Python developers often use Git for version control to manage changes in their code effectively, ensuring collaboration and tracking of their project progress.
Here’s a simple Git command to add and commit changes in a Python project:
git add my_script.py
git commit -m "Fix bug in data processing function"
Understanding Git Basics
What is Git?
Git is a distributed version control system that allows multiple developers to work on a project simultaneously without overwriting each other's changes. It tracks modifications to files over time, enabling you to revert to previous versions if needed. Using Git in programming, especially with Python, is vital for managing source code, collaborating with others, and maintaining a clean history of project changes.
Setting Up Git
Setting up Git is the first step towards integrating it into your Python projects. Installation varies based on the operating system:
- Windows: Download the Git installer and follow the setup wizard.
- macOS: You can install Git using Homebrew with the following command:
brew install git
- Linux: Use the package manager for your distribution:
sudo apt-get install git # For Debian/Ubuntu sudo yum install git # For CentOS/RHEL
Creating Your First Repository
To begin utilizing Git, you need to create a Git repository. This can be done either by initializing a new repository or cloning an existing one. Here’s how to create a new repository:
git init my-python-project
This command creates a new directory named `my-python-project`, initializing it for Git use. Understanding the workflow of Git is crucial, which typically involves staging changes, committing them, and finally pushing them to a remote repository.
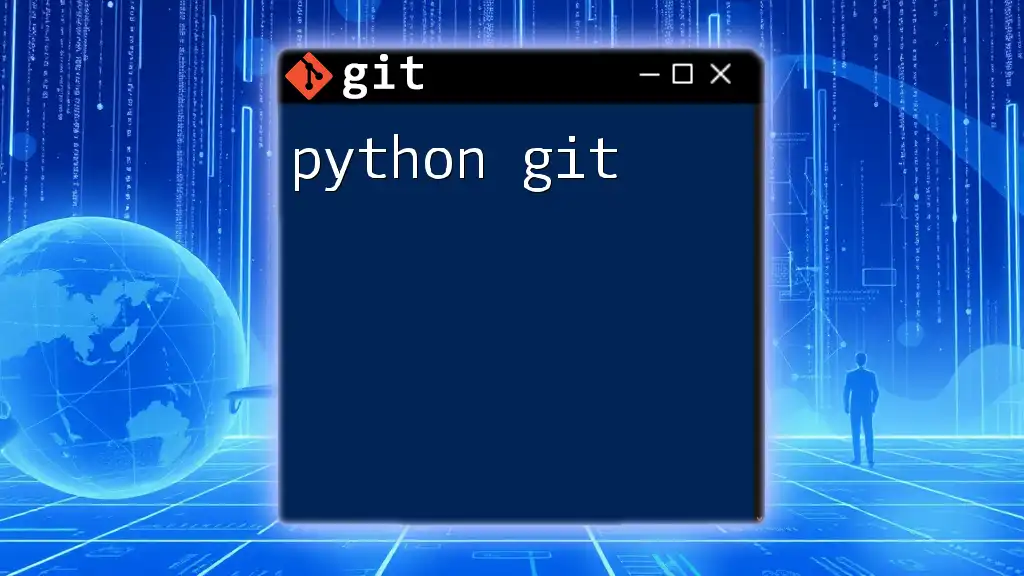
Setting Up a Python Project
Creating a Virtual Environment
Using a virtual environment is essential in Python projects to manage dependencies effectively. This isolates your project’s libraries from the global Python environment, preventing conflicts.
To create a virtual environment, use the following command:
python -m venv venv
Activate the environment with the appropriate command:
source venv/bin/activate # On macOS/Linux
venv\Scripts\activate # On Windows
Once activated, installing libraries within this environment keeps your project tidy.
Installing Dependencies
Managing dependencies with a `requirements.txt` file is a best practice in Python development. This file lists all the packages your project depends on, allowing others (or you in the future) to replicate the environment easily. To create a `requirements.txt` file with currently installed dependencies, run:
pip freeze > requirements.txt
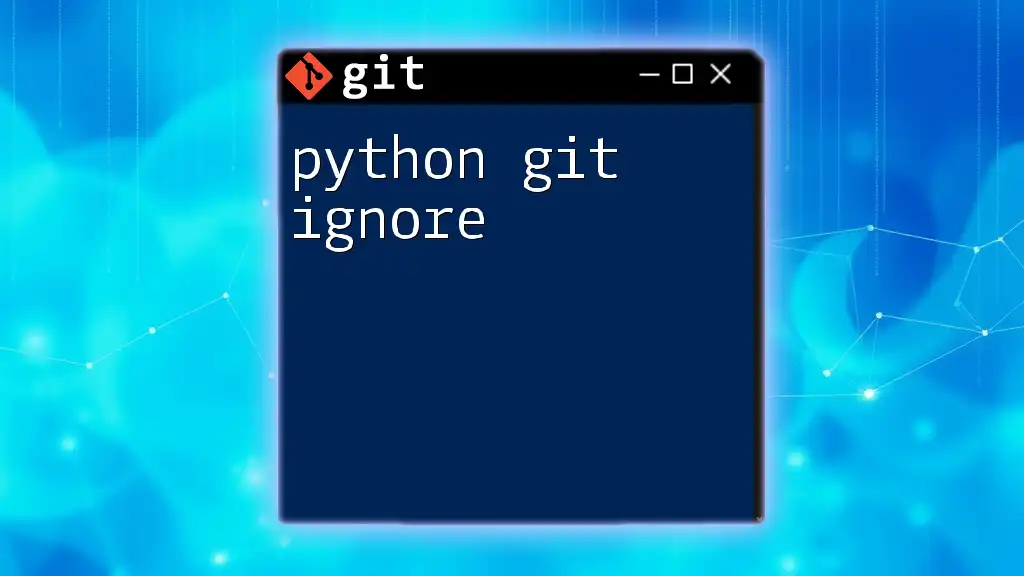
Integrating Git into Your Python Workflow
Initializing Git in Your Python Project
Once your Python project is set up, you’ll want to initialize Git within it. Navigate to your project’s directory and execute:
git init
This command sets up the necessary Git files that allow version control.
Best Practices for Git in Python Development
Effective Git usage involves writing clear and descriptive commit messages that explain what changes were made and why. This habit not only helps you track the evolution of your project but also assists team members in understanding the context of changes.
Moreover, structuring commits around specific features or bug fixes keeps your history clean and comprehensible.
Ignoring Files with .gitignore
Utilizing a `.gitignore` file is crucial to prevent unwanted files from being tracked by Git. This file tells Git which files or directories to ignore in a repository. Typical entries in a Python project might include:
__pycache__/
*.pyc
venv/
Creating a `.gitignore` file in your repository ensures that only the necessary files are versioned, keeping the commit history relevant and uncluttered.
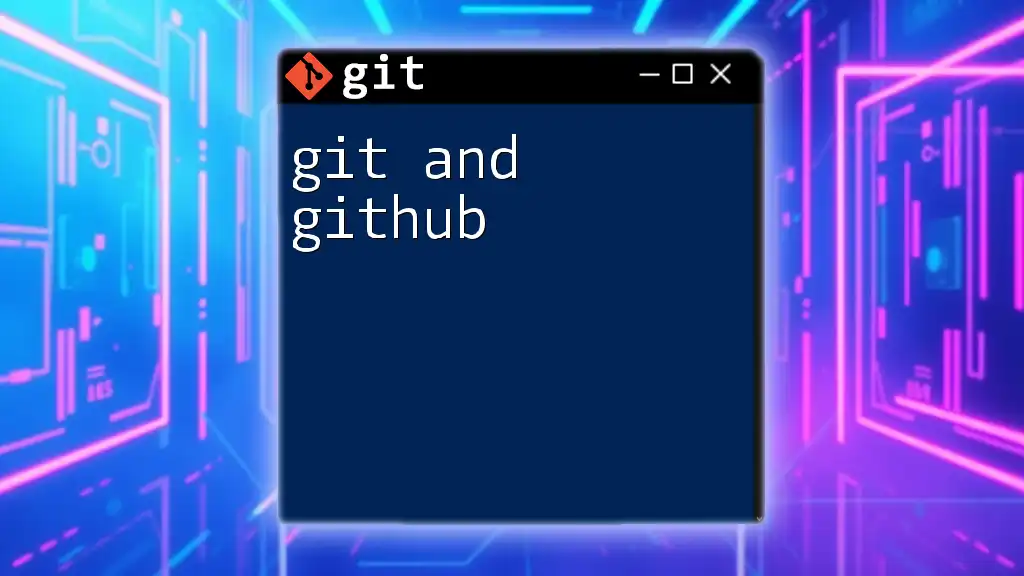
Working with Branches
Creating and Using Branches
Branching allows you to work on new features or bug fixes in isolation. To create a new branch, you use:
git checkout -b feature/new-feature
This command not only creates the branch but also switches you to it, encouraging a focused workflow without disrupting the main codebase.
Merging Branches
Once your feature is complete, merging it back into the main branch is the next step. Switch back to the main branch usually named `main` or `master`:
git checkout main
Then, execute the merge command:
git merge feature/new-feature
This integrates all changes made in the `feature/new-feature` branch into the main branch without losing the history of the feature branch.
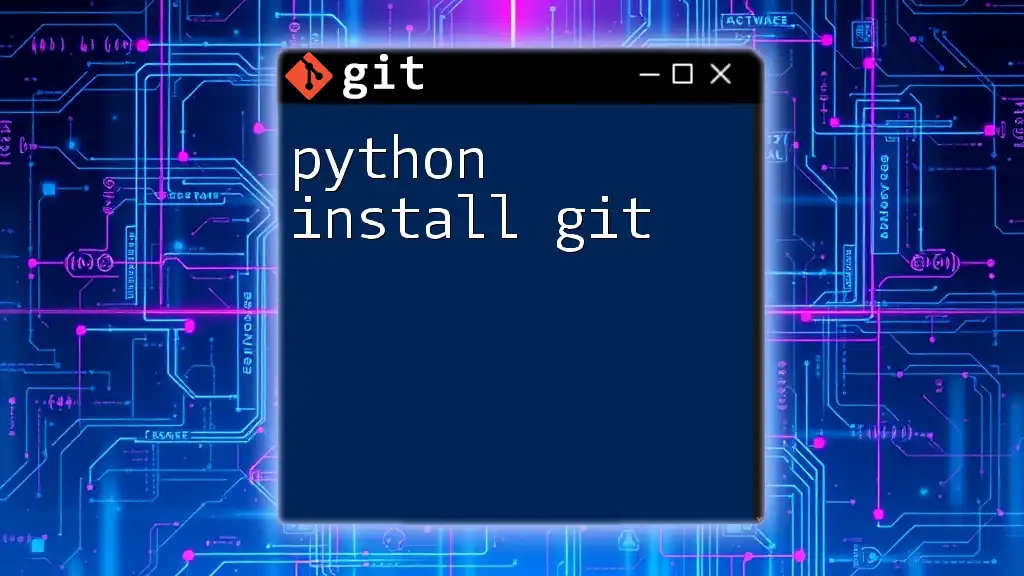
Collaborating with Git
Cloning a Repository
Collaboration often begins with cloning an existing repository to your local machine. To clone a repository, use the following command:
git clone https://github.com/username/repo.git
This command creates a copy of the repository, complete with its history, on your local machine, allowing you to contribute effectively.
Understanding Pull Requests
Pull Requests (PRs) are essential for collaboration, particularly in environments like GitHub. A pull request initiates a discussion about changes you want to merge into the main branch of a project. Creating a PR allows team members to review code changes, suggest modifications, or approve them for merging, facilitating teamwork and enhancing code quality.
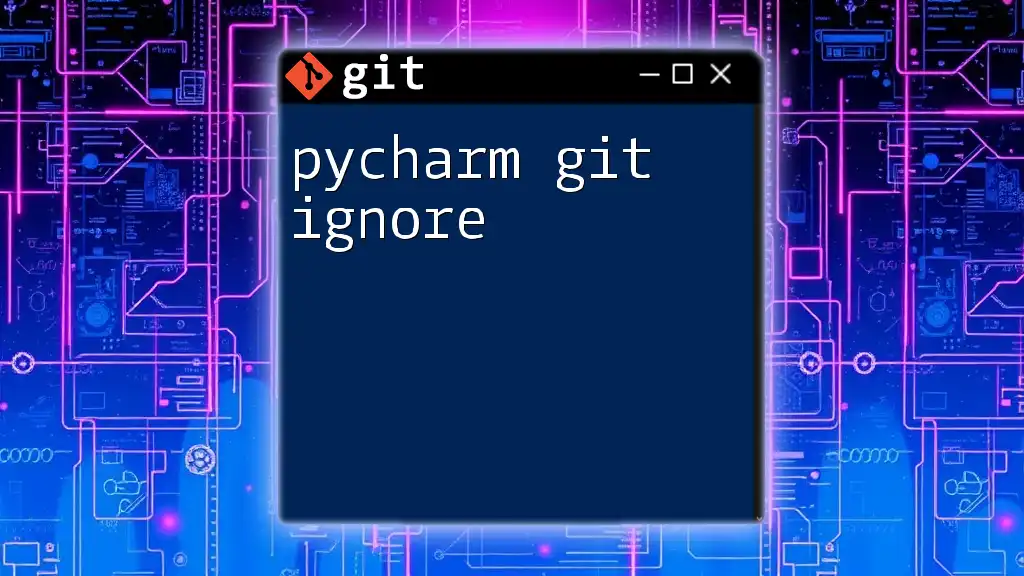
Common Git Commands for Python Developers
Status and Logs
Monitoring the state of your repository is crucial for effective version control. The command `git status` helps you keep track of which changes have been staged, which are in the working directory, and which files aren't being tracked.
To view the history of your commits, use:
git log
This command displays a chronological list of commits, making it easier to track and reference changes over time.
Undoing Changes
Mistakes are part of the development process. Git provides several commands to undo changes, such as:
- Discarding changes in the working directory with:
git checkout -- filename.py
- Resetting commits with:
This command undoes the last commit but keeps your changes intact in the staging area.git reset --soft HEAD~1
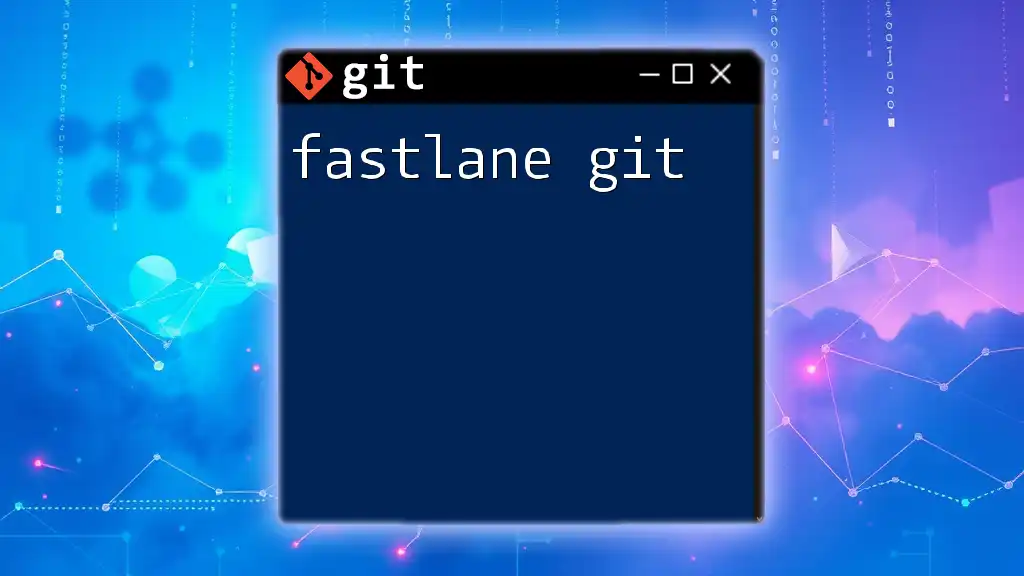
Advanced Git Features
Tagging Releases
Tags in Git serve as a way to mark specific points in your project history as important, typically used for release versions. To tag a release, you would run:
git tag -a v1.0 -m "First release"
This creates an annotated tag with a message, allowing you to easily refer back to this point.
Stashing Changes
If you need to switch branches but aren’t ready to commit your changes, stashing can save your progress temporarily. Use:
git stash
This command will store your changes safely so you can return to them later without making a commit.
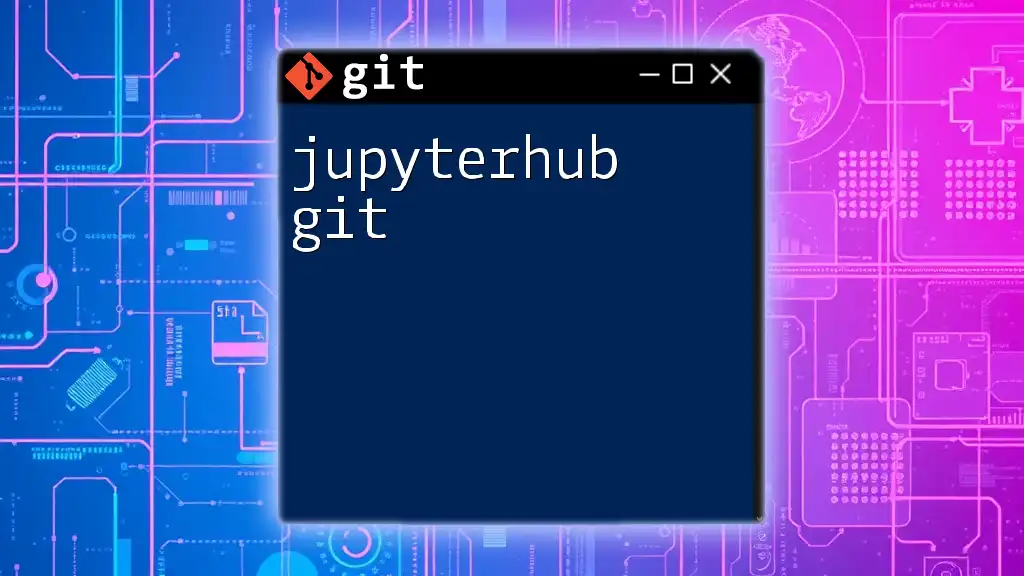
Conclusion
Integrating Python and Git into your development workflow provides a robust framework for version control and collaboration. By following the best practices outlined in this guide, you can effectively manage your Python projects, collaborate with others, and maintain an organized repository. Embracing Git enhances not only your coding efficiency but also the quality of your code, paving the way for smoother development processes.
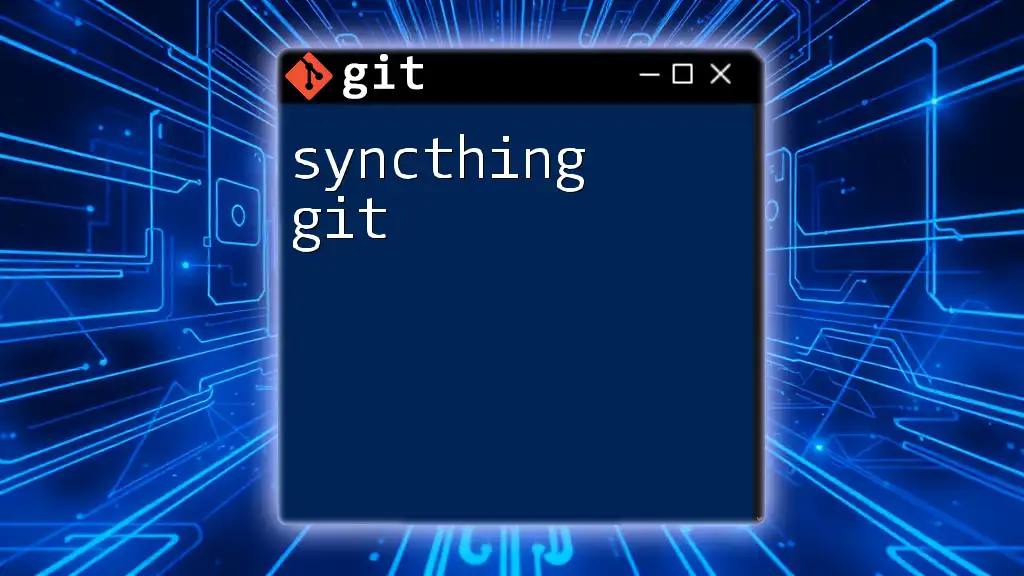
Additional Resources
To deepen your understanding of Git and Python, consider exploring tutorials, books, and online platforms dedicated to these tools. Engaging with community forums can also provide valuable insights and tips from experienced developers.