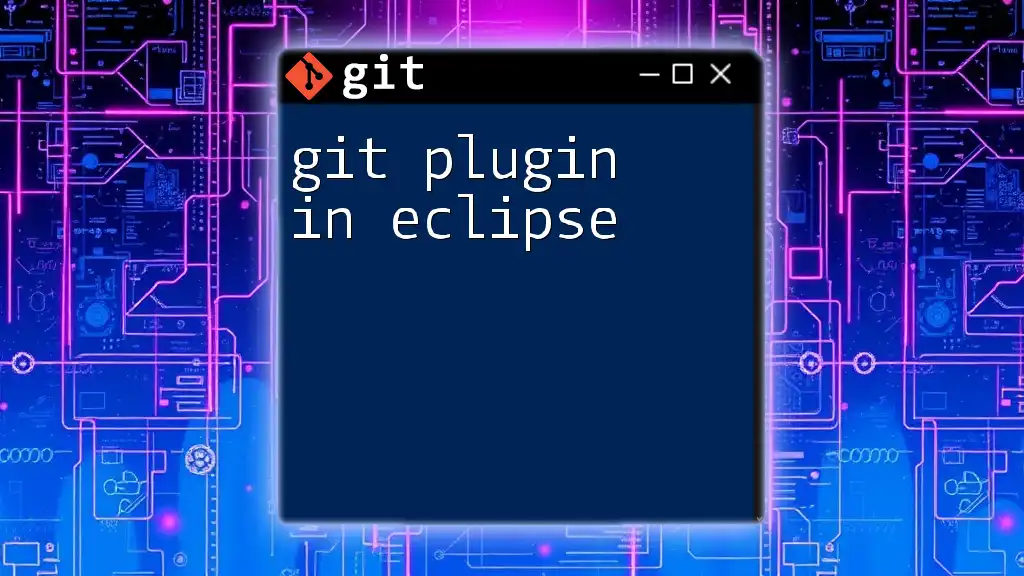
Troubleshooting Common Issues
Plugin Errors and Solutions
Even with the Jenkins Git Plugin, errors can occur. Common issues include:
- Authentication Failures: Ensure that your credentials in Jenkins are correct.
- Invalid Repository URL: Double-check the repository URL for typos.
Connectivity Issues
If Jenkins cannot connect to your Git repository, verify:
- Network settings, such as firewalls or VPNs that might be hindering access.
- Ensure the Jenkins server itself has the rights and access to the Git repositories.
Performance Considerations
To enhance the performance of Git operations in Jenkins:
- Reduce the polling frequency when unnecessary, as this might strain resources.
- Regularly prune old builds and logs to maintain a clean environment.
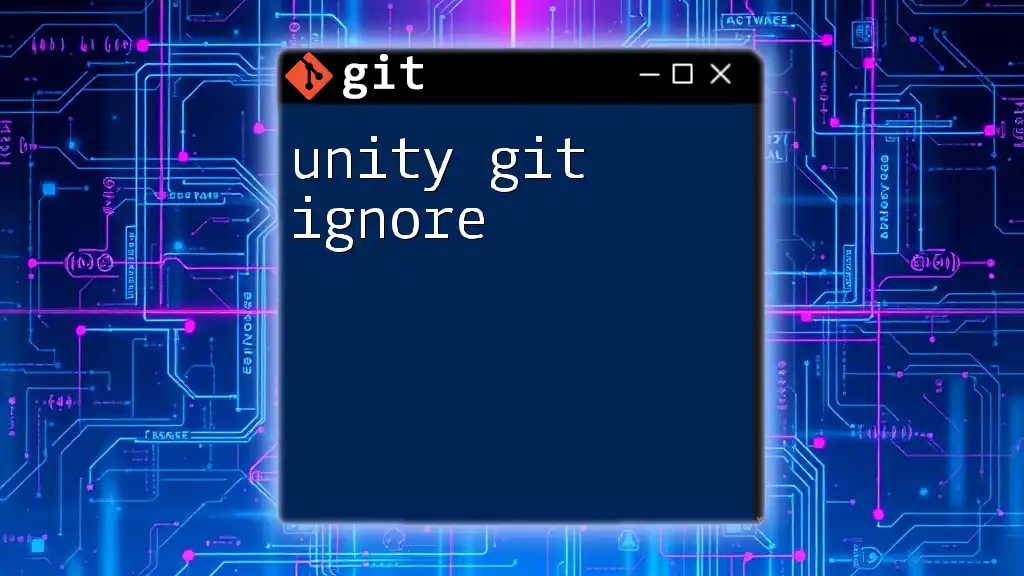
Advanced Features of the Jenkins Git Plugin
Using Git Submodules
When working with projects that have dependencies on other repositories, Git submodules can be used. To configure submodules in your Jenkins job, enable the "Advanced" settings in the "Git" SCM section, where you can specify the handling of submodules.
Tagging and Versioning Builds
Automate versioning within your Jenkins pipelines by integrating tagging methods. For example, you can add a stage in your Jenkinsfile to create a new tag based on the build number:
pipeline {
...
stages {
...
stage('Tag Release') {
steps {
script {
def newTag = "v${env.BUILD_NUMBER}"
sh "git tag -a ${newTag} -m 'Release version ${newTag}'"
sh "git push origin ${newTag}"
}
}
}
}
}
This example creates a new tag each time a successful build is made, helping in tracking versions over time.
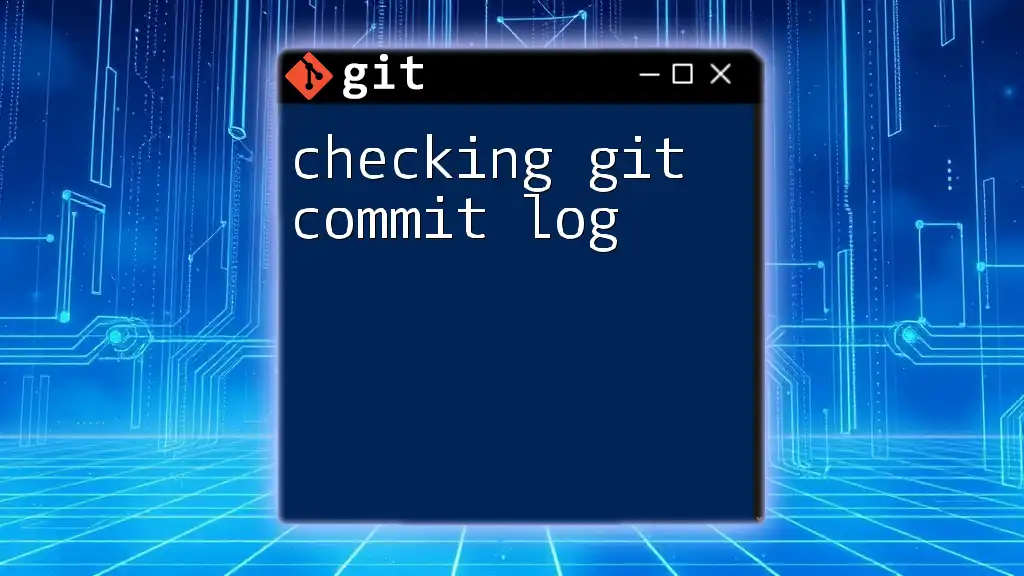
Conclusion
The Jenkins Git Plugin is an invaluable tool for automating the integration of Git repositories into CI/CD workflows. It simplifies code management, allows for sophisticated build configurations, and enhances the overall development experience. By understanding its features and configuration steps, you'll be able to leverage the full potential of automated workflows and continuous integration.
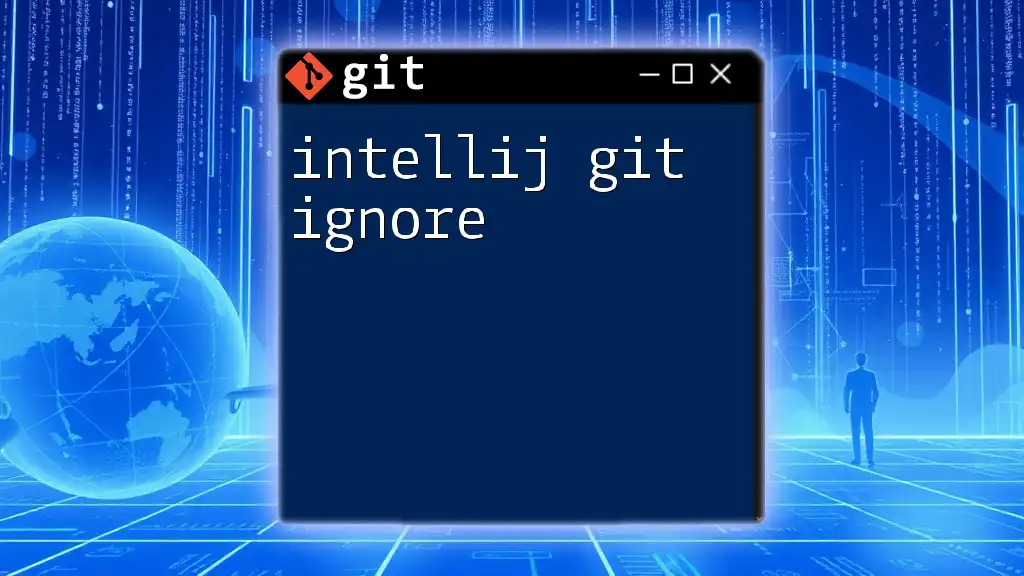
Further Resources
For more information, check the following resources:
- Official Jenkins Git Plugin documentation
- Git and Jenkins learning materials from online platforms
- Community-driven forums and discussion boards for support and best practices
By integrating the Jenkins Git Plugin into your development process, you are taking a significant step towards a more efficient and automated coding environment!