In PyCharm, you can create a `.gitignore` file to exclude specific files or directories from being tracked by Git, ensuring that unneeded files don't clutter your repository.
Here's a simple code snippet to create a `.gitignore` file that ignores the `*.log` files and the `venv/` directory:
echo "*.log" >> .gitignore
echo "venv/" >> .gitignore
Understanding Git Ignore in PyCharm
What is a .gitignore File?
A .gitignore file is a text file that tells Git which files or directories to ignore in a project. This is crucial for maintaining a clean repository by avoiding the tracking of files that do not contribute to the source code—such as temporary files, log files, or personal IDE configurations.
Why Use .gitignore in PyCharm?
Using a .gitignore file in PyCharm provides several benefits. It helps keep your repository organized by excluding unwanted files that can clutter your version history and potentially reveal sensitive information or large temporary files that don’t need to be versioned. Effectively managing untracked files will lead to a smoother collaboration for teams when sharing and developing code together.
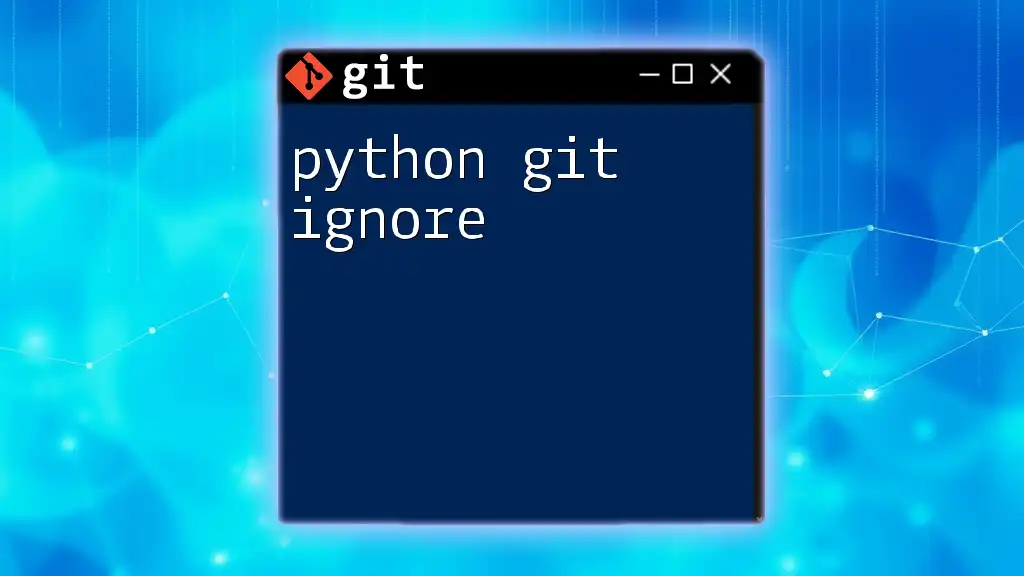
Setting Up a .gitignore File in PyCharm
Creating a .gitignore File
Creating a .gitignore file is straightforward. Here are the steps to create it directly in PyCharm:
- Right-click on your project root folder in the Project view.
- Select New > File and name it .gitignore.
Alternatively, you can create it from the terminal by navigating to your project directory and using the following command:
touch .gitignore
This will create an empty .gitignore file.
Adding a .gitignore File to a PyCharm Project
After creating a .gitignore file, you need to add it to your project. Simply ensure that it's located in your project’s root directory. PyCharm will automatically recognize this file, and you can start adding your ignore patterns right away. You can open the file by double-clicking it and entering the necessary rules as needed.
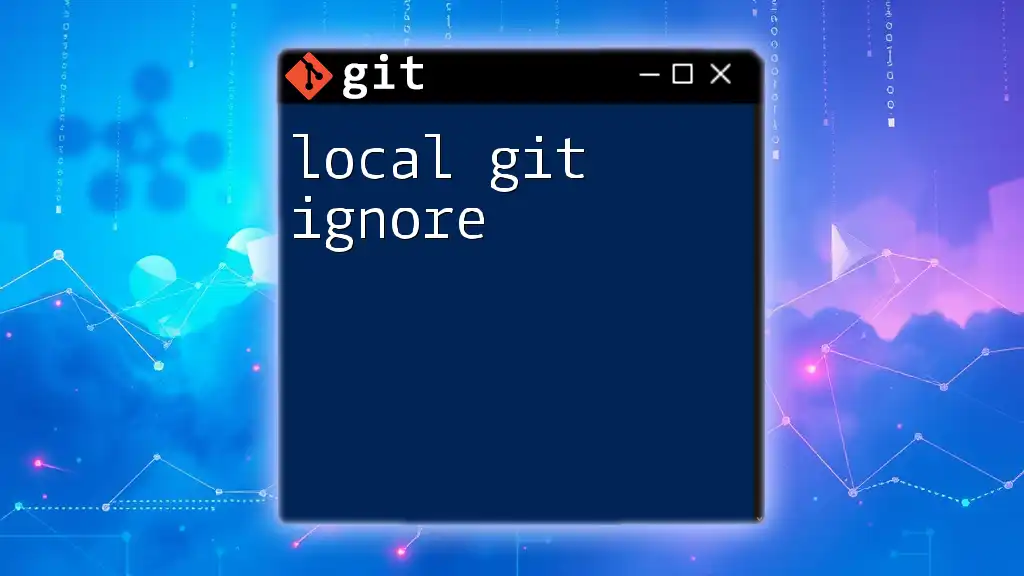
Common Patterns for a .gitignore File
Ignoring Python Bytecode
Python generates bytecode files that aren’t necessary to be included in your repository. These are typically located in the `pycache` directories with a `.pyc` extension. To ignore these files, you can add the following lines to your .gitignore file:
__pycache__/
*.pyc
This ensures that your version control does not track these compiled files.
Ignoring Virtual Environment Files
Virtual environments are essential for managing dependencies in Python projects, but they can take up significant space and are often environment-specific. Therefore, it’s a best practice to ignore them in your repository. To do this, include the following line in your .gitignore file:
venv/
or, for some configurations:
.env/
Ignoring IDE and OS Files
It's common for IDEs and operating systems to create hidden files that aren’t necessary for your project. For PyCharm, you might want to ignore the `.idea/` directory, which contains project-specific settings. Additionally, if you're on a Mac, you might want to ignore `.DS_Store` files. Incorporate these into your .gitignore:
.idea/
.DS_Store
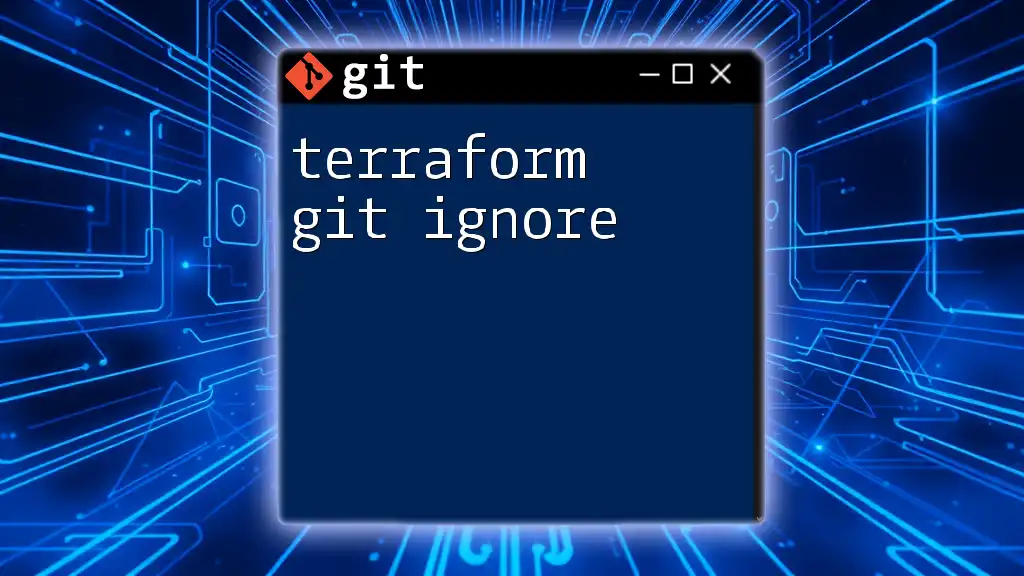
Customizing Your .gitignore File
Adding Custom Ignore Rules
A .gitignore file can accommodate custom ignore rules based on the needs of your project. You can utilize wildcards to ignore groups of files and folders. For instance, to ignore all log files in your project:
*.log
If you want to prevent certain files from being tracked while ignoring others, use the negation pattern. For example, to ignore all `.tmp` files except `important.tmp`, you would write:
*.tmp
!important.tmp
Examples of Specific Rules
There are various scenarios where you might want to customize your .gitignore file. Some useful rules could include ignoring temporary files generated by applications or scripts. Here are a couple of examples you might consider for your .gitignore file:
*.tmp
*.bak
This will disregard temporary and backup files that often clutter your project directory.
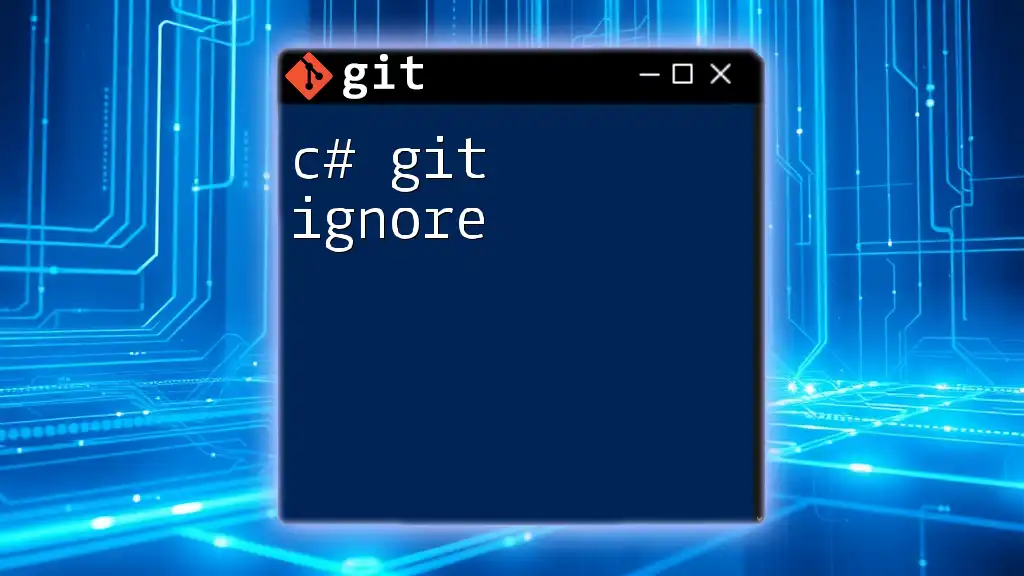
Best Practices for Using .gitignore
Keeping Your .gitignore Organized
For ease of readability, it’s crucial to keep your .gitignore file organized. Commenting lines can help clarify why certain files or directories are being ignored. Group related entries, and use section headers. For example:
# Python settings
__pycache__/
*.pyc
# Virtual environment
venv/
Regularly Updating Your .gitignore
Your project is likely to evolve over time, and so should your .gitignore file. Regularly assess your .gitignore to reflect changes in your project structure or development practices. This will help ensure that you keep your repository clean and free of unnecessary files. Utilize version control tools to maintain an effortless repository and manage contributions effectively.
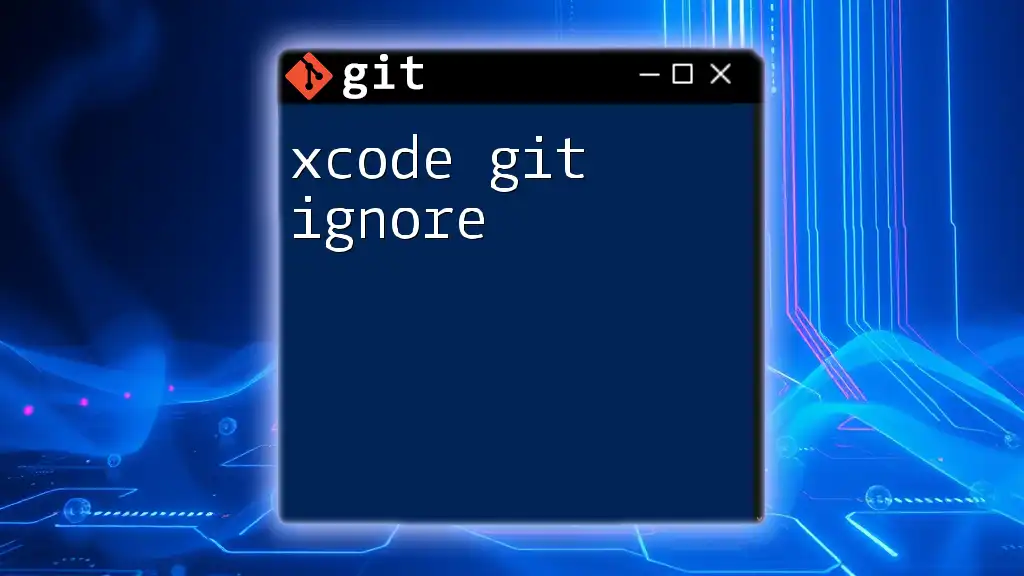
Troubleshooting Common .gitignore Issues
Why Files Are Still Tracked Despite .gitignore
If you find that files you wish to ignore are still being tracked, it's possible that they have already been staged or committed to the repository. To fix this, you will need to clear the Git cache for those files. The following command will do this:
git rm --cached <file-or-directory>
This command will untrack the specified files without deleting them from your local file system.
Using Command Line Tools with .gitignore
To see which files are currently ignored by your .gitignore file, you can use the following command:
git check-ignore -v *
This will provide a list of the files being ignored and the corresponding rules from your .gitignore that caused them to be excluded.
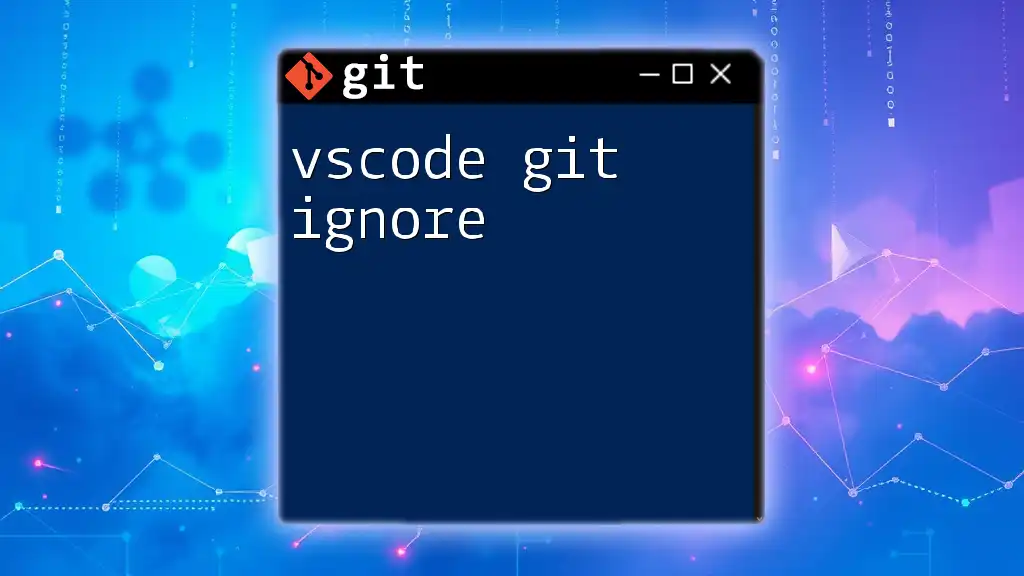
Conclusion
The importance of a well-maintained .gitignore file in a PyCharm project cannot be overstated. It not only streamlines collaboration in teams by filtering out unnecessary data but also keeps your version history clean and manageable. By following the guidelines and tips outlined in this article, you can customize your .gitignore effectively to suit your project needs.
If you want to delve deeper into Git or PyCharm, consider exploring further resources such as the official Git documentation or engaging with community forums dedicated to version control and IDE usage.