To check the Git commit log, use the `git log` command, which displays a list of all the commits in the repository along with their details.
git log
Understanding Git Commit Logs
What is a Git Commit?
A commit in Git represents a snapshot of your project at a certain point in time. It's an essential part of version control, as it records changes made to files in your repository, allowing you to track progress, collaborate with others, and revert to previous states if necessary. Each commit saves the state of all your files, providing a historical context for your development work.
Structure of a Git Commit
Every commit in Git has a specific structure consisting of several key components:
- Hash: A unique identifier for each commit (e.g., `d2c981e`).
- Author: The person who made the commit, typically displayed with their name and email.
- Date: Timestamp indicating when the commit was created.
- Message: A brief description summarizing the changes made.
An example of a commit output might look like this:
commit d2c981e6a57951e94d2549ed6b938c56fa431e17
Author: Jane Doe <jane@example.com>
Date: Tue Sep 26 14:35:52 2023 -0700
Fix bug in user authentication
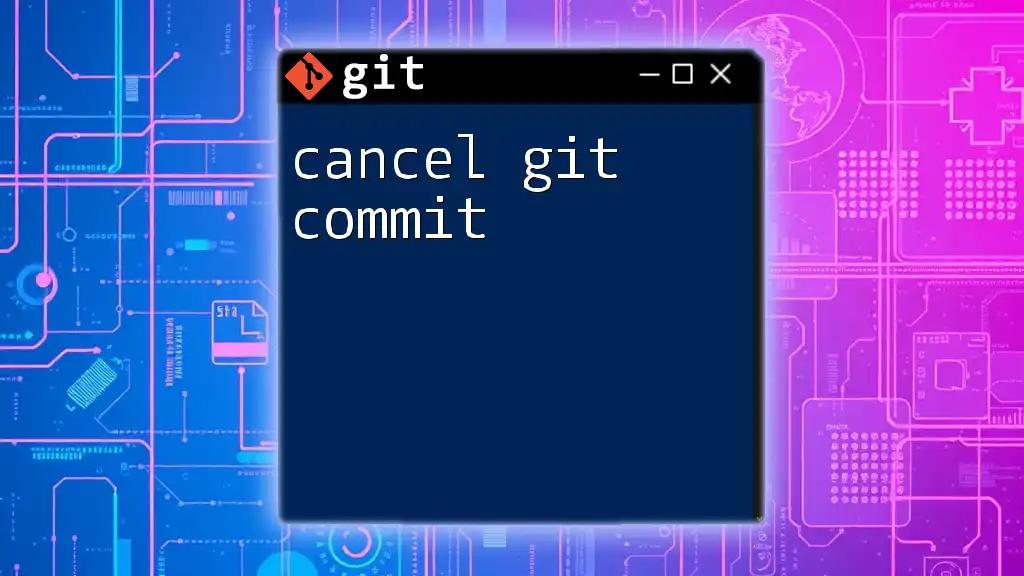
Checking the Commit Log
The `git log` Command
To view the commit history in your repository, you use the `git log` command. This command retrieves and displays the log of commits made in the current branch, enabling you to review past work.
You can execute the command simply with:
git log
By running this command, you'll see the detailed history of your commits, including the hashes, authors, dates, and messages.
Formatting the Log Output
Default Log Format
By default, `git log` presents a lot of information in a structured format. However, sometimes you may want a clearer view that's easier to navigate.
Customizing Output with Options
-
`--oneline`: This option condenses each commit to a single line. It’s particularly useful when you want a quick overview of the commit history.
git log --oneline
-
`--graph`: When you want to see a visual representation of both branches and merges, this flag is your best friend. It adds a graphical representation of the commit history alongside the commit messages.
git log --graph
-
`--stat`: If you're interested in knowing which files were changed in each commit and the number of lines added/removed, the `--stat` option provides that detail.
git log --stat
Filtering Commits
By Author
You can narrow down the commit log by specific authors using the `--author` flag. This is especially handy when collaborating in a team setting.
git log --author="Author Name"
By Date
Filtering commits by date range helps you focus on a specific period. The flags `--since` and `--until` facilitate this.
git log --since="2023-01-01" --until="2023-01-31"
This command will return commits made only during January 2023.
Searching for Specific Commits
By Commit Message
Looking for specific changes or bug fixes can be done efficiently using the `--grep` flag to search through commit messages.
git log --grep="fix bug"
This will display all commits that contain the phrase "fix bug" in their messages.
By File Changes
To examine the changes made to a particular file, you can view its history by specifying the file path:
git log -- path/to/file
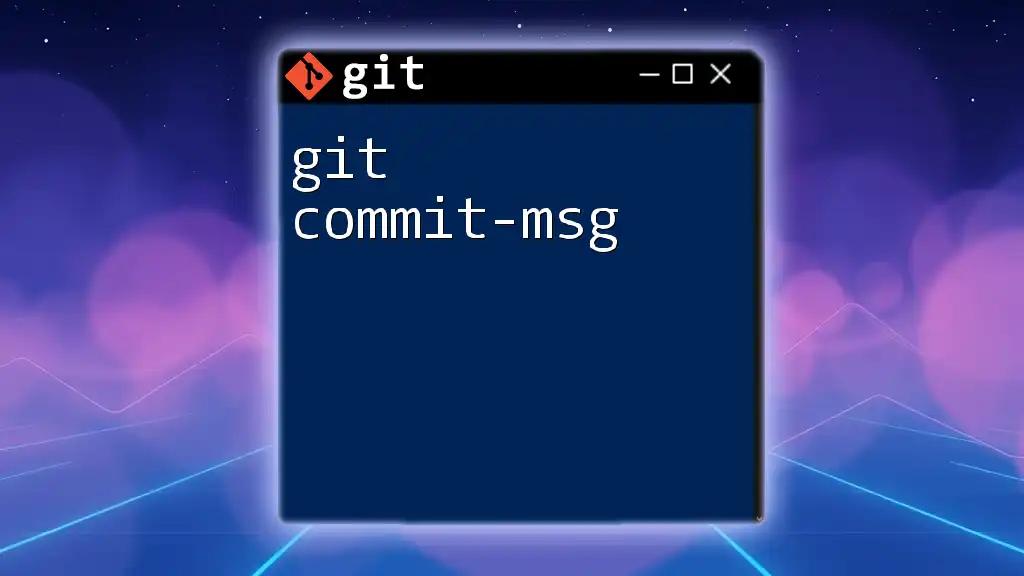
Advanced Log Options
Pretty Formatting
For users who need more tailored outputs, the `--pretty` option allows you to customize the output format of the log.
git log --pretty=format:"%h %s (%an, %ar)"
This example will display each commit's short hash, message, author name, and relative date.
Limiting Number of Commits
If you're only interested in the most recent commits, you can limit the output using the `-n` option.
git log -n 5
This command will show only the last five commits.
Showing Patch Changes
To visualize the changes made in each commit, the `-p` option can be used. This shows the changes introduced in each commit along with the commit message.
git log -p
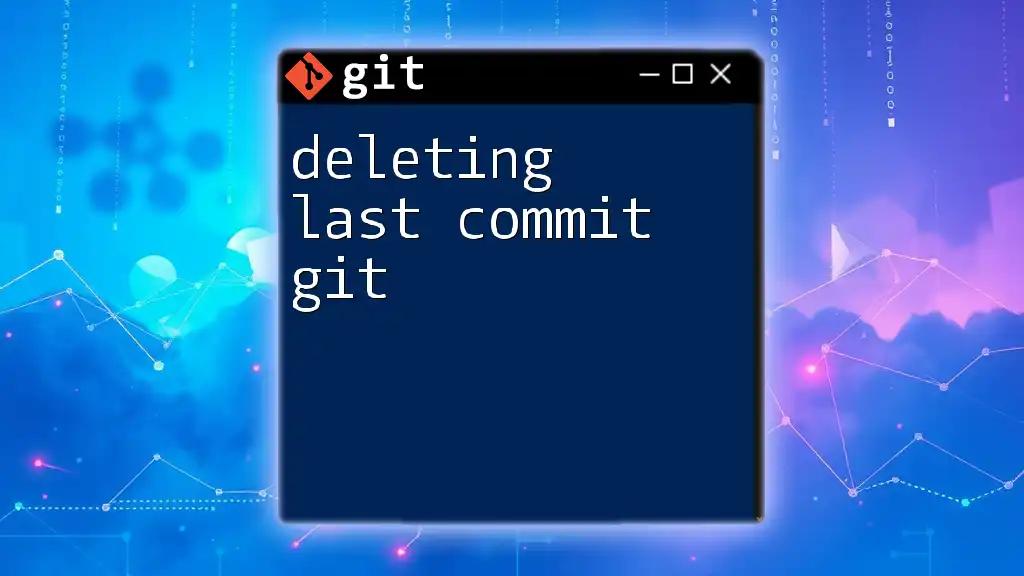
Using Aliases for Efficiency
Creating Custom Git Aliases
To save time, especially if you frequently check the commit log, consider creating custom Git aliases. Aliases allow you to set shortcuts for common commands.
For instance, you might want to create an alias for a quick log view:
git config --global alias.lg "log --oneline --graph"
Using this alias, you can now check the log simply by typing:
git lg
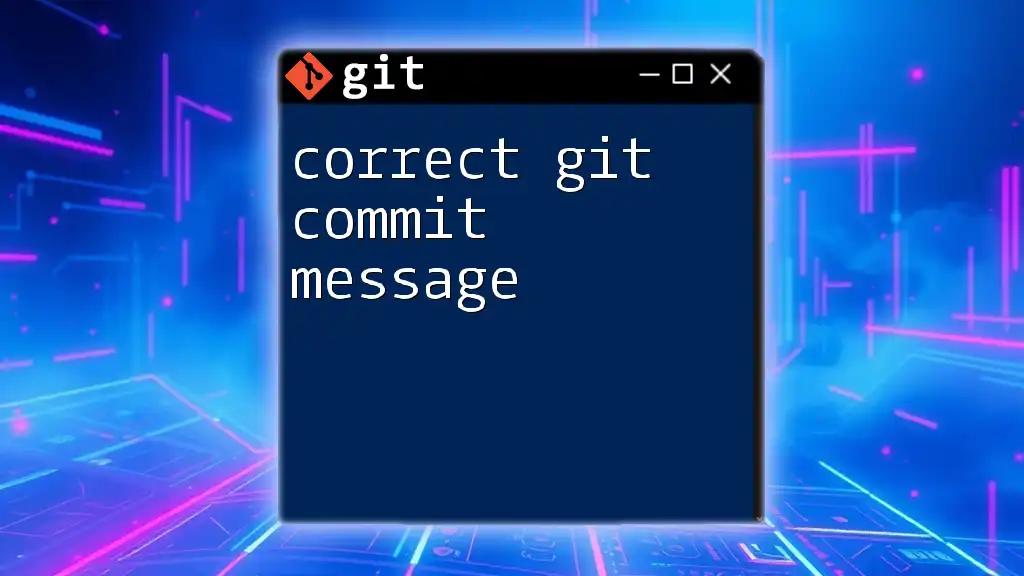
Practical Tips for Effective Log Checking
Reviewing Commit History Regularly
Make it a habit to check your commit history regularly. This keeps you informed about what has been done and allows you to reflect on past decisions and changes made throughout the project lifecycle.
Collaborating with Teams
Effective use of commit logs can significantly enhance collaboration. Encourage your team to write meaningful commit messages, as clear messages can help others (and your future self) understand the context behind changes.
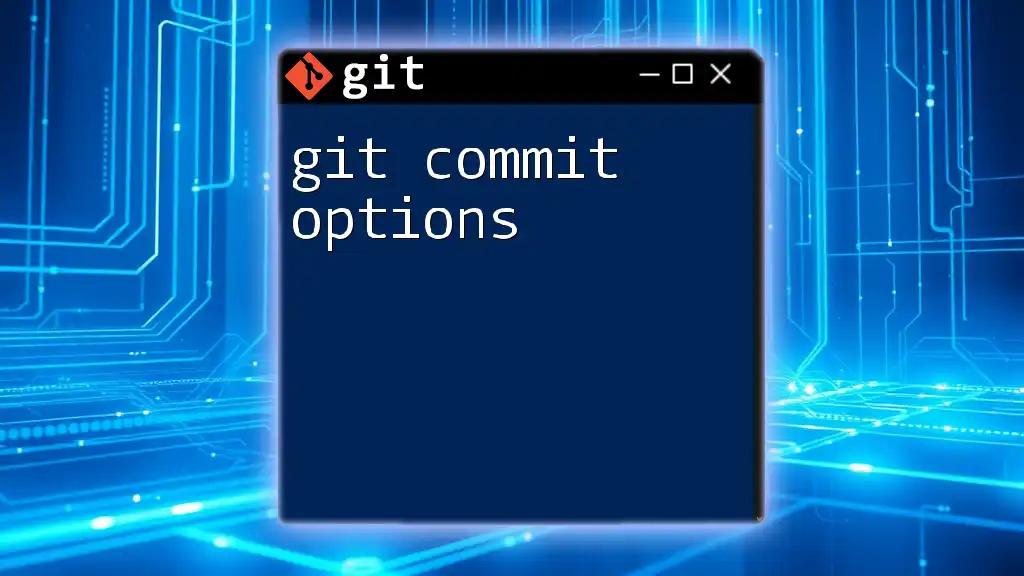
Conclusion
Understanding how to efficiently check Git commit logs is crucial in making the most out of Git's version control capabilities. By mastering the `git log` command and its various options, you will enhance your workflow and maintain a clear understanding of your project's history. For deeper insights and hands-on practice, consider joining our course where we dive deeper into Git commands and best practices for effective version control!
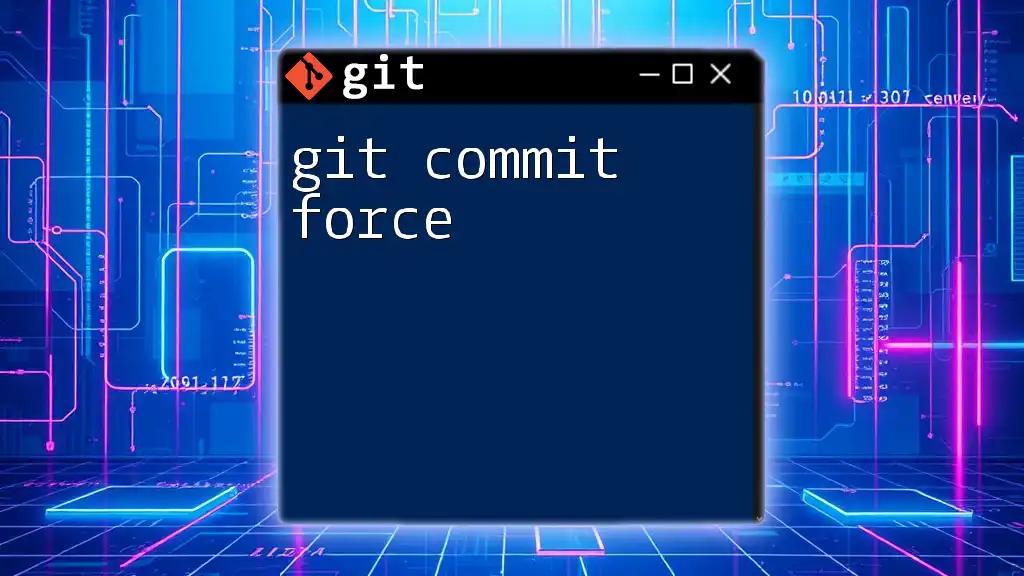
Additional Resources
For further learning, explore recommended books or online courses specific to Git and version control. Official Git documentation is also a treasure trove of information, ensuring that you can always find the most accurate and updated guidance.