In C# projects, a `.gitignore` file is used to specify which files and directories Git should ignore, helping to keep the repository clean from unnecessary files, such as binaries or user-specific settings.
Here's a simple example of a `.gitignore` file for a C# project:
# Ignore build directories
bin/
obj/
# Ignore user-specific files
*.user
*.suo
# Ignore Visual Studio Code settings
.vscode/
Understanding the `.gitignore` File
What is a `.gitignore` file?
A `.gitignore` file is a special file in Git that tells the system which files or directories to ignore when committing changes to a repository. When a file is listed in the `.gitignore`, any changes made to it won't be tracked by Git, making it easier to manage which elements of your codebase are versioned. This is particularly useful for keeping repositories clean and focused on significant files while avoiding clutter from unnecessary elements.
Why Use a `.gitignore` File in C# Projects?
The importance of a C# `.gitignore` cannot be overstated. Ignoring specific files helps to protect confidential information; for example, sensitive API keys or database configurations shouldn't be included in version control to avoid exposing them inadvertently. Additionally, it helps keep your repository clean by only including files relevant to the source code and project structure. This, in turn, ensures fewer conflicts and a smoother collaboration experience between team members who are working on the same project. Keeping the repository focused on essential files enhances its overall maintainability and functionality.
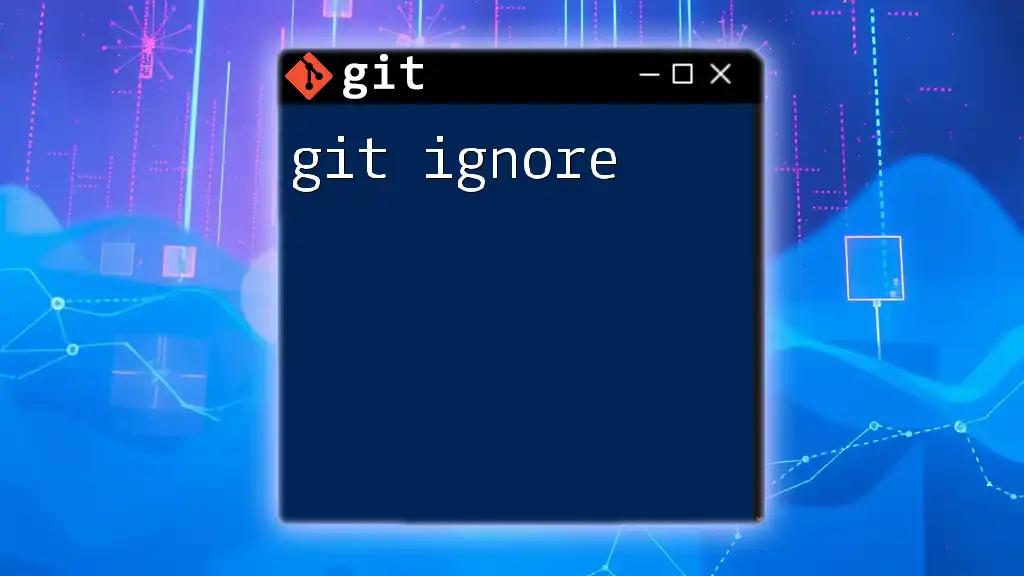
Common File Types to Ignore in C# Projects
Temporary files
C# projects can often generate temporary files during development or execution, such as log files and crash dumps. These files contain ephemeral data that does not need to be included in version control. For example:
*.tmp
*.log
Ignoring these file types helps maintain a clean repository and avoids committing unwanted noise into your version history.
Build and Output files
When you build a C# project, the build process produces output files like binaries and object files that should not be tracked because they can be generated from your source code. Ignoring these files is essential for a cleaner commit history and ensures that no unnecessary files are pushed to your repository. For example, adding the following lines to your `.gitignore` file can effectively ignore common build output directories:
bin/
obj/
User-specific files
Integrated Development Environments (IDEs) such as Visual Studio create user-specific files that contain personal settings and configurations. These files can vary from one user to another and do not need to be shared with the team. Common files to ignore include:
*.suo
*.user
.vs/
Ignoring these files ensures that each team member can maintain their preferred settings without affecting others.
Dependency files
When working with external libraries or packages, it's vital to manage dependencies properly. Ignoring specific dependency files can prevent cluttering the repository with unnecessary data. For instance, it is common to ignore:
packages/
*.nupkg
By monitoring your dependencies through a package manager like NuGet instead, you can keep your repository lightweight and maintainable.
Configurations and Other Files
Certain configuration files should also be excluded from version control, especially if they contain sensitive items or are environment-specific. For example:
appsettings.Development.json
*.config
By ignoring these files, you protect sensitive information while still maintaining collaboration on the overall project structure.
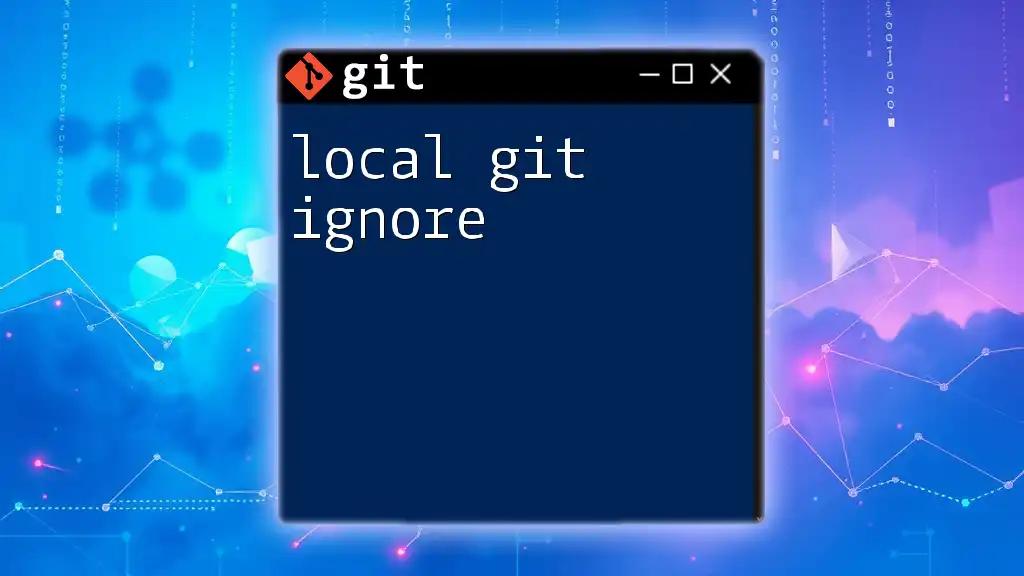
Creating a `.gitignore` File for C# Projects
Step-by-step guide to creating `.gitignore`
-
Open the root of your C# project. Locate your project folder using your preferred file explorer.
-
Create a new file named `.gitignore`. Use the following commands depending on your operating system:
- For macOS/Linux:
touch .gitignore
- For Windows:
echo.> .gitignore
- For macOS/Linux:
-
Edit the `.gitignore` file. Open the file in your favorite text editor, like Visual Studio Code or Notepad++, to add the necessary entries.
Sample `.gitignore` Entry for C# Projects
When configuring your `.gitignore`, a basic structure might look as follows:
# Build results
[Bb]in/
[Oo]bj/
# Visual Studio files
*.suo
*.user
*.userosscache
*.sln.docstates
.vs/
# NuGet packages
packages/
*.nupkg
# Log files
*.log
This simple example encapsulates fundamental entries necessary for a standard C# project.
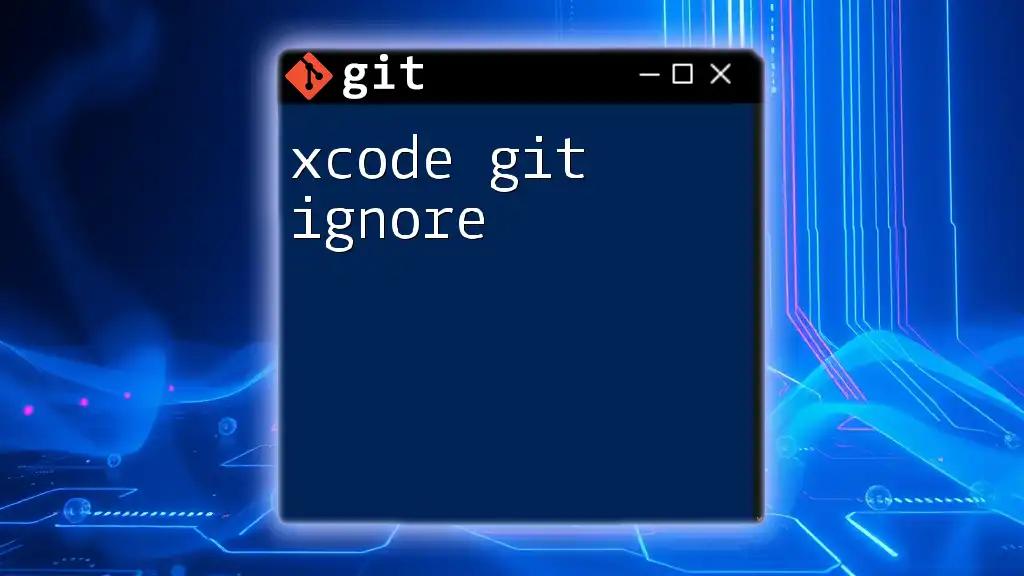
Advanced `.gitignore` Techniques for C# Developers
Comments in `.gitignore`
Adding comments in your `.gitignore` file can assist in maintaining clarity for anyone reading the file. Use the `#` symbol for comments. For example:
# Ignore build folders
[Bb]in/
[Oo]bj/
This practice enhances readability and makes it easier to update the `.gitignore` as project requirements change.
Conditional Ignoring
Another powerful feature of `.gitignore` is its ability to implement conditional entries. For example, you may want to ignore environment-specific config files such as development settings:
# Ignore environment-specific configs
appsettings*.json
This capability allows developers to customize what gets ignored based on the context of their working environment.
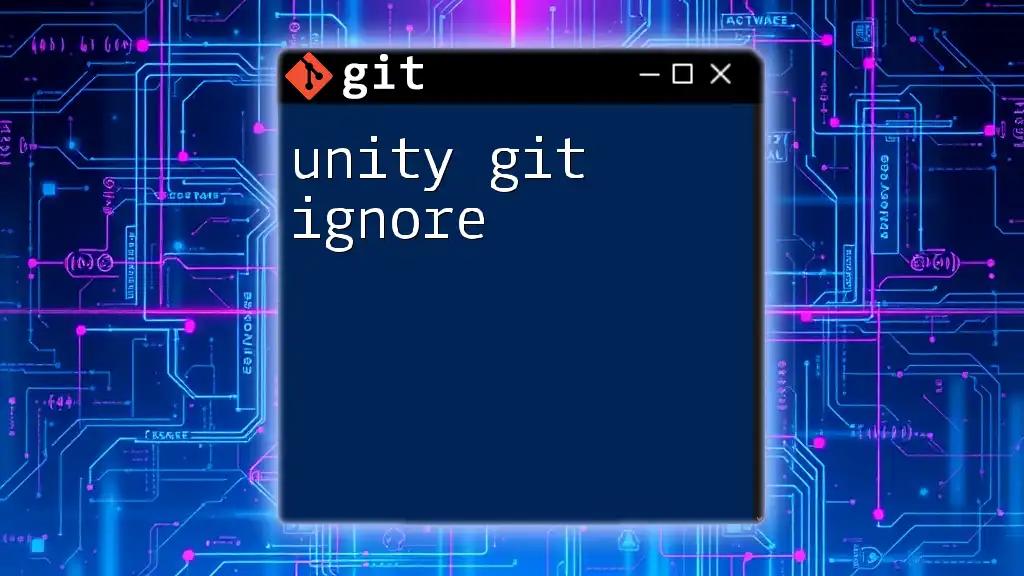
Tips and Best Practices for Using `.gitignore` in C# Projects
Regular Maintenance of `.gitignore`
It’s essential to regularly review and update your `.gitignore` file as your project evolves. New files or directories that may require ignoring can emerge over time, so maintaining this file ensures that your version history remains clean and relevant. For example, closely monitor newly added libraries or configuration changes during development.
Use of Global `.gitignore`
For a more streamlined approach, particularly if you work across multiple Git repositories, consider setting up a global `.gitignore`. This file applies to all Git projects on your machine, which can save time and reduce redundancy. Create a global `.gitignore` with the following command:
git config --global core.excludesfile ~/.gitignore_global
In this file, you can add common entries that you wish to ignore across all your projects.
Resources for `.gitignore` Templates
There are excellent resources availabel online that offer `.gitignore` templates tailored for C# projects. One such valuable source is GitHub’s own `.gitignore` repository, where you can find community-driven templates that can suit your specific needs.
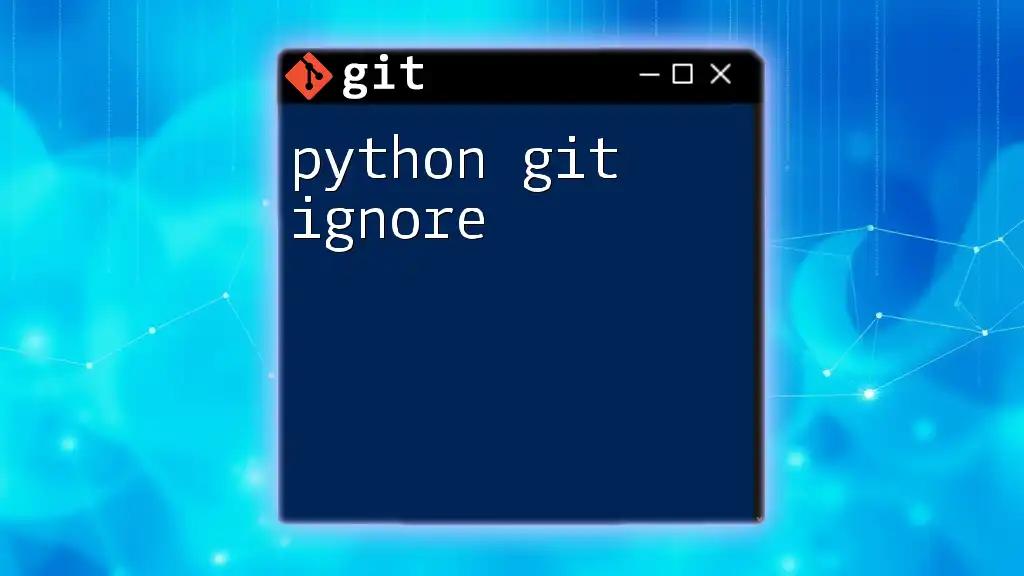
Conclusion
In summary, utilizing a `.gitignore` file in your C# projects is critical for maintaining a clean and manageable version control environment. By focusing only on relevant files and keeping sensitive or unnecessary data out of your repository, you enhance collaboration and improve the overall quality of your codebase. Remember to review your `.gitignore` regularly and consider leveraging global settings to simplify your workflow. Start implementing personalized `.gitignore` files today to ensure your projects remain streamlined and effective!
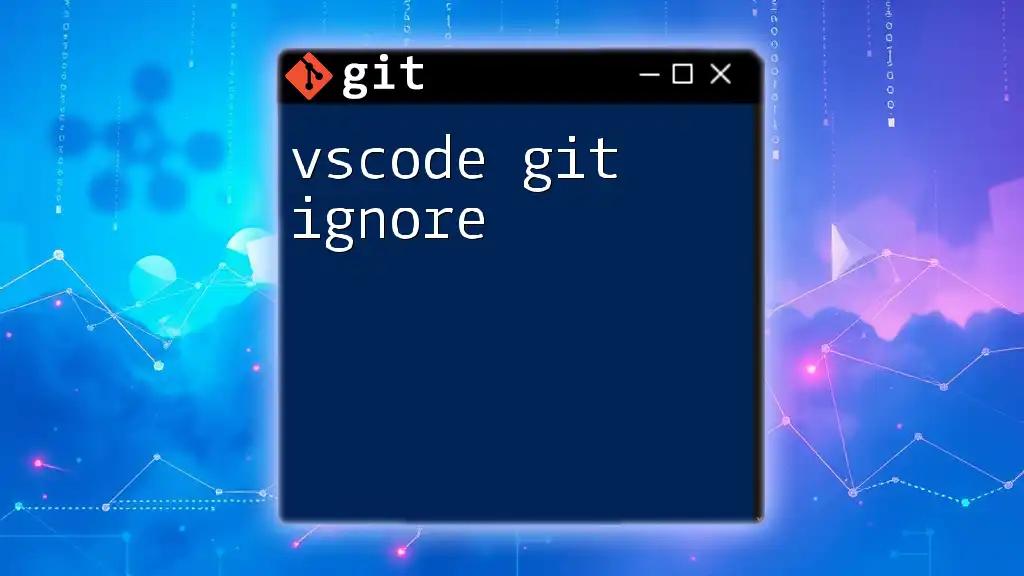
FAQs about C# `.gitignore`
What happens if I commit files that should be ignored?
If you accidentally commit files that should have been ignored, they will still be tracked by Git. You can remove them from tracking by using the `git rm --cached <file>` command, which will untrack the file while keeping it in your directory.
Can I still track a file if I add it to `.gitignore`?
Yes, if a file was already tracked by Git before adding it to `.gitignore`, it will continue being tracked unless you explicitly untrack it.
Where can I find more information about `.gitignore`?
For further learning about `.gitignore`, you can explore the official Git documentation or visit resources like the GitHub Guides, which provide in-depth understandings and examples of using `.gitignore` effectively.