"The git up" typically refers to the command `git pull` which fetches changes from a remote repository and merges them into your current branch.
Here’s the command in a code snippet:
git pull origin main
What is “The Git Up”?
"The Git Up" refers to a streamlined workflow in Git that emphasizes efficient version control practices, allowing developers to collaborate seamlessly on projects. The term has evolved within the developer community to represent the best methodologies for using Git commands effectively. By adopting "The Git Up", teams achieve greater productivity, clarity, and reduced errors in their coding processes.
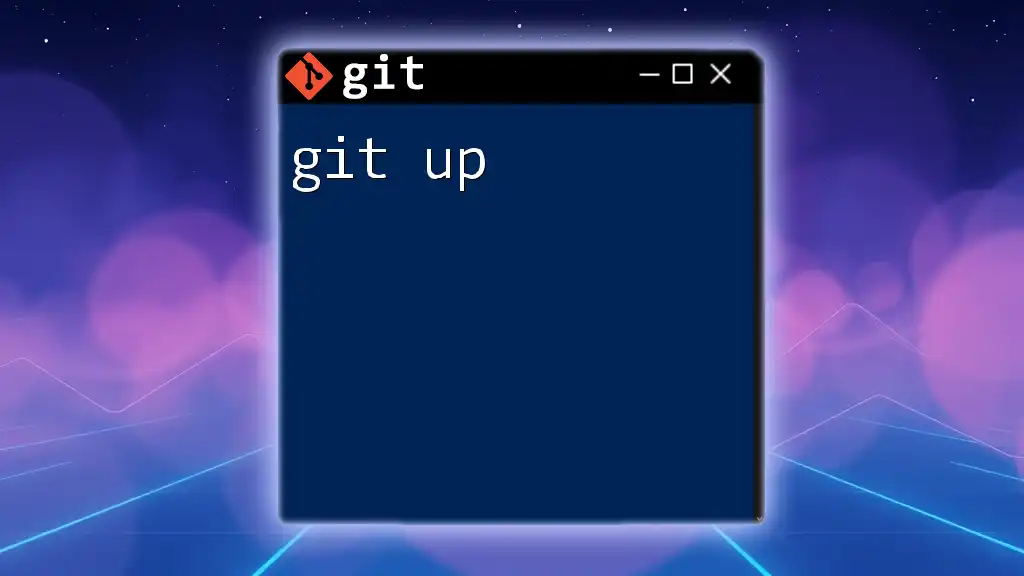
Why Use “The Git Up”?
Using "The Git Up" brings several benefits that enhance both individual and team productivity. It significantly improves collaboration by ensuring that all team members are on the same page and can seamlessly integrate their contributions. With clear practices around branching and merging, developers can work concurrently on features without stepping on each other's toes. This method also reduces errors, as it encourages smaller, more concentrated commits—allowing for easier tracking and reverting when necessary.
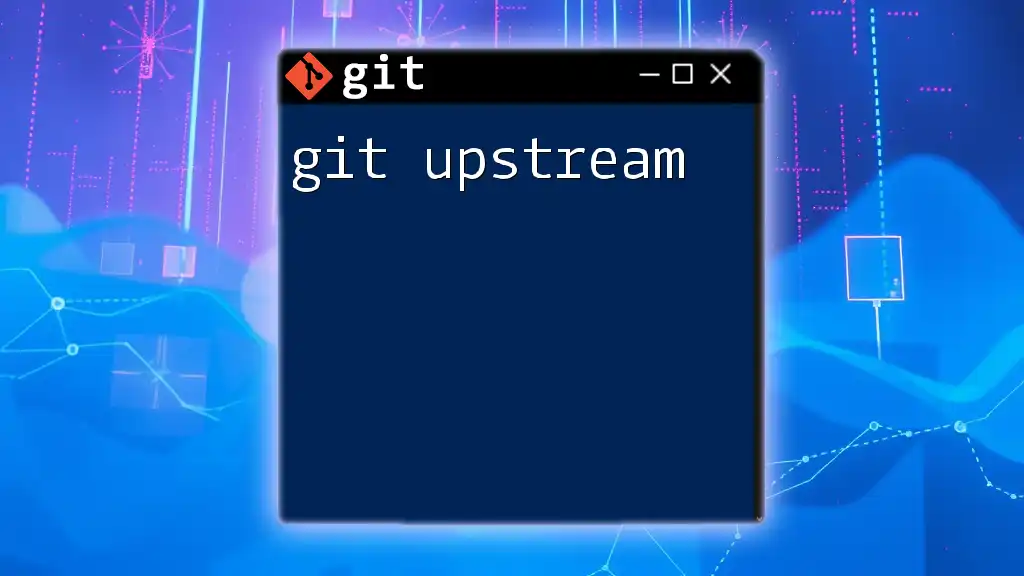
Setting Up Your Environment
Installing Git
To effectively use "The Git Up", the first step is to install Git. Below are installation commands for the major operating systems:
For Windows:
- Download the Git installer from the official Git website.
- Run the installer and follow the prompts to complete the installation.
For macOS:
brew install git
For Linux (Debian/Ubuntu-based):
sudo apt update
sudo apt install git
Configuring Git
After installation, it’s crucial to configure Git with your user information. This ensures that your commits reflect your identity in the project history.
To set your name and email, use the following commands:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Setting these configurations globally applies them to all repositories on your machine.
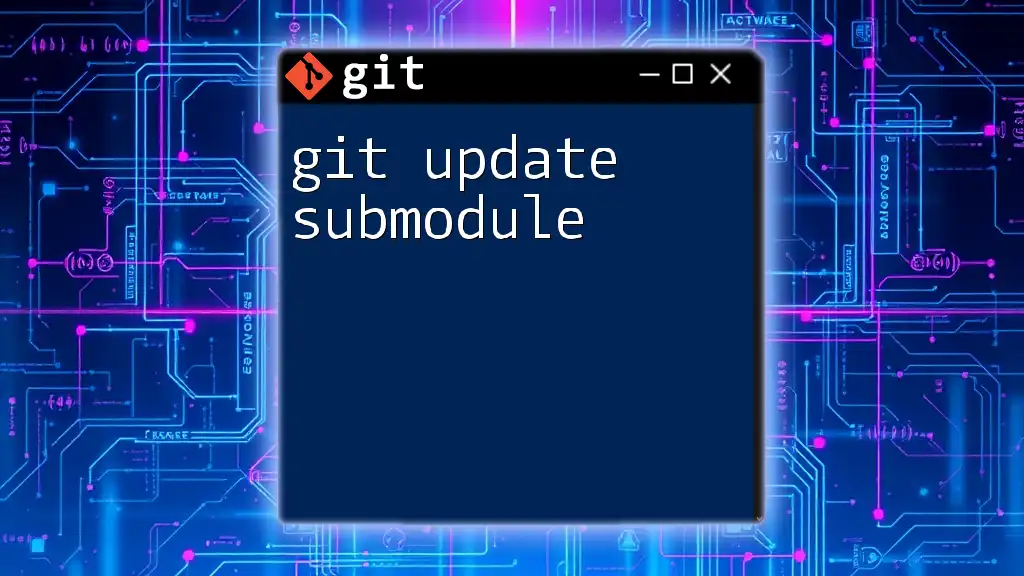
The Mechanics of “The Git Up”
Understanding Branches
Branches are vital in Git as they allow developers to diverge from the main line of development. "The Git Up" promotes the effective use of branches, enabling teams to work on features independently without disrupting the main codebase. Each feature or bug fix is developed in its own branch, streamlining the overall workflow.
Commits Explained
Committing code is a significant part of version control, as it captures the state of your project at specific points in time. It’s essential to write clear and descriptive commit messages that succinctly communicate what changes were made. Good commit messages enhance collaboration and ease the review process.
Example of a Clear Commit Message:
git commit -m "Fix bug in user authentication flow"
On the other hand, vague messages like "Update stuff" should be avoided, as they do not provide useful context.
Rebasing vs. Merging
Both rebasing and merging are methods to integrate changes from one branch into another. Merging is straightforward but can lead to a cluttered project history. In contrast, rebasing offers a cleaner history by moving your entire branch to begin at the tip of the main branch.
When to use:
- Merging is best for larger teams or when you need to maintain context from the feature branches.
- Rebasing is preferable for solo development or smaller teams where you want to keep the history linear.
Example Commands:
git merge branch-name
git rebase branch-name
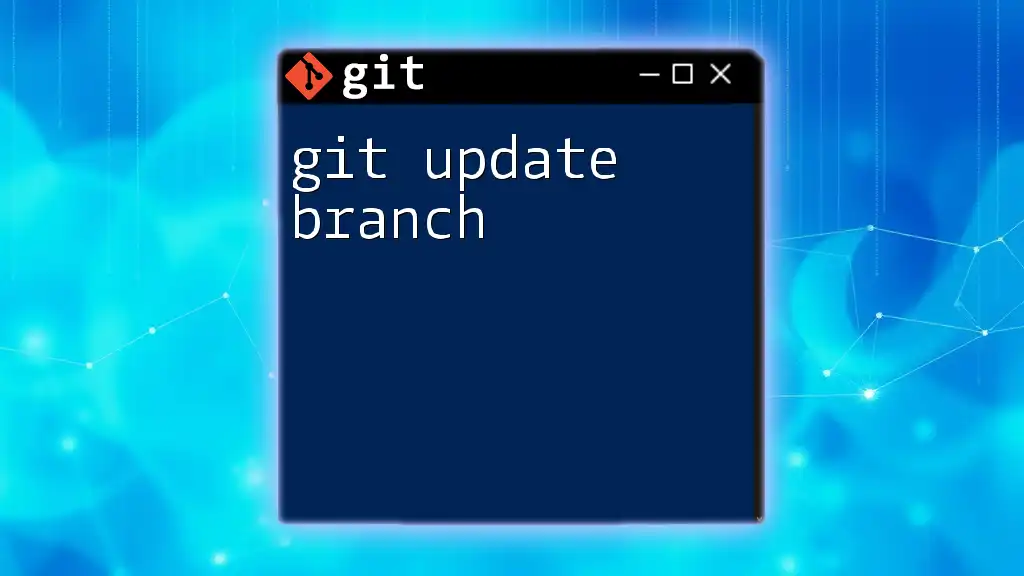
Implementing “The Git Up” in Your Workflow
Create a Branch
Starting the "The Git Up" process involves creating a new branch for your feature or fix. This helps keep the main branch clean and stable:
git checkout -b feature-branch
Make Changes and Stage Your Files
As you work on your changes, you will stage the files that are ready to be committed. This is where you select which modifications you want to include in your next commit.
To stage all modified files, use:
git add .
Committing Changes
After staging your files, it's time to commit your changes. A commit captures your changes in the project history. Always ensure your commit messages are meaningful:
git commit -m "Implement responsive design for mobile view"
Pushing to Remote Repository
Once your local changes are committed, push them to the remote repository to make your updates available to your team:
git push origin feature-branch
Creating a Pull Request
After pushing your branch, the next essential step is to create a pull request (PR). A PR allows team members to review your work before merging it into the main branch. Clear code reviews lead to improved code quality and team collaboration.
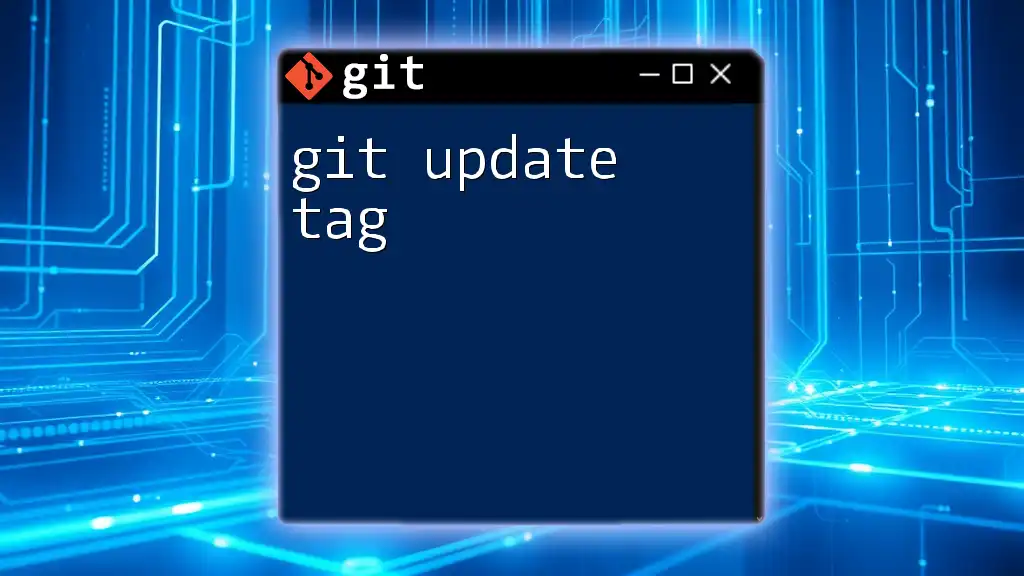
Best Practices for “The Git Up”
- Keep Commits Small: Small, logical commits simplify the code review and history-tracking processes.
- Regularly Sync with Remote: Frequent pulling and pushing of changes ensure that you are always working with the latest codebase.
- Descriptive Branch Names: Effective branch names summarize their purpose and help team members understand the context without additional explanations.
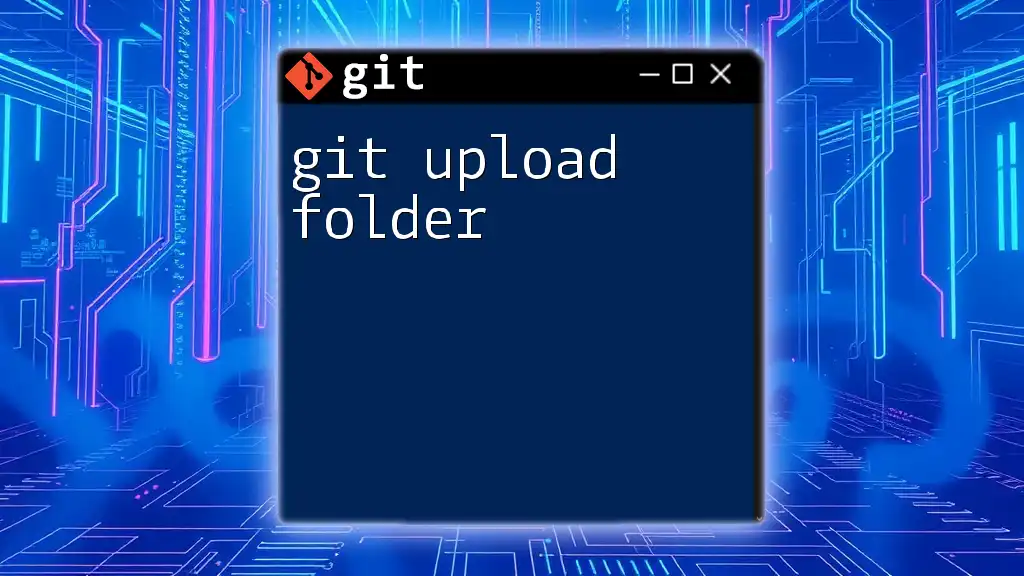
Common Mistakes to Avoid
A crucial part of mastering "The Git Up" is being aware of common pitfalls. One frequent mistake is merging without pulling changes first. This can lead to conflicts and lost work.
Another typical error is ignoring the .gitignore file, which can result in unwanted files being committed into the repository. Additionally, avoid making large commits, as these can complicate the history and make it difficult to pinpoint the introduction of bugs.
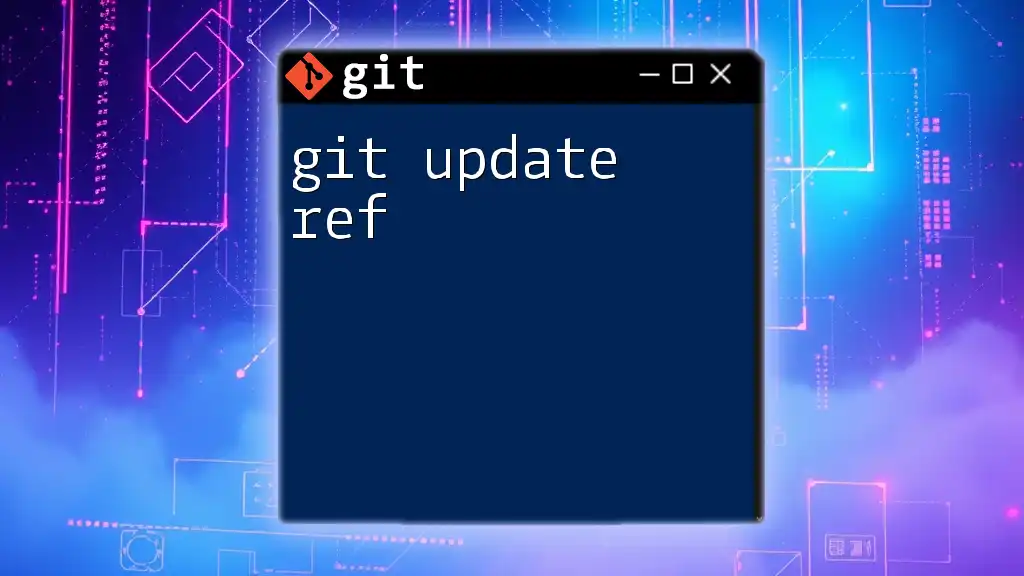
Troubleshooting Common Issues
Resolving Merge Conflicts
Merge conflicts occur when changes to the same lines of code are made in different branches. To resolve conflicts, you’ll typically need to manually edit the files, test them, and then stage the resolved files:
git status
Undoing Mistakes
Mistakes happen even in the best workflows. Git provides commands to help you revert your changes or unstage files. To undo your last commit and all associated changes:
git reset --hard HEAD~1
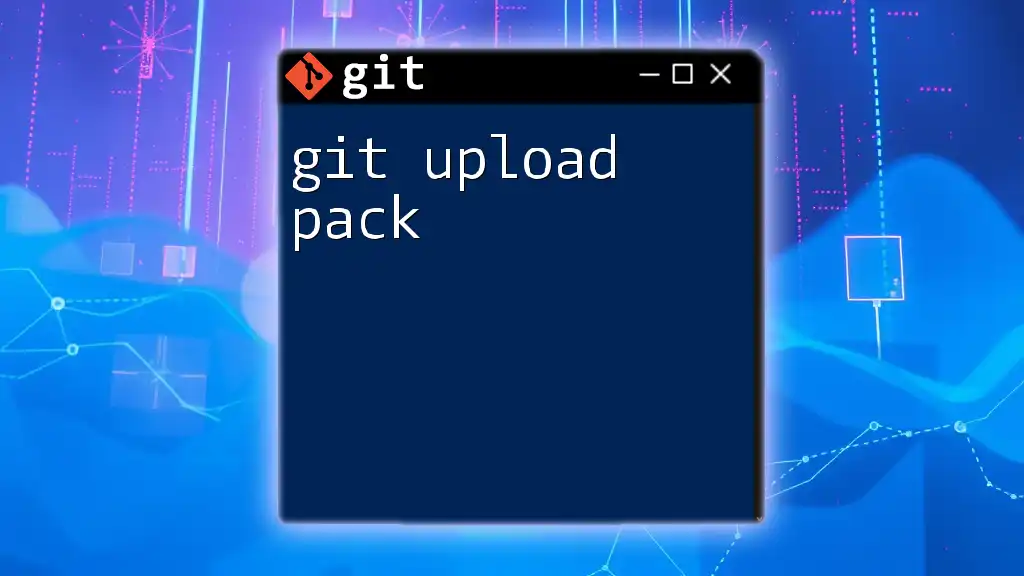
Conclusion
"The Git Up" is an indispensable approach to enhancing your workflow with Git. By adopting this strategy, you will improve collaboration, reduce errors, and streamline your development process. Implementing the practices discussed in this guide will not only simplify your development journey but also empower your team dynamics. Embrace "The Git Up" and watch your productivity soar!
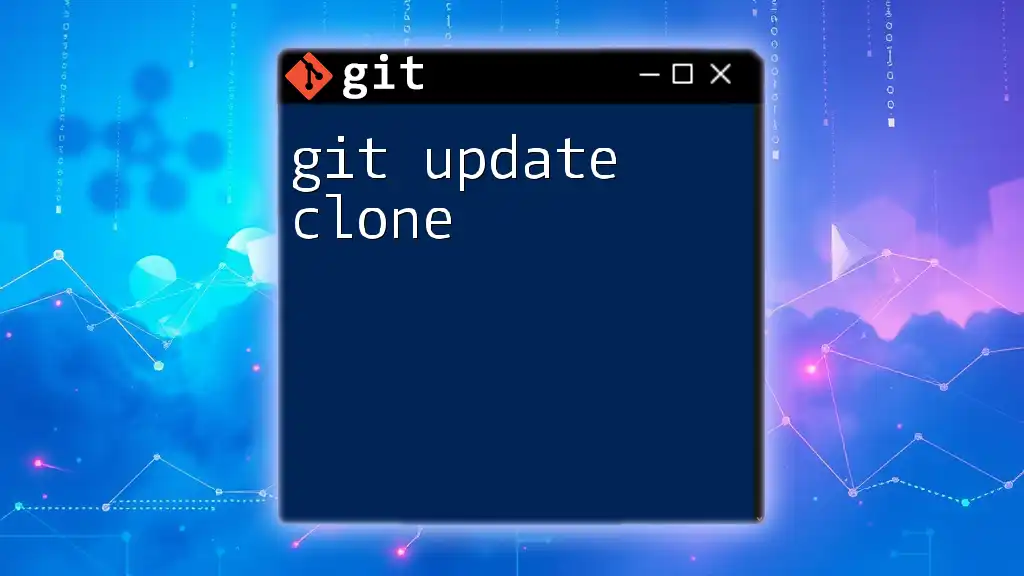
Additional Resources
For further learning, refer to the official Git documentation and explore various online courses tailored to both beginners and advanced users. Engaging with community forums can also provide valuable insights and support as you master Git.
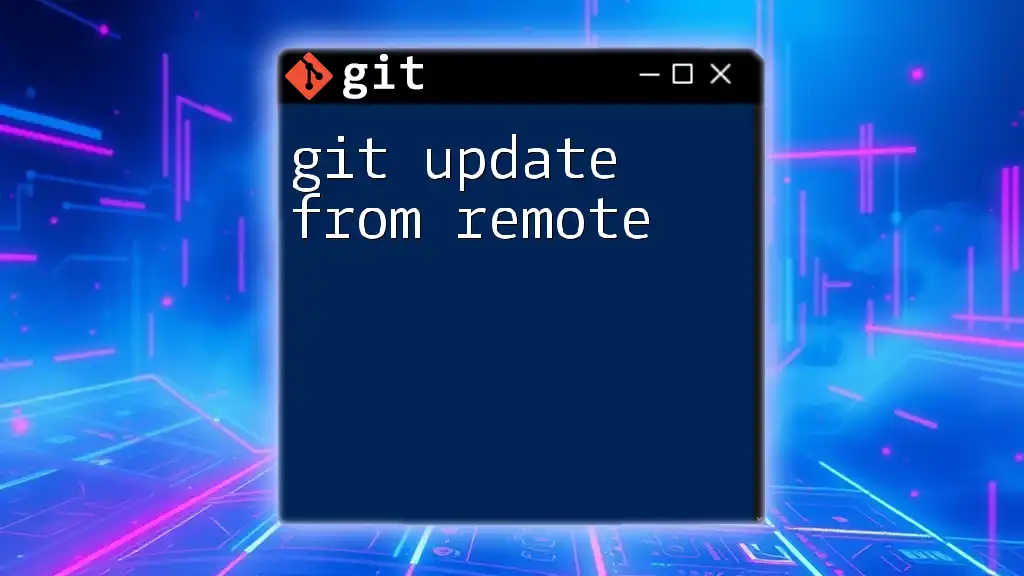
FAQs
This section will delve into common questions surrounding "The Git Up", fortifying your understanding and application of this powerful Git workflow.
By adhering to the guidelines and practices outlined here, you’ll be well on your way to mastering "The Git Up" and enhancing your Git proficiency.